在Python中设置阈值并发出警告,可以通过使用条件语句、日志记录模块或警告模块来实现,常见的方法包括使用if条件语句、使用logging模块、使用warnings模块。 其中,使用if条件语句是最简单且直接的方法,而使用logging和warnings模块则提供了更专业的警告和日志记录功能。以下是详细介绍及示例:
一、使用if条件语句
使用if条件语句是最简单直接的方法,当某个变量超过设定的阈值时,可以通过print函数或者其他方式发出警告。
threshold = 100
value = 120
if value > threshold:
print(f"Warning: Value {value} exceeds the threshold of {threshold}")
在这个示例中,我们设定了一个阈值threshold
为100,当value
超过这个阈值时,程序将输出一条警告信息。
二、使用logging模块
logging模块提供了更专业的日志记录功能,可以记录不同级别的日志信息,如DEBUG、INFO、WARNING、ERROR和CRITICAL。我们可以使用logging模块来记录超过阈值的警告信息。
import logging
配置日志记录
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
threshold = 100
value = 120
if value > threshold:
logger.warning(f"Value {value} exceeds the threshold of {threshold}")
在这个示例中,我们首先配置了日志记录的基本设置,并设定了日志级别为INFO。然后,通过logger.warning
方法记录超过阈值的警告信息。
三、使用warnings模块
warnings模块用于发出警告信息,并且可以控制警告的显示方式和行为。可以使用warnings.warn
函数发出警告。
import warnings
threshold = 100
value = 120
if value > threshold:
warnings.warn(f"Value {value} exceeds the threshold of {threshold}", UserWarning)
在这个示例中,我们使用warnings.warn
函数发出了一个UserWarning类型的警告信息。当value
超过阈值时,程序将显示一条警告。
四、具体应用示例
数据监控
在实际应用中,我们常常需要监控数据,例如传感器数据、服务器性能数据等。当数据超过设定的阈值时,需要发出警告以便及时处理。
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def monitor_data(data, threshold):
for value in data:
if value > threshold:
logger.warning(f"Data value {value} exceeds the threshold of {threshold}")
data = [95, 102, 85, 110, 99]
threshold = 100
monitor_data(data, threshold)
在这个示例中,我们定义了一个monitor_data
函数来监控数据列表中的每一个值。当某个值超过阈值时,程序将记录一条警告信息。
服务器性能监控
在服务器性能监控中,可以设置CPU使用率、内存使用率等的阈值,当超过阈值时发出警告。
import psutil
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def monitor_server_performance(cpu_threshold, memory_threshold):
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
memory_usage = memory_info.percent
if cpu_usage > cpu_threshold:
logger.warning(f"CPU usage {cpu_usage}% exceeds the threshold of {cpu_threshold}%")
if memory_usage > memory_threshold:
logger.warning(f"Memory usage {memory_usage}% exceeds the threshold of {memory_threshold}%")
cpu_threshold = 80
memory_threshold = 80
monitor_server_performance(cpu_threshold, memory_threshold)
在这个示例中,我们使用psutil库来获取CPU和内存的使用率,并设定阈值。当使用率超过阈值时,程序将记录一条警告信息。
五、使用其他报警方式
除了日志记录和警告模块,我们还可以使用其他方式来发出警告,例如发送电子邮件、短信或者通过其他报警系统。
发送电子邮件
我们可以使用smtplib模块来发送电子邮件,当数据超过阈值时,发送一封警告邮件。
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_warning_email(subject, message, to_email):
from_email = "your_email@example.com"
password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(message, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("Warning email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
threshold = 100
value = 120
if value > threshold:
subject = "Threshold Exceeded Warning"
message = f"Value {value} exceeds the threshold of {threshold}"
to_email = "recipient@example.com"
send_warning_email(subject, message, to_email)
在这个示例中,我们定义了一个send_warning_email
函数来发送警告邮件。当value
超过阈值时,程序将发送一封警告邮件到指定的收件人邮箱。
发送短信
我们可以使用第三方服务提供的API,如Twilio,来发送短信警告。
from twilio.rest import Client
def send_warning_sms(message, to_phone):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
from_phone = 'your_twilio_phone'
client = Client(account_sid, auth_token)
try:
