要使用Python连接WiFi,可以使用一些系统命令行工具并通过Python脚本调用它们。最常用的方法是使用subprocess
模块来执行系统命令。
1、使用subprocess模块调用系统命令:Python中的subprocess
模块允许你启动新的应用程序,并连接到它们的输入/输出/错误管道。通过调用系统的网络配置工具,你可以连接到WiFi网络。Windows下使用netsh
命令,Linux下使用nmcli
命令。
2、使用第三方库:比如wifi
库。该库可以在Python中直接调用WiFi连接功能,非常方便。
详细描述subprocess模块的使用
subprocess
模块允许你启动新进程,连接它们的输入/输出/错误管道,并获得返回码。以下是一个在Windows平台上使用netsh
命令连接WiFi的示例:
import subprocess
def connect_to_wifi(ssid, password):
# 创建一个配置文件
config = f'''
<WLANProfile xmlns="http://www.microsoft.com/networking/WLAN/profile/v1">
<name>{ssid}</name>
<SSIDConfig>
<SSID>
<name>{ssid}</name>
</SSID>
</SSIDConfig>
<connectionType>ESS</connectionType>
<connectionMode>auto</connectionMode>
<MSM>
<security>
<authEncryption>
<authentication>WPA2PSK</authentication>
<encryption>AES</encryption>
<useOneX>false</useOneX>
</authEncryption>
<sharedKey>
<keyMaterial>{password}</keyMaterial>
</sharedKey>
</security>
</MSM>
</WLANProfile>
'''
# 保存配置文件
with open('wifi_config.xml', 'w') as file:
file.write(config)
# 添加配置文件
subprocess.run(['netsh', 'wlan', 'add', 'profile', 'filename="wifi_config.xml"'])
# 连接到WiFi
result = subprocess.run(['netsh', 'wlan', 'connect', 'name={}'.format(ssid)], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
使用第三方库wifi
在某些平台上,你可以使用wifi
库。这是一个第三方库,可以更方便地处理WiFi连接。
首先,你需要安装这个库:
pip install wifi
然后,你可以使用以下代码连接WiFi:
from wifi import Cell, Scheme
def connect_to_wifi(ssid, password):
cells = Cell.all('wlan0')
for cell in cells:
if cell.ssid == ssid:
scheme = Scheme.for_cell('wlan0', ssid, cell, password)
scheme.save()
scheme.activate()
print('Connected to WiFi successfully.')
return
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
其他平台的连接方式
在Linux系统上,你可以使用nmcli
命令来连接WiFi,具体代码如下:
import subprocess
def connect_to_wifi(ssid, password):
# 使用nmcli命令连接WiFi
result = subprocess.run(['nmcli', 'dev', 'wifi', 'connect', ssid, 'password', password], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
Windows下更多细节
在Windows操作系统上,使用netsh
命令行工具可以非常方便地管理无线网络连接。netsh
命令可以配置和控制本地或远程计算机上的网络设置。
创建和应用WiFi配置文件
首先,需要创建一个WiFi配置文件。这个配置文件包含了WiFi网络的SSID和密码等信息。以下是一个典型的WiFi配置文件的内容:
<WLANProfile xmlns="http://www.microsoft.com/networking/WLAN/profile/v1">
<name>SSID_Name</name>
<SSIDConfig>
<SSID>
<name>SSID_Name</name>
</SSID>
</SSIDConfig>
<connectionType>ESS</connectionType>
<connectionMode>auto</connectionMode>
<MSM>
<security>
<authEncryption>
<authentication>WPA2PSK</authentication>
<encryption>AES</encryption>
<useOneX>false</useOneX>
</authEncryption>
<sharedKey>
<keyMaterial>Password</keyMaterial>
</sharedKey>
</security>
</MSM>
</WLANProfile>
将上述内容保存到一个XML文件中,例如wifi_config.xml
。
使用netsh命令连接WiFi
通过Python的subprocess
模块调用netsh
命令来添加和连接WiFi配置:
import subprocess
def connect_to_wifi(ssid, password):
# 创建WiFi配置文件
config = f'''
<WLANProfile xmlns="http://www.microsoft.com/networking/WLAN/profile/v1">
<name>{ssid}</name>
<SSIDConfig>
<SSID>
<name>{ssid}</name>
</SSID>
</SSIDConfig>
<connectionType>ESS</connectionType>
