Python判断循环体主要通过使用for循环、while循环,条件判断语句、break和continue语句来实现。
通过for循环遍历一个序列(如列表、元组或字符串)并对每个元素执行特定操作;while循环则根据条件的真假执行循环体内部的代码;条件判断语句用于决定是否执行某些操作;break和continue用于控制循环的执行流程。例如,for循环可以用来遍历列表,while循环可以用来不断执行某些操作直到条件不满足,break可以用来提前退出循环,continue可以用来跳过本次循环的剩余代码。
下面将详细描述Python中如何使用这些方法来判断和控制循环体。
一、FOR循环
Python中的for循环用于遍历一个序列(如列表、元组或字符串),并对每个元素执行特定操作。
1、基本用法
for循环的基本语法如下:
for element in sequence:
# 执行操作
例如,遍历一个列表并打印每个元素:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
在这个例子中,for循环遍历列表fruits
中的每个元素,并将其赋值给变量fruit
,然后打印该变量的值。
2、使用range函数
range函数生成一个整数序列,常用于for循环中:
for i in range(5):
print(i)
这段代码将打印0到4的整数。
二、WHILE循环
while循环在条件为真时重复执行代码块。
1、基本用法
while循环的基本语法如下:
while condition:
# 执行操作
例如,打印从1到5的数字:
count = 1
while count <= 5:
print(count)
count += 1
在这个例子中,while循环在变量count
小于或等于5时执行循环体内部的代码,并在每次循环后将count
加1。
2、无限循环
如果条件一直为真,while循环将无限执行。可以使用break
语句来退出循环:
while True:
user_input = input("Enter 'exit' to quit: ")
if user_input == 'exit':
break
在这个例子中,while循环一直等待用户输入,直到用户输入'exit'时退出循环。
三、CONDITIONAL STATEMENTS
条件判断语句用于根据条件的真假来决定是否执行某些操作。
1、IF语句
if语句的基本语法如下:
if condition:
# 执行操作
例如,检查一个数字是否大于0:
number = 5
if number > 0:
print("The number is positive.")
2、IF-ELSE语句
如果条件为假,可以使用else语句执行其他操作:
number = -3
if number > 0:
print("The number is positive.")
else:
print("The number is not positive.")
3、IF-ELIF-ELSE语句
检查多个条件,可以使用elif语句:
number = 0
if number > 0:
print("The number is positive.")
elif number == 0:
print("The number is zero.")
else:
print("The number is negative.")
在这个例子中,if语句检查第一个条件,如果为假,则检查elif语句中的条件,如果仍为假,则执行else语句中的操作。
四、BREAK和CONTINUE语句
break和continue语句用于控制循环的执行流程。
1、BREAK语句
break语句用于提前退出循环:
for i in range(10):
if i == 5:
break
print(i)
这段代码将打印0到4的整数,当i等于5时退出循环。
2、CONTINUE语句
continue语句用于跳过本次循环的剩余代码,并开始下一次循环:
for i in range(10):
if i % 2 == 0:
continue
print(i)
这段代码将打印奇数0到9的整数。
五、嵌套循环
在Python中,可以将一个循环嵌套在另一个循环中。
1、嵌套FOR循环
例如,打印一个3×3的矩阵:
for i in range(3):
for j in range(3):
print(f"({i}, {j})")
在这个例子中,外层for循环控制行,内层for循环控制列。
2、嵌套WHILE循环
例如,打印一个3×3的矩阵:
i = 0
while i < 3:
j = 0
while j < 3:
print(f"({i}, {j})")
j += 1
i += 1
在这个例子中,外层while循环控制行,内层while循环控制列。
六、循环体中的条件判断
在循环体中,可以使用条件判断语句来控制循环的执行流程。
1、FOR循环中的条件判断
例如,遍历一个列表并打印其中的偶数:
numbers = [1, 2, 3, 4, 5, 6]
for number in numbers:
if number % 2 == 0:
print(number)
在这个例子中,for循环遍历列表numbers
中的每个元素,并使用if语句检查其是否为偶数,如果是,则打印该元素。
2、WHILE循环中的条件判断
例如,不断读取用户输入,直到输入为'exit':
while True:
user_input = input("Enter 'exit' to quit: ")
if user_input == 'exit':
break
else:
print(f"You entered: {user_input}")
在这个例子中,while循环不断读取用户输入,并使用if语句检查其是否为'exit',如果是,则使用break语句退出循环,否则打印用户输入的内容。
七、循环中的异常处理
在循环中,可以使用try-except语句来处理异常。
1、FOR循环中的异常处理
例如,遍历一个列表并处理可能出现的异常:
numbers = [1, 2, 'a', 4, 5]
for number in numbers:
try:
print(number + 1)
except TypeError:
print("Error: Invalid type")
在这个例子中,for循环遍历列表numbers
中的每个元素,并尝试将其加1,如果出现TypeError异常,则打印错误信息。
2、WHILE循环中的异常处理
例如,不断读取用户输入并处理可能出现的异常:
while True:
try:
user_input = int(input("Enter a number: "))
print(f"You entered: {user_input}")
except ValueError:
print("Error: Invalid input")
在这个例子中,while循环不断读取用户输入,并尝试将其转换为整数,如果出现ValueError异常,则打印错误信息。
八、循环中的函数调用
在循环中,可以调用函数来执行特定操作。
1、FOR循环中的函数调用
例如,定义一个函数并在for循环中调用:
def square(x):
return x * x
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(square(number))
在这个例子中,定义了一个函数square
,并在for循环中调用该函数来计算每个元素的平方。
2、WHILE循环中的函数调用
例如,定义一个函数并在while循环中调用:
def greet(name):
print(f"Hello, {name}!")
