要提高Python下载速度,可以采用多线程、多进程、优化网络请求、使用高效下载库等方法。其中,多线程是一种常见且高效的方式。以下是对多线程方法的详细描述:
多线程方法:在下载大文件或者多个文件时,将下载任务分割成多个小任务,每个小任务由一个线程负责。这样可以利用多线程并行处理,显著提高下载速度。Python的threading
库和concurrent.futures
库都可以实现多线程下载。
以下将详细介绍Python提高下载速度的多种方法:
一、多线程、多进程下载
1、多线程下载
多线程下载是一种通过并行处理来提高下载速度的技术。Python内置的threading
库可以很方便地实现多线程下载。下面是一个使用threading
库实现多线程下载的示例代码:
import threading
import requests
def download_segment(url, start, end, thread_id):
headers = {'Range': f'bytes={start}-{end}'}
response = requests.get(url, headers=headers, stream=True)
filename = f'temp_{thread_id}.part'
with open(filename, 'wb') as f:
f.write(response.content)
print(f'Thread {thread_id} finished downloading')
def multi_thread_download(url, num_threads):
response = requests.head(url)
file_size = int(response.headers['Content-Length'])
segment_size = file_size // num_threads
threads = []
for i in range(num_threads):
start = i * segment_size
end = (i + 1) * segment_size - 1 if i < num_threads - 1 else file_size - 1
thread = threading.Thread(target=download_segment, args=(url, start, end, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
with open('output_file', 'wb') as f:
for i in range(num_threads):
with open(f'temp_{i}.part', 'rb') as part_file:
f.write(part_file.read())
url = 'https://example.com/largefile.zip'
multi_thread_download(url, 4)
2、多进程下载
多进程下载是另一种并行处理技术,适用于多核处理器。Python的multiprocessing
库支持多进程下载。以下是一个示例代码:
import multiprocessing
import requests
def download_segment(url, start, end, queue):
headers = {'Range': f'bytes={start}-{end}'}
response = requests.get(url, headers=headers, stream=True)
queue.put(response.content)
def multi_process_download(url, num_processes):
response = requests.head(url)
file_size = int(response.headers['Content-Length'])
segment_size = file_size // num_processes
processes = []
queue = multiprocessing.Queue()
for i in range(num_processes):
start = i * segment_size
end = (i + 1) * segment_size - 1 if i < num_processes - 1 else file_size - 1
process = multiprocessing.Process(target=download_segment, args=(url, start, end, queue))
processes.append(process)
process.start()
for process in processes:
process.join()
with open('output_file', 'wb') as f:
while not queue.empty():
f.write(queue.get())
url = 'https://example.com/largefile.zip'
multi_process_download(url, 4)
二、优化网络请求
1、使用合适的请求库
选择高效的请求库可以显著提高下载速度。Python的requests
库非常易用,但在某些场景下,aiohttp
等异步HTTP库可能会表现更好。以下是使用aiohttp
进行异步下载的示例:
import aiohttp
import asyncio
async def download_file(url, session):
async with session.get(url) as response:
with open('output_file', 'wb') as f:
while chunk := await response.content.read(1024):
f.write(chunk)
async def main(url):
async with aiohttp.ClientSession() as session:
await download_file(url, session)
url = 'https://example.com/largefile.zip'
asyncio.run(main(url))
2、使用合适的超时和重试策略
在网络状况不佳的情况下,设置合适的超时和重试策略可以提高下载的稳定性和速度。以下是一个示例:
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
def download_file_with_retry(url):
session = requests.Session()
retries = Retry(total=5, backoff_factor=0.1, status_forcelist=[500, 502, 503, 504])
session.mount('http://', HTTPAdapter(max_retries=retries))
session.mount('https://', HTTPAdapter(max_retries=retries))
response = session.get(url, timeout=10)
with open('output_file', 'wb') as f:
f.write(response.content)
url = 'https://example.com/largefile.zip'
download_file_with_retry(url)
三、使用高效下载库
1、使用aria2
aria2
是一个轻量级的多协议、多源命令行下载工具,支持HTTP、FTP、BitTorrent等协议。可以通过Python的subprocess
库调用aria2
来实现高效下载。以下是一个示例:
import subprocess
def download_with_aria2(url, output_file):
subprocess.run(['aria2c', '-x', '16', '-s', '16', '-o', output_file, url])
