在Python中使用多线程并结合代理进行网络请求,可以提高网络爬虫或其他网络操作的效率。使用Python多线程进行代理的主要步骤包括:创建代理池、定义线程任务函数、初始化线程并启动。接下来,我们将详细讨论如何在Python中实现这些步骤。
一、创建代理池
首先,我们需要创建一个代理池,用于存储多个代理服务器地址。代理池可以是一个简单的列表,包含多个代理服务器的IP地址和端口号。我们可以通过以下方式来创建一个代理池:
proxy_pool = [
{"http": "http://123.45.67.89:8080", "https": "https://123.45.67.89:8080"},
{"http": "http://98.76.54.32:8080", "https": "https://98.76.54.32:8080"},
# 添加更多代理
]
二、定义线程任务函数
接下来,我们需要定义一个线程任务函数,该函数将使用代理进行网络请求。我们可以使用requests
库来发送HTTP请求,并使用代理进行这些请求:
import requests
import threading
def fetch_url(proxy, url):
try:
response = requests.get(url, proxies=proxy)
print(f"URL: {url}, Status Code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"Error fetching {url} with proxy {proxy}: {e}")
三、初始化线程并启动
最后,我们需要初始化多个线程,并为每个线程分配一个代理和一个URL。可以使用threading.Thread
类来创建和启动线程:
urls = [
"http://example.com",
"http://example.org",
# 添加更多URL
]
threads = []
for i in range(len(urls)):
proxy = proxy_pool[i % len(proxy_pool)]
url = urls[i]
thread = threading.Thread(target=fetch_url, args=(proxy, url))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
以上代码展示了如何使用Python多线程和代理进行网络请求。接下来,我们将详细介绍每个步骤的具体实现和注意事项。
一、创建代理池
在实际应用中,代理池的创建可能会更加复杂。我们需要从多个代理服务器提供商那里获取代理,并确保这些代理是可用的。可以编写一个简单的函数来验证代理的可用性:
def validate_proxy(proxy):
test_url = "http://example.com"
try:
response = requests.get(test_url, proxies=proxy, timeout=5)
if response.status_code == 200:
return True
except requests.exceptions.RequestException:
return False
return False
proxy_pool = [
{"http": "http://123.45.67.89:8080", "https": "https://123.45.67.89:8080"},
{"http": "http://98.76.54.32:8080", "https": "https://98.76.54.32:8080"},
]
valid_proxies = [proxy for proxy in proxy_pool if validate_proxy(proxy)]
通过这种方式,我们可以确保代理池中的代理是可用的,从而提高网络请求的成功率。
二、定义线程任务函数
在定义线程任务函数时,需要考虑到异常处理和重试机制。网络请求可能会因为各种原因失败,例如代理服务器不可用、请求超时等。可以使用try-except
块来捕获异常,并在必要时进行重试:
import time
def fetch_url(proxy, url, retries=3):
for _ in range(retries):
try:
response = requests.get(url, proxies=proxy, timeout=10)
print(f"URL: {url}, Status Code: {response.status_code}")
return response.text
except requests.exceptions.RequestException as e:
print(f"Error fetching {url} with proxy {proxy}: {e}")
time.sleep(1)
return None
三、初始化线程并启动
在初始化线程时,可以使用Queue
来管理URL和代理,从而更加灵活地分配任务。使用Queue
可以确保线程之间不会出现竞争条件:
from queue import Queue
url_queue = Queue()
proxy_queue = Queue()
for url in urls:
url_queue.put(url)
for proxy in valid_proxies:
proxy_queue.put(proxy)
def worker():
while not url_queue.empty():