while True:
user_input = input("Enter your name (or 'exit' to quit): ")
if user_input == 'exit':
break
greet(user_input)
在这个例子中,定义了一个函数greet
,并在while循环中调用该函数来打印用户输入的名字。
九、循环中的列表生成器和字典生成器
在Python中,可以使用列表生成器和字典生成器在循环中生成新的列表或字典。
1、列表生成器
例如,生成一个包含1到10的平方的列表:
squares = [x * x for x in range(1, 11)]
print(squares)
在这个例子中,使用列表生成器在for循环中生成一个包含1到10的平方的列表。
2、字典生成器
例如,生成一个包含数字及其平方的字典:
squares_dict = {x: x * x for x in range(1, 11)}
print(squares_dict)
在这个例子中,使用字典生成器在for循环中生成一个包含数字及其平方的字典。
十、循环中的递归调用
在循环中,可以使用递归调用来解决问题。
1、递归函数
例如,定义一个递归函数计算阶乘:
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
2、在循环中调用递归函数
例如,使用递归函数计算多个数字的阶乘:
numbers = [3, 4, 5]
for number in numbers:
print(f"Factorial of {number} is {factorial(number)}")
在这个例子中,定义了一个递归函数factorial
,并在for循环中调用该函数来计算多个数字的阶乘。
十一、循环中的多线程和多进程
在循环中,可以使用多线程和多进程来提高程序的执行效率。
1、多线程
例如,使用多线程在循环中执行并发操作:
import threading
def print_number(number):
print(f"Number: {number}")
threads = []
for i in range(5):
thread = threading.Thread(target=print_number, args=(i,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在这个例子中,使用多线程在for循环中执行并发操作。
2、多进程
例如,使用多进程在循环中执行并发操作:
import multiprocessing
def print_number(number):
print(f"Number: {number}")
processes = []
for i in range(5):
process = multiprocessing.Process(target=print_number, args=(i,))
processes.append(process)
process.start()
for process in processes:
process.join()
在这个例子中,使用多进程在for循环中执行并发操作。
十二、循环中的日志记录
在循环中,可以使用日志记录来跟踪程序的执行情况。
1、配置日志记录
例如,配置日志记录并在循环中记录日志:
import logging
logging.basicConfig(level=logging.INFO)
for i in range(5):
logging.info(f"Loop iteration: {i}")
在这个例子中,配置了日志记录,并在for循环中记录日志信息。
2、使用不同的日志级别
例如,在循环中使用不同的日志级别记录日志:
import logging
logging.basicConfig(level=logging.DEBUG)
for i in range(5):
if i % 2 == 0:
logging.debug(f"Even number: {i}")
else:
logging.warning(f"Odd number: {i}")
在这个例子中,使用不同的日志级别记录循环中的日志信息。
十三、循环中的文件操作
在循环中,可以执行文件操作,如读取和写入文件。
1、读取文件
例如,在循环中逐行读取文件内容:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
在这个例子中,使用with语句打开文件,并在for循环中逐行读取文件内容。
2、写入文件
例如,在循环中写入文件内容:
with open('output.txt', 'w') as file:
for i in range(5):
file.write(f"Line {i}\n")
在这个例子中,使用with语句打开文件,并在for循环中写入文件内容。
十四、循环中的数据处理
在循环中,可以执行数据处理操作,如过滤和转换数据。
1、数据过滤
例如,过滤列表中的偶数:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [number for number in numbers if number % 2 == 0]
print(even_numbers)
在这个例子中,使用列表生成器在for循环中过滤列表中的偶数。
2、数据转换
例如,将列表中的字符串转换为大写:
words = ['apple', 'banana', 'cherry']
uppercase_words = [word.upper() for word in words]
