编写Python挖矿程序需要具备基本的编程技能、了解区块链和加密货币的原理、选择适合的挖矿算法、优化计算效率。这些步骤和技巧有助于你创建一个有效的挖矿程序。其中,选择适合的挖矿算法是关键,因为不同的加密货币使用不同的算法,如比特币使用SHA-256,以太坊使用Ethash。下面将详细介绍如何编写一个Python挖矿程序,并解释相关的技术细节。
一、了解区块链和加密货币的基本原理
1. 区块链的概念
区块链是一种分布式账本技术,通过将数据块按时间顺序串联起来形成链条,确保数据的安全性和不可篡改性。每个区块包含上一个区块的哈希值、时间戳和交易数据。
2. 挖矿的基本原理
挖矿是通过计算哈希值来验证和记录交易的过程。矿工需要找到一个满足特定条件的哈希值,这个过程需要大量的计算资源。成功找到哈希值的矿工将获得一定数量的加密货币作为奖励。
3. 挖矿算法
不同的加密货币使用不同的挖矿算法。常见的算法包括SHA-256(用于比特币)、Ethash(用于以太坊)、Scrypt(用于莱特币)等。这些算法的主要目标是确保哈希计算的难度和安全性。
二、选择适合的挖矿算法
1. SHA-256算法
SHA-256(Secure Hash Algorithm 256-bit)是比特币使用的挖矿算法。它是一种加密散列函数,通过对数据进行多次迭代计算,生成一个256位的哈希值。SHA-256的计算过程如下:
- 数据预处理:将数据填充到512位的倍数。
- 初始化哈希值:定义8个32位的初始哈希值。
- 消息分块处理:将数据分成512位的块,每个块进行64轮的哈希计算。
- 哈希合并:将每轮计算的结果合并,生成最终的哈希值。
2. Ethash算法
Ethash是以太坊使用的挖矿算法。它是一种基于内存的算法,通过大量的内存访问来提高计算难度和抵抗ASIC(专用集成电路)挖矿。Ethash的计算过程如下:
- 创建DAG(有向无环图):每个矿工需要生成一个约1GB大小的DAG文件。
- 初始化种子值:根据区块高度生成种子值。
- 计算哈希值:通过多次迭代计算哈希值,并与目标值进行比较。
3. Scrypt算法
Scrypt是一种基于密钥派生函数的挖矿算法,主要用于莱特币。它通过大量的内存访问来提高计算难度和抵抗ASIC挖矿。Scrypt的计算过程如下:
- 初始化参数:定义内存大小和迭代次数。
- 生成密钥流:通过多次哈希计算生成密钥流。
- 哈希计算:使用密钥流和数据进行多次哈希计算,生成最终的哈希值。
三、编写Python挖矿程序
1. 环境准备
在开始编写挖矿程序之前,需要安装Python和相关的库。常用的库包括hashlib
、requests
等。以下是安装Python和库的步骤:
- 安装Python:从Python官方网站下载并安装最新版的Python。
- 安装库:使用
pip
命令安装所需的库。例如,pip install requests
。
2. 编写SHA-256挖矿程序
下面是一个简单的SHA-256挖矿程序示例:
import hashlib
import time
def mine_block(previous_hash, transactions, difficulty):
nonce = 0
start_time = time.time()
while True:
block_data = str(previous_hash) + str(transactions) + str(nonce)
block_hash = hashlib.sha256(block_data.encode()).hexdigest()
if block_hash.startswith('0' * difficulty):
end_time = time.time()
print(f"Block mined: {block_hash}")
print(f"Nonce: {nonce}")
print(f"Time taken: {end_time - start_time} seconds")
return block_hash
nonce += 1
previous_hash = '0000000000000000000'
transactions = ['Alice pays Bob 10 BTC', 'Bob pays Charlie 5 BTC']
difficulty = 4
mine_block(previous_hash, transactions, difficulty)
这个程序通过计算哈希值,寻找满足难度条件的区块。难度值越高,挖矿难度越大。
3. 编写Ethash挖矿程序
Ethash算法较为复杂,需要生成DAG文件和进行大量的内存访问。下面是一个简单的Ethash挖矿程序示例:
import hashlib
import time
def generate_dag(seed, size):
dag = []
for i in range(size):
data = str(seed) + str(i)
dag.append(hashlib.sha256(data.encode()).hexdigest())
return dag
def ethash_mine(seed, transactions, difficulty):
dag = generate_dag(seed, 1000000)
nonce = 0
start_time = time.time()
while True:
block_data = str(seed) + str(transactions) + str(nonce)
block_hash = hashlib.sha256(block_data.encode()).hexdigest()
hash_value = int(block_hash, 16)
for i in range(len(dag)):
hash_value ^= int(dag[i], 16)
if bin(hash_value).count('1') <= difficulty:
end_time = time.time()
print(f"Block mined: {block_hash}")
print(f"Nonce: {nonce}")
print(f"Time taken: {end_time - start_time} seconds")
return block_hash
nonce += 1
seed = '0000000000000000000'
transactions = ['Alice pays Bob 10 ETH', 'Bob pays Charlie 5 ETH']
difficulty = 10000
ethash_mine(seed, transactions, difficulty)
这个程序通过生成DAG文件和计算哈希值,寻找满足难度条件的区块。
4. 编写Scrypt挖矿程序
