用Python绘制爱心循环,可以使用多种方法,其中最常见的包括使用turtle库、matplotlib库、以及Pygame库。 在此,我们将详细介绍如何使用turtle库来绘制一个动态的爱心循环。turtle库适用于绘制简单的图形和动画,是Python中一个非常友好的绘图库。
一、使用turtle库绘制爱心循环
1、安装turtle库
turtle库是Python内置的标准库,通常不需要额外安装。如果你使用的是标准的Python环境,可以直接使用。如果你使用的是某些精简的Python环境(如某些在线编译器),可能需要检查是否包含turtle库。
2、绘制静态爱心
在开始绘制动态爱心之前,我们需要首先了解如何用turtle库绘制一个静态的爱心图形。以下是一个简单的示例代码:
import turtle
def draw_heart():
window = turtle.Screen()
window.bgcolor("white")
pen = turtle.Turtle()
pen.color("red")
pen.begin_fill()
pen.left(50)
pen.forward(133)
pen.circle(50, 200)
pen.right(140)
pen.circle(50, 200)
pen.forward(133)
pen.end_fill()
pen.hideturtle()
window.exitonclick()
draw_heart()
在这段代码中,我们首先创建一个窗口,然后使用turtle库绘制一个心形图案。pen.begin_fill()和pen.end_fill()之间的代码指定绘制的区域填充颜色。
3、创建动态爱心循环
为了创建一个动态的爱心循环,我们需要在绘制完一个爱心后,清除屏幕并重新绘制。以下是实现动态爱心循环的代码:
import turtle
import time
def draw_heart(pen):
pen.color("red")
pen.begin_fill()
pen.left(50)
pen.forward(133)
pen.circle(50, 200)
pen.right(140)
pen.circle(50, 200)
pen.forward(133)
pen.end_fill()
def clear_heart(pen):
pen.clear()
def main():
window = turtle.Screen()
window.bgcolor("white")
pen = turtle.Turtle()
while True:
draw_heart(pen)
time.sleep(1)
clear_heart(pen)
time.sleep(1)
window.exitonclick()
if __name__ == "__main__":
main()
在这段代码中,我们创建了一个无限循环,每次绘制完爱心后等待一秒,然后清除屏幕并再次绘制。这就实现了爱心的动态循环效果。
二、使用matplotlib库绘制爱心循环
1、安装matplotlib库
如果尚未安装matplotlib库,可以使用以下命令进行安装:
pip install matplotlib
2、绘制静态爱心
使用matplotlib库绘制静态爱心的代码如下:
import matplotlib.pyplot as plt
import numpy as np
def draw_heart():
t = np.linspace(0, 2 * np.pi, 100)
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
plt.plot(x, y, 'r')
plt.fill(x, y, 'r')
plt.axis('equal')
plt.show()
draw_heart()
在这段代码中,我们使用参数方程绘制心形曲线,并填充颜色。
3、创建动态爱心循环
为了创建动态爱心循环,我们可以使用matplotlib的动画功能。以下是一个示例代码:
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
def draw_heart():
fig, ax = plt.subplots()
t = np.linspace(0, 2 * np.pi, 100)
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
line, = ax.plot(x, y, 'r')
ax.fill(x, y, 'r')
ax.axis('equal')
def update(frame):
if frame % 2 == 0:
line.set_visible(False)
ax.fill(x, y, 'w')
else:
line.set_visible(True)
ax.fill(x, y, 'r')
return line,
ani = animation.FuncAnimation(fig, update, frames=np.arange(0, 10), interval=500, blit=True)