message = client.messages.create(
body=message,
from_=from_phone,
to=to_phone
)
print("Warning SMS sent successfully")
except Exception as e:
print(f"Failed to send SMS: {e}")
threshold = 100
value = 120
if value > threshold:
message = f"Value {value} exceeds the threshold of {threshold}"
to_phone = "+1234567890"
send_warning_sms(message, to_phone)
在这个示例中,我们使用Twilio的API来发送警告短信。当value
超过阈值时,程序将发送一条警告短信到指定的手机号码。
六、综合应用
在实际应用中,可能需要综合使用多种方法来设置阈值并发出警告。例如,可以先记录日志,然后发送电子邮件或短信通知相关人员。以下是一个综合应用的示例:
import logging
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from twilio.rest import Client
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def send_warning_email(subject, message, to_email):
from_email = "your_email@example.com"
password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(message, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("Warning email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
def send_warning_sms(message, to_phone):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
from_phone = 'your_twilio_phone'
client = Client(account_sid, auth_token)
try:
message = client.messages.create(
body=message,
from_=from_phone,
to=to_phone
)
print("Warning SMS sent successfully")
except Exception as e:
print(f"Failed to send SMS: {e}")
threshold = 100
value = 120
if value > threshold:
logger.warning(f"Value {value} exceeds the threshold of {threshold}")
subject = "Threshold Exceeded Warning"
message = f"Value {value} exceeds the threshold of {threshold}"
to_email = "recipient@example.com"
send_warning_email(subject, message, to_email)
to_phone = "+1234567890"
send_warning_sms(message, to_phone)
在这个综合应用示例中,我们使用了日志记录、发送电子邮件和发送短信三种方式。当value
超过阈值时,程序将记录警告日志,并发送一封警告邮件和一条警告短信。
七、总结
通过以上内容,我们详细介绍了在Python中设置阈值并发出警告的多种方法,包括使用if条件语句、logging模块、warnings模块,以及发送电子邮件和短信等报警方式。具体选择哪种方法,取决于实际应用场景和需求。在实际应用中,可以结合多种方法来实现更加全面和有效的报警机制,以便及时发现和处理问题。
八、补充内容
使用第三方库进行监控
除了上述方法,我们还可以使用一些第三方库进行更加专业和全面的监控。例如,Prometheus和Grafana是常用的监控和报警系统,可以与Python程序结合使用。
使用Prometheus
Prometheus是一个开源的系统监控和报警工具,可以通过HTTP拉取数据进行监控。我们可以使用prometheus_client库将Python程序的数据暴露给Prometheus进行监控。
from prometheus_client import start_http_server, Summary
import random
import time
REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request')
def process_request(t):
time.sleep(t)
if __name__ == '__main__':
start_http_server(8000)
while True:
t = random.random()
REQUEST_TIME.observe(t)
process_request(t)
在这个示例中,我们使用prometheus_client库将请求处理时间暴露给Prometheus进行监控。Prometheus将定期拉取数据进行监控和报警。
使用Grafana
Grafana是一个开源的可视化工具,可以与Prometheus结合使用,提供丰富的监控和报警功能。通过Grafana的界面,可以方便地设置阈值和报警规则,当数据超过阈值时,可以通过电子邮件、短信等方式发出警告。
使用第三方服务进行报警
除了自建监控系统,还可以使用一些第三方服务进行报警。例如,PagerDuty、OpsGenie等都是常用的报警服务,可以与Python程序结合使用,通过API发送报警信息。
使用PagerDuty
PagerDuty是一个专业的报警服务,可以通过API发送报警信息。
import requests
def send_pagerduty_alert(message):
url = "https://events.pagerduty.com/v2/enqueue"
headers = {
"Content-Type": "application/json"
}
payload = {
"routing_key": "your_integration_key",
"event_action": "trigger",
"payload": {
"summary": message,
"source": "your_application",
"severity": "critical"
}
}
try:
response = requests.post(url, json=payload, headers=headers)
response.raise_for_status()
print("PagerDuty alert sent successfully")
