Python比对返回的报文可以使用多种方法,包括字符串比较、正则表达式、JSON解析与比对、XML解析与比对、以及专门的报文比对库等。其中,最常见的方法是字符串比较与JSON解析与比对。字符串比较适用于简单的报文内容比对,JSON解析与比对适用于结构化数据报文。以下将详细介绍JSON解析与比对的方法。
JSON解析与比对的方法:
- 解析报文为JSON对象:使用Python的json模块将报文解析为JSON对象。
- 比对JSON对象:遍历JSON对象,逐一比对各个字段的值。
- 提取差异:记录并输出不相同的字段及其值。
一、解析报文为JSON对象
Python提供了一个内置的json模块,可以方便地进行JSON数据的解析与生成。首先,我们需要将返回的报文解析为JSON对象。
import json
def parse_json_response(response):
try:
return json.loads(response)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
return None
二、比对JSON对象
一旦我们将报文解析为JSON对象,接下来就可以进行比对。比对的方式可以是递归遍历所有的键值对,并记录所有的差异。
def compare_json_objects(json1, json2, path=""):
differences = []
if isinstance(json1, dict) and isinstance(json2, dict):
for key in json1:
if key in json2:
sub_diffs = compare_json_objects(json1[key], json2[key], path + f".{key}")
differences.extend(sub_diffs)
else:
differences.append(f"Key {path}.{key} is missing in the second JSON")
for key in json2:
if key not in json1:
differences.append(f"Key {path}.{key} is missing in the first JSON")
elif isinstance(json1, list) and isinstance(json2, list):
for index, (item1, item2) in enumerate(zip(json1, json2)):
sub_diffs = compare_json_objects(item1, item2, path + f"[{index}]")
differences.extend(sub_diffs)
if len(json1) != len(json2):
differences.append(f"Lists at {path} have different lengths")
else:
if json1 != json2:
differences.append(f"Value at {path} differs: {json1} != {json2}")
return differences
三、提取差异
最后,将所有的差异记录并输出。
def main():
response1 = '{"name": "John", "age": 30, "city": "New York"}'
response2 = '{"name": "John", "age": 31, "city": "New York", "country": "USA"}'
json1 = parse_json_response(response1)
json2 = parse_json_response(response2)
if json1 and json2:
differences = compare_json_objects(json1, json2)
if differences:
print("Differences found:")
for diff in differences:
print(diff)
else:
print("No differences found")
if __name__ == "__main__":
main()
四、字符串比较
字符串比较是最简单的报文比对方法,适用于简单报文内容比对。可以直接使用Python的字符串操作函数进行比较,例如==
操作符、split
函数、以及re
正则表达式模块。
字符串比较示例
response1 = "name=John&age=30&city=New York"
response2 = "name=John&age=31&city=New York"
if response1 == response2:
print("The responses are identical")
else:
print("The responses are different")
使用正则表达式进行字符串比对
正则表达式可以用来匹配复杂的字符串模式,从而进行更细粒度的报文内容比对。
import re
pattern = r"name=(\w+)&age=(\d+)&city=([\w\s]+)"
match1 = re.match(pattern, response1)
match2 = re.match(pattern, response2)
if match1 and match2:
if match1.groups() == match2.groups():
print("The responses match the pattern and are identical")
else:
print("The responses match the pattern but are different")
else:
print("The responses do not match the pattern")
五、XML解析与比对
对于XML格式的报文,可以使用Python的xml.etree.ElementTree模块进行解析与比对。
解析XML报文
import xml.etree.ElementTree as ET
def parse_xml_response(response):
try:
return ET.fromstring(response)
except ET.ParseError as e:
print(f"Error parsing XML: {e}")
return None
比对XML节点
类似于JSON比对,可以递归遍历XML节点,并记录所有的差异。
def compare_xml_elements(elem1, elem2, path="/"):
differences = []
if elem1.tag != elem2.tag:
