利用Python采集高考数据的方式主要包括使用网络爬虫、调用API、解析HTML、处理数据等几个步骤。使用网络爬虫是最常见的方法,通过自动化脚本来访问特定的网站,提取网页中的信息。下面将详细描述如何使用Python来采集高考数据。
一、使用网络爬虫
1、安装必要的库
首先,我们需要安装一些Python库来帮助我们进行网络爬取和数据处理。常用的库包括requests、BeautifulSoup、pandas等。
pip install requests
pip install beautifulsoup4
pip install pandas
2、发送HTTP请求
使用requests库发送HTTP请求,获取网页内容。我们可以使用GET方法来获取网页的HTML内容。
import requests
url = 'http://example.com/highschool-exam-data'
response = requests.get(url)
if response.status_code == 200:
page_content = response.text
else:
print('Failed to retrieve the page')
3、解析HTML内容
使用BeautifulSoup库来解析HTML内容,提取我们所需要的数据。通过分析网页的结构,找到包含高考数据的标签和属性。
from bs4 import BeautifulSoup
soup = BeautifulSoup(page_content, 'html.parser')
data_rows = soup.find_all('tr', {'class': 'data-row'})
for row in data_rows:
columns = row.find_all('td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
print(exam_data)
二、调用API
1、查找API
有些网站提供公开的API接口,可以直接获取高考数据。我们需要查找这些API的文档,了解如何进行调用。
2、发送API请求
使用requests库调用API,获取高考数据。通常API会返回JSON格式的数据,我们可以使用requests库的json()方法来解析。
api_url = 'http://example.com/api/highschool-exam-data'
response = requests.get(api_url, headers={'Authorization': 'Bearer YOUR_API_KEY'})
if response.status_code == 200:
data = response.json()
for item in data['results']:
print(item)
else:
print('Failed to retrieve data from API')
三、解析HTML
1、静态网页解析
对于静态网页,可以直接使用BeautifulSoup来解析HTML内容,提取数据。
soup = BeautifulSoup(page_content, 'html.parser')
data_table = soup.find('table', {'id': 'exam-data-table'})
for row in data_table.find_all('tr')[1:]:
columns = row.find_all('td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
print(exam_data)
2、动态网页解析
对于动态网页(使用JavaScript加载数据),可以使用Selenium库来模拟浏览器行为,获取网页内容。
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('http://example.com/highschool-exam-data')
data_rows = driver.find_elements(By.CLASS_NAME, 'data-row')
for row in data_rows:
columns = row.find_elements(By.TAG_NAME, 'td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
print(exam_data)
driver.quit()
四、处理数据
1、存储数据
我们可以使用pandas库将采集到的数据存储到CSV文件中,便于后续分析和处理。
import pandas as pd
data = []
for row in data_rows:
columns = row.find_all('td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
data.append(exam_data)
df = pd.DataFrame(data)
df.to_csv('highschool_exam_data.csv', index=False)
2、数据清洗
在存储数据之前,我们可能需要对数据进行清洗,包括去除空值、处理异常值等。
df.dropna(inplace=True) # 去除空值
df['score'] = df['score'].astype(int) # 将分数转换为整数
df = df[df['score'] >= 0] # 去除异常值
五、实例演示
为了更好地理解如何利用Python采集高考数据,下面我们将通过一个具体的实例进行演示。
1、目标网站分析
假设我们要采集某个网站上的高考成绩数据。首先,我们需要分析该网站的结构,找到包含高考数据的HTML标签。
2、编写爬虫脚本
根据网站结构编写爬虫脚本,提取高考数据并存储到CSV文件中。
import requests
from bs4 import BeautifulSoup
import pandas as pd
def get_exam_data(url):
response = requests.get(url)
if response.status_code != 200:
print('Failed to retrieve the page')
return None
