在Python中,有多种方法可以循环输出文字,主要包括for循环、while循环、通过递归函数实现循环等。for循环最常用、最简单、适用于已知循环次数的情况,例如遍历一个列表或者字符串。下面详细介绍for循环的使用。
一、for 循环
1、遍历列表
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
在这个例子中,for
循环遍历了列表fruits
中的每个元素,并将每个元素打印出来。
2、遍历字符串
word = "hello"
for letter in word:
print(letter)
这个例子展示了如何通过for
循环遍历字符串中的每个字符,并逐个打印出来。
二、while 循环
1、基本用法
count = 0
while count < 5:
print("This is loop iteration", count)
count += 1
在这个例子中,while
循环每次迭代都会检查条件count < 5
是否为真,然后打印当前的迭代次数,并将count
的值加1,直到条件为假时退出循环。
2、无限循环
while True:
print("This will print forever until stopped manually")
这种循环会一直执行,直到手动停止程序(例如,按Ctrl+C)。通常使用在需要不断运行的后台任务中。
三、通过递归函数实现循环
递归函数是一种函数调用自身的技术,适用于某些特定场景。尽管不直接是循环,但能实现类似的效果。
1、基本递归
def recursive_print(n):
if n > 0:
print("Recursion count:", n)
recursive_print(n-1)
recursive_print(5)
在这个例子中,函数recursive_print
调用自身,直到参数n
小于或等于0时停止。
四、其他循环技巧
1、循环中的break
和continue
for i in range(10):
if i == 5:
break # 终止循环
print(i)
for i in range(10):
if i % 2 == 0:
continue # 跳过当前迭代
print(i)
break
语句用于立即终止循环,而continue
语句用于跳过当前迭代并继续下一次迭代。
2、循环中的else
for i in range(5):
print(i)
else:
print("Loop completed successfully")
如果for
循环没有被break
终止,else
代码块会在循环结束后执行。
五、实际应用示例
1、生成乘法表
for i in range(1, 10):
for j in range(1, 10):
print(f"{i} * {j} = {i * j}", end="\t")
print()
这个例子展示了如何使用嵌套for
循环生成并打印一个乘法表。
2、处理文件
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
在这个例子中,for
循环遍历文件中的每一行,并逐行打印。
六、循环中的性能优化
1、避免不必要的计算
values = [1, 2, 3, 4, 5]
total = sum(values) # 预先计算总和
for value in values:
print(value, total)
预先计算不会改变的值,避免在循环中重复计算,可以提高性能。
2、使用生成器
def count_up_to(max):
count = 1
while count <= max:
yield count
count += 1
for number in count_up_to(5):
print(number)
生成器在需要大量数据时能节省内存,提高效率。
七、错误处理
1、捕获异常
try:
for i in range(5):
print(10 / i)
except ZeroDivisionError:
print("Cannot divide by zero")
在这个例子中,try
和except
块捕获并处理了除零错误。
2、确保资源释放
try:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
except IOError:
print("Error reading file")
确保在操作文件或网络资源时,正确处理可能的IO错误。
八、并发循环
1、使用多线程
import threading
def print_numbers():
for i in range(5):
print(i)
thread = threading.Thread(target=print_numbers)
thread.start()
thread.join()
多线程允许同时运行多个循环,提高程序的并发能力。
2、使用多进程
import multiprocessing
def print_numbers():
for i in range(5):
print(i)
process = multiprocessing.Process(target=print_numbers)
process.start()
process.join()
多进程与多线程类似,但每个进程有独立的内存空间,适用于CPU密集型任务。
九、总结
循环是Python编程中的基础操作,通过for
循环和while
循环,我们可以轻松实现对列表、字符串等数据结构的遍历操作。递归函数虽然不常见,但在特定场景下也能发挥作用。通过合理的错误处理和性能优化,我们可以使循环更加高效和健壮。并发编程则提供了在多任务环境下提升性能的手段。掌握这些技巧,可以大大提升编程效率和代码质量。
相关问答FAQs:
如何在Python中使用循环输出文本?
在Python中,可以使用for
循环或while
循环来重复输出文本。通过定义循环的次数,您可以轻松控制输出的频率。以下是一个简单的例子:
for i in range(5): # 输出5次
print("Hello, World!")
使用while
循环也很简单:
count = 0
while count < 5:
print("Hello, World!")
count += 1
这两种方法都能实现循环输出。
如何在循环中添加变量以动态输出文本?
在循环中,您可以通过引入变量来动态生成文本输出。例如,您可以使用一个列表来存储不同的文本,并在循环中逐一输出:
messages = ["Hello, World!", "Welcome to Python!", "Happy Coding!"]
for message in messages:
print(message)
这样,您就可以根据需要输出不同的文本内容。
如何在循环中实现条件输出?
您可以在循环中使用条件语句来控制哪些文本被输出。例如,您可以根据某个条件输出特定的消息:
for i in range(10):
if i % 2 == 0: # 判断是否为偶数
print(f"{i} 是偶数")
else:
print(f"{i} 是奇数")
这种方式使您能够根据特定条件灵活地输出不同的内容。
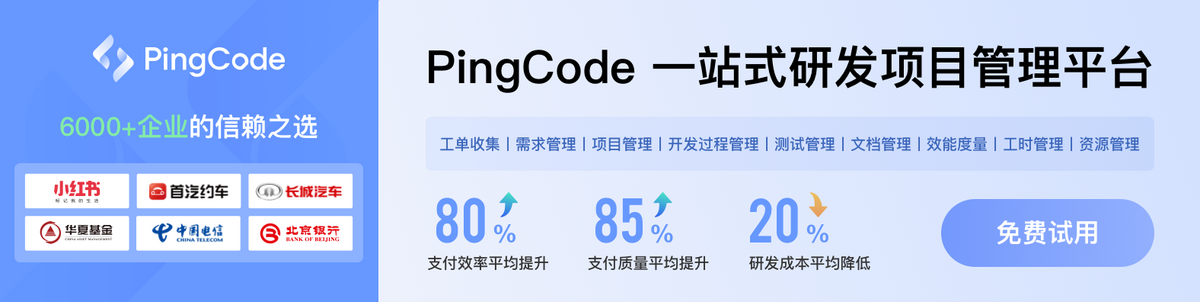