在Python实现粒子特效的方法包括:使用Pygame库、使用Pyglet库、利用OpenGL与Python结合。 其中,使用Pygame库是较为常见和容易实现的一种方法。Pygame是一个跨平台的Python模块,专门用来编写视频游戏,包含了计算机图形和声音的功能。接下来,我们将详细介绍如何使用Pygame库来实现粒子特效。
一、PYGAME库简介
Pygame是一个免费的跨平台Python模块,专门用来编写视频游戏。它包含了计算机图形和声音的功能,适合用来制作简单的2D游戏和图形应用程序。Pygame基于SDL(Simple DirectMedia Layer)库,因此可以在多个平台上运行。
1、安装Pygame
首先,我们需要安装Pygame库。可以使用pip命令进行安装:
pip install pygame
2、Pygame基本结构
Pygame程序的基本结构包括初始化Pygame模块、创建游戏窗口、处理事件、更新游戏状态和渲染图形。以下是一个简单的Pygame程序框架:
import pygame
import sys
初始化Pygame
pygame.init()
创建游戏窗口
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Particle Effect Example")
主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 更新游戏状态
# 渲染图形
screen.fill((0, 0, 0)) # 清屏
pygame.display.flip() # 刷新屏幕
二、创建粒子类
为了实现粒子特效,我们需要定义一个粒子类。粒子类包含粒子的属性,如位置、速度、颜色和生命周期。以下是一个简单的粒子类:
import random
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = random.uniform(-1, 1)
self.vy = random.uniform(-1, 1)
self.color = (255, 255, 255)
self.lifetime = 100
def update(self):
self.x += self.vx
self.y += self.vy
self.lifetime -= 1
def is_alive(self):
return self.lifetime > 0
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), 2)
在这个粒子类中,我们定义了粒子的初始位置、速度、颜色和生命周期。update
方法用于更新粒子的状态,is_alive
方法用于判断粒子是否存活,draw
方法用于在屏幕上绘制粒子。
三、生成和更新粒子
在主循环中,我们需要生成和更新粒子,并在屏幕上绘制它们。以下是一个简单的粒子系统实现:
import pygame
import sys
import random
初始化Pygame
pygame.init()
创建游戏窗口
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Particle Effect Example")
粒子列表
particles = []
主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 生成新的粒子
for _ in range(5):
particles.append(Particle(random.uniform(0, 800), random.uniform(0, 600)))
# 更新粒子状态
for particle in particles:
particle.update()
# 移除死亡的粒子
particles = [particle for particle in particles if particle.is_alive()]
# 渲染图形
screen.fill((0, 0, 0)) # 清屏
for particle in particles:
particle.draw(screen)
pygame.display.flip() # 刷新屏幕
在这个例子中,我们在每一帧生成一些新的粒子,并更新所有粒子的状态,移除已经死亡的粒子,最后在屏幕上绘制所有存活的粒子。
四、粒子特效的改进
我们可以对粒子特效进行一些改进,以实现更复杂和有趣的效果。例如,我们可以调整粒子的颜色、形状和运动轨迹,或者添加一些交互效果。
1、调整粒子颜色
我们可以根据粒子的生命周期来调整粒子的颜色。例如,粒子在生命周期的开始时是白色,随着生命周期的结束逐渐变为红色:
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = random.uniform(-1, 1)
self.vy = random.uniform(-1, 1)
self.lifetime = 100
def update(self):
self.x += self.vx
self.y += self.vy
self.lifetime -= 1
def is_alive(self):
return self.lifetime > 0
def draw(self, screen):
color = (255, int(255 * (self.lifetime / 100)), int(255 * (self.lifetime / 100)))
pygame.draw.circle(screen, color, (int(self.x), int(self.y)), 2)
2、调整粒子形状
我们可以使用不同的形状来绘制粒子,例如使用方形或三角形:
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = random.uniform(-1, 1)
self.vy = random.uniform(-1, 1)
self.lifetime = 100
def update(self):
self.x += self.vx
self.y += self.vy
self.lifetime -= 1
def is_alive(self):
return self.lifetime > 0
def draw(self, screen):
color = (255, int(255 * (self.lifetime / 100)), int(255 * (self.lifetime / 100)))
pygame.draw.rect(screen, color, (int(self.x), int(self.y), 4, 4))
3、调整粒子运动轨迹
我们可以调整粒子的运动轨迹,例如模拟重力效果,使粒子逐渐向下移动:
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = random.uniform(-1, 1)
self.vy = random.uniform(-1, 1)