soup = BeautifulSoup(response.text, 'html.parser')
data_rows = soup.find_all('tr', {'class': 'data-row'})
data = []
for row in data_rows:
columns = row.find_all('td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
data.append(exam_data)
return data
def save_to_csv(data, filename):
df = pd.DataFrame(data)
df.to_csv(filename, index=False)
url = 'http://example.com/highschool-exam-data'
exam_data = get_exam_data(url)
if exam_data:
save_to_csv(exam_data, 'highschool_exam_data.csv')
print('Data saved to highschool_exam_data.csv')
3、执行脚本
执行编写好的爬虫脚本,采集数据并存储到CSV文件中。
python highschool_exam_scraper.py
六、进一步优化
1、处理分页数据
有些网站的数据会分布在多个分页中,我们需要处理分页,确保采集到所有的数据。
def get_exam_data(url, page=1):
response = requests.get(url, params={'page': page})
if response.status_code != 200:
print('Failed to retrieve the page')
return None
soup = BeautifulSoup(response.text, 'html.parser')
data_rows = soup.find_all('tr', {'class': 'data-row'})
data = []
for row in data_rows:
columns = row.find_all('td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
data.append(exam_data)
return data
all_data = []
page = 1
while True:
exam_data = get_exam_data(url, page)
if not exam_data:
break
all_data.extend(exam_data)
page += 1
save_to_csv(all_data, 'highschool_exam_data.csv')
2、处理验证码和登录
有些网站在访问数据前需要登录或输入验证码,我们可以使用Selenium库来处理这些情况。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome()
driver.get('http://example.com/login')
username = driver.find_element(By.NAME, 'username')
password = driver.find_element(By.NAME, 'password')
captcha = driver.find_element(By.NAME, 'captcha')
username.send_keys('your_username')
password.send_keys('your_password')
假设我们已经通过其他方法获取了验证码图片并识别出验证码
recognized_captcha = '1234'
captcha.send_keys(recognized_captcha)
captcha.send_keys(Keys.RETURN)
time.sleep(3)
data_rows = driver.find_elements(By.CLASS_NAME, 'data-row')
data = []
for row in data_rows:
columns = row.find_elements(By.TAG_NAME, 'td')
exam_data = {
'subject': columns[0].text,
'score': columns[1].text,
'rank': columns[2].text
}
data.append(exam_data)
driver.quit()
save_to_csv(data, 'highschool_exam_data.csv')
七、总结
利用Python采集高考数据主要包括使用网络爬虫、调用API、解析HTML、处理数据等几个步骤。通过requests库发送HTTP请求获取网页内容,使用BeautifulSoup库解析HTML内容提取数据,调用API获取数据,使用pandas库存储和处理数据。对于复杂情况,可以使用Selenium库处理动态网页、验证码和登录等问题。通过以上方法,我们可以高效地采集高考数据,并进行进一步的数据分析和处理。
相关问答FAQs:
如何选择合适的库来采集高考数据?
在Python中,有多个库可以帮助你高效地采集高考数据。常用的库包括Requests和BeautifulSoup。Requests库用于发送HTTP请求,获取网页内容,而BeautifulSoup则可以解析HTML文档,提取所需的数据。使用这两个库可以方便地抓取和解析高考相关的信息。
在采集高考数据时,如何处理网页反爬虫机制?
许多网站为了保护数据,实施了反爬虫机制。在采集高考数据时,可以通过模拟用户行为、使用代理服务器、设置请求头以及适当的时间间隔来减少被检测的风险。此外,使用随机的User-Agent字符串也可以帮助避免被识别为爬虫。
如何存储采集到的高考数据以便后续分析?
采集到的高考数据可以存储在多种格式中。常见的存储方式包括CSV文件、Excel表格或数据库(如SQLite、MySQL)。选择合适的存储方式应考虑数据的规模和后续分析的需求。例如,对于小规模数据,CSV或Excel文件足够使用;而对于大规模数据,使用数据库会更为高效和灵活。
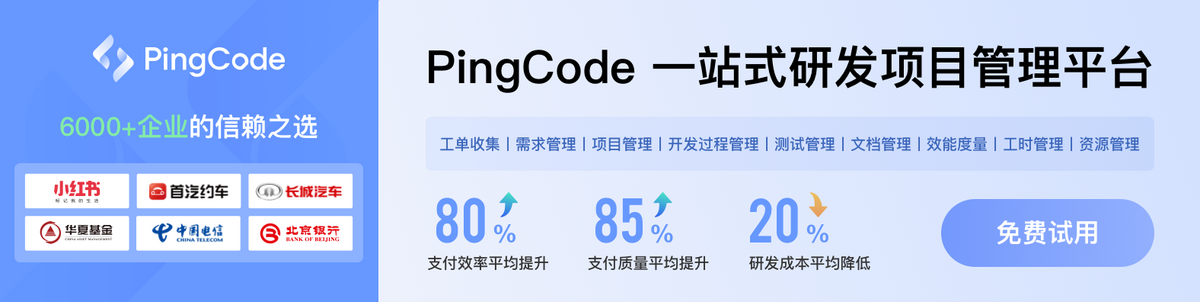