要用Python写工具类,你需要掌握面向对象编程、代码重用、模块化设计、代码清洁等方面的知识。、首先,你需要定义一个类,然后在类中定义一些实用的方法,这些方法可以是静态方法或实例方法、工具类通常不需要维护状态,所以大多数方法可以使用@staticmethod装饰器。接下来,我将详细解释如何创建一个Python工具类,并提供一些示例代码。
一、面向对象编程基础
在Python中,面向对象编程(OOP)是一种编程范式,它使用“类”和“对象”来创建模型以实现代码的组织、重用和封装。以下是一些基本概念:
1、类和对象
- 类(Class):类是创建对象的蓝图或模板。它定义了一些属性和方法,供对象实例化时使用。
- 对象(Object):对象是类的实例。每个对象都有其自己的属性值。
2、方法
- 实例方法:第一个参数是self,表示实例本身。
- 类方法:使用@classmethod装饰,第一个参数是cls,表示类本身。
- 静态方法:使用@staticmethod装饰,不需要任何默认参数。
3、继承
继承允许一个类继承另一个类的属性和方法,从而实现代码重用。
二、定义工具类
工具类通常包含一组静态方法,这些方法可以直接通过类名调用,而不需要实例化类。下面是一个简单的工具类示例:
class StringUtils:
@staticmethod
def to_uppercase(s):
return s.upper()
@staticmethod
def to_lowercase(s):
return s.lower()
@staticmethod
def reverse_string(s):
return s[::-1]
三、使用工具类
你可以直接调用工具类中的静态方法,而不需要创建类的实例:
print(StringUtils.to_uppercase('hello')) # 输出: HELLO
print(StringUtils.to_lowercase('WORLD')) # 输出: world
print(StringUtils.reverse_string('Python')) # 输出: nohtyP
四、扩展工具类
工具类可以包含各种各样的实用方法,以下是一些常见的扩展:
1、字符串工具
class StringUtils:
@staticmethod
def to_uppercase(s):
return s.upper()
@staticmethod
def to_lowercase(s):
return s.lower()
@staticmethod
def reverse_string(s):
return s[::-1]
@staticmethod
def is_palindrome(s):
return s == s[::-1]
@staticmethod
def count_vowels(s):
vowels = 'aeiouAEIOU'
return sum(1 for char in s if char in vowels)
2、数学工具
class MathUtils:
@staticmethod
def factorial(n):
if n == 0:
return 1
return n * MathUtils.factorial(n-1)
@staticmethod
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
elif n == 2:
return [0, 1]
fibs = [0, 1]
for i in range(2, n):
fibs.append(fibs[-1] + fibs[-2])
return fibs
@staticmethod
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n0.5) + 1):
if n % i == 0:
return False
return True
五、文件操作工具
文件操作是编程中常见的任务,工具类可以帮助简化这些操作:
import os
class FileUtils:
@staticmethod
def read_file(filepath):
with open(filepath, 'r') as file:
return file.read()
@staticmethod
def write_file(filepath, content):
with open(filepath, 'w') as file:
file.write(content)
@staticmethod
def file_exists(filepath):
return os.path.exists(filepath)
@staticmethod
def delete_file(filepath):
if FileUtils.file_exists(filepath):
os.remove(filepath)
六、日期和时间工具
日期和时间处理也是编程中常见的任务,工具类可以包含一些常用的日期时间操作:
from datetime import datetime, timedelta
class DateTimeUtils:
@staticmethod
def current_datetime():
return datetime.now()
@staticmethod
def format_datetime(dt, format_string):
return dt.strftime(format_string)
@staticmethod
def add_days(dt, days):
return dt + timedelta(days=days)
@staticmethod
def days_between(date1, date2):
delta = date2 - date1
return delta.days
七、网络请求工具
网络请求是编程中的另一个常见任务,工具类可以简化这些请求操作:
import requests
class NetworkUtils:
@staticmethod
def get(url, params=None, headers=None):
response = requests.get(url, params=params, headers=headers)
return response.json()
@staticmethod
def post(url, data=None, json=None, headers=None):
response = requests.post(url, data=data, json=json, headers=headers)
return response.json()
@staticmethod
def download_file(url, filepath):
response = requests.get(url, stream=True)
with open(filepath, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
八、加密和解密工具
在处理敏感信息时,加密和解密是非常重要的,工具类可以包含一些常用的加密和解密方法:
import hashlib
import base64
class CryptoUtils:
@staticmethod
def md5_hash(s):
return hashlib.md5(s.encode()).hexdigest()
@staticmethod
def sha256_hash(s):
return hashlib.sha256(s.encode()).hexdigest()
@staticmethod
def base64_encode(s):
return base64.b64encode(s.encode()).decode()
@staticmethod
def base64_decode(s):
return base64.b64decode(s.encode()).decode()
九、配置管理工具
在实际项目中,配置管理是非常重要的,工具类可以帮助管理配置文件:
import json
class ConfigUtils:
@staticmethod
