Python字符串赋值方法多样、赋值操作简单、可以直接通过等号赋值、可以使用str()函数进行类型转换。
在Python中,字符串是一种常用的数据类型,并且赋值操作非常简单。可以直接使用等号(=)将一个字符串赋值给变量,也可以通过一些函数和方法实现更复杂的赋值操作。下面我们详细介绍这些方法。
一、直接赋值
直接赋值是最常见的字符串赋值方式。使用等号(=)将一个字符串赋值给一个变量。
my_string = "Hello, World!"
print(my_string) # 输出:Hello, World!
这种方式是最简单的赋值方法,适用于大多数场景。
二、使用str()函数
在Python中,str()
函数可以将其他数据类型转换为字符串,然后赋值给变量。
number = 123
number_str = str(number)
print(number_str) # 输出:123
print(type(number_str)) # 输出:<class 'str'>
str()
函数适用于需要将其他类型的数据转换为字符串的场景,例如数字、列表、字典等。
三、字符串拼接赋值
字符串拼接也是一种常见的赋值方式,可以通过加号(+)将多个字符串拼接成一个字符串,然后赋值给变量。
str1 = "Hello"
str2 = "World"
combined_str = str1 + ", " + str2 + "!"
print(combined_str) # 输出:Hello, World!
这种方式适用于需要将多个字符串拼接在一起的场景。
四、使用格式化字符串
Python提供了多种字符串格式化方法,例如%
操作符、str.format()
方法和f-strings(格式化字符串字面值)。这些方法可以用于生成复杂的字符串并赋值给变量。
1. %操作符
name = "Alice"
age = 30
formatted_str = "My name is %s and I am %d years old." % (name, age)
print(formatted_str) # 输出:My name is Alice and I am 30 years old.
2. str.format()方法
name = "Bob"
age = 25
formatted_str = "My name is {} and I am {} years old.".format(name, age)
print(formatted_str) # 输出:My name is Bob and I am 25 years old.
3. f-strings
name = "Charlie"
age = 35
formatted_str = f"My name is {name} and I am {age} years old."
print(formatted_str) # 输出:My name is Charlie and I am 35 years old.
f-strings是Python 3.6引入的功能,使用起来更加简洁和直观。
五、从用户输入获取字符串
通过input()
函数可以从用户输入获取字符串,并赋值给变量。
user_input = input("Enter a string: ")
print("You entered:", user_input)
这种方式适用于交互式程序,需要从用户获取输入的场景。
六、从文件读取字符串
可以通过文件操作函数从文件中读取字符串,并赋值给变量。
with open("example.txt", "r") as file:
file_content = file.read()
print(file_content)
这种方式适用于需要从文件中读取数据的场景。
七、使用正则表达式处理字符串
正则表达式(regular expressions)是一种强大的字符串处理工具,可以用于搜索、替换和拆分字符串。Python的re
模块提供了正则表达式的支持。
1. 搜索和替换
import re
original_str = "The rain in Spain stays mainly in the plain."
pattern = "ain"
replacement = "xxx"
new_str = re.sub(pattern, replacement, original_str)
print(new_str) # 输出:The rxxx in Spxxx stays mxxxly in the plxxx.
2. 拆分字符串
import re
original_str = "apple,banana,orange"
pattern = ","
split_list = re.split(pattern, original_str)
print(split_list) # 输出:['apple', 'banana', 'orange']
正则表达式适用于需要对字符串进行复杂操作的场景。
八、使用字符串方法进行处理
Python字符串对象提供了丰富的方法,可以用于字符串处理和赋值。例如,upper()
, lower()
, replace()
, split()
等方法。
1. 转换为大写
original_str = "hello"
upper_str = original_str.upper()
print(upper_str) # 输出:HELLO
2. 替换子字符串
original_str = "Hello, World!"
new_str = original_str.replace("World", "Python")
print(new_str) # 输出:Hello, Python!
