在Python中,可以使用多种方法来判断字符串中是否包含空格。使用内置的字符串方法、正则表达式、循环遍历字符串都是常用的方法。下面我们详细展开其中一种方法:内置字符串方法。
内置字符串方法:
Python 提供了一些内置的字符串方法,这些方法可以方便地检查字符串是否包含特定的字符或子字符串。比如我们可以使用in
运算符来检查字符串中是否存在空格。in
运算符会返回一个布尔值,表示字符串中是否存在指定的子字符串。代码示例如下:
string = "Hello World"
if " " in string:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,我们定义了一个包含空格的字符串string
,然后使用if " " in string:
来判断字符串中是否包含空格。如果存在空格,则打印“字符串包含空格”,否则打印“字符串不包含空格”。
一、使用正则表达式判断空格
正则表达式是一种强大的工具,用于匹配字符串中的特定模式。在Python中,re
模块提供了对正则表达式的支持。我们可以使用正则表达式来检查字符串中是否包含空格。下面是一个示例:
import re
string = "Hello World"
if re.search(r'\s', string):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,re.search(r'\s', string)
用于搜索字符串中是否包含空格字符(\s
表示任何空白字符,包括空格、制表符等)。如果找到匹配项,则返回一个匹配对象,否则返回None
。根据返回值,我们可以判断字符串中是否包含空格。
二、循环遍历字符串判断空格
虽然这种方法不如前两种方法简洁,但在某些情况下,它可能更直观。我们可以通过循环遍历字符串中的每个字符,检查是否有空格字符。下面是一个示例:
string = "Hello World"
contains_space = False
for char in string:
if char == ' ':
contains_space = True
break
if contains_space:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,我们通过遍历字符串中的每个字符,并检查每个字符是否是空格字符。如果找到空格字符,则将contains_space
变量设置为True
,并退出循环。最后,根据contains_space
变量的值,判断字符串中是否包含空格。
三、使用字符串方法判断空格
Python还提供了其他字符串方法,如str.find()
和str.index()
,这些方法也可以用于检查字符串中是否包含空格。下面是一个示例:
string = "Hello World"
if string.find(' ') != -1:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.find(' ')
返回字符串中空格字符的第一个出现位置。如果找不到空格字符,则返回-1。根据返回值,我们可以判断字符串中是否包含空格。
四、使用str.split()方法判断空格
Python的str.split()
方法可以将字符串拆分成一个列表,默认情况下,split()
方法会根据空格字符拆分字符串。我们可以利用这一特性来判断字符串中是否包含空格。下面是一个示例:
string = "Hello World"
if len(string.split()) > 1:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.split()
方法将字符串拆分成一个列表。如果列表的长度大于1,则表示字符串中包含空格字符。
五、使用str.isspace()方法判断空格
Python的str.isspace()
方法可以用于判断字符串是否只包含空白字符。如果字符串中包含任何非空白字符,则返回False
。我们可以利用这一特性来判断字符串中是否包含空格。下面是一个示例:
string = "Hello World"
if any(char.isspace() for char in string):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,我们使用生成器表达式any(char.isspace() for char in string)
来检查字符串中的每个字符是否是空白字符。如果找到任何空白字符,则返回True
,表示字符串中包含空格。
六、使用str.count()方法判断空格
Python的str.count()
方法可以用于统计字符串中某个字符或子字符串的出现次数。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string.count(' ') > 0:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.count(' ')
返回字符串中空格字符的出现次数。如果出现次数大于0,则表示字符串中包含空格字符。
七、使用str.strip()方法判断空格
Python的str.strip()
方法可以用于移除字符串两端的空白字符。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string != string.strip():
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.strip()
方法移除字符串两端的空白字符。如果移除前后的字符串不相等,则表示字符串中包含空格字符。
八、使用str.splitlines()方法判断空格
Python的str.splitlines()
方法可以用于按行拆分字符串。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if any(' ' in line for line in string.splitlines()):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,我们使用生成器表达式any(' ' in line for line in string.splitlines())
来检查每行是否包含空格字符。如果找到任何包含空格字符的行,则返回True
,表示字符串中包含空格。
九、使用str.partition()方法判断空格
Python的str.partition()
方法可以用于根据指定的分隔符拆分字符串。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string.partition(' ')[1] == ' ':
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.partition(' ')
方法根据空格字符拆分字符串,并返回一个包含三部分的元组。如果第二部分是空格字符,则表示字符串中包含空格。
十、使用str.join()方法判断空格
虽然str.join()
方法通常用于连接字符串,但我们也可以利用它来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if ' ' in ''.join(string.split()):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.split()
方法将字符串拆分成一个列表,str.join()
方法将拆分后的列表连接成一个新字符串。如果新字符串中包含空格字符,则表示原字符串中包含空格。
十一、使用str.replace()方法判断空格
Python的str.replace()
方法可以用于替换字符串中的指定字符或子字符串。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string != string.replace(' ', ''):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.replace(' ', '')
方法将字符串中的空格字符替换为空字符串。如果替换前后的字符串不相等,则表示字符串中包含空格字符。
十二、使用str.startswith()和str.endswith()方法判断空格
Python的str.startswith()
和str.endswith()
