Python可以通过使用随机数生成器、运算符和变量来随机生成数学式。这些数学式可以包括简单的加减乘除运算、复杂的多项式或函数。 其中一种方法是使用random
模块生成随机数和运算符,然后将这些元素组合成一个数学表达式。接下来详细介绍一种简单的方法,即通过生成随机数和随机选择运算符来创建简单的数学式。
详细描述:
使用Python生成随机数学式的基础方法包括以下几个步骤:
- 导入
random
模块。 - 生成随机的数字和运算符。
- 将数字和运算符组合成一个数学表达式。
例如,可以使用random.randint()
生成随机整数,使用random.choice()
从运算符列表中选择一个随机运算符,然后将这些元素组合成一个字符串表示的数学式。
以下是一个简单的示例代码:
import random
def generate_random_expression():
operators = ['+', '-', '*', '/']
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
operator = random.choice(operators)
expression = f"{num1} {operator} {num2}"
return expression
print(generate_random_expression())
这个函数会生成一个随机的数学表达式,例如3 + 7
或5 * 2
。
一、导入必要的模块
Python自带的random
模块包含了各种生成随机数和选择随机元素的方法,是生成随机数学式的核心工具。此外,还可以使用numpy
模块来生成更复杂的随机数和进行数学运算。
import random
import numpy as np
二、生成随机数
生成随机数是创建随机数学式的第一步。可以使用random.randint()
生成指定范围内的随机整数,或者使用numpy
生成各种类型的随机数。
# 使用random模块生成随机整数
num1 = random.randint(1, 100)
num2 = random.randint(1, 100)
使用numpy模块生成随机浮点数
num3 = np.random.uniform(1, 10)
num4 = np.random.normal(0, 1)
三、选择随机运算符
使用random.choice()
从一个包含所有可能运算符的列表中随机选择一个运算符,例如加号、减号、乘号和除号。
operators = ['+', '-', '*', '/']
operator = random.choice(operators)
四、组合数字和运算符生成表达式
将生成的随机数和随机运算符组合成一个数学表达式。可以使用字符串格式化来实现这一点。
expression = f"{num1} {operator} {num2}"
print(expression)
五、评估表达式
为了验证生成的表达式是否正确,可以使用Python的eval()
函数来计算表达式的值。这一步虽然简单,但需要注意安全性,eval()
函数会执行传入的字符串,因此在实际应用中应谨慎使用。
result = eval(expression)
print(f"The result of the expression {expression} is {result}")
六、生成复杂的数学式
上述方法适用于生成简单的二元运算表达式。如果需要生成更复杂的数学式,可以递归地生成嵌套的表达式或使用更复杂的生成策略。
def generate_complex_expression(depth=2):
operators = ['+', '-', '*', '/']
if depth == 0:
return str(random.randint(1, 10))
else:
left_expr = generate_complex_expression(depth - 1)
right_expr = generate_complex_expression(depth - 1)
operator = random.choice(operators)
return f"({left_expr} {operator} {right_expr})"
complex_expression = generate_complex_expression()
print(complex_expression)
print(eval(complex_expression))
七、生成特定类型的数学式
除了简单的算术表达式,还可以生成多项式、三角函数等特定类型的数学式。以下是生成一个随机多项式的示例:
def generate_polynomial(degree=2):
terms = []
for i in range(degree + 1):
coefficient = random.randint(1, 10)
if i == 0:
terms.append(f"{coefficient}")
else:
terms.append(f"{coefficient}*x^{i}")
return " + ".join(terms)
polynomial = generate_polynomial(3)
print(f"The generated polynomial is: {polynomial}")
八、生成带有变量的表达式
可以生成包含变量的数学式,并通过替换变量值来计算表达式。例如生成一个含变量x
的表达式:
def generate_expression_with_variable():
operators = ['+', '-', '*', '/']
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
operator = random.choice(operators)
expression = f"{num1} {operator} x"
return expression
expression_with_variable = generate_expression_with_variable()
print(f"The generated expression with variable is: {expression_with_variable}")
替换变量并计算表达式的值
x = 5
expression_evaluated = expression_with_variable.replace('x', str(x))
print(f"The result of the expression {expression_with_variable} with x={x} is {eval(expression_evaluated)}")
九、生成函数表达式
还可以生成带有函数的数学式,例如三角函数、指数函数等。使用numpy
模块可以方便地生成这些函数表达式。
def generate_function_expression():
functions = ['np.sin', 'np.cos', 'np.exp', 'np.log']
num = random.uniform(1, 10)
function = random.choice(functions)
expression = f"{function}({num})"
return expression
function_expression = generate_function_expression()
print(f"The generated function expression is: {function_expression}")
print(f"The result of the function expression is: {eval(function_expression)}")
十、生成带有约束条件的表达式
有时需要生成带有特定约束条件的数学式,例如特定范围内的结果或特定形式的表达式。可以通过检查生成的表达式是否满足约束条件来实现这一点。