differences.append(f"Different tags at {path}: {elem1.tag} != {elem2.tag}")
if elem1.text != elem2.text:
differences.append(f"Different text at {path}: {elem1.text} != {elem2.text}")
if elem1.attrib != elem2.attrib:
differences.append(f"Different attributes at {path}: {elem1.attrib} != {elem2.attrib}")
children1 = list(elem1)
children2 = list(elem2)
for index, (child1, child2) in enumerate(zip(children1, children2)):
sub_diffs = compare_xml_elements(child1, child2, path + f"{elem1.tag}[{index}]/")
differences.extend(sub_diffs)
if len(children1) != len(children2):
differences.append(f"Different number of children at {path}: {len(children1)} != {len(children2)}")
return differences
提取差异
def main():
response1 = "<person><name>John</name><age>30</age><city>New York</city></person>"
response2 = "<person><name>John</name><age>31</age><city>New York</city><country>USA</country></person>"
xml1 = parse_xml_response(response1)
xml2 = parse_xml_response(response2)
if xml1 and xml2:
differences = compare_xml_elements(xml1, xml2)
if differences:
print("Differences found:")
for diff in differences:
print(diff)
else:
print("No differences found")
if __name__ == "__main__":
main()
六、专门的报文比对库
Python社区提供了一些专门用于报文比对的库,例如DeepDiff、JsonDiff、xmltodict等。这些库提供了更高级的功能,可以简化报文比对的过程。
使用DeepDiff进行JSON比对
DeepDiff是一个功能强大的库,用于比较复杂的Python对象,包括JSON数据。
from deepdiff import DeepDiff
response1 = '{"name": "John", "age": 30, "city": "New York"}'
response2 = '{"name": "John", "age": 31, "city": "New York", "country": "USA"}'
json1 = json.loads(response1)
json2 = json.loads(response2)
diff = DeepDiff(json1, json2)
if diff:
print("Differences found:")
print(diff)
else:
print("No differences found")
使用xmltodict进行XML比对
xmltodict是一个将XML数据转换为Python字典的库,可以结合DeepDiff进行XML比对。
import xmltodict
from deepdiff import DeepDiff
response1 = "<person><name>John</name><age>30</age><city>New York</city></person>"
response2 = "<person><name>John</name><age>31</age><city>New York</city><country>USA</country></person>"
xml1 = xmltodict.parse(response1)
xml2 = xmltodict.parse(response2)
diff = DeepDiff(xml1, xml2)
if diff:
print("Differences found:")
print(diff)
else:
print("No differences found")
七、总结
Python提供了多种方法用于比对返回的报文,包括字符串比较、正则表达式、JSON解析与比对、XML解析与比对、以及专门的报文比对库。根据具体的报文格式与比对需求,可以选择合适的方法进行比对。
- 字符串比较:适用于简单的报文内容比对,操作简单,适用范围有限。
- 正则表达式:适用于复杂的字符串模式匹配,可以进行更细粒度的报文内容比对。
- JSON解析与比对:适用于结构化数据报文,通过递归遍历键值对进行比对。
- XML解析与比对:适用于XML格式的报文,通过递归遍历XML节点进行比对。
- 专门的报文比对库:例如DeepDiff、JsonDiff、xmltodict等,提供了更高级的功能,可以简化报文比对的过程。
选择合适的比对方法,可以提高报文比对的效率与准确性。
相关问答FAQs:
如何使用Python比较两个字符串的报文?
在Python中,可以使用==
运算符直接比较两个字符串。如果报文的格式是JSON,使用json
模块中的json.loads()
函数将字符串转换为字典,然后进行字典的比较。此外,difflib
模块也提供了更为详细的差异比较功能,可以获取两个报文之间的具体差异。
在Python中如何处理格式不一致的报文?
如果两个报文的格式不一致,例如一个是JSON格式,另一个是XML格式,可以使用相应的解析库,如json
和xml.etree.ElementTree
,将其解析为Python对象(如字典或列表)。在标准化报文格式后,就可以进行比较。
有什么库可以帮助简化Python中的报文比对过程?
可以考虑使用deepdiff
库,它提供了强大的功能,能够深度比较两个Python对象(包括字典、列表等),并返回详细的差异报告。安装该库后,可以通过简单的函数调用实现复杂的报文比对,节省大量时间和精力。
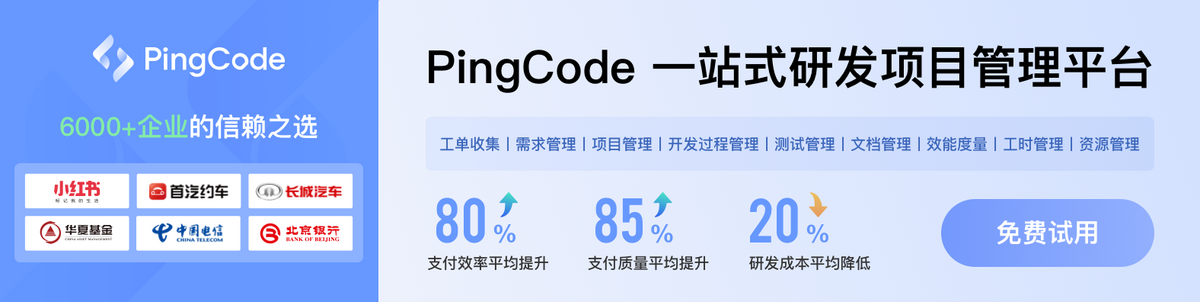