Scrypt算法需要大量的内存访问和哈希计算。下面是一个简单的Scrypt挖矿程序示例:
import hashlib
import time
def scrypt_mine(previous_hash, transactions, difficulty, memory_size, iterations):
nonce = 0
start_time = time.time()
while True:
block_data = str(previous_hash) + str(transactions) + str(nonce)
block_hash = hashlib.scrypt(block_data.encode(), salt=previous_hash.encode(), n=memory_size, r=8, p=1, dklen=32).hex()
if block_hash.startswith('0' * difficulty):
end_time = time.time()
print(f"Block mined: {block_hash}")
print(f"Nonce: {nonce}")
print(f"Time taken: {end_time - start_time} seconds")
return block_hash
nonce += 1
previous_hash = '0000000000000000000'
transactions = ['Alice pays Bob 10 LTC', 'Bob pays Charlie 5 LTC']
difficulty = 4
memory_size = 1024
iterations = 1024
scrypt_mine(previous_hash, transactions, difficulty, memory_size, iterations)
这个程序通过大量的内存访问和哈希计算,寻找满足难度条件的区块。
四、优化计算效率
1. 多线程并行计算
为了提高挖矿效率,可以使用多线程并行计算。通过分配多个线程同时进行哈希计算,可以显著减少挖矿时间。以下是使用多线程优化SHA-256挖矿程序的示例:
import hashlib
import time
import threading
def mine_block(previous_hash, transactions, difficulty, start_nonce, step):
nonce = start_nonce
while True:
block_data = str(previous_hash) + str(transactions) + str(nonce)
block_hash = hashlib.sha256(block_data.encode()).hexdigest()
if block_hash.startswith('0' * difficulty):
end_time = time.time()
print(f"Block mined: {block_hash}")
print(f"Nonce: {nonce}")
print(f"Time taken: {end_time - start_time} seconds")
return block_hash
nonce += step
previous_hash = '0000000000000000000'
transactions = ['Alice pays Bob 10 BTC', 'Bob pays Charlie 5 BTC']
difficulty = 4
num_threads = 4
threads = []
start_time = time.time()
for i in range(num_threads):
t = threading.Thread(target=mine_block, args=(previous_hash, transactions, difficulty, i, num_threads))
threads.append(t)
t.start()
for t in threads:
t.join()
这个程序通过创建多个线程同时进行哈希计算,提高了挖矿效率。
2. 使用GPU加速计算
GPU(图形处理单元)具有强大的并行计算能力,可以显著提高哈希计算速度。可以使用CUDA或OpenCL等技术在GPU上运行哈希计算。以下是使用PyOpenCL进行GPU加速SHA-256挖矿的示例:
import pyopencl as cl
import numpy as np
import time
def gpu_mine_block(previous_hash, transactions, difficulty):
ctx = cl.create_some_context()
queue = cl.CommandQueue(ctx)
program = cl.Program(ctx, """
__kernel void mine(__global const char *previous_hash, __global const char *transactions, __global const int *nonce, __global char *result) {
int i = get_global_id(0);
char block_data[256];
sprintf(block_data, "%s%s%d", previous_hash, transactions, nonce[i]);
char block_hash[65];
sha256(block_data, block_hash);
if (strncmp(block_hash, "0000", 4) == 0) {
strcpy(result, block_hash);
}
}
""").build()
nonce = np.arange(1000000).astype(np.int32)
result = np.chararray(65)
mf = cl.mem_flags
previous_hash_buf = cl.Buffer(ctx, mf.READ_ONLY | mf.COPY_HOST_PTR, hostbuf=previous_hash.encode())
transactions_buf = cl.Buffer(ctx, mf.READ_ONLY | mf.COPY_HOST_PTR, hostbuf=transactions.encode())
nonce_buf = cl.Buffer(ctx, mf.READ_ONLY | mf.COPY_HOST_PTR, hostbuf=nonce)
result_buf = cl.Buffer(ctx, mf.WRITE_ONLY, result.nbytes)