print(uppercase_words)
在这个例子中,使用列表生成器在for循环中将列表中的字符串转换为大写。
十五、循环中的并行计算
在循环中,可以使用并行计算来提高计算效率。
1、使用多线程并行计算
例如,使用多线程在循环中执行并行计算:
import concurrent.futures
def square(x):
return x * x
numbers = [1, 2, 3, 4, 5]
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(square, numbers))
print(results)
在这个例子中,使用concurrent.futures模块在for循环中执行并行计算。
2、使用多进程并行计算
例如,使用多进程在循环中执行并行计算:
import concurrent.futures
def square(x):
return x * x
numbers = [1, 2, 3, 4, 5]
with concurrent.futures.ProcessPoolExecutor() as executor:
results = list(executor.map(square, numbers))
print(results)
在这个例子中,使用concurrent.futures模块在for循环中执行并行计算。
十六、循环中的图形用户界面(GUI)
在循环中,可以创建和更新图形用户界面。
1、使用Tkinter创建简单的GUI
例如,使用Tkinter创建一个简单的GUI窗口:
import tkinter as tk
root = tk.Tk()
root.title("Simple GUI")
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
root.mainloop()
在这个例子中,使用Tkinter模块创建一个简单的GUI窗口,并在窗口中显示一个标签。
2、在循环中更新GUI
例如,在循环中更新GUI窗口中的标签:
import tkinter as tk
root = tk.Tk()
root.title("Counter")
counter = tk.IntVar()
label = tk.Label(root, textvariable=counter)
label.pack()
def update_counter():
for i in range(10):
counter.set(i)
root.update()
root.after(500)
button = tk.Button(root, text="Start", command=update_counter)
button.pack()
root.mainloop()
在这个例子中,使用Tkinter模块创建一个GUI窗口,并在for循环中更新窗口中的标签。
十七、循环中的网络操作
在循环中,可以执行网络操作,如发送HTTP请求和处理响应。
1、发送HTTP请求
例如,在循环中发送多个HTTP请求:
import requests
urls = ['http://example.com', 'http://example.org', 'http://example.net']
for url in urls:
response = requests.get(url)
print(f"URL: {url}, Status Code: {response.status_code}")
在这个例子中,使用requests模块在for循环中发送多个HTTP请求,并打印响应状态码。
2、处理HTTP响应
例如,在循环中处理HTTP响应数据:
import requests
urls = ['http://example.com', 'http://example.org', 'http://example.net']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
print(f"URL: {url}, Content Length: {len(response.content)}")
在这个例子中,使用requests模块在for循环中处理HTTP响应数据,并打印响应内容的长度。
十八、循环中的数据库操作
在循环中,可以执行数据库操作,如查询和插入数据。
1、查询数据库
例如,在循环中查询数据库中的数据:
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
相关问答FAQs:
如何在Python中判断一个循环是否会执行?
在Python中,可以通过检查循环条件的初始状态来判断一个循环体是否会执行。对于for
循环,检查可迭代对象是否为空;对于while
循环,查看条件表达式的初始值是否为真。如果条件不满足,循环体将不会执行。
在Python中如何优化循环体的性能?
要优化循环体的性能,可以考虑使用列表推导式、生成器表达式或内置函数如map()
和filter()
。这些方法通常比传统的for
循环更高效。此外,避免在循环中进行重复计算和不必要的函数调用也能显著提升性能。
如何在Python循环中判断特定条件并提前退出?
在Python的循环中,可以使用break
语句提前退出循环。当在循环体内某个条件成立时,可以通过if
语句结合break
来实现。例如,在搜索特定元素时,一旦找到目标,就可以立刻结束循环,从而提高效率。
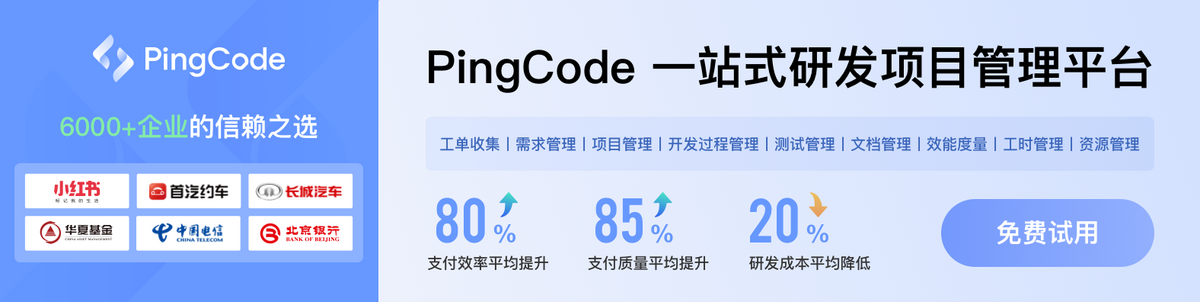