def generate_expression_with_constraints(min_value, max_value):
while True:
expression = generate_random_expression()
result = eval(expression)
if min_value <= result <= max_value:
return expression, result
expression_with_constraints, result = generate_expression_with_constraints(10, 20)
print(f"The generated expression with constraints is: {expression_with_constraints}")
print(f"The result of the expression is: {result}")
十一、生成随机矩阵和线性代数表达式
在科学计算和工程应用中,生成随机矩阵和线性代数表达式也是常见的需求。可以使用numpy
模块生成随机矩阵,并进行矩阵运算。
def generate_random_matrix(rows, cols):
return np.random.randint(1, 10, size=(rows, cols))
matrix_a = generate_random_matrix(3, 3)
matrix_b = generate_random_matrix(3, 3)
matrix_expression = f"A + B"
print(f"Matrix A:\n{matrix_a}")
print(f"Matrix B:\n{matrix_b}")
print(f"The result of the matrix expression {matrix_expression} is:\n{matrix_a + matrix_b}")
十二、生成随机微积分表达式
生成随机的微积分表达式可以用于数学教学和研究。可以使用sympy
模块生成符号表达式,并进行微积分运算。
import sympy as sp
def generate_random_calculus_expression():
x = sp.symbols('x')
coefficients = [random.randint(1, 5) for _ in range(3)]
expression = sum(coef * xi for i, coef in enumerate(coefficients))
return expression
calculus_expression = generate_random_calculus_expression()
derivative = sp.diff(calculus_expression, 'x')
integral = sp.integrate(calculus_expression, 'x')
print(f"The generated calculus expression is: {calculus_expression}")
print(f"The derivative of the expression is: {derivative}")
print(f"The integral of the expression is: {integral}")
十三、生成随机数列和级数表达式
可以生成随机数列和级数表达式,用于研究数列收敛性和级数求和等问题。
def generate_random_series(length=5):
terms = [random.randint(1, 10) for _ in range(length)]
series_expression = " + ".join([f"{term}/n^{i+1}" for i, term in enumerate(terms)])
return series_expression
series_expression = generate_random_series()
print(f"The generated series expression is: {series_expression}")
十四、结合正则表达式生成随机数学式
正则表达式可以用来生成和验证随机数学式的格式。可以使用re
模块定义一个数学式的格式,并生成满足该格式的表达式。
import re
def generate_expression_with_regex(pattern):
while True:
expression = generate_random_expression()
if re.match(pattern, expression):
return expression
示例:生成一个加减法的数学式
pattern = r"\d+ [+|-] \d+"
expression_with_regex = generate_expression_with_regex(pattern)
print(f"The generated expression with regex is: {expression_with_regex}")
十五、生成随机逻辑表达式
生成随机逻辑表达式可以用于测试逻辑电路和布尔代数的应用。可以使用随机数生成器和逻辑运算符生成逻辑表达式。
def generate_random_logical_expression():
operators = ['and', 'or', 'not']
variables = ['A', 'B', 'C']
variable1 = random.choice(variables)
variable2 = random.choice(variables)
operator = random.choice(operators)
if operator == 'not':
expression = f"{operator} {variable1}"
else:
expression = f"{variable1} {operator} {variable2}"
return expression
logical_expression = generate_random_logical_expression()
print(f"The generated logical expression is: {logical_expression}")
十六、生成随机概率和统计表达式
在概率和统计学中,生成随机的概率和统计表达式可以用于模拟和分析数据。可以使用numpy
和scipy
模块生成这些表达式。
from scipy.stats import norm
def generate_random_probability_expression():
mu, sigma = random.uniform(0, 10), random.uniform(1, 5)
x = random.uniform(0, 10)
expression = f"norm.pdf({x}, {mu}, {sigma})"
return expression, norm.pdf(x, mu, sigma)
probability_expression, result = generate_random_probability_expression()
print(f"The generated probability expression is: {probability_expression}")
print(f"The result of the probability expression is: {result}")
十七、生成随机几何表达式
在几何学中,生成随机的几何表达式可以用于研究图形的性质和计算图形的面积、周长等。可以使用随机数生成器和几何公式生成这些表达式。