start_time = time.time()
program.mine(queue, nonce.shape, None, previous_hash_buf, transactions_buf, nonce_buf, result_buf)
cl.enqueue_copy(queue, result, result_buf).wait()
end_time = time.time()
print(f"Block mined: {result.tostring().decode()}")
print(f"Time taken: {end_time - start_time} seconds")
previous_hash = '0000000000000000000'
transactions = 'Alice pays Bob 10 BTC, Bob pays Charlie 5 BTC'
difficulty = 4
gpu_mine_block(previous_hash, transactions, difficulty)
这个程序通过使用PyOpenCL在GPU上运行哈希计算,提高了挖矿效率。
五、监控和管理挖矿过程
1. 监控挖矿状态
为了及时了解挖矿进度和状态,可以使用日志记录和监控工具。例如,可以使用Python的logging
模块记录挖矿日志,并定期输出挖矿进度。以下是一个记录挖矿日志的示例:
import hashlib
import time
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def mine_block(previous_hash, transactions, difficulty):
nonce = 0
start_time = time.time()
while True:
block_data = str(previous_hash) + str(transactions) + str(nonce)
block_hash = hashlib.sha256(block_data.encode()).hexdigest()
if block_hash.startswith('0' * difficulty):
end_time = time.time()
logging.info(f"Block mined: {block_hash}")
logging.info(f"Nonce: {nonce}")
logging.info(f"Time taken: {end_time - start_time} seconds")
return block_hash
nonce += 1
if nonce % 100000 == 0:
logging.info(f"Nonce: {nonce}")
previous_hash = '0000000000000000000'
transactions = ['Alice pays Bob 10 BTC', 'Bob pays Charlie 5 BTC']
difficulty = 4
mine_block(previous_hash, transactions, difficulty)
这个程序通过记录挖矿日志,可以实时监控挖矿状态。
2. 管理挖矿资源
为了合理分配和管理挖矿资源,可以使用任务调度和资源管理工具。例如,可以使用Celery
和Redis
等工具实现分布式任务调度和资源管理。以下是一个使用Celery
调度挖矿任务的示例:
from celery import Celery
import hashlib
app = Celery('mining', broker='redis://localhost:6379/0')
@app.task
def mine_block(previous_hash, transactions, difficulty, start_nonce, step):
nonce = start_nonce
while True:
block_data = str(previous_hash) + str(transactions) + str(nonce)
block_hash = hashlib.sha256(block_data.encode()).hexdigest()
if block_hash.startswith('0' * difficulty):
return block_hash
nonce += step
previous_hash = '0000000000000000000'
transactions = ['Alice pays Bob 10 BTC', 'Bob pays Charlie 5 BTC']
difficulty = 4
num_tasks = 4
tasks = []
for i in range(num_tasks):
tasks.append(mine_block.delay(previous_hash, transactions, difficulty, i, num_tasks))
for task in tasks:
result = task.get()
if result:
print(f"Block mined: {result}")
break
这个程序通过使用Celery
调度挖矿任务,可以合理分配和管理挖矿资源。
六、总结
编写Python挖矿程序需要具备基本的编程技能、了解区块链和加密货币的原理、选择适合的挖矿算法、优化计算效率。通过多线程并行计算和GPU加速计算,可以显著提高挖矿效率。同时,通过监控和管理挖矿过程,可以及时了解挖矿状态并合理分配资源。希望以上内容能帮助你更好地理解和编写Python挖矿程序。
相关问答FAQs:
挖矿程序的基本原理是什么?
挖矿程序的基本原理是通过计算哈希值来验证交易并维护区块链的安全性。挖矿者需要不断尝试不同的输入数据,直到找到一个哈希值符合网络规定的难度目标。这个过程需要消耗大量的计算资源和电力,因此选择合适的算法和优化计算过程是非常重要的。
使用Python编写挖矿程序需要哪些库和工具?
在Python中编写挖矿程序,通常需要使用一些特定的库,例如hashlib
用于生成哈希值,requests
用于与区块链网络进行数据交互。对于更复杂的挖矿任务,可能还需要使用numpy
或pandas
来处理数据,甚至使用asyncio
来提高网络请求的效率。
如何提高Python挖矿程序的效率?
提高Python挖矿程序的效率可以通过多种方式实现。优化算法是关键,例如使用更快的哈希函数或实现多线程处理来并行计算。此外,还可以考虑使用专用的挖矿硬件(如ASIC矿机)与Python程序结合,来提升整体的挖矿性能。合理的代码结构和减少不必要的计算也能显著提高效率。
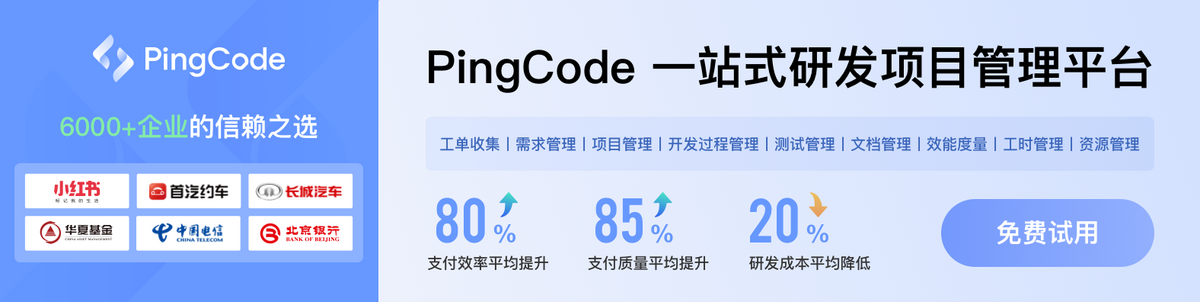