要下载 .tar.gz
文件,可以使用 Python 中的多种库和工具,如 requests
、urllib
、wget
等。其中使用 requests 库下载、urllib 库下载、wget 库下载和使用 shutil 解压缩是一些常见的方法。下面将详细介绍其中的一种方法,即使用 requests
库来下载并解压 .tar.gz
文件。
使用 requests 库下载
requests
是一个非常流行的 HTTP 库,可以用来发送 HTTP 请求。使用它下载文件非常简单。下面是一个示例代码:
import requests
url = 'http://example.com/somefile.tar.gz'
response = requests.get(url)
with open('somefile.tar.gz', 'wb') as file:
file.write(response.content)
这个代码将从指定的 URL 下载 .tar.gz
文件并保存到本地文件系统中。接下来,我们将介绍如何使用 shutil
库来解压缩这个 .tar.gz
文件。
使用 shutil 解压缩
Python 标准库 shutil
提供了许多文件操作功能,包括解压缩。下面是一个示例代码:
import tarfile
with tarfile.open('somefile.tar.gz', 'r:gz') as tar:
tar.extractall(path='destination_folder')
这个代码将解压缩 .tar.gz
文件到指定的目标文件夹中。接下来,我们将详细介绍其他方法和一些注意事项。
一、使用 urllib 库下载
urllib
是 Python 标准库的一部分,可以用来处理 URL 和发送 HTTP 请求。以下是使用 urllib
下载 .tar.gz
文件的示例代码:
import urllib.request
url = 'http://example.com/somefile.tar.gz'
urllib.request.urlretrieve(url, 'somefile.tar.gz')
这个代码将从指定的 URL 下载 .tar.gz
文件并保存到本地文件系统中。urllib.request.urlretrieve
函数会直接将文件保存到指定的路径。
二、使用 wget 库下载
wget
是一个命令行工具,但也有 Python 版本。可以用来轻松下载文件。首先,你需要安装 wget
库:
pip install wget
然后,使用以下代码下载 .tar.gz
文件:
import wget
url = 'http://example.com/somefile.tar.gz'
filename = wget.download(url)
这个代码将从指定的 URL 下载 .tar.gz
文件并保存到当前工作目录中。
三、使用 requests 库下载并解压缩
结合 requests
和 tarfile
库,我们可以实现下载并解压缩 .tar.gz
文件的功能。下面是一个完整的示例代码:
import requests
import tarfile
import os
def download_file(url, destination):
response = requests.get(url, stream=True)
with open(destination, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
file.write(chunk)
def extract_tar_gz(file_path, destination):
with tarfile.open(file_path, 'r:gz') as tar:
tar.extractall(path=destination)
url = 'http://example.com/somefile.tar.gz'
destination = 'somefile.tar.gz'
extract_path = 'destination_folder'
download_file(url, destination)
extract_tar_gz(destination, extract_path)
删除下载的压缩文件
os.remove(destination)
这个代码包含两个函数:download_file
用于下载文件,extract_tar_gz
用于解压缩文件。最后,我们调用这两个函数来完成下载并解压缩操作。
四、其他注意事项
- 网络连接问题:下载文件时可能会遇到网络连接问题,如超时或连接被拒绝。可以在代码中添加异常处理来应对这些问题。
- 文件完整性:下载文件后,可以使用哈希算法(如 MD5 或 SHA256)验证文件的完整性,以确保文件没有被篡改或损坏。
- 安全性:从不可信的来源下载文件可能存在安全风险。确保仅从可信的来源下载文件,并在下载后进行必要的安全检查。
五、完整的示例代码
为了帮助您更好地理解,我们将所有内容整合到一个完整的示例代码中:
import requests
import tarfile
import os
import hashlib
def download_file(url, destination):
response = requests.get(url, stream=True)
with open(destination, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
file.write(chunk)
def extract_tar_gz(file_path, destination):
with tarfile.open(file_path, 'r:gz') as tar:
tar.extractall(path=destination)
def verify_file(file_path, expected_hash):
sha256 = hashlib.sha256()
with open(file_path, 'rb') as file:
while chunk := file.read(8192):
sha256.update(chunk)
return sha256.hexdigest() == expected_hash
url = 'http://example.com/somefile.tar.gz'
destination = 'somefile.tar.gz'
extract_path = 'destination_folder'
expected_hash = 'your_expected_sha256_hash_here'
download_file(url, destination)
if verify_file(destination, expected_hash):
extract_tar_gz(destination, extract_path)
print('File downloaded and extracted successfully.')
else:
print('File verification failed.')
删除下载的压缩文件
os.remove(destination)
这个代码不仅下载并解压缩 .tar.gz
文件,还验证文件的完整性。如果文件验证失败,则不会解压缩文件。
六、总结
使用 Python 下载和解压缩 .tar.gz
文件可以通过多种方法实现,包括 requests
、urllib
和 wget
库。下载文件后,可以使用 tarfile
库进行解压缩,并使用哈希算法验证文件的完整性。在实际应用中,选择适合自己的方法和工具,并根据具体需求进行调整和优化。希望这篇文章能帮助您更好地理解和实现 Python 下载 .tar.gz
文件的功能。
相关问答FAQs:
如何在Python中下载tar.gz文件?
在Python中,可以使用内置的urllib
库或第三方库如requests
来下载tar.gz文件。使用requests
库的示例代码如下:
import requests
url = 'http://example.com/file.tar.gz' # 替换为实际的tar.gz文件链接
response = requests.get(url)
with open('file.tar.gz', 'wb') as file:
file.write(response.content)
这种方法简单直接,适合大多数下载需求。
下载tar.gz文件后如何解压?
解压tar.gz文件可以使用Python的tarfile
库。以下是解压文件的示例代码:
import tarfile
with tarfile.open('file.tar.gz', 'r:gz') as tar:
tar.extractall(path='destination_folder') # 替换为解压目标文件夹
确保目标文件夹存在,解压后的文件将保存在指定位置。
在下载tar.gz文件时,如何处理异常情况?
在下载文件时,可能会遇到网络问题或链接失效等情况。可以通过添加异常处理来确保程序的健壮性。例如:
import requests
url = 'http://example.com/file.tar.gz'
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('file.tar.gz', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载过程中发生错误: {e}")
这种方式能帮助你更好地管理下载过程中的潜在问题。
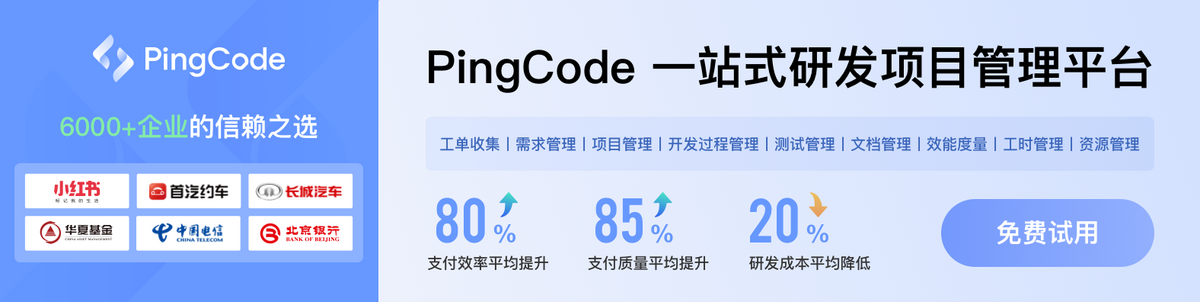