url = url_queue.get()
proxy = proxy_queue.get()
fetch_url(proxy, url)
url_queue.task_done()
proxy_queue.task_done()
threads = []
for _ in range(len(valid_proxies)):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
url_queue.join()
proxy_queue.join()
for thread in threads:
thread.join()
通过这种方式,我们可以确保每个线程都能从队列中获取URL和代理,并在任务完成后将其标记为完成。
四、优化与扩展
在实际应用中,可能需要进一步优化和扩展多线程代理的使用。例如,可以考虑以下几点:
1、动态代理池
代理池中的代理可能会随时失效,因此需要定期更新代理池。可以编写一个独立的线程,负责定期从代理服务器提供商获取新的代理,并验证其可用性:
import time
def update_proxy_pool():
while True:
new_proxies = get_new_proxies()
valid_proxies = [proxy for proxy in new_proxies if validate_proxy(proxy)]
with proxy_lock:
proxy_pool.extend(valid_proxies)
time.sleep(600)
proxy_lock = threading.Lock()
update_thread = threading.Thread(target=update_proxy_pool)
update_thread.start()
2、限速与请求间隔
为了避免过于频繁的请求导致目标服务器封禁,可以设置请求间隔和限速:
import random
def fetch_url(proxy, url, retries=3):
for _ in range(retries):
try:
response = requests.get(url, proxies=proxy, timeout=10)
print(f"URL: {url}, Status Code: {response.status_code}")
time.sleep(random.uniform(1, 3)) # 随机间隔1到3秒
return response.text
except requests.exceptions.RequestException as e:
print(f"Error fetching {url} with proxy {proxy}: {e}")
time.sleep(1)
return None
3、日志记录
在实际应用中,记录日志是非常重要的。可以使用logging
库来记录每次请求的详细信息,包括URL、代理、状态码等:
import logging
logging.basicConfig(level=logging.INFO, filename='proxy_requests.log')
def fetch_url(proxy, url, retries=3):
for _ in range(retries):
try:
response = requests.get(url, proxies=proxy, timeout=10)
logging.info(f"URL: {url}, Proxy: {proxy}, Status Code: {response.status_code}")
time.sleep(random.uniform(1, 3))
return response.text
except requests.exceptions.RequestException as e:
logging.error(f"Error fetching {url} with proxy {proxy}: {e}")
time.sleep(1)
return None
4、错误处理与重试机制
进一步完善错误处理与重试机制,确保在代理失效或请求超时时能够及时切换到下一个可用代理,提高请求的成功率:
def fetch_url(proxy, url, retries=3):
for attempt in range(retries):
try:
response = requests.get(url, proxies=proxy, timeout=10)
logging.info(f"URL: {url}, Proxy: {proxy}, Status Code: {response.status_code}")
time.sleep(random.uniform(1, 3))
return response.text
except requests.exceptions.RequestException as e:
logging.error(f"Error fetching {url} with proxy {proxy} on attempt {attempt + 1}: {e}")
time.sleep(1)
return None
通过以上优化和扩展措施,可以进一步提高多线程代理的效率和稳定性,从而更好地完成网络请求任务。
五、多线程与多进程结合
在某些情况下,单纯使用多线程可能无法充分利用多核CPU的优势,可以考虑将多线程与多进程结合使用。Python的multiprocessing
