在Python中,转文件编码的方法主要有使用内置的open
函数、codecs
模块和chardet
模块等。其中,使用open
函数和codecs
模块是最常见的方法,而chardet
模块则用于自动检测文件编码。下面将详细介绍这些方法,并给出具体的实现代码。
一、使用内置的open
函数
Python 提供了内置的open
函数来读取和写入文件,通过设置不同的编码参数,可以实现文件编码的转换。
读取文件并转换编码
首先,使用指定编码读取文件内容,然后以目标编码写入新的文件。
def convert_file_encoding(input_file, output_file, input_encoding, output_encoding):
with open(input_file, 'r', encoding=input_encoding) as f:
content = f.read()
with open(output_file, 'w', encoding=output_encoding) as f:
f.write(content)
示例
convert_file_encoding('input.txt', 'output.txt', 'utf-8', 'gbk')
以上代码读取了input.txt
文件,并将其编码从utf-8
转换为gbk
,然后写入output.txt
。
二、使用codecs
模块
codecs
模块是Python的标准库,专门用于处理编码转换。它提供了更丰富的编码方式和处理方法。
使用codecs.open
函数
codecs.open
函数可以指定文件的编码,这样在读取和写入时就可以自动进行转换。
import codecs
def convert_file_encoding(input_file, output_file, input_encoding, output_encoding):
with codecs.open(input_file, 'r', encoding=input_encoding) as f:
content = f.read()
with codecs.open(output_file, 'w', encoding=output_encoding) as f:
f.write(content)
示例
convert_file_encoding('input.txt', 'output.txt', 'utf-8', 'gbk')
三、使用chardet
模块检测编码
在某些情况下,文件的编码未知,此时可以使用chardet
模块来自动检测文件编码,然后再进行转换。
安装chardet
模块
在使用chardet
模块之前,需要先安装它。可以使用以下命令进行安装:
pip install chardet
自动检测并转换编码
使用chardet
模块检测文件编码,然后进行转换。
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as f:
raw_data = f.read()
result = chardet.detect(raw_data)
return result['encoding']
def convert_file_encoding(input_file, output_file, output_encoding):
input_encoding = detect_encoding(input_file)
with open(input_file, 'r', encoding=input_encoding) as f:
content = f.read()
with open(output_file, 'w', encoding=output_encoding) as f:
f.write(content)
示例
convert_file_encoding('input.txt', 'output.txt', 'utf-8')
以上代码先使用chardet
模块检测文件的原始编码,然后将其转换为目标编码。
四、处理大文件
对于大文件,读取整个文件内容可能会导致内存不足的问题。此时可以逐行读取和写入,避免一次性加载整个文件。
def convert_file_encoding_line_by_line(input_file, output_file, input_encoding, output_encoding):
with open(input_file, 'r', encoding=input_encoding) as infile, open(output_file, 'w', encoding=output_encoding) as outfile:
for line in infile:
outfile.write(line)
示例
convert_file_encoding_line_by_line('input.txt', 'output.txt', 'utf-8', 'gbk')
五、批量处理多个文件
如果需要转换多个文件的编码,可以使用循环来遍历文件列表,并调用转换函数。
import os
def batch_convert_encoding(file_list, input_encoding, output_encoding, output_dir):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for input_file in file_list:
output_file = os.path.join(output_dir, os.path.basename(input_file))