self.lifetime = 100
def update(self):
self.vy += 0.1 # 模拟重力
self.x += self.vx
self.y += self.vy
self.lifetime -= 1
def is_alive(self):
return self.lifetime > 0
def draw(self, screen):
color = (255, int(255 * (self.lifetime / 100)), int(255 * (self.lifetime / 100)))
pygame.draw.circle(screen, color, (int(self.x), int(self.y)), 2)
五、添加交互效果
为了使粒子特效更加有趣,我们可以添加一些交互效果。例如,粒子在鼠标点击位置生成,或者粒子在靠近鼠标时会受到吸引力的影响。
1、在鼠标点击位置生成粒子
我们可以在主循环中检测鼠标点击事件,并在点击位置生成粒子:
import pygame
import sys
import random
初始化Pygame
pygame.init()
创建游戏窗口
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Particle Effect Example")
粒子列表
particles = []
主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
for _ in range(50):
particles.append(Particle(x, y))
# 更新粒子状态
for particle in particles:
particle.update()
# 移除死亡的粒子
particles = [particle for particle in particles if particle.is_alive()]
# 渲染图形
screen.fill((0, 0, 0)) # 清屏
for particle in particles:
particle.draw(screen)
pygame.display.flip() # 刷新屏幕
在这个例子中,我们在鼠标点击事件中生成一批新的粒子。
2、粒子靠近鼠标时受到吸引力的影响
我们可以在粒子更新时计算粒子与鼠标位置的距离,并根据距离调整粒子的速度,使粒子受到鼠标的吸引力:
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.vx = random.uniform(-1, 1)
self.vy = random.uniform(-1, 1)
self.lifetime = 100
def update(self, mouse_x, mouse_y):
dx = mouse_x - self.x
dy = mouse_y - self.y
distance = (dx<strong>2 + dy</strong>2)0.5
if distance < 100:
self.vx += dx / distance * 0.1
self.vy += dy / distance * 0.1
self.x += self.vx
self.y += self.vy
self.lifetime -= 1
def is_alive(self):
return self.lifetime > 0
def draw(self, screen):
color = (255, int(255 * (self.lifetime / 100)), int(255 * (self.lifetime / 100)))
pygame.draw.circle(screen, color, (int(self.x), int(self.y)), 2)
在主循环中,我们需要传递鼠标位置给粒子的更新方法:
import pygame
import sys
import random
初始化Pygame
pygame.init()
创建游戏窗口
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Particle Effect Example")
粒子列表
particles = []
主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
for _ in range(50):
particles.append(Particle(x, y))
mouse_x, mouse_y = pygame.mouse.get_pos()
# 更新粒子状态
for particle in particles:
particle.update(mouse_x, mouse_y)
# 移除死亡的粒子
particles = [particle for particle in particles if particle.is_alive()]
# 渲染图形
screen.fill((0, 0, 0)) # 清屏
for particle in particles:
particle.draw(screen)
pygame.display.flip() # 刷新屏幕
通过这些改进,我们可以实现更加复杂和有趣的粒子特效。
六、总结
通过本文的介绍,我们学习了如何使用Python和Pygame库实现粒子特效。我们从Pygame的基本结构开始,定义了一个简单的粒子类,然后生成和更新粒子,并在屏幕上绘制它们。我们还对粒子特效进行了改进,调整了粒子的颜色、形状和运动轨迹,并添加了一些交互效果。通过这些步骤,我们可以实现各种有趣的粒子特效,为我们的游戏和图形应用程序增添更多的视觉效果。希望这些内容对你有所帮助,并能够激发你进一步探索和创造更多精彩的粒子特效。
相关问答FAQs:
在Python中实现粒子特效的基本步骤是什么?
在Python中实现粒子特效通常涉及几个关键步骤:首先,选择一个合适的图形库,如Pygame或Panda3D。这些库提供了强大的功能来创建和管理图形效果。接下来,定义粒子的属性,包括位置、速度、生命周期和颜色等。然后,创建一个粒子生成器,它能够根据设定的规则生成粒子。最后,通过更新粒子的位置和状态,以及在每一帧中绘制粒子,来实现动态的粒子效果。
有哪些常用的Python库可以用来创建粒子特效?
有几个流行的Python库可以用来创建粒子特效。Pygame是一个简单且易于使用的库,适合初学者进行2D游戏开发。Panda3D是一个更为复杂的引擎,适合需要3D特效的项目。其他库如Arcade和PyOpenGL也可以用于实现粒子系统,提供更高级的图形处理能力。
粒子特效在游戏开发中的应用有哪些?
粒子特效在游戏开发中应用广泛,能够增强游戏的视觉效果。例如,火焰、烟雾、雨雪等自然现象通常通过粒子系统来实现。它们可以为游戏场景增添生动的动态元素,使玩家的体验更加沉浸。此外,粒子特效还可以用于表现游戏中的特殊事件,如技能施放、角色受伤等,提升游戏的整体表现力和趣味性。
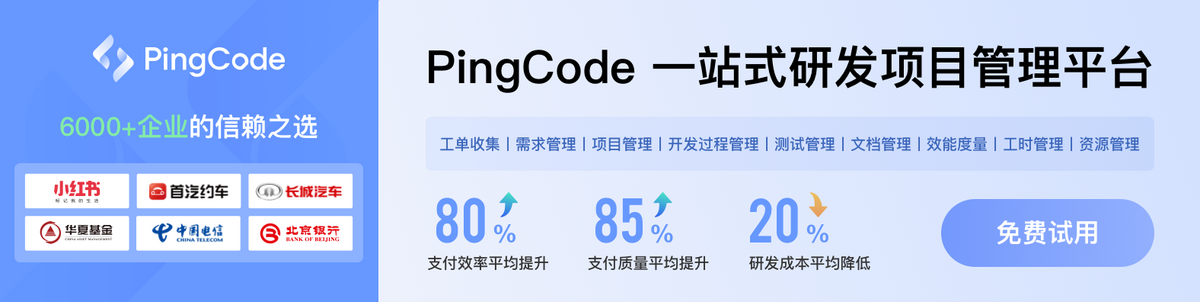