Python识别目标图形的方法包括:使用OpenCV库进行图像处理、应用卷积神经网络(CNN)进行深度学习、利用形状检测技术、借助预训练模型、采用传统的机器学习方法。 其中,使用OpenCV库进行图像处理是最基础且广泛应用的方法之一。OpenCV提供了丰富的图像处理功能,可以进行图像的预处理、轮廓检测、特征提取等操作,从而实现目标图形的识别。
利用OpenCV进行目标图形识别时,首先需要对图像进行预处理,例如灰度化、二值化等操作。接下来,通过轮廓检测算法(如Canny边缘检测)提取图像中的轮廓,并根据轮廓的形状、面积等特征进行目标图形的识别。此外,还可以结合形状匹配算法(如Hu矩不变特征)进行进一步的匹配和识别。
一、图像预处理
1、灰度化
灰度化是将彩色图像转换为灰度图像的过程。彩色图像包含三个通道(红、绿、蓝),灰度化后图像仅包含一个通道,降低了图像处理的复杂度。OpenCV提供了cv2.cvtColor
函数来实现灰度化。
import cv2
读取彩色图像
image = cv2.imread('image.jpg')
将图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
显示灰度图像
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
2、二值化
二值化是将灰度图像转换为黑白图像的过程,通过设定一个阈值,将像素值高于阈值的部分设为白色(255),低于阈值的部分设为黑色(0)。OpenCV提供了cv2.threshold
函数来实现二值化。
# 将灰度图像二值化
ret, binary_image = cv2.threshold(gray_image, 127, 255, cv2.THRESH_BINARY)
显示二值化图像
cv2.imshow('Binary Image', binary_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
二、轮廓检测
轮廓检测是从图像中提取物体轮廓的过程。OpenCV提供了cv2.findContours
函数来实现轮廓检测,该函数返回图像中所有轮廓的列表。
# 检测轮廓
contours, hierarchy = cv2.findContours(binary_image, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
绘制轮廓
contour_image = cv2.drawContours(image.copy(), contours, -1, (0, 255, 0), 2)
显示轮廓图像
cv2.imshow('Contours', contour_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
三、特征提取
提取轮廓后,可以根据轮廓的形状、面积等特征来识别目标图形。
1、形状特征
可以利用轮廓的周长、面积、近似多边形等特征来描述轮廓的形状。例如,可以通过cv2.arcLength
函数计算轮廓的周长,通过cv2.contourArea
函数计算轮廓的面积,通过cv2.approxPolyDP
函数将轮廓近似为多边形。
for contour in contours:
# 计算轮廓的周长
perimeter = cv2.arcLength(contour, True)
# 计算轮廓的面积
area = cv2.contourArea(contour)
# 近似多边形
epsilon = 0.02 * perimeter
approx = cv2.approxPolyDP(contour, epsilon, True)
# 根据多边形的顶点数识别形状
if len(approx) == 3:
shape = 'Triangle'
elif len(approx) == 4:
shape = 'Rectangle'
elif len(approx) > 4:
shape = 'Circle'
else:
shape = 'Unknown'
# 绘制识别结果
cv2.drawContours(image, [approx], -1, (0, 255, 0), 2)
cv2.putText(image, shape, tuple(approx[0][0]), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
显示识别结果
cv2.imshow('Shape Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、形状匹配
形状匹配是将待识别的轮廓与已知形状进行匹配的过程。OpenCV提供了cv2.matchShapes
函数来计算两个形状之间的相似度。
# 读取模板图像并提取轮廓
template_image = cv2.imread('template.jpg', cv2.IMREAD_GRAYSCALE)
ret, template_binary = cv2.threshold(template_image, 127, 255, cv2.THRESH_BINARY)
template_contours, _ = cv2.findContours(template_binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
template_contour = template_contours[0]
for contour in contours:
# 计算形状匹配分数
match_score = cv2.matchShapes(template_contour, contour, 1, 0.0)
# 根据匹配分数判断是否为目标形状
if match_score < 0.2:
shape = 'Target Shape'
else:
shape = 'Unknown'
# 绘制识别结果
cv2.drawContours(image, [contour], -1, (0, 255, 0), 2)
