在Linux系统中编写Python脚本的方法有很多种,通常包括安装Python、创建脚本文件、编写代码、设置文件权限和执行脚本等步骤。 这些步骤看似简单,但每一步都有其重要性和技巧,下面将详细介绍如何在Linux中编写和运行Python脚本。
一、安装Python环境
在Linux系统上,大多数发行版默认都会预装Python。你可以通过以下命令检查Python是否已经安装,以及查看其版本:
python --version
python3 --version
如果系统中没有安装Python,可以使用包管理工具进行安装。例如,在Debian/Ubuntu系统中,可以使用以下命令安装Python:
sudo apt update
sudo apt install python3
在RedHat/CentOS系统中,可以使用以下命令安装Python:
sudo yum install python3
二、创建脚本文件
在Linux中,Python脚本文件通常使用“.py”作为扩展名。你可以使用任何文本编辑器来创建和编辑Python脚本文件,如vim、nano、gedit等。以下是使用nano编辑器创建Python脚本文件的示例:
nano myscript.py
三、编写Python代码
打开脚本文件后,你可以在其中编写Python代码。以下是一个简单的Python脚本示例,它打印出“Hello, World!”:
#!/usr/bin/env python3
print("Hello, World!")
在脚本的第一行,#!/usr/bin/env python3
是一个 shebang,它告诉操作系统使用哪个解释器来执行该脚本。
四、设置文件权限
为了使脚本可执行,你需要设置文件的执行权限。可以使用以下命令设置权限:
chmod +x myscript.py
五、执行Python脚本
设置好权限后,你可以通过以下命令执行Python脚本:
./myscript.py
或者直接使用Python解释器来运行脚本:
python3 myscript.py
六、使用虚拟环境
在开发Python项目时,使用虚拟环境可以避免包管理的混乱和依赖冲突。 你可以使用venv
模块来创建虚拟环境。以下是创建和使用虚拟环境的步骤:
- 创建虚拟环境:
python3 -m venv myenv
- 激活虚拟环境:
source myenv/bin/activate
- 在虚拟环境中安装依赖包:
pip install requests
- 运行Python脚本:
python myscript.py
- 退出虚拟环境:
deactivate
七、使用包管理工具
Python有强大的包管理工具,如pip,可以方便地安装和管理第三方库。 以下是一些常用的pip命令:
- 安装包:
pip install package_name
- 查看已安装的包:
pip list
- 升级包:
pip install --upgrade package_name
- 卸载包:
pip uninstall package_name
八、调试Python脚本
在开发过程中,调试是非常重要的一环。Python提供了多种调试工具和方法:
-
使用print语句:最简单的方法是在代码中插入print语句,打印变量的值和程序的执行流。
-
使用pdb模块:pdb是Python内置的调试器,提供了单步执行、设置断点、检查变量等功能。以下是使用pdb调试的示例:
import pdb
def my_function():
x = 10
y = 20
pdb.set_trace() # 设置断点
z = x + y
return z
my_function()
在运行脚本时,程序会在设置断点的地方暂停,你可以输入调试命令进行调试。
- 使用IDE:很多集成开发环境(IDE)如PyCharm、Visual Studio Code等都提供了强大的调试功能,可以设置断点、查看变量、单步执行代码等。
九、编写模块和包
在编写较大项目时,将代码组织成模块和包可以提高代码的可读性和可维护性。
- 模块:一个Python文件就是一个模块,可以通过import语句导入使用。例如,创建一个名为
mymodule.py
的文件:
def greet(name):
return f"Hello, {name}!"
在另一个脚本中导入并使用该模块:
import mymodule
print(mymodule.greet("World"))
- 包:包是一个包含多个模块的文件夹,文件夹中必须包含一个
__init__.py
文件。以下是一个包的示例结构:
mypackage/
__init__.py
module1.py
module2.py
在__init__.py
文件中可以导入包中的模块:
from .module1 import foo
from .module2 import bar
在使用时可以导入整个包:
import mypackage
mypackage.foo()
mypackage.bar()
十、使用版本控制
在开发过程中,使用版本控制系统(如Git)可以跟踪代码的变化、管理多个开发分支,并与团队成员协作。
- 初始化Git仓库:
git init
- 添加文件到仓库:
git add myscript.py
- 提交更改:
git commit -m "Initial commit"
- 远程仓库:
git remote add origin <repository_url>
git push -u origin master
- 分支管理:
git branch new-feature
git checkout new-feature
- 合并分支:
git checkout master
git merge new-feature
十一、使用Lint工具
为了保持代码的一致性和高质量,使用代码检查工具(如pylint、flake8)可以帮助发现代码中的潜在问题和不规范之处。
- 安装pylint:
pip install pylint
- 使用pylint检查代码:
pylint myscript.py
十二、编写单元测试
编写单元测试可以确保代码的正确性和稳定性。Python的unittest模块提供了一个内置的测试框架。
- 创建测试文件
test_myscript.py
:
import unittest
from myscript import my_function
class TestMyFunction(unittest.TestCase):
def test_case1(self):
self.assertEqual(my_function(2, 3), 5)
def test_case2(self):
self.assertEqual(my_function(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
- 运行单元测试:
python -m unittest test_myscript.py
十三、处理异常
在编写Python脚本时,处理异常可以提高代码的健壮性和用户体验。
- 使用try-except语句捕获异常:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero.")
