Python可以通过多种方式生成和写入HTML文件,其中包括使用内置的文件操作功能、使用模板引擎如Jinja2、以及使用专门的库如BeautifulSoup。使用Python内置文件操作功能、使用模板引擎、使用BeautifulSoup库是三种常见的方法。下面将详细介绍其中一种方法——使用模板引擎Jinja2。
Jinja2是一个现代的、设计优雅的Python模板引擎,它能够让你通过模板和数据的分离,快速生成HTML文件。Jinja2支持变量替换、控制结构、过滤器等功能,非常适合用于生成动态HTML内容。
一、使用Python内置文件操作功能
使用Python内置的文件操作功能可以直接写入HTML文件。这种方法适用于简单的HTML生成任务。
创建并写入HTML文件
首先,你需要创建一个新的HTML文件,并向文件中写入HTML内容。以下是一个简单的示例:
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Sample HTML Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a sample HTML page generated using Python.</p>
</body>
</html>
"""
with open("sample.html", "w") as file:
file.write(html_content)
读取并修改HTML文件
你还可以读取现有的HTML文件并进行修改。例如:
with open("sample.html", "r") as file:
html_content = file.read()
修改HTML内容
html_content = html_content.replace("Hello, World!", "Hello, Python!")
with open("sample.html", "w") as file:
file.write(html_content)
二、使用模板引擎Jinja2
Jinja2模板引擎适用于生成复杂的动态HTML内容。它支持变量替换、条件语句、循环等功能。
安装Jinja2
首先,你需要安装Jinja2库:
pip install Jinja2
创建Jinja2模板
接下来,创建一个Jinja2模板文件(例如template.html
):
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ message }}</p>
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
</body>
</html>
渲染Jinja2模板
使用Python代码渲染模板并生成HTML文件:
from jinja2 import Environment, FileSystemLoader
创建一个Jinja2环境
env = Environment(loader=FileSystemLoader('.'))
加载模板文件
template = env.get_template('template.html')
渲染模板
html_content = template.render(
title="Sample HTML Page",
heading="Hello, World!",
message="This is a sample HTML page generated using Python and Jinja2.",
items=["Item 1", "Item 2", "Item 3"]
)
写入HTML文件
with open("output.html", "w") as file:
file.write(html_content)
三、使用BeautifulSoup库
BeautifulSoup库主要用于解析和操作HTML和XML文档,但也可以用于生成HTML内容。
安装BeautifulSoup
首先,你需要安装BeautifulSoup库:
pip install beautifulsoup4
生成HTML内容
使用BeautifulSoup生成HTML内容:
from bs4 import BeautifulSoup
创建一个BeautifulSoup对象
soup = BeautifulSoup('<html></html>', 'html.parser')
创建head和body标签
head = soup.new_tag('head')
body = soup.new_tag('body')
soup.html.append(head)
soup.html.append(body)
添加title标签
title = soup.new_tag('title')
title.string = "Sample HTML Page"
head.append(title)
添加h1和p标签
h1 = soup.new_tag('h1')
h1.string = "Hello, World!"
body.append(h1)
p = soup.new_tag('p')
p.string = "This is a sample HTML page generated using Python and BeautifulSoup."
body.append(p)
输出HTML内容
html_content = str(soup)
写入HTML文件
with open("output_beautifulsoup.html", "w") as file:
file.write(html_content)
四、使用Flask生成HTML页面
Flask是一个轻量级的Web框架,适用于构建Web应用程序。你可以使用Flask来生成和渲染HTML页面。
安装Flask
首先,你需要安装Flask库:
pip install Flask
创建Flask应用
创建一个Flask应用,定义路由并渲染HTML模板:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html', title="Sample HTML Page", heading="Hello, World!", message="This is a sample HTML page generated using Flask.")
if __name__ == '__main__':
app.run(debug=True)
创建HTML模板
创建一个HTML模板文件(例如templates/index.html
):
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ message }}</p>
</body>
</html>
五、使用Django生成HTML页面
Django是一个功能强大的Web框架,适用于构建复杂的Web应用程序。你可以使用Django来生成和渲染HTML页面。
安装Django
首先,你需要安装Django库:
pip install Django
创建Django项目和应用
创建一个Django项目和应用:
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
配置Django项目
编辑myproject/settings.py
文件,添加myapp
到INSTALLED_APPS
:
INSTALLED_APPS = [
...