cv2.putText(image, shape, tuple(contour[0][0]), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
显示识别结果
cv2.imshow('Shape Matching', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
五、卷积神经网络(CNN)
卷积神经网络(CNN)是一种深度学习模型,广泛应用于图像识别任务。通过训练CNN模型,可以自动提取图像中的特征,实现目标图形的识别。
1、数据准备
首先需要准备训练数据,包括目标图形和非目标图形的图像,并将其划分为训练集和测试集。
import os
import numpy as np
from sklearn.model_selection import train_test_split
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.utils import to_categorical
数据目录
data_dir = 'data'
categories = ['target', 'non_target']
图像尺寸
img_size = 64
加载图像数据
def load_data(data_dir, categories, img_size):
data = []
labels = []
for category in categories:
category_dir = os.path.join(data_dir, category)
label = categories.index(category)
for img_name in os.listdir(category_dir):
img_path = os.path.join(category_dir, img_name)
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (img_size, img_size))
data.append(img)
labels.append(label)
data = np.array(data).reshape(-1, img_size, img_size, 1)
labels = np.array(labels)
return data, labels
data, labels = load_data(data_dir, categories, img_size)
data = data / 255.0
labels = to_categorical(labels, num_classes=len(categories))
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data, labels, test_size=0.2, random_state=42)
2、模型构建
构建一个简单的卷积神经网络模型,包括卷积层、池化层和全连接层。
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(img_size, img_size, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(len(categories), activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
3、模型训练
使用训练数据训练卷积神经网络模型。
# 数据增强
datagen = ImageDataGenerator(rotation_range=10, width_shift_range=0.1, height_shift_range=0.1, zoom_range=0.1)
datagen.fit(X_train)
训练模型
history = model.fit(datagen.flow(X_train, y_train, batch_size=32), validation_data=(X_test, y_test), epochs=10)
4、模型评估
使用测试数据评估训练好的模型性能。
test_loss, test_acc = model.evaluate(X_test, y_test)
print(f'Test Accuracy: {test_acc}')
5、模型预测
使用训练好的模型对新图像进行预测,识别目标图形。
# 读取新图像并预处理
new_image = cv2.imread('new_image.jpg', cv2.IMREAD_GRAYSCALE)
new_image = cv2.resize(new_image, (img_size, img_size))
new_image = new_image.reshape(1, img_size, img_size, 1) / 255.0
预测目标图形
prediction = model.predict(new_image)
predicted_class = categories[np.argmax(prediction)]
print(f'Predicted Class: {predicted_class}')
六、传统机器学习方法
除了深度学习方法,还可以采用传统的机器学习方法(如支持向量机、随机森林等)进行目标图形识别。首先需要提取图像的特征,然后使用机器学习算法进行分类。
1、特征提取
可以使用SIFT、SURF、ORB等算法提取图像的局部特征,也可以使用HOG、LBP等算法提取图像的全局特征。
from skimage.feature import hog
def extract_hog_features(images):
hog_features = []
for image in images:
feature, _ = hog(image, orientations=9, pixels_per_cell=(8, 8), cells_per_block=(2, 2), visualize=True)
hog_features.append(feature)
return np.array(hog_features)
