使用Python统计文本词频可以通过导入文本、清理文本、分词、统计词频等步骤来实现。 首先,我们需要读取和清理文本数据,其次是进行分词操作,然后使用字典或Counter来统计每个词出现的频率。在处理过程中,注意去除停用词(如'的','是'等)和标点符号,以提高词频统计的准确性。下面将详细描述如何实现这些步骤。
一、读取和清理文本数据
为了统计文本中的词频,首先需要读取文本数据。Python提供了多种读取文本文件的方法,比如使用open
函数或pandas
库。以下是使用open
函数读取文本文件的示例代码:
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
读取文本后,我们需要进行清理工作,去除标点符号和特殊字符。可以使用Python的re
库进行正则表达式匹配和替换:
import re
def clean_text(text):
# 去除标点符号
cleaned_text = re.sub(r'[^\w\s]', '', text)
# 转换为小写
cleaned_text = cleaned_text.lower()
return cleaned_text
二、分词操作
在清理文本后,我们需要将文本分割成单词列表。对于英文文本,使用Python的split
方法即可将文本按空格分割成单词:
def tokenize(text):
tokens = text.split()
return tokens
对于中文文本,可以使用jieba
库进行分词:
import jieba
def tokenize_chinese(text):
tokens = jieba.lcut(text)
return tokens
三、统计词频
在获取到单词列表后,我们可以使用Python的字典或collections.Counter
类来统计每个单词出现的频率。以下是使用字典统计词频的示例代码:
def count_word_frequency(tokens):
word_freq = {}
for word in tokens:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
return word_freq
或者使用collections.Counter
类,可以更简洁地实现词频统计:
from collections import Counter
def count_word_frequency_with_counter(tokens):
word_freq = Counter(tokens)
return word_freq
四、去除停用词
在统计词频的过程中,我们需要去除一些常见的停用词,以提高词频统计的准确性。可以使用一个停用词列表来过滤掉这些词:
def remove_stopwords(tokens, stopwords):
filtered_tokens = [word for word in tokens if word not in stopwords]
return filtered_tokens
停用词列表可以从网络上获取或者自行定义:
stopwords = ['的', '是', '在', '和', '了', '我', '有', '他', '这', '中']
五、综合实现
将以上各个步骤综合起来,我们可以实现一个完整的文本词频统计程序。以下是完整的代码示例:
import re
from collections import Counter
import jieba
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
def clean_text(text):
cleaned_text = re.sub(r'[^\w\s]', '', text)
cleaned_text = cleaned_text.lower()
return cleaned_text
def tokenize_chinese(text):
tokens = jieba.lcut(text)
return tokens
def remove_stopwords(tokens, stopwords):
filtered_tokens = [word for word in tokens if word not in stopwords]
return filtered_tokens
def count_word_frequency_with_counter(tokens):
word_freq = Counter(tokens)
return word_freq
def main(file_path, stopwords):
text = read_text_file(file_path)
cleaned_text = clean_text(text)
tokens = tokenize_chinese(cleaned_text)
filtered_tokens = remove_stopwords(tokens, stopwords)
word_freq = count_word_frequency_with_counter(filtered_tokens)
return word_freq
if __name__ == "__main__":
file_path = 'your_text_file.txt'
stopwords = ['的', '是', '在', '和', '了', '我', '有', '他', '这', '中']
word_freq = main(file_path, stopwords)
for word, freq in word_freq.most_common(10):
print(f"{word}: {freq}")
通过以上步骤和代码示例,我们可以使用Python统计文本中的词频。需要注意的是,不同语言的文本处理方法有所不同,本文以中文和英文为例进行了说明。在实际应用中,根据需要选择合适的分词和清理方法。
六、可视化词频
为了更直观地展示词频统计结果,我们可以使用matplotlib
或wordcloud
库进行可视化。以下是使用matplotlib
绘制词频柱状图的示例代码:
import matplotlib.pyplot as plt
def plot_word_frequency(word_freq, top_n=10):
most_common_words = word_freq.most_common(top_n)
words = [item[0] for item in most_common_words]
frequencies = [item[1] for item in most_common_words]
plt.figure(figsize=(10, 6))
plt.barh(words, frequencies, color='skyblue')
plt.xlabel('Frequency')
plt.title('Top Word Frequencies')
plt.gca().invert_yaxis()
plt.show()
也可以使用wordcloud
库生成词云图:
from wordcloud import WordCloud
def generate_wordcloud(word_freq):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_freq)
plt.figure(figsize=(10, 6))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
在主函数中调用这些可视化函数:
if __name__ == "__main__":
file_path = 'your_text_file.txt'
stopwords = ['的', '是', '在', '和', '了', '我', '有', '他', '这', '中']
word_freq = main(file_path, stopwords)
# 绘制词频柱状图
plot_word_frequency(word_freq, top_n=10)
# 生成词云图
generate_wordcloud(word_freq)
通过以上代码,我们不仅能够统计文本中的词频,还能够以图形化的方式展示词频统计结果,从而更直观地分析文本内容。无论是用于自然语言处理、数据分析还是其他文本处理任务,词频统计都是一个重要的基础步骤。希望本文能够帮助你更好地掌握使用Python进行文本词频统计的方法和技巧。
相关问答FAQs:
如何使用Python统计文本中的词频?
要统计文本中的词频,您可以使用Python的内置库和一些第三方库。常用的方法是使用collections.Counter
来统计单词出现的次数。首先,您需要将文本分割成单词,然后使用Counter来获取每个单词的频率。以下是一个简单的代码示例:
from collections import Counter
import re
text = "这是一个示例文本。这是一个关于Python的示例文本。"
words = re.findall(r'\w+', text)
word_counts = Counter(words)
print(word_counts)
是否需要对文本进行预处理才能进行词频统计?
文本预处理是统计词频的一个重要步骤,特别是在处理自然语言时。预处理可以包括去除标点符号、转换为小写、去除停用词(如“是”、“的”等)等。这样可以提高统计的准确性和有效性。您可以使用nltk
库来实现停用词的过滤。
有哪些Python库可以帮助进行词频统计?
有几个流行的Python库可以帮助进行词频统计,其中包括:
- NLTK:自然语言处理库,提供了许多功能,包括文本分词和停用词过滤。
- spaCy:一个快速和高效的自然语言处理库,适合大规模文本处理。
- Pandas:可以用来处理数据表格,结合
Counter
可以快速统计词频。
这些库各有特点,您可以根据项目需求选择适合的库。
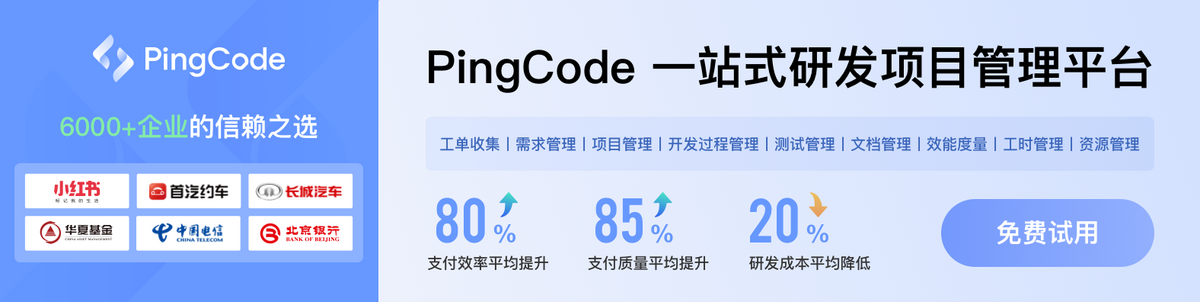