库提供了多进程支持,可以创建多个进程,每个进程中运行多个线程,从而提高并发能力:
from multiprocessing import Process, Queue
def worker(url_queue, proxy_queue):
while not url_queue.empty():
url = url_queue.get()
proxy = proxy_queue.get()
fetch_url(proxy, url)
url_queue.task_done()
proxy_queue.task_done()
url_queue = Queue()
proxy_queue = Queue()
for url in urls:
url_queue.put(url)
for proxy in valid_proxies:
proxy_queue.put(proxy)
processes = []
for _ in range(4): # 创建4个进程
process = Process(target=worker, args=(url_queue, proxy_queue))
process.start()
processes.append(process)
for process in processes:
process.join()
六、代理池管理
在实际应用中,代理池管理是一个复杂的任务,需要考虑代理的获取、验证、更新和失效处理。可以使用第三方库如proxypool
来简化代理池管理:
from proxypool import ProxyPool
proxy_pool = ProxyPool()
def fetch_url_with_proxypool(url):
proxy = proxy_pool.get()
try:
response = requests.get(url, proxies=proxy, timeout=10)
logging.info(f"URL: {url}, Proxy: {proxy}, Status Code: {response.status_code}")
return response.text
except requests.exceptions.RequestException as e:
logging.error(f"Error fetching {url} with proxy {proxy}: {e}")
proxy_pool.remove(proxy)
return None
def worker():
while not url_queue.empty():
url = url_queue.get()
fetch_url_with_proxypool(url)
url_queue.task_done()
threads = []
for _ in range(len(valid_proxies)):
thread = threading.Thread(target=worker)
thread.start()
threads.append(thread)
url_queue.join()
for thread in threads:
thread.join()
七、总结
在Python中使用多线程结合代理进行网络请求,可以显著提高网络爬虫或其他网络操作的效率。核心步骤包括:创建代理池、定义线程任务函数、初始化线程并启动。此外,可以通过动态代理池、限速与请求间隔、日志记录、错误处理与重试机制、多线程与多进程结合以及代理池管理等措施,进一步优化和扩展多线程代理的使用。
在实际应用中,需要根据具体需求和网络环境,灵活调整和优化多线程代理的实现,从而提高网络请求的成功率和效率。希望本文的介绍和代码示例能够对您在Python多线程与代理的使用中有所帮助。
相关问答FAQs:
如何在Python多线程中设置代理?
在Python的多线程环境中使用代理,通常需要在每个线程中单独配置代理。可以使用requests库和threading模块来实现。首先,确保你有一个可用的代理地址。然后,在每个线程的任务中,使用requests库的proxies参数来指定代理。示例代码如下:
import requests
import threading
def fetch(url, proxy):
response = requests.get(url, proxies={"http": proxy, "https": proxy})
print(response.text)
url = "http://example.com"
proxy = "http://your_proxy:port"
threads = []
for i in range(5): # 创建5个线程
thread = threading.Thread(target=fetch, args=(url, proxy))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
多线程使用代理时需要注意哪些问题?
在多线程中使用代理时,可能会遇到一些问题,如代理服务器的连接限制、请求速率限制等。确保使用的代理能够处理并发请求,避免由于超出代理的连接数而导致请求失败。此外,监控线程的异常情况,确保在代理出现问题时能够进行错误处理和重试。
如何选择合适的代理服务?
选择代理服务时,应考虑以下几个因素:稳定性、速度、匿名性和价格。免费代理通常不够稳定,可能会导致请求失败或速度慢。付费代理服务通常提供更好的性能和支持。可以通过查看用户评价和测试代理的速度来评估其合适性。
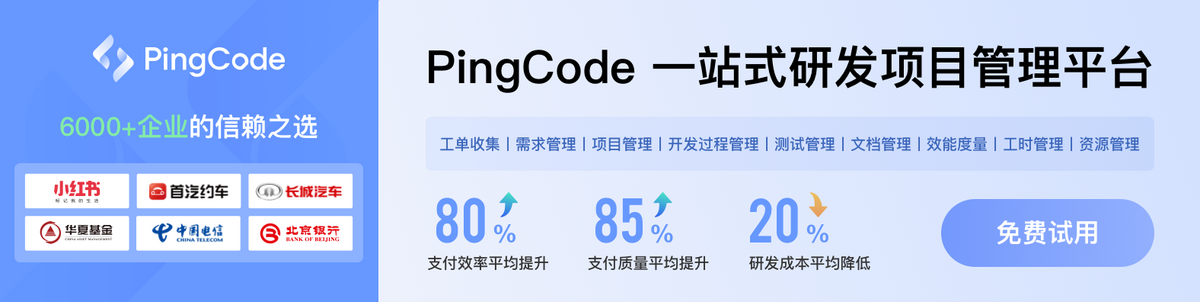