def generate_random_geometric_expression():
shapes = ['circle', 'rectangle', 'triangle']
shape = random.choice(shapes)
if shape == 'circle':
radius = random.uniform(1, 10)
expression = f"π * {radius}^2"
result = np.pi * radius2
elif shape == 'rectangle':
length = random.uniform(1, 10)
width = random.uniform(1, 10)
expression = f"{length} * {width}"
result = length * width
elif shape == 'triangle':
base = random.uniform(1, 10)
height = random.uniform(1, 10)
expression = f"0.5 * {base} * {height}"
result = 0.5 * base * height
return expression, result
geometric_expression, result = generate_random_geometric_expression()
print(f"The generated geometric expression is: {geometric_expression}")
print(f"The result of the geometric expression is: {result}")
十八、生成随机微分方程表达式
生成随机的微分方程表达式可以用于研究微分方程的解法和应用。可以使用sympy
模块生成符号微分方程,并求解这些方程。
def generate_random_differential_equation():
x = sp.symbols('x')
y = sp.Function('y')
coefficients = [random.randint(1, 5) for _ in range(3)]
equation = sum(coef * y(x).diff(x, i) for i, coef in enumerate(coefficients))
return equation
differential_equation = generate_random_differential_equation()
solution = sp.dsolve(differential_equation)
print(f"The generated differential equation is: {differential_equation}")
print(f"The solution of the differential equation is: {solution}")
十九、生成随机复数表达式
生成随机的复数表达式可以用于研究复数的性质和应用。可以使用随机数生成器和复数运算符生成这些表达式。
def generate_random_complex_expression():
real_part = random.uniform(0, 10)
imag_part = random.uniform(0, 10)
complex_number = complex(real_part, imag_part)
expression = f"{complex_number} + {complex_number}"
result = complex_number + complex_number
return expression, result
complex_expression, result = generate_random_complex_expression()
print(f"The generated complex expression is: {complex_expression}")
print(f"The result of the complex expression is: {result}")
二十、生成随机向量和矩阵表达式
生成随机的向量和矩阵表达式可以用于线性代数和多维数据分析。可以使用numpy
模块生成这些表达式,并进行向量和矩阵运算。
def generate_random_vector_expression():
vector_a = np.random.randint(1, 10, size=3)
vector_b = np.random.randint(1, 10, size=3)
expression = f"A . B"
result = np.dot(vector_a, vector_b)
return expression, result
vector_expression, result = generate_random_vector_expression()
print(f"The generated vector expression is: {vector_expression}")
print(f"The result of the vector expression is: {result}")
总结
通过使用随机数生成器、运算符和变量,可以在Python中生成各种类型的随机数学式。这些数学式可以用于教学、研究和应用中的模拟和分析。无论是简单的算术表达式、复杂的多项式、带有函数的表达式,还是几何、概率和统计表达式,都可以通过Python的random
、numpy
、sympy
等模块轻松生成。通过不断扩展和组合这些基本方法,可以生成满足不同需求的随机数学式。
相关问答FAQs:
如何使用Python生成简单的数学表达式?
可以使用Python的random
模块结合字符串格式化来生成随机的数学表达式。通过选择运算符和数字,可以创建简单的加法、减法、乘法和除法运算。下面是一个基本示例:
import random
def generate_expression():
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
operator = random.choice(['+', '-', '*', '/'])
expression = f"{num1} {operator} {num2}"
return expression
print(generate_expression())
这段代码会输出一个随机的数学表达式,例如“3 + 7”。
如何生成更复杂的数学式?
对于复杂的数学表达式,可以引入括号和更高的运算优先级。通过递归或循环的方式,可以构建多层表达式。以下是一个示例:
def generate_complex_expression(depth):
if depth == 0:
return str(random.randint(1, 10))
operator = random.choice(['+', '-', '*', '/'])
left = generate_complex_expression(depth - 1)
right = generate_complex_expression(depth - 1)
return f"({left} {operator} {right})"
print(generate_complex_expression(2))
这段代码会生成如“(3 + (4 * 2))”的复杂表达式。
生成数学表达式时如何确保表达式的有效性?
在生成数学表达式时,需要注意运算的有效性,尤其是在涉及除法时,确保不会出现除以零的情况。可以通过在生成随机数字时设置条件来避免这种情况,例如:
num2 = random.randint(1, 10) # 确保num2不为零
这样的处理可以确保生成的表达式在计算时不会出错。
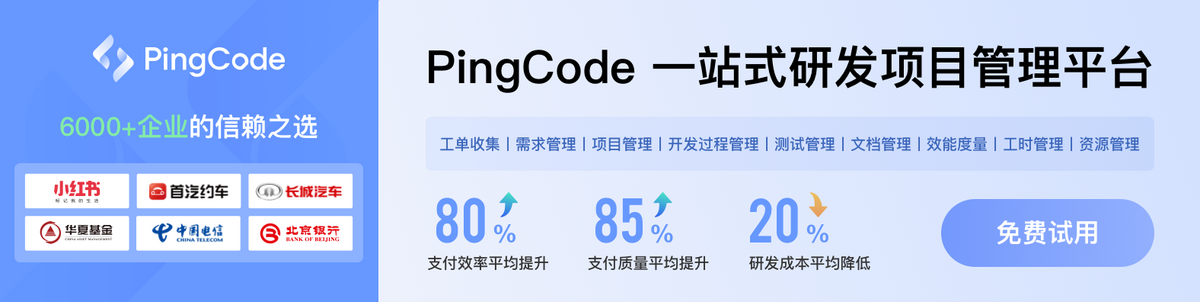