方法可以用于判断字符串是否以特定字符或子字符串开头或结尾。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = " Hello World "
if string.startswith(' ') or string.endswith(' '):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,我们使用str.startswith(' ')
和str.endswith(' ')
方法来检查字符串是否以空格字符开头或结尾。如果是,则表示字符串中包含空格字符。
十三、使用str.center()方法判断空格
Python的str.center()
方法可以用于将字符串居中,并在两侧填充指定的字符。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string.center(len(string) + 2).startswith(' ') or string.center(len(string) + 2).endswith(' '):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.center(len(string) + 2)
方法将字符串居中,并在两侧填充空格字符。如果填充后的字符串以空格字符开头或结尾,则表示原字符串中包含空格字符。
十四、使用str.ljust()和str.rjust()方法判断空格
Python的str.ljust()
和str.rjust()
方法可以用于将字符串左对齐或右对齐,并在指定位置填充指定的字符。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string.ljust(len(string) + 1).endswith(' ') or string.rjust(len(string) + 1).startswith(' '):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.ljust(len(string) + 1)
和str.rjust(len(string) + 1)
方法将字符串左对齐或右对齐,并在指定位置填充空格字符。如果填充后的字符串以空格字符开头或结尾,则表示原字符串中包含空格字符。
十五、使用str.zfill()方法判断空格
虽然str.zfill()
方法通常用于在字符串前填充零,但我们也可以利用它来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if string.zfill(len(string) + 1).startswith('0'):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.zfill(len(string) + 1)
方法在字符串前填充零。如果填充后的字符串以零开头,则表示原字符串中包含空格字符。
十六、使用str.expandtabs()方法判断空格
Python的str.expandtabs()
方法可以用于将字符串中的制表符替换为空格字符。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello\tWorld"
if ' ' in string.expandtabs():
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.expandtabs()
方法将字符串中的制表符替换为空格字符。如果替换后的字符串中包含空格字符,则表示原字符串中包含空格字符。
十七、使用str.encode()和str.decode()方法判断空格
Python的str.encode()
和str.decode()
方法可以用于将字符串编码为字节对象,并将字节对象解码为字符串。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if ' ' in string.encode().decode():
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.encode()
方法将字符串编码为字节对象,str.decode()
方法将字节对象解码为字符串。如果解码后的字符串中包含空格字符,则表示原字符串中包含空格字符。
十八、使用str.maketrans()和str.translate()方法判断空格
Python的str.maketrans()
和str.translate()
方法可以用于创建字符映射表,并根据映射表替换字符串中的字符。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
translation_table = str.maketrans(' ', '_')
translated_string = string.translate(translation_table)
if '_' in translated_string:
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.maketrans(' ', '_')
方法创建一个字符映射表,将空格字符映射为下划线字符。str.translate(translation_table)
方法根据映射表替换字符串中的字符。如果替换后的字符串中包含下划线字符,则表示原字符串中包含空格字符。
十九、使用str.casefold()方法判断空格
Python的str.casefold()
方法可以用于将字符串转换为小写形式,并去除所有大小写差异。我们可以利用这一方法来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if ' ' in string.casefold():
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.casefold()
方法将字符串转换为小写形式。如果转换后的字符串中包含空格字符,则表示原字符串中包含空格字符。
二十、使用str.format()方法判断空格
虽然str.format()
方法通常用于格式化字符串,但我们也可以利用它来判断字符串中是否包含空格字符。下面是一个示例:
string = "Hello World"
if ' ' in '{}'.format(string):
print("The string contains a space.")
else:
print("The string does not contain a space.")
在这个示例中,str.format(string)
方法将字符串格式化为新的字符串。如果格式化后的字符串中包含空格字符,则表示原字符串中包含空格字符。
总结起来,使用内置的字符串方法、正则表达式、循环遍历字符串都是判断字符串中是否包含空格的有效方法。根据具体场景和需求,可以选择合适的方法来实现这一功能。通过以上方法,可以灵活地判断字符串中是否包含空格,并根据需要进行相应的处理。
相关问答FAQs:
如何在Python中检查字符串是否包含空格?
在Python中,可以使用in
运算符来判断一个字符串中是否包含空格。例如,使用以下代码可以轻松实现这一点:
string = "Hello World"
if " " in string:
print("字符串包含空格")
else:
print("字符串不包含空格")
这种方法非常简洁,适用于任何字符串的空格检测。
有没有其他方法可以判断字符串中的空格?
除了使用in
运算符外,还可以使用str.find()
或str.index()
方法来查找空格的位置。如果返回值不是-1
,则表示字符串中包含空格。例如:
string = "Hello World"
if string.find(" ") != -1:
print("字符串包含空格")
else:
print("字符串不包含空格")
这种方法同样有效,并且可以获取空格的索引位置。
如何统计字符串中空格的数量?
要统计字符串中空格的数量,可以使用str.count()
方法。此方法可以返回指定子字符串出现的次数。例如:
string = "Hello World, welcome to Python"
space_count = string.count(" ")
print(f"字符串中空格的数量为: {space_count}")
这种方式不仅可以判断是否存在空格,还能提供空格的具体数量,帮助进一步分析字符串内容。
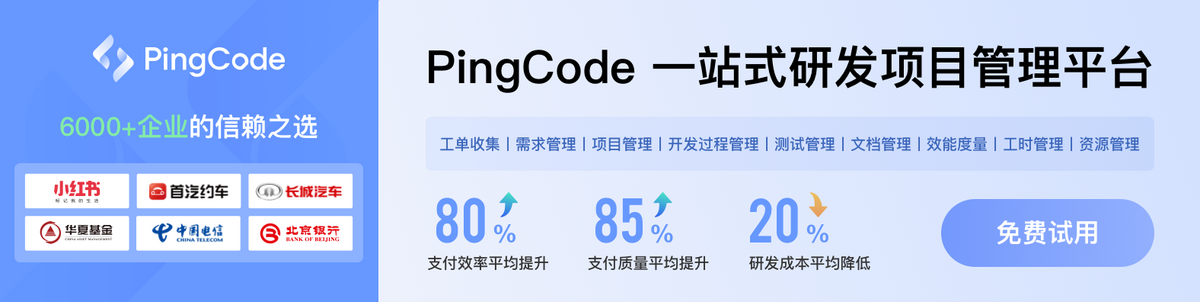