except requests.exceptions.RequestException as e:
print(f"Failed to send PagerDuty alert: {e}")
threshold = 100
value = 120
if value > threshold:
message = f"Value {value} exceeds the threshold of {threshold}"
send_pagerduty_alert(message)
在这个示例中,我们使用requests库调用PagerDuty的API发送报警信息。当value
超过阈值时,程序将发送一条报警信息到PagerDuty。
九、扩展应用
实时数据流监控
在一些实时数据流应用中,如物联网、金融交易等,需要对数据流进行实时监控并及时发出警告。可以使用流处理框架如Apache Kafka、Apache Flink等结合Python实现实时数据流监控。
使用Apache Kafka
Apache Kafka是一个分布式流处理平台,可以处理实时数据流。我们可以使用kafka-python库将数据发送到Kafka,并在Kafka消费者中进行监控和报警。
from kafka import KafkaProducer, KafkaConsumer
import json
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
producer = KafkaProducer(bootstrap_servers='localhost:9092', value_serializer=lambda v: json.dumps(v).encode('utf-8'))
def send_data_to_kafka(topic, data):
producer.send(topic, data)
producer.flush()
consumer = KafkaConsumer('test_topic', bootstrap_servers='localhost:9092', value_deserializer=lambda v: json.loads(v.decode('utf-8')))
threshold = 100
def monitor_kafka_data():
for message in consumer:
value = message.value['value']
if value > threshold:
logger.warning(f"Kafka data value {value} exceeds the threshold of {threshold}")
if __name__ == '__main__':
data = {'value': 120}
send_data_to_kafka('test_topic', data)
monitor_kafka_data()
在这个示例中,我们使用kafka-python库将数据发送到Kafka的test_topic
主题,并在消费者中监控数据。当数据值超过阈值时,程序将记录警告信息。
使用Apache Flink
Apache Flink是一个分布式流处理框架,可以处理实时数据流。我们可以使用pyflink库将数据流发送到Flink,并在Flink中进行监控和报警。
from pyflink.datastream import StreamExecutionEnvironment
from pyflink.table import StreamTableEnvironment, DataTypes
from pyflink.table.descriptors import Schema, Json, Kafka
env = StreamExecutionEnvironment.get_execution_environment()
t_env = StreamTableEnvironment.create(env)
t_env.connect(
Kafka()
.version("universal")
.topic("test_topic")
.start_from_latest()
.property("bootstrap.servers", "localhost:9092")
.property("group.id", "test_group")
) \
.with_format(
Json()
.json_schema("{type: 'object', properties: {value: {type: 'number'}}}")
.fail_on_missing_field(True)
) \
.with_schema(
Schema()
.field("value", DataTypes.FLOAT())
) \
.in_append_mode() \
.create_temporary_table("source_table")
source_table = t_env.from_path("source_table")
threshold = 100.0
result_table = source_table.select("value") \
.filter(f"value > {threshold}")
result_table.execute().print()
在这个示例中,我们使用pyflink库将Kafka的数据流连接到Flink,并在Flink中进行监控。当数据值超过阈值时,程序将输出警告信息。
十、总结与展望
通过本文的详细介绍,我们了解了在Python中设置阈值并发出警告的多种方法,包括使用if条件语句、logging模块、warnings模块,发送电子邮件和短信等报警方式,以及使用Prometheus、Grafana、PagerDuty等第三方服务进行监控和报警。同时,我们还探讨了在实时数据流应用中如何进行监控和报警。
在实际应用中,根据具体需求和场景,可以综合使用多种方法和工具,构建更加全面和高效的监控和报警系统。未来,随着技术的发展,监控和报警的方式和工具将更加丰富和智能化,为我们提供更加可靠的保障。
希望本文对您在Python中设置阈值并发出警告有所帮助。如果有任何问题或建议,欢迎交流和讨论。
相关问答FAQs:
如何在Python中设置阈值以监控特定数据?
在Python中设置阈值可以通过简单的条件判断来实现。可以使用if语句检查数据是否超过预设的阈值。如果超过了,可以使用打印语句或日志模块发出警告。例如,针对温度监测,可以设置一个阈值,如果温度超过该值,就打印警告信息或发送通知。
Python中可以使用哪些库来实现阈值警告功能?
有多个Python库可以帮助实现阈值警告功能。比如,NumPy和Pandas可用于处理和分析数据,结合条件判断可以轻松设置阈值。另外,Matplotlib可以用于可视化数据,帮助更直观地理解阈值的设定和警报的触发。
如何将阈值警告功能集成到一个实时监测系统中?
要将阈值警告功能集成到实时监测系统中,可以使用Python的定时任务库如Schedule或APScheduler。通过定期检查数据源的值,并与阈值进行比较,一旦发现超过阈值的情况,就可以触发相应的警告机制,比如发送电子邮件或推送通知。
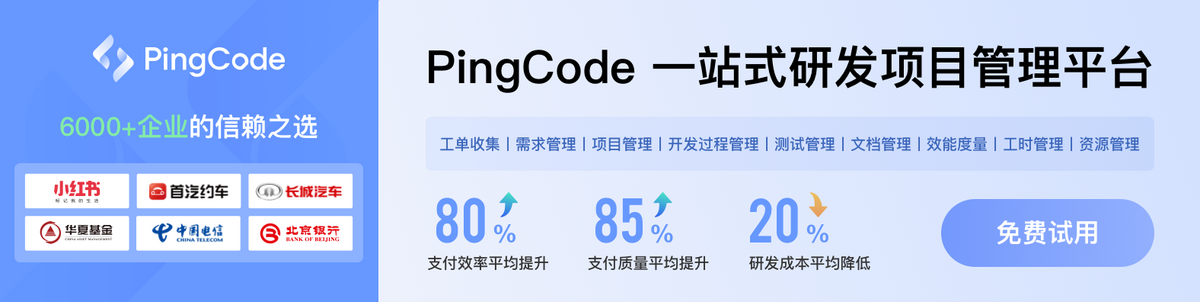