- 自定义异常:
class CustomError(Exception):
pass
try:
raise CustomError("This is a custom error.")
except CustomError as e:
print(e)
十四、使用日志
使用日志记录重要的运行信息,可以帮助排查问题并监控程序的运行状态。Python的logging模块提供了丰富的日志记录功能。
- 设置日志记录:
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
logging.info("This is an info message.")
logging.warning("This is a warning message.")
logging.error("This is an error message.")
- 将日志记录到文件:
logging.basicConfig(filename='app.log', level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
logging.info("This is an info message.")
十五、使用配置文件
在编写Python脚本时,将一些配置信息提取到配置文件中,可以提高代码的灵活性和可维护性。Python的configparser模块提供了处理配置文件的功能。
- 创建配置文件
config.ini
:
[settings]
debug = True
logfile = app.log
- 读取配置文件:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
debug = config.getboolean('settings', 'debug')
logfile = config.get('settings', 'logfile')
十六、处理命令行参数
在编写Python脚本时,处理命令行参数可以使脚本更加灵活和通用。Python的argparse模块提供了一个强大的命令行参数解析工具。
- 使用argparse解析命令行参数:
import argparse
parser = argparse.ArgumentParser(description='A simple script.')
parser.add_argument('--name', type=str, help='Your name')
parser.add_argument('--age', type=int, help='Your age')
args = parser.parse_args()
print(f"Name: {args.name}")
print(f"Age: {args.age}")
- 运行脚本并传递参数:
python myscript.py --name John --age 30
十七、使用面向对象编程
在编写Python脚本时,使用面向对象编程(OOP)可以提高代码的可重用性和可维护性。
- 定义类和方法:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, my name is {self.name} and I am {self.age} years old."
person = Person("John", 30)
print(person.greet())
- 继承和多态:
class Employee(Person):
def __init__(self, name, age, employee_id):
super().__init__(name, age)
self.employee_id = employee_id
def greet(self):
return f"Hello, my name is {self.name}, I am {self.age} years old and my employee ID is {self.employee_id}."
employee = Employee("Jane", 28, "E12345")
print(employee.greet())
十八、使用上下文管理器
上下文管理器可以简化资源管理,例如文件操作和网络连接,确保资源在使用完毕后正确释放。Python的with语句可以方便地使用上下文管理器。
- 使用with语句管理文件操作:
with open('file.txt', 'r') as file:
content = file.read()
print(content)
- 自定义上下文管理器:
class MyContextManager:
def __enter__(self):
print("Entering the context.")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context.")
with MyContextManager():
print("Inside the context.")
十九、使用生成器
生成器是一种特殊的迭代器,可以在循环中生成值,而不是一次性返回所有值。生成器可以提高代码的效率和节省内存。
- 定义生成器函数:
def my_generator():
yield 1
yield 2
yield 3
for value in my_generator():
print(value)
- 使用生成器表达式:
squares = (x * x for x in range(10))
for square in squares:
print(square)
二十、处理日期和时间
在编写Python脚本时,处理日期和时间是常见的需求。Python的datetime模块提供了强大的日期和时间处理功能。
- 获取当前日期和时间:
from datetime import datetime
now = datetime.now()
print(now)
- 格式化日期和时间:
formatted_date = now.strftime('%Y-%m-%d %H:%M:%S')
print(formatted_date)
- 解析日期和时间字符串:
date_str = '2023-01-01 12:00:00'
parsed_date = datetime.strptime(date_str, '%Y-%m-%d %H:%M:%S')
print(parsed_date)
二十一、网络编程
在编写Python脚本时,进行网络编程可以实现数据的传输和通信。Python的socket模块提供了底层的网络编程接口,而requests模块提供了高级的HTTP请求接口。
- 使用socket模块进行TCP通信:
import socket
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind(('localhost', 8080))
server.listen(1)
client, address = server.accept()
print(f"Connection from {address}")
data = client.recv(1024)
print(f"Received: {data.decode()}")
client.send(b"Hello, Client!")
