使用Python保存数据文件的方式有很多种,包括使用文件I/O操作、CSV文件、JSON文件、二进制文件、数据库等。选择哪种方式取决于数据的类型和用途。 其中,使用文件I/O操作是最基本的方式,可以保存文本和二进制数据;使用CSV文件可以方便地保存和读取表格数据;使用JSON文件可以保存和读取结构化数据;使用数据库可以保存大量数据并进行复杂查询。接下来,我们将详细介绍如何使用这些方法保存数据文件。
一、文件I/O操作
1、文本文件
文本文件是最简单的文件格式,可以用来保存任何文本数据。Python提供了内置的open()
函数来进行文件操作。
# 写入文本文件
with open('example.txt', 'w') as file:
file.write('Hello, world!\n')
file.write('This is a text file.\n')
上述代码使用open()
函数以写入模式('w'
)打开一个文件,并使用write()
方法将文本写入文件。使用with
语句可以确保文件在操作结束后自动关闭。
# 读取文本文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
上述代码以读取模式('r'
)打开文件,并使用read()
方法读取整个文件内容。
2、二进制文件
二进制文件用于保存二进制数据,如图片、音频、视频等。可以使用'wb'
模式写入和'rb'
模式读取。
# 写入二进制文件
with open('example.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03\x04')
读取二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
二、CSV文件
CSV(Comma Separated Values)文件是一种常用的表格数据格式,每行表示一条记录,字段之间用逗号分隔。Python的csv
模块可以方便地读写CSV文件。
import csv
写入CSV文件
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'City'])
writer.writerow(['Alice', 30, 'New York'])
writer.writerow(['Bob', 25, 'Los Angeles'])
读取CSV文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
三、JSON文件
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于人阅读和编写,也易于机器解析和生成。Python的json
模块可以方便地读写JSON文件。
import json
写入JSON文件
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.json', 'w') as file:
json.dump(data, file)
读取JSON文件
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
四、数据库
使用数据库保存数据的优势在于可以方便地进行数据查询和管理。Python提供了多种数据库接口,如SQLite、MySQL、PostgreSQL等。这里以SQLite为例,介绍如何使用Python的sqlite3
模块操作数据库。
import sqlite3
创建数据库连接
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER,
city TEXT
)
''')
插入数据
cursor.execute('''
INSERT INTO users (name, age, city)
VALUES ('Alice', 30, 'New York')
''')
提交事务
conn.commit()
查询数据
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭数据库连接
conn.close()
五、Pickle模块
Python的pickle
模块用于序列化和反序列化Python对象,可以将Python对象保存到文件中,也可以从文件中读取Python对象。
import pickle
创建一个字典对象
data = {'name': 'Alice', 'age': 30, 'city': 'New York'}
序列化对象并保存到文件
with open('example.pkl', 'wb') as file:
pickle.dump(data, file)
从文件中读取并反序列化对象
with open('example.pkl', 'rb') as file:
data = pickle.load(file)
print(data)
六、Pandas库
Pandas库是Python中常用的数据分析库,提供了强大的数据结构和数据分析工具。Pandas可以方便地读写多种文件格式,如CSV、Excel、JSON等。
import pandas as pd
创建DataFrame对象
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25],
'City': ['New York', 'Los Angeles']
}
df = pd.DataFrame(data)
保存DataFrame到CSV文件
df.to_csv('example.csv', index=False)
从CSV文件读取DataFrame
df = pd.read_csv('example.csv')
print(df)
七、Excel文件
Excel文件是另一种常用的表格数据格式。Python的openpyxl
和pandas
库可以方便地读写Excel文件。
from openpyxl import Workbook
创建Excel工作簿和工作表
wb = Workbook()
ws = wb.active
ws.title = "Sheet1"
写入数据到Excel文件
ws.append(['Name', 'Age', 'City'])
ws.append(['Alice', 30, 'New York'])
ws.append(['Bob', 25, 'Los Angeles'])
保存Excel文件
wb.save('example.xlsx')
读取Excel文件
df = pd.read_excel('example.xlsx')