提取训练集和测试集的HOG特征
X_train_hog = extract_hog_features(X_train)
X_test_hog = extract_hog_features(X_test)
2、模型训练
使用支持向量机(SVM)训练分类模型。
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
训练SVM模型
svm_model = SVC(kernel='linear')
svm_model.fit(X_train_hog, y_train.argmax(axis=1))
测试模型
y_pred = svm_model.predict(X_test_hog)
test_acc = accuracy_score(y_test.argmax(axis=1), y_pred)
print(f'Test Accuracy: {test_acc}')
3、模型预测
使用训练好的SVM模型对新图像进行预测,识别目标图形。
# 提取新图像的HOG特征
new_image_hog = extract_hog_features([new_image])[0]
预测目标图形
predicted_class = svm_model.predict([new_image_hog])
print(f'Predicted Class: {categories[predicted_class[0]]}')
七、预训练模型
利用预训练的深度学习模型(如VGG、ResNet等)进行目标图形识别,可以避免从头开始训练模型,提高识别精度和效率。
1、加载预训练模型
使用TensorFlow或PyTorch加载预训练模型,并进行微调。
from tensorflow.keras.applications import VGG16
from tensorflow.keras.models import Model
from tensorflow.keras.layers import GlobalAveragePooling2D, Dense
加载预训练的VGG16模型
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(img_size, img_size, 3))
添加自定义的全连接层
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(len(categories), activation='softmax')(x)
构建模型
model = Model(inputs=base_model.input, outputs=predictions)
冻结预训练模型的卷积层
for layer in base_model.layers:
layer.trainable = False
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
2、模型训练
使用训练数据微调预训练模型。
# 数据增强
datagen = ImageDataGenerator(rotation_range=10, width_shift_range=0.1, height_shift_range=0.1, zoom_range=0.1)
datagen.fit(X_train)
训练模型
history = model.fit(datagen.flow(X_train, y_train, batch_size=32), validation_data=(X_test, y_test), epochs=10)
3、模型评估
使用测试数据评估微调后的模型性能。
test_loss, test_acc = model.evaluate(X_test)
print(f'Test Accuracy: {test_acc}')
4、模型预测
使用微调后的模型对新图像进行预测,识别目标图形。
# 读取新图像并预处理
new_image = cv2.imread('new_image.jpg')
new_image = cv2.resize(new_image, (img_size, img_size))
new_image = new_image.reshape(1, img_size, img_size, 3) / 255.0
预测目标图形
prediction = model.predict(new_image)
predicted_class = categories[np.argmax(prediction)]
print(f'Predicted Class: {predicted_class}')
综上所述,Python识别目标图形的方法多种多样,可以根据具体需求选择合适的方法进行实现。对于简单的图像处理任务,可以使用OpenCV进行预处理、轮廓检测和特征提取;对于复杂的图像识别任务,可以采用卷积神经网络、传统机器学习方法或预训练模型进行识别。通过不断尝试和优化,可以提高目标图形识别的准确性和效率。
相关问答FAQs:
如何使用Python识别图形?
Python可以通过多种库来识别目标图形,例如OpenCV和scikit-image。OpenCV提供了图像处理的强大功能,可以进行边缘检测、轮廓查找等操作,从而帮助识别图形。使用scikit-image库也能实现图像分析与处理,利用其特征提取功能来识别不同的形状。
在Python中识别图形需要哪些库和工具?
要在Python中实现图形识别,通常使用OpenCV、NumPy和Matplotlib等库。OpenCV是主要用于图像处理的库,NumPy用于数值计算,Matplotlib则可以帮助可视化识别结果。安装这些库后,您可以轻松处理和分析图像数据。
识别图形时有哪些常见的技术和方法?
识别图形时,可以采用几种常见的技术,包括边缘检测、轮廓检测和模板匹配。边缘检测方法(如Canny算法)可以找出图形的边缘;轮廓检测可以帮助提取图形的轮廓信息;模板匹配则是通过与已知模板进行比较来识别图形。这些方法结合使用,往往能提高识别的准确性。
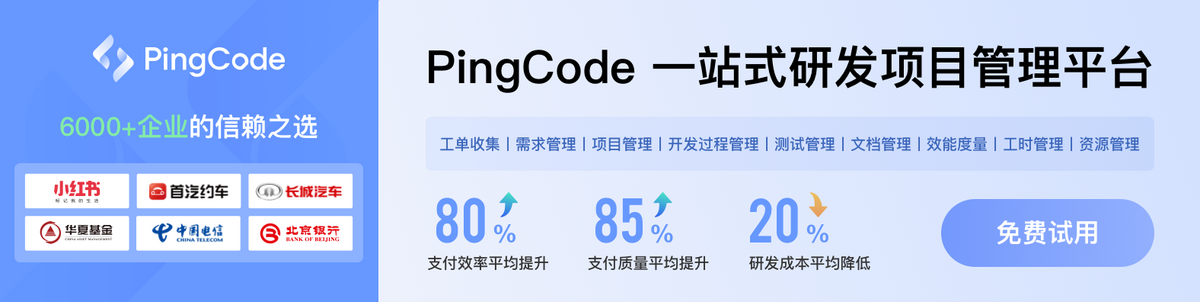