3. 拆分字符串
original_str = "apple,banana,orange"
split_list = original_str.split(",")
print(split_list) # 输出:['apple', 'banana', 'orange']
这些方法适用于需要对字符串进行特定操作的场景。
九、字符串赋值的注意事项
1. 字符串是不可变对象
在Python中,字符串是不可变对象,这意味着一旦创建,字符串的内容就不能被修改。每次对字符串进行操作时,都会创建一个新的字符串对象。
original_str = "hello"
new_str = original_str.upper()
print(original_str) # 输出:hello
print(new_str) # 输出:HELLO
2. 使用多行字符串
可以使用三重引号("""或''')创建多行字符串,并赋值给变量。
multi_line_str = """This is a
multi-line
string."""
print(multi_line_str)
多行字符串适用于需要包含换行符的字符串。
十、字符串赋值的实际应用
1. 数据处理和清洗
在数据处理和清洗过程中,字符串赋值是一个常见操作。例如,从文件读取数据并进行清洗处理。
with open("data.txt", "r") as file:
raw_data = file.read()
clean_data = raw_data.replace("\n", " ").strip()
print(clean_data)
2. 动态生成SQL查询
在数据库操作中,可以使用字符串赋值生成动态SQL查询语句。
table_name = "employees"
column_name = "salary"
query = f"SELECT {column_name} FROM {table_name} WHERE {column_name} > 50000"
print(query) # 输出:SELECT salary FROM employees WHERE salary > 50000
3. 动态生成HTML内容
在Web开发中,可以使用字符串赋值动态生成HTML内容。
title = "Welcome"
body = "This is a dynamic HTML page."
html_content = f"<html><head><title>{title}</title></head><body>{body}</body></html>"
print(html_content)
十一、总结
通过以上内容,我们详细介绍了Python字符串赋值的多种方法,包括直接赋值、使用str()函数、字符串拼接赋值、格式化字符串、从用户输入获取字符串、从文件读取字符串、使用正则表达式处理字符串、使用字符串方法进行处理等。每种方法都有其适用的场景和优势,希望这些内容能帮助你更好地理解和使用Python字符串赋值。
在实际应用中,选择合适的字符串赋值方法可以提高代码的可读性和效率。希望这篇文章能够为你在Python编程中处理字符串提供一些有价值的参考。
相关问答FAQs:
如何在Python中创建和赋值字符串?
在Python中,字符串可以通过简单的赋值语句来创建。可以使用单引号或双引号来定义字符串。例如,my_string = 'Hello, World!'
或 my_string = "Hello, World!"
都是有效的字符串赋值方式。赋值后,可以通过变量名来访问字符串的内容。
Python字符串是否可以被修改?
Python中的字符串是不可变的,这意味着一旦创建,就不能修改其中的字符。要“修改”字符串,通常需要创建一个新的字符串。例如,如果你想在一个现有字符串的基础上添加内容,可以使用字符串连接,如new_string = my_string + " How are you?"
。
在Python中如何进行字符串格式化?
字符串格式化可以通过多种方式实现,包括使用f-字符串、format()
方法和百分号格式化。f-字符串是Python 3.6及更高版本的推荐方式,例如,name = "Alice"; greeting = f"Hello, {name}!"
。这种方法使得代码更加清晰易懂,方便在字符串中插入变量。
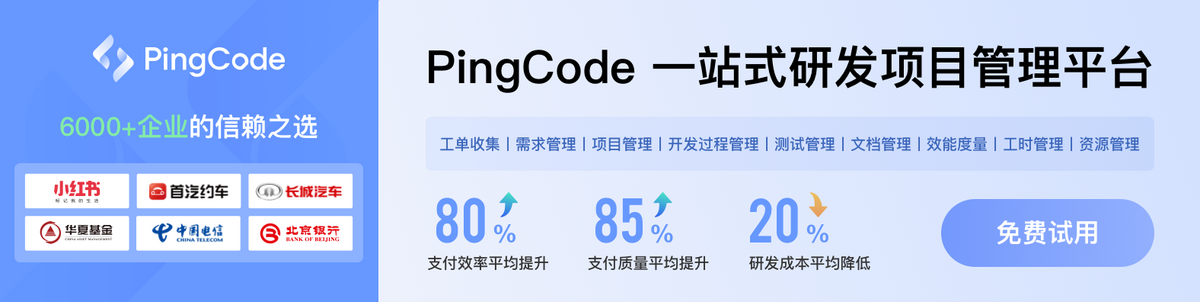