print(df)
八、HDF5文件
HDF5是一种用于存储和组织大规模数据的文件格式。Python的h5py
库可以用于读写HDF5文件。
import h5py
import numpy as np
创建HDF5文件并写入数据
with h5py.File('example.h5', 'w') as file:
file.create_dataset('dataset', data=np.arange(100))
读取HDF5文件
with h5py.File('example.h5', 'r') as file:
data = file['dataset'][:]
print(data)
九、YAML文件
YAML(YAML Ain't Markup Language)是一种常用于配置文件的数据序列化格式。Python的PyYAML
库可以用于读写YAML文件。
import yaml
创建数据
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
写入YAML文件
with open('example.yaml', 'w') as file:
yaml.dump(data, file)
读取YAML文件
with open('example.yaml', 'r') as file:
data = yaml.load(file, Loader=yaml.FullLoader)
print(data)
十、XML文件
XML(Extensible Markup Language)是一种用于标记文档的通用格式。Python的xml.etree.ElementTree
模块可以用于解析和生成XML文件。
import xml.etree.ElementTree as ET
创建XML数据
root = ET.Element('root')
child1 = ET.SubElement(root, 'child')
child1.text = 'This is child1'
child2 = ET.SubElement(root, 'child')
child2.text = 'This is child2'
写入XML文件
tree = ET.ElementTree(root)
tree.write('example.xml')
读取XML文件
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.text)
十一、SQLAlchemy库
SQLAlchemy是Python的一个SQL工具包和对象关系映射(ORM)库。它提供了对关系数据库的高效和高层次的抽象。
from sqlalchemy import create_engine, Column, Integer, String, Base
from sqlalchemy.orm import sessionmaker
创建数据库连接
engine = create_engine('sqlite:///example.db')
Base = declarative_base()
定义User类
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
city = Column(String)
创建表
Base.metadata.create_all(engine)
创建会话
Session = sessionmaker(bind=engine)
session = Session()
插入数据
new_user = User(name='Alice', age=30, city='New York')
session.add(new_user)
session.commit()
查询数据
users = session.query(User).all()
for user in users:
print(user.name, user.age, user.city)
关闭会话
session.close()
十二、Feather文件
Feather是一种快速、轻量级的列式数据存储格式,适用于在Python和R之间进行数据传输。Python的pyarrow
库可以用于读写Feather文件。
import pyarrow.feather as feather
import pandas as pd
创建DataFrame对象
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25],
'City': ['New York', 'Los Angeles']
}
df = pd.DataFrame(data)
保存DataFrame到Feather文件
feather.write_feather(df, 'example.feather')
从Feather文件读取DataFrame
df = feather.read_feather('example.feather')
print(df)
以上介绍了Python中保存数据文件的多种方法,涵盖了文本文件、二进制文件、CSV文件、JSON文件、数据库、Pickle模块、Pandas库、Excel文件、HDF5文件、YAML文件、XML文件、SQLAlchemy库、Feather文件等。每种方法都有其适用的场景和优势,选择合适的方法可以提高数据处理的效率和灵活性。
相关问答FAQs:
如何在Python中选择合适的文件格式来保存数据?
在Python中,选择合适的文件格式对于数据的保存至关重要。常见的文件格式包括CSV、JSON、Excel等。CSV格式适合保存表格型数据,易于查看和编辑;JSON适合保存结构化数据,尤其是在Web应用中;Excel则适用于需要复杂表格和公式的数据。根据数据的结构和后续使用场景,选择适合的格式可以提高数据的可读性和可用性。
在Python中如何处理大数据文件的保存问题?
处理大数据文件时,建议使用分块保存的方法。使用Pandas的to_csv
或to_hdf
等函数时,可以设置chunksize
参数,将数据分批写入文件。这不仅可以避免内存溢出,还能提高写入效率。此外,使用二进制格式(如HDF5)也可以有效减少文件大小并加快读写速度。
如何确保保存的数据文件的安全性和完整性?
为了确保保存的数据文件的安全性和完整性,可以采取多个措施。首先,使用版本控制工具(如Git)来跟踪文件的变化,确保可以恢复到之前的版本。其次,定期备份数据文件,将其存储在不同的位置。最后,考虑使用加密技术来保护敏感数据,确保只有授权用户能够访问这些文件。
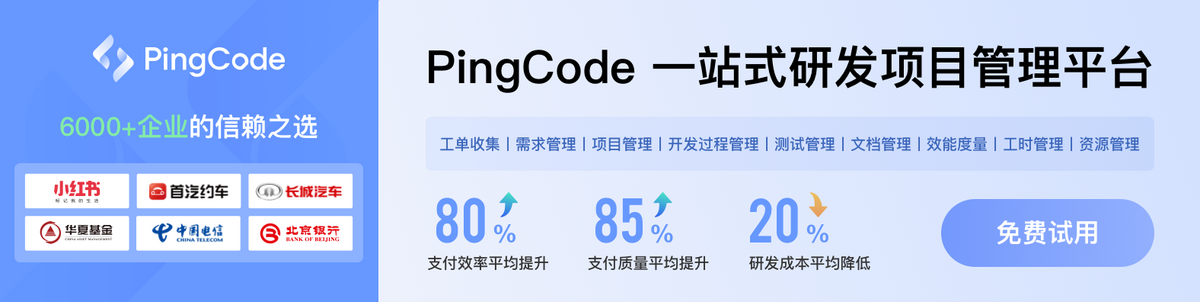