url = 'https://example.com/largefile.zip'
output_file = 'output_file.zip'
download_with_aria2(url, output_file)
2、使用pySmartDL
pySmartDL
是一个智能下载库,支持断点续传、多线程下载等功能。以下是一个使用pySmartDL
的示例:
from pySmartDL import SmartDL
def download_with_pySmartDL(url, output_file):
obj = SmartDL(url, output_file)
obj.start()
print(f'Download completed: {obj.get_dl_path()}')
url = 'https://example.com/largefile.zip'
output_file = 'output_file.zip'
download_with_pySmartDL(url, output_file)
四、优化文件分块策略
1、动态调整分块大小
动态调整分块大小可以提高下载效率。可以根据网络速度和文件大小动态调整分块的大小,以最大化下载速度。以下是一个示例:
import requests
def download_with_dynamic_chunks(url, chunk_size):
response = requests.get(url, stream=True)
with open('output_file', 'wb') as f:
for chunk in response.iter_content(chunk_size=chunk_size):
if chunk:
f.write(chunk)
url = 'https://example.com/largefile.zip'
initial_chunk_size = 1024 * 1024 # 1MB
download_with_dynamic_chunks(url, initial_chunk_size)
2、基于网络状况调整分块大小
可以根据实时网络状况调整分块大小,以提高下载速度。以下是一个示例:
import requests
import time
def get_network_speed():
# 这里可以使用实际的网络速度测试方法
return 10 * 1024 * 1024 # 假设网络速度为10MB/s
def download_with_adaptive_chunks(url):
chunk_size = 1024 * 1024 # 初始分块大小为1MB
response = requests.get(url, stream=True)
with open('output_file', 'wb') as f:
for chunk in response.iter_content(chunk_size=chunk_size):
if chunk:
f.write(chunk)
# 动态调整分块大小
network_speed = get_network_speed()
chunk_size = min(chunk_size * 2, network_speed)
url = 'https://example.com/largefile.zip'
download_with_adaptive_chunks(url)
五、使用下载加速器
1、使用CDN加速
内容分发网络(CDN)可以显著提高下载速度,尤其是在地理位置较远的情况下。CDN会将文件缓存到多个地理位置,从而减少下载延迟。可以选择使用CDN服务提供商,如Cloudflare、Akamai等。
2、使用镜像下载
镜像下载是一种通过多个镜像站点同时下载文件的方法,可以显著提高下载速度。以下是一个示例:
import requests
import concurrent.futures
def download_from_mirror(url, output_file):
response = requests.get(url)
with open(output_file, 'wb') as f:
f.write(response.content)
def download_with_mirrors(urls, output_file):
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(download_from_mirror, url, output_file) for url in urls]
for future in concurrent.futures.as_completed(futures):
if future.result():
break
urls = ['https://mirror1.example.com/largefile.zip',
'https://mirror2.example.com/largefile.zip']
output_file = 'output_file.zip'
download_with_mirrors(urls, output_file)
六、优化网络配置
1、使用代理服务器
使用代理服务器可以提高下载速度,特别是在网络状况不佳的情况下。以下是一个示例:
import requests
def download_with_proxy(url, proxy):
proxies = {'http': proxy, 'https': proxy}
response = requests.get(url, proxies=proxies)
with open('output_file', 'wb') as f:
f.write(response.content)
url = 'https://example.com/largefile.zip'
proxy = 'http://proxy.example.com:8080'
download_with_proxy(url, proxy)
2、调整网络参数
调整系统的网络参数可以提高下载速度。例如,可以调整TCP窗口大小等参数。以下是一个示例:
import os
def adjust_network_parameters():
os.system('sysctl -w net.ipv4.tcp_window_scaling=1')
os.system('sysctl -w net.core.wmem_max=12582912')
os.system('sysctl -w net.core.rmem_max=12582912')
adjust_network_parameters()
七、总结
通过多线程、多进程、优化网络请求、使用高效下载库、优化文件分块策略、使用下载加速器和优化网络配置等方法,可以显著提高Python的下载速度。每种方法都有其适用的场景和优缺点,实际应用时可以根据具体需求进行选择和组合使用。
希望以上内容能够帮助你提高Python的下载速度,提升开发和使用体验。
相关问答FAQs:
如何使用Python实现多线程下载以提高速度?
多线程下载是提升下载速度的有效方法。在Python中,可以使用threading
模块或concurrent.futures
库来创建多个线程。同时,可以使用requests
库进行HTTP请求,结合多线程将不同的文件部分并行下载,然后合并它们。这样能显著缩短下载时间。
Python中有哪些库可以帮助我加速下载?
Python有多个库可以帮助加速下载,例如aiohttp
用于异步HTTP请求,能够在处理多个请求时提高效率。requests
库也支持会话保持,能提高下载的速度。对于大文件,aria2
是一个强大的下载工具,可以通过Python接口进行调用,以实现更快的下载速度。
在下载大文件时,如何处理网络中断问题?
下载大文件时,网络中断可能导致下载失败。可以通过实现断点续传来解决这一问题。在Python中,可以使用requests
库的Range
头部来请求特定的字节范围,从而实现从上次中断的地方继续下载。此外,定期保存下载进度也是一种有效的策略,确保在意外中断后可以轻松恢复。
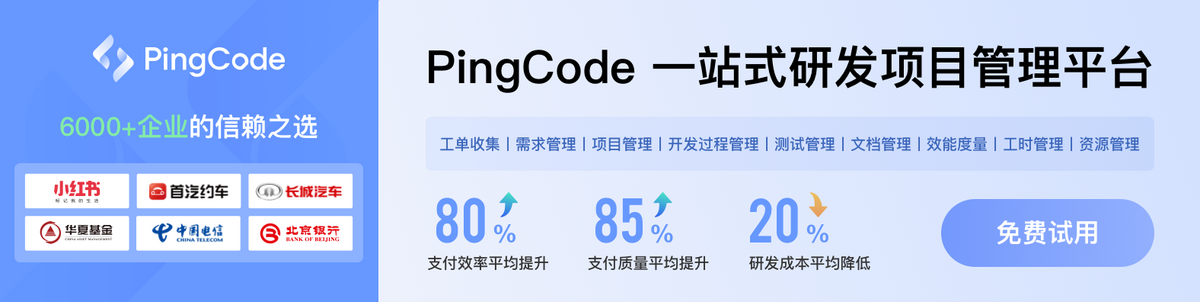