convert_file_encoding(input_file, output_file, input_encoding, output_encoding)
示例
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
batch_convert_encoding(file_list, 'utf-8', 'gbk', 'output_dir')
以上代码创建了一个输出目录,并将文件列表中的每个文件编码转换后保存到该目录中。
六、处理特殊字符和异常
在实际操作中,可能会遇到文件中包含特殊字符或者编码转换过程中出现异常的情况。可以通过捕获异常并处理特殊字符来增强代码的鲁棒性。
def convert_file_encoding_with_error_handling(input_file, output_file, input_encoding, output_encoding):
try:
with open(input_file, 'r', encoding=input_encoding, errors='ignore') as f:
content = f.read()
with open(output_file, 'w', encoding=output_encoding, errors='ignore') as f:
f.write(content)
except Exception as e:
print(f"Error converting {input_file}: {e}")
示例
convert_file_encoding_with_error_handling('input.txt', 'output.txt', 'utf-8', 'gbk')
以上代码使用errors='ignore'
参数忽略了读取和写入过程中可能出现的编码错误,并捕获了异常进行处理。
七、结合多种方法
在实际应用中,可能需要结合多种方法来处理复杂的编码转换需求。例如,先使用chardet
检测编码,再使用逐行读取的方法进行转换。
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as f:
raw_data = f.read()
result = chardet.detect(raw_data)
return result['encoding']
def convert_file_encoding_line_by_line_with_detection(input_file, output_file, output_encoding):
input_encoding = detect_encoding(input_file)
with open(input_file, 'r', encoding=input_encoding) as infile, open(output_file, 'w', encoding=output_encoding) as outfile:
for line in infile:
outfile.write(line)
示例
convert_file_encoding_line_by_line_with_detection('input.txt', 'output.txt', 'utf-8')
以上代码先检测文件的原始编码,然后逐行读取和写入,避免大文件导致的内存问题。
八、总结
通过上述方法,可以灵活地在Python中实现文件编码的转换。使用内置的open
函数、codecs
模块和chardet
模块是常见的解决方案,具体选择哪种方法可以根据实际需求和文件大小进行判断。在处理过程中,注意捕获异常和处理特殊字符,以确保代码的鲁棒性和可靠性。
相关问答FAQs:
如何判断一个文件的当前编码?
在Python中,可以使用chardet
库来检测文件的编码。通过读取文件的一部分内容,chardet
会返回一个可能的编码类型及其置信度。您可以安装该库并使用以下代码示例:
import chardet
with open('yourfile.txt', 'rb') as f:
result = chardet.detect(f.read(10000))
print(result)
这段代码会输出检测到的编码和置信度,可以帮助您了解文件的当前编码格式。
如何将文件从一种编码转换为另一种编码?
可以使用Python的内置功能来实现编码转换。以下是一个示例,演示如何将UTF-8编码的文件转换为ISO-8859-1编码:
with open('input_file.txt', 'r', encoding='utf-8') as infile:
content = infile.read()
with open('output_file.txt', 'w', encoding='iso-8859-1') as outfile:
outfile.write(content)
这段代码读取一个UTF-8编码的文件,并将内容写入一个新的ISO-8859-1编码的文件中。
使用Python处理文件编码转换时有哪些常见问题?
在进行文件编码转换时,可能会遇到一些常见问题,例如:
- 编码错误:如果源文件的实际编码与您指定的编码不匹配,可能会导致读取或写入错误。
- 字符丢失:某些编码可能无法表示特定字符,导致数据丢失或变形。
为避免这些问题,建议在转换前确认源文件的编码,并在读取或写入时使用适当的错误处理选项,例如errors='ignore'
或errors='replace'
。
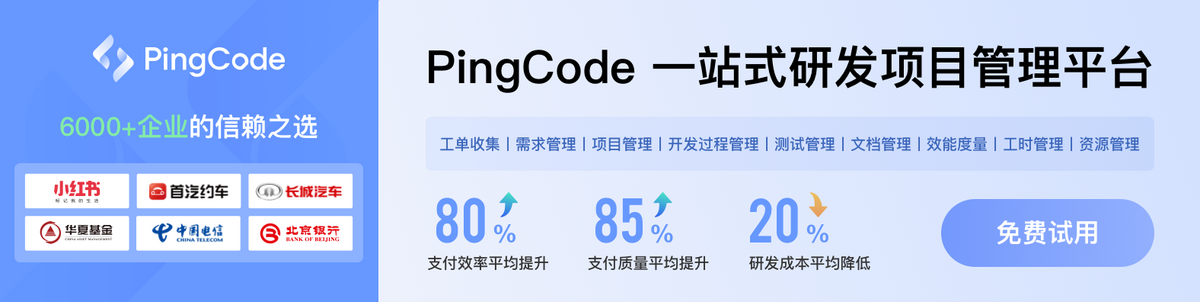