<connectionMode>auto</connectionMode>
<MSM>
<security>
<authEncryption>
<authentication>WPA2PSK</authentication>
<encryption>AES</encryption>
<useOneX>false</useOneX>
</authEncryption>
<sharedKey>
<keyMaterial>{password}</keyMaterial>
</sharedKey>
</security>
</MSM>
</WLANProfile>
'''
# 保存配置文件
with open('wifi_config.xml', 'w') as file:
file.write(config)
# 添加配置文件
subprocess.run(['netsh', 'wlan', 'add', 'profile', 'filename="wifi_config.xml"'])
# 连接到WiFi
result = subprocess.run(['netsh', 'wlan', 'connect', 'name={}'.format(ssid)], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
Linux下更多细节
在Linux系统上,nmcli
命令是NetworkManager的命令行工具。它可以管理网络连接,包括WiFi连接。
使用nmcli命令连接WiFi
你可以使用以下命令来连接WiFi:
nmcli dev wifi connect 'SSID' password 'PASSWORD'
以下是一个使用Python脚本调用nmcli
命令的示例:
import subprocess
def connect_to_wifi(ssid, password):
# 使用nmcli命令连接WiFi
result = subprocess.run(['nmcli', 'dev', 'wifi', 'connect', ssid, 'password', password], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
macOS下更多细节
在macOS系统上,你可以使用networksetup
命令来管理WiFi连接。以下是一个使用Python脚本调用networksetup
命令的示例:
import subprocess
def connect_to_wifi(ssid, password):
# 使用networksetup命令连接WiFi
result = subprocess.run(['networksetup', '-setairportnetwork', 'en0', ssid, password], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
处理连接失败的情况
无论使用哪种方法连接WiFi,你都需要处理连接失败的情况。通常情况下,连接失败可能是由于以下原因:
- SSID或密码错误
- WiFi信号较弱
- 网络适配器未启用
- 其他未知错误
你可以在脚本中添加一些错误处理逻辑,以便更好地应对这些情况。例如:
import subprocess
def connect_to_wifi(ssid, password):
# 使用nmcli命令连接WiFi
result = subprocess.run(['nmcli', 'dev', 'wifi', 'connect', ssid, 'password', password], capture_output=True)
if result.returncode == 0:
print('Connected to WiFi successfully.')
else:
print('Failed to connect to WiFi.')
# 处理连接失败的情况
error_message = result.stderr.decode('utf-8')
if 'No network with SSID' in error_message:
print('SSID not found. Please check the SSID and try again.')
elif 'Wrong password' in error_message:
print('Incorrect password. Please check the password and try again.')
else:
print(f'Unknown error: {error_message}')
使用函数连接WiFi
connect_to_wifi('Your_SSID', 'Your_Password')
总结
通过以上示例,你应该已经了解了如何使用Python连接WiFi。无论是在Windows、Linux还是macOS平台上,你都可以使用subprocess
模块来调用系统命令,并通过这些命令来管理WiFi连接。在实际应用中,你需要根据具体的平台和需求选择合适的工具和方法。此外,处理连接失败的情况和错误信息也是非常重要的,以确保你的脚本在各种情况下都能稳定运行。
相关问答FAQs:
如何使用Python连接到WiFi网络?
要使用Python连接WiFi网络,您可以使用subprocess
模块来调用系统命令,或者使用wifi
库来实现。通过subprocess
模块,您可以在Windows或Linux系统上执行命令行指令,添加或连接到WiFi网络。使用wifi
库则可以更方便地管理WiFi连接,适合需要更复杂操作的用户。
需要安装哪些Python库以便连接WiFi?
如果您选择使用wifi
库,您需要先安装它。可以通过以下命令安装:
pip install wifi
另外,使用subprocess
模块不需要额外安装任何库,但请确保您的Python环境配置正确。
在连接WiFi时,如何处理连接失败的情况?
在连接WiFi的过程中,如果出现连接失败的情况,您可以通过捕获异常来处理。例如,如果使用wifi
库,可以使用try...except
语句来捕获连接错误,并输出相应的错误信息,以便用户能够了解问题所在并进行相应的调整。
Python如何获取当前已连接的WiFi网络信息?
要获取当前已连接的WiFi网络信息,可以使用subprocess
模块调用系统命令,例如在Windows上使用netsh wlan show interfaces
命令,或在Linux上使用iwgetid
命令。这些命令可以返回当前连接的网络名称、信号强度等信息,您可以在Python代码中处理这些信息并以用户友好的方式显示。
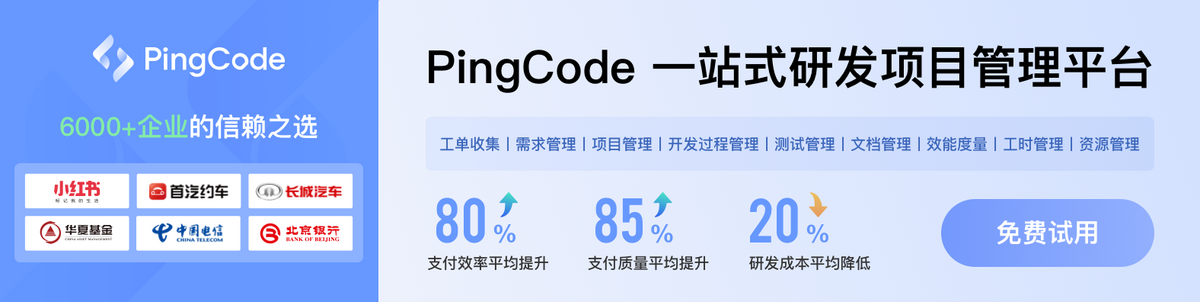