使用Python测试IP是否可用,可以通过ping测试、端口扫描、网络请求等方法。在本文中,我们将详细探讨这些方法,并提供相应的代码示例。ping测试是一种常见的方法,可以快速检查IP是否可达。下面我们将详细介绍如何在Python中实现这些方法。
一、PING测试
ping测试是通过向目标IP地址发送ICMP Echo请求包,并等待响应来判断目标是否可达的一种方法。Python可以使用os
模块或subprocess
模块来实现ping测试。
使用os模块
os.system
可以直接调用操作系统的ping命令。在Windows和Linux系统中,ping命令的参数略有不同。
import os
def ping_ip(ip):
# Ping参数-w 1表示等待1秒响应,-n 1表示发送1个数据包(Windows)
# Ping参数-c 1表示发送1个数据包,-W 1表示等待1秒响应(Linux)
response = os.system("ping -c 1 -W 1 " + ip)
if response == 0:
print(f"{ip} is reachable")
else:
print(f"{ip} is not reachable")
ping_ip('8.8.8.8')
使用subprocess模块
subprocess
模块可以更灵活地执行系统命令,并获取命令的输出和状态码。
import subprocess
def ping_ip(ip):
try:
# Ping参数-c 1表示发送1个数据包,-W 1表示等待1秒响应(Linux)
# Ping参数-n 1表示发送1个数据包,-w 1000表示等待1秒响应(Windows)
response = subprocess.run(["ping", "-c", "1", "-W", "1", ip], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
if response.returncode == 0:
print(f"{ip} is reachable")
else:
print(f"{ip} is not reachable")
except Exception as e:
print(f"An error occurred: {e}")
ping_ip('8.8.8.8')
二、端口扫描
端口扫描可以通过尝试连接目标IP地址的特定端口来测试IP是否可用。Python可以使用socket
模块实现端口扫描。
import socket
def scan_port(ip, port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(1) # 设置超时时间为1秒
try:
result = sock.connect_ex((ip, port))
if result == 0:
print(f"Port {port} on {ip} is open")
else:
print(f"Port {port} on {ip} is closed or filtered")
except Exception as e:
print(f"An error occurred: {e}")
finally:
sock.close()
scan_port('8.8.8.8', 80)
三、网络请求
通过发送HTTP请求可以测试Web服务器IP是否可用。Python可以使用requests
库发送HTTP请求。
import requests
def test_ip(ip):
url = f"http://{ip}"
try:
response = requests.get(url, timeout=1) # 设置超时时间为1秒
if response.status_code == 200:
print(f"IP {ip} is reachable, HTTP status code: {response.status_code}")
else:
print(f"IP {ip} is reachable, but returned HTTP status code: {response.status_code}")
except requests.RequestException as e:
print(f"IP {ip} is not reachable, error: {e}")
test_ip('8.8.8.8')
四、综合方法
结合以上方法,可以编写一个综合测试IP可用性的函数,包括ping测试、端口扫描和网络请求。
import os
import subprocess
import socket
import requests
def test_ip_reachability(ip):
# Ping测试
def ping_test(ip):
try:
response = subprocess.run(["ping", "-c", "1", "-W", "1", ip], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
return response.returncode == 0
except Exception as e:
print(f"Ping test error: {e}")
return False
# 端口扫描
def port_scan(ip, port=80):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(1)
try:
result = sock.connect_ex((ip, port))
return result == 0
except Exception as e:
print(f"Port scan error: {e}")
return False
finally:
sock.close()
# 网络请求
def http_request(ip):
url = f"http://{ip}"
try:
response = requests.get(url, timeout=1)
return response.status_code == 200
except requests.RequestException as e:
print(f"HTTP request error: {e}")
return False
# 综合判断
is_reachable = ping_test(ip) or port_scan(ip) or http_request(ip)
if is_reachable:
print(f"IP {ip} is reachable")
else:
print(f"IP {ip} is not reachable")
test_ip_reachability('8.8.8.8')
五、使用多线程提高效率
对于大量IP地址的测试,可以使用多线程来提高效率。Python的threading
模块可以帮助我们实现多线程。
import threading
def test_ip_reachability_multithread(ips):
def worker(ip):
test_ip_reachability(ip)
threads = []
for ip in ips:
thread = threading.Thread(target=worker, args=(ip,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
ips = ['8.8.8.8', '8.8.4.4', '1.1.1.1', '192.168.1.1']
test_ip_reachability_multithread(ips)
六、总结
本文介绍了多种测试IP是否可用的方法,包括ping测试、端口扫描和网络请求。ping测试可以快速检查IP是否可达,端口扫描可以进一步确认特定端口是否开放,网络请求可以测试Web服务器的可达性。通过结合这些方法,我们可以编写一个综合测试IP可用性的函数,并使用多线程提高测试效率。
希望本文能够帮助您更好地理解如何使用Python测试IP是否可用,并为您的实际应用提供参考。
相关问答FAQs:
如何使用Python检测某个IP地址的可用性?
使用Python检测IP地址可用性可以通过多种方式实现。常用的方法包括使用socket库尝试连接到特定的端口,或者使用ping命令。以下是一个简单的示例,使用socket库连接到指定IP和端口进行测试:
import socket
def is_ip_available(ip, port=80):
try:
socket.create_connection((ip, port), timeout=2)
return True
except OSError:
return False
ip_address = "192.168.1.1" # 替换为您要测试的IP地址
if is_ip_available(ip_address):
print(f"{ip_address} 是可用的.")
else:
print(f"{ip_address} 不可用.")
是否有现成的Python库可以帮助检测IP地址?
是的,Python有许多库可以帮助进行IP地址可用性测试,例如ping3
和scapy
。使用这些库可以更方便地实现IP地址的测试。例如,ping3
库允许用户轻松地发送ping请求并获取响应。
from ping3 import ping
ip_address = "192.168.1.1" # 替换为您要测试的IP地址
response = ping(ip_address)
if response is not None:
print(f"{ip_address} 是可用的,延迟为 {response} 秒.")
else:
print(f"{ip_address} 不可用.")
如何处理在测试IP可用性时可能遇到的错误?
在进行IP地址测试时,可能会遇到多种网络错误,比如连接超时或目标不可达。为了处理这些情况,可以使用异常处理机制来捕捉错误并返回相应的提示。例如,使用try-except结构来捕捉socket.error
和其他常见的网络异常。这样可以确保程序不会因为一个错误而崩溃,同时为用户提供明确的错误信息。
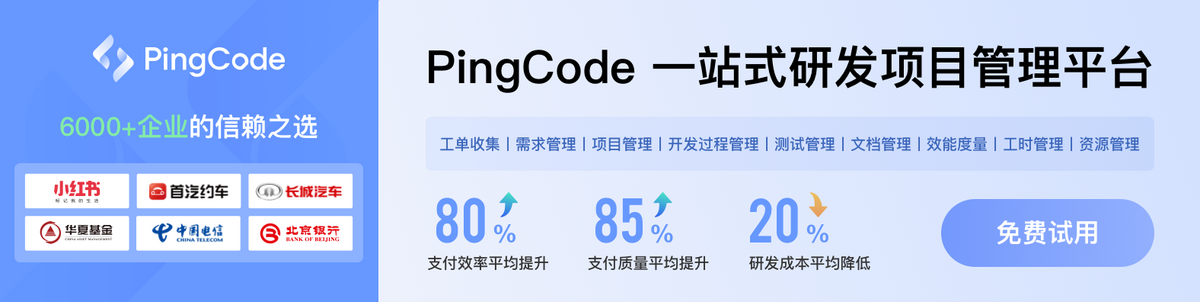