client.close()
- 使用requests模块发送HTTP请求:
import requests
response = requests.get('https://api.github.com')
print(response.json())
二十二、并发编程
在编写Python脚本时,并发编程可以提高程序的性能和效率。Python提供了多线程(threading模块)和多进程(multiprocessing模块)两种并发编程方式。
- 使用多线程:
import threading
def worker(num):
print(f"Worker {num}")
threads = []
for i in range(5):
t = threading.Thread(target=worker, args=(i,))
threads.append(t)
t.start()
for t in threads:
t.join()
- 使用多进程:
import multiprocessing
def worker(num):
print(f"Worker {num}")
processes = []
for i in range(5):
p = multiprocessing.Process(target=worker, args=(i,))
processes.append(p)
p.start()
for p in processes:
p.join()
二十三、数据库编程
在编写Python脚本时,进行数据库编程可以实现数据的存储和查询。Python提供了多个数据库接口模块,如sqlite3、MySQLdb、psycopg2等。
- 使用sqlite3模块操作SQLite数据库:
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)''')
cursor.execute('''INSERT INTO users (name) VALUES ('John')''')
conn.commit()
cursor.execute('''SELECT * FROM users''')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
- 使用MySQLdb模块操作MySQL数据库:
import MySQLdb
conn = MySQLdb.connect(host='localhost', user='root', passwd='password', db='test')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(100))''')
cursor.execute('''INSERT INTO users (name) VALUES ('John')''')
conn.commit()
cursor.execute('''SELECT * FROM users''')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
- 使用psycopg2模块操作PostgreSQL数据库:
import psycopg2
conn = psycopg2.connect(host='localhost', dbname='test', user='postgres', password='password')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id SERIAL PRIMARY KEY, name VARCHAR(100))''')
cursor.execute('''INSERT INTO users (name) VALUES ('John')''')
conn.commit()
cursor.execute('''SELECT * FROM users''')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
二十四、图形用户界面编程
在编写Python脚本时,进行图形用户界面(GUI)编程可以提高用户体验。Python提供了多个GUI编程库,如tkinter、PyQt、wxPython等。
- 使用tkinter创建简单的GUI应用:
import tkinter as tk
def greet():
print(f"Hello, {entry.get()}!")
root = tk.Tk()
root.title("Simple GUI")
label = tk.Label(root, text="Enter your name:")
label.pack()
entry = tk.Entry(root)
entry.pack()
button = tk.Button(root, text="Greet", command=greet)
button.pack()
root.mainloop()
- 使用PyQt创建简单的GUI应用:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout, QLineEdit, QPushButton
def greet():
print(f"Hello, {entry
相关问答FAQs:
如何在Linux环境中安装Python?
在Linux系统中,Python通常会预装在大多数发行版中。您可以通过在终端中输入python --version
或python3 --version
来检查已安装的Python版本。如果未安装,可以使用包管理器,例如在Debian/Ubuntu上使用sudo apt-get install python3
,在Fedora上使用sudo dnf install python3
。
如何创建和运行Python脚本?
创建Python脚本非常简单。您可以使用任何文本编辑器(如Vim、Nano或VSCode)编写代码,保存为.py
文件。例如,使用Nano编辑器创建脚本:nano myscript.py
。在文件中输入Python代码,保存并退出后,通过命令python3 myscript.py
来运行脚本。
在Linux中如何管理Python包?
Python提供了pip
工具来管理包和依赖。可以通过命令pip install package_name
来安装所需的包,使用pip list
查看已安装的包。确保使用pip3
来管理Python 3的包,以避免与Python 2的包冲突。若要更新包,可以使用pip install --upgrade package_name
命令。
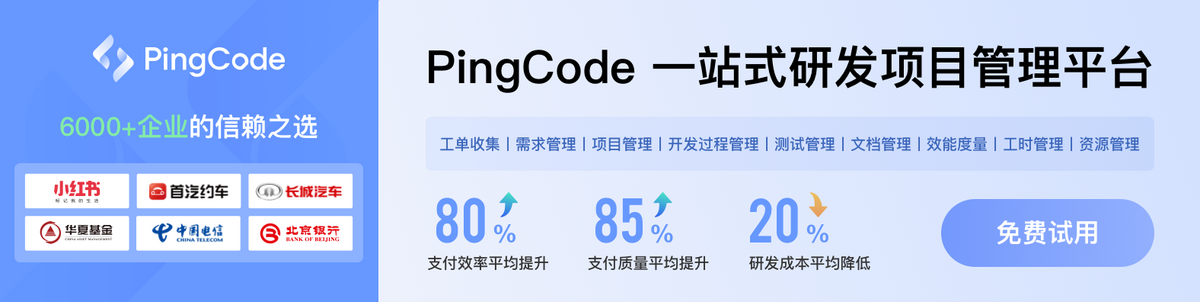