'myapp',
]
定义视图
编辑myapp/views.py
文件,定义一个视图函数:
from django.shortcuts import render
def index(request):
return render(request, 'index.html', {'title': "Sample HTML Page", 'heading': "Hello, World!", 'message': "This is a sample HTML page generated using Django."})
配置URL
编辑myproject/urls.py
文件,配置URL路由:
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index, name='index'),
]
创建HTML模板
创建一个HTML模板文件(例如myapp/templates/index.html
):
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ message }}</p>
</body>
</html>
运行Django服务器
运行Django开发服务器:
python manage.py runserver
然后在浏览器中访问http://127.0.0.1:8000/
,你将看到生成的HTML页面。
六、使用WeasyPrint生成PDF
WeasyPrint是一个将HTML和CSS转换为PDF的工具。你可以使用WeasyPrint生成PDF文件。
安装WeasyPrint
首先,你需要安装WeasyPrint库:
pip install WeasyPrint
生成PDF文件
使用WeasyPrint生成PDF文件:
from weasyprint import HTML
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Sample PDF Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a sample PDF page generated using WeasyPrint.</p>
</body>
</html>
"""
生成PDF文件
HTML(string=html_content).write_pdf("output.pdf")
七、使用Pandas生成HTML表格
Pandas是一个强大的数据处理库,你可以使用Pandas生成HTML表格。
安装Pandas
首先,你需要安装Pandas库:
pip install pandas
生成HTML表格
使用Pandas生成HTML表格:
import pandas as pd
创建数据
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
创建DataFrame
df = pd.DataFrame(data)
生成HTML表格
html_table = df.to_html()
创建并写入HTML文件
html_content = f"""
<!DOCTYPE html>
<html>
<head>
<title>Sample HTML Table</title>
</head>
<body>
<h1>Sample HTML Table</h1>
{html_table}
</body>
</html>
"""
with open("output_table.html", "w") as file:
file.write(html_content)
八、使用Plotly生成交互式图表
Plotly是一个用于绘制交互式图表的库。你可以使用Plotly生成交互式图表并将其嵌入HTML文件。
安装Plotly
首先,你需要安装Plotly库:
pip install plotly
生成交互式图表
使用Plotly生成交互式图表:
import plotly.express as px
创建示例数据
df = px.data.iris()
创建交互式图表
fig = px.scatter(df, x='sepal_width', y='sepal_length', color='species')
生成HTML文件
fig.write_html("output_plot.html")
九、使用Matplotlib生成静态图表
Matplotlib是一个用于绘制静态图表的库。你可以使用Matplotlib生成静态图表并将其嵌入HTML文件。
安装Matplotlib
首先,你需要安装Matplotlib库:
pip install matplotlib
生成静态图表
使用Matplotlib生成静态图表:
import matplotlib.pyplot as plt
创建示例数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
创建静态图表
plt.plot(x, y)
plt.title('Sample Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
保存图表为图像文件
plt.savefig('plot.png')
创建并写入HTML文件
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Sample Plot</title>
</head>
<body>
<h1>Sample Plot</h1>
<img src="plot.png" alt="Sample Plot">
</body>
</html>
"""
with open("output_plot.html", "w") as file:
file.write(html_content)
十、使用Dash构建数据应用
Dash是一个用于构建数据应用的框架。你可以使用Dash构建交互式数据应用并生成HTML页面。
安装Dash
首先,你需要安装Dash库:
pip install dash
创建Dash应用
创建一个Dash应用,定义布局并生成HTML页面:
import dash
import dash_core_components as dcc
import dash_html_components as html
from dash.dependencies import Input, Output
创建Dash应用
app = dash.Dash(__name__)
定义应用布局
app.layout = html.Div([
html.H1('Sample Dash Application'),
dcc.Input(id='input-box', type='text', value='Hello, Dash!'),
html.Button('Submit', id='button'),
html.Div(id='output')
])
定义回调函数
@app.callback(
Output('output', 'children'),
[Input('button', 'n_clicks')],
[dash.dependencies.State('input-box', 'value')]
)
def update_output(n_clicks, value):
return f'You have entered: {value}'
运行应用
if __name__ == '__main__':
app.run_server(debug=True)
总结
以上介绍了多种使用Python生成和写入HTML文件的方法,包括使用内置文件操作功能、模板引擎Jinja2、BeautifulSoup库、Flask、Django、WeasyPrint、Pandas、Plotly、Matplotlib和Dash。每种方法都有其适用的场景和优缺点,选择合适的方法可以提高开发效率和代码质量。希望本文对你了解和使用Python生成HTML文件有所帮助。
相关问答FAQs:
如何使用Python生成HTML文件?
可以使用Python的内置文件操作来创建HTML文件。首先,打开一个文件并以写模式('w')创建新文件。接着,写入HTML内容,比如基本的HTML结构、标题、段落等。最后,记得关闭文件以保存更改。示例代码如下:
html_content = '''<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</body>
</html>'''
with open('mypage.html', 'w') as file:
file.write(html_content)
Python中有哪些库可以帮助生成HTML?
Python有多个库可以简化HTML生成的过程,比如Flask和Django用于Web开发,Jinja2是一个模板引擎,可以帮助动态生成HTML内容。使用这些库,可以更灵活地处理用户输入和数据展示,同时提高开发效率。
如何在Python中使用模板引擎生成动态HTML内容?
可以使用Jinja2等模板引擎来生成动态HTML。首先,定义一个HTML模板文件,其中包含占位符。接着,在Python代码中加载模板,传入所需的数据,最终渲染出完整的HTML。例如:
from jinja2 import Environment, FileSystemLoader
env = Environment(loader=FileSystemLoader('templates'))
template = env.get_template('template.html')
data = {'title': 'My Page', 'content': 'This is a dynamic paragraph.'}
output = template.render(data)
with open('output.html', 'w') as file:
file.write(output)
这种方式使得内容更新更加方便,且HTML结构与数据逻辑分离。
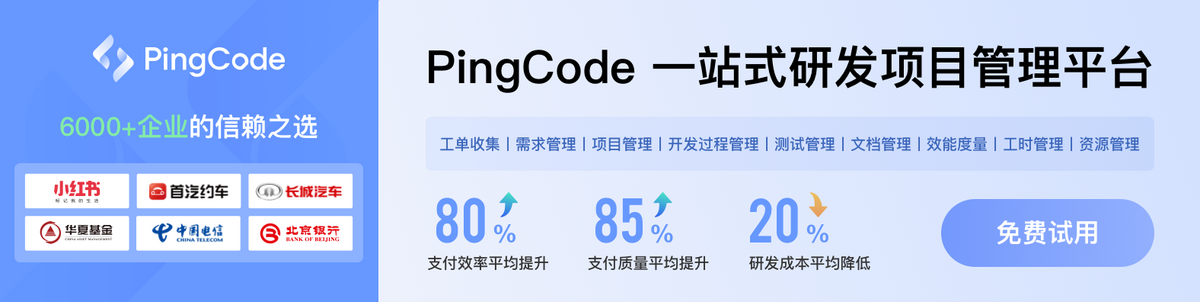