plt.show()
draw_heart()
在这段代码中,我们使用FuncAnimation来创建动画效果,每隔500毫秒更新一次画面,实现在屏幕上动态显示爱心图案。
三、使用Pygame库绘制爱心循环
1、安装Pygame库
如果尚未安装Pygame库,可以使用以下命令进行安装:
pip install pygame
2、绘制静态爱心
使用Pygame库绘制静态爱心的代码如下:
import pygame
import math
def draw_heart(surface):
for angle in range(0, 360, 1):
x = 16 * math.sin(math.radians(angle)) 3
y = 13 * math.cos(math.radians(angle)) - 5 * math.cos(math.radians(2 * angle)) - 2 * math.cos(math.radians(3 * angle)) - math.cos(math.radians(4 * angle))
pygame.draw.circle(surface, (255, 0, 0), (int(300 + 10 * x), int(300 - 10 * y)), 2)
def main():
pygame.init()
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("Heart Animation")
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
draw_heart(screen)
pygame.display.flip()
clock.tick(60)
pygame.quit()
if __name__ == "__main__":
main()
在这段代码中,我们使用Pygame库绘制心形曲线,并在屏幕上显示。
3、创建动态爱心循环
为了创建动态爱心循环,我们可以通过在主循环中添加暂停和清除屏幕的逻辑来实现。以下是一个示例代码:
import pygame
import math
import time
def draw_heart(surface):
for angle in range(0, 360, 1):
x = 16 * math.sin(math.radians(angle)) 3
y = 13 * math.cos(math.radians(angle)) - 5 * math.cos(math.radians(2 * angle)) - 2 * math.cos(math.radians(3 * angle)) - math.cos(math.radians(4 * angle))
pygame.draw.circle(surface, (255, 0, 0), (int(300 + 10 * x), int(300 - 10 * y)), 2)
def main():
pygame.init()
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("Heart Animation")
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
draw_heart(screen)
pygame.display.flip()
time.sleep(1)
screen.fill((255, 255, 255))
pygame.display.flip()
time.sleep(1)
pygame.quit()
if __name__ == "__main__":
main()
在这段代码中,我们在每次绘制完爱心后暂停一秒,然后清除屏幕并再次绘制,实现了动态爱心循环效果。
四、总结
综上所述,我们可以使用turtle库、matplotlib库和Pygame库来绘制爱心循环。turtle库适用于绘制简单的图形和动画,matplotlib库适用于绘制高质量的图形和动画,Pygame库适用于创建复杂的游戏和动画。 根据具体需求选择合适的绘图库,可以更高效地实现绘制爱心循环的效果。
相关问答FAQs:
如何使用Python绘制爱心形状?
在Python中,可以使用Matplotlib库来绘制爱心形状。您可以通过定义一个心形的方程,利用极坐标系或笛卡尔坐标系来绘制。具体步骤包括设置坐标轴、定义爱心的数学方程,并调用绘图函数进行绘制。
使用哪些库或工具可以绘制爱心图案?
绘制爱心图案最常用的库是Matplotlib。除此之外,您还可以使用NumPy库来处理数组和数学运算,或者Pygame库来实现图形界面的绘制。结合这些工具,可以实现更多样化和复杂的爱心图案。
是否可以自定义爱心的颜色和样式?
当然可以!在Matplotlib中,您可以通过设置绘图函数的参数来自定义颜色、线型和填充样式。例如,可以使用fill()
函数来填充颜色,或使用plot()
函数来改变线条的粗细和样式。这使得您能够创造出独特的爱心图案,满足个人的审美需求。
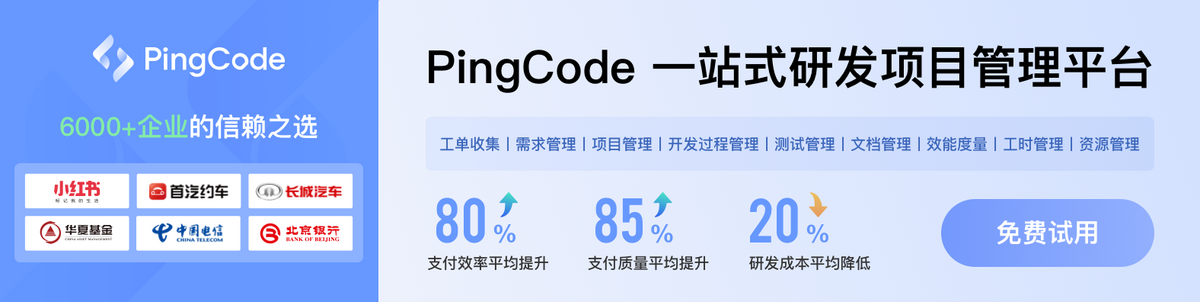