def load_config(filepath):
with open(filepath, 'r') as file:
return json.load(file)
@staticmethod
def save_config(filepath, config):
with open(filepath, 'w') as file:
json.dump(config, file, indent=4)
十、日志工具
日志记录是软件开发中的重要部分,工具类可以帮助简化日志记录操作:
import logging
class LogUtils:
@staticmethod
def setup_logger(name, log_file, level=logging.INFO):
formatter = logging.Formatter('%(asctime)s %(levelname)s %(message)s')
handler = logging.FileHandler(log_file)
handler.setFormatter(formatter)
logger = logging.getLogger(name)
logger.setLevel(level)
logger.addHandler(handler)
return logger
使用示例
logger = LogUtils.setup_logger('my_logger', 'my_log.log')
logger.info('This is an info message')
logger.error('This is an error message')
十一、数据处理工具
在数据科学和机器学习领域,数据处理是非常重要的任务,工具类可以包含一些常用的数据处理方法:
import pandas as pd
class DataUtils:
@staticmethod
def load_csv(filepath):
return pd.read_csv(filepath)
@staticmethod
def save_csv(df, filepath):
df.to_csv(filepath, index=False)
@staticmethod
def filter_data(df, column, value):
return df[df[column] == value]
@staticmethod
def group_data(df, column):
return df.groupby(column).mean()
十二、图像处理工具
图像处理在计算机视觉领域非常重要,工具类可以包含一些常用的图像处理方法:
from PIL import Image
class ImageUtils:
@staticmethod
def open_image(filepath):
return Image.open(filepath)
@staticmethod
def save_image(image, filepath):
image.save(filepath)
@staticmethod
def resize_image(image, size):
return image.resize(size)
@staticmethod
def convert_to_grayscale(image):
return image.convert('L')
十三、数据库工具
数据库操作是Web开发和数据科学中常见的任务,工具类可以包含一些常用的数据库操作方法:
import sqlite3
class DatabaseUtils:
@staticmethod
def connect(db_name):
return sqlite3.connect(db_name)
@staticmethod
def execute_query(conn, query, params=None):
cursor = conn.cursor()
if params:
cursor.execute(query, params)
else:
cursor.execute(query)
conn.commit()
return cursor
@staticmethod
def fetch_all(cursor):
return cursor.fetchall()
@staticmethod
def fetch_one(cursor):
return cursor.fetchone()
使用示例
conn = DatabaseUtils.connect('my_database.db')
cursor = DatabaseUtils.execute_query(conn, 'SELECT * FROM my_table')
results = DatabaseUtils.fetch_all(cursor)
十四、正则表达式工具
正则表达式是处理字符串的强大工具,工具类可以包含一些常用的正则表达式操作方法:
import re
class RegexUtils:
@staticmethod
def match_pattern(pattern, string):
return re.match(pattern, string)
@staticmethod
def search_pattern(pattern, string):
return re.search(pattern, string)
@staticmethod
def find_all(pattern, string):
return re.findall(pattern, string)
@staticmethod
def replace_pattern(pattern, repl, string):
return re.sub(pattern, repl, string)
十五、系统操作工具
系统操作是开发中常见的任务,工具类可以包含一些常用的系统操作方法:
import os
import shutil
class SystemUtils:
@staticmethod
def get_current_directory():
return os.getcwd()
@staticmethod
def list_directory(path='.'):
return os.listdir(path)
@staticmethod
def create_directory(path):
os.makedirs(path, exist_ok=True)
@staticmethod
def delete_directory(path):
shutil.rmtree(path)
结论
创建一个Python工具类可以极大地提高代码的可读性、可维护性和可重用性。通过将常用的操作封装到工具类中,你可以在不同项目中轻松地复用这些代码。本文介绍了如何创建各种类型的工具类,包括字符串工具、数学工具、文件操作工具、日期时间工具、网络请求工具、加密和解密工具、配置管理工具、日志工具、数据处理工具、图像处理工具、数据库工具、正则表达式工具和系统操作工具。希望这些示例能帮助你在实际项目中创建和使用工具类。
相关问答FAQs:
如何在Python中创建一个工具类以实现代码重用?
在Python中,创建工具类的关键在于定义一个包含静态方法的类,这些方法可以被多次调用而无需实例化类。通常,这些方法被用于执行特定的功能,例如数据处理、文件操作等。使用@staticmethod
装饰器可以方便地定义静态方法,从而使工具类更加简洁易用。
在工具类中实现哪些常用功能是比较实用的?
工具类可以用于多种常用功能,比如字符串处理、日期时间格式化、网络请求、文件读取和写入等。例如,你可以创建一个工具类StringUtils
,其中包含方法如reverse_string
、is_palindrome
等,帮助用户快速处理字符串相关任务。
如何保证我的工具类在不同项目中都能正常工作?
为了确保工具类在多个项目中都能正常工作,建议遵循一些最佳实践。首先,保持工具类功能的独立性,避免与特定业务逻辑耦合。其次,编写清晰的文档和示例,以便其他开发者可以轻松理解和使用你的工具类。最后,进行充分的单元测试,以确保所有功能在不同情况下都能正常运行。
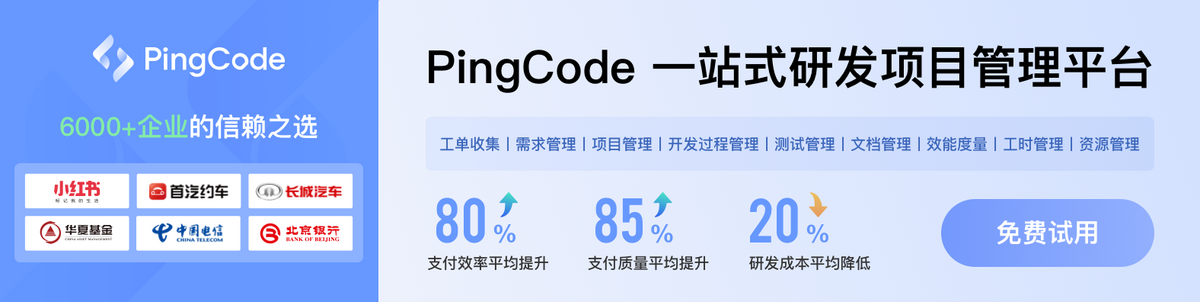