Python爬取网页音乐的主要步骤包括:选择合适的网页爬虫库、解析网页内容、获取音乐链接、处理反爬机制。 其中,选择合适的网页爬虫库是最重要的。推荐使用requests
和BeautifulSoup
库来进行网页请求和解析。下面我将详细介绍如何使用这些工具爬取网页音乐。
一、选择合适的网页爬虫库
-
Requests库:
requests
库是一个简单易用的HTTP库,可以用来发送HTTP请求,获取网页内容。相比于Python自带的urllib
库,requests
更为简洁和强大。安装方法:
pip install requests
-
BeautifulSoup库:
BeautifulSoup
是一个用于解析HTML和XML文档的库,可以用来提取网页中的特定内容。与正则表达式相比,BeautifulSoup
更容易理解和使用。安装方法:
pip install beautifulsoup4
-
lxml库:
lxml
库是一个高效的HTML和XML解析库,可以与BeautifulSoup
配合使用,提升解析速度。安装方法:
pip install lxml
二、解析网页内容
-
发送HTTP请求:
使用
requests
库发送HTTP请求,获取网页内容。例如:import requests
url = 'https://example.com/music-page'
response = requests.get(url)
html_content = response.text
-
解析HTML内容:
使用
BeautifulSoup
解析获取到的HTML内容。例如:from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
-
提取音乐链接:
使用
BeautifulSoup
提取网页中包含音乐链接的标签。例如:music_links = []
for link in soup.find_all('a'):
href = link.get('href')
if href and href.endswith('.mp3'):
music_links.append(href)
三、获取音乐链接
-
分析网页结构:
在提取音乐链接之前,需要分析网页结构,确定音乐链接所在的标签。例如,许多音乐网站的音乐链接可能位于
<a>
标签或<audio>
标签中。 -
编写提取规则:
根据分析结果,编写提取规则。例如:
for audio in soup.find_all('audio'):
src = audio.get('src')
if src:
music_links.append(src)
四、处理反爬机制
-
设置请求头:
为了避免被网站的反爬机制拦截,可以设置请求头,模拟浏览器发送请求。例如:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'
}
response = requests.get(url, headers=headers)
-
使用代理:
如果网站对IP访问频率有限制,可以使用代理来避免被封。例如:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, headers=headers, proxies=proxies)
-
模拟登录:
有些网站需要登录才能访问音乐链接,可以使用
requests
库模拟登录。例如:login_url = 'https://example.com/login'
payload = {
'username': 'your_username',
'password': 'your_password'
}
session = requests.Session()
session.post(login_url, data=payload)
response = session.get(url)
五、下载音乐文件
- 编写下载函数:
编写函数下载提取到的音乐文件。例如:
import os
def download_music(url, folder='music'):
if not os.path.exists(folder):
os.makedirs(folder)
response = requests.get(url)
file_name = os.path.join(folder, url.split('/')[-1])
with open(file_name, 'wb') as file:
file.write(response.content)
for link in music_links:
download_music(link)
六、实例演示
以下是一个完整的实例演示:
import requests
from bs4 import BeautifulSoup
import os
def fetch_music_links(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'
}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'lxml')
music_links = []
for link in soup.find_all('a'):
href = link.get('href')
if href and href.endswith('.mp3'):
music_links.append(href)
return music_links
def download_music(url, folder='music'):
if not os.path.exists(folder):
os.makedirs(folder)
response = requests.get(url)
file_name = os.path.join(folder, url.split('/')[-1])
with open(file_name, 'wb') as file:
file.write(response.content)
if __name__ == '__main__':
music_page_url = 'https://example.com/music-page'
links = fetch_music_links(music_page_url)
for link in links:
download_music(link)
七、处理其他复杂情况
-
处理动态内容:
有些网站的内容是通过JavaScript动态加载的,使用
requests
库可能无法获取完整的内容。这时可以使用Selenium
库模拟浏览器操作,获取动态加载的内容。安装方法:
pip install selenium
使用示例:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = 'https://example.com/music-page'
driver = webdriver.Chrome()
driver.get(url)
music_links = []
for element in driver.find_elements(By.TAG_NAME, 'a'):
href = element.get_attribute('href')
if href and href.endswith('.mp3'):
music_links.append(href)
driver.quit()
-
处理分页:
有些网站的音乐列表分布在多个分页中,需要处理分页逻辑。例如:
base_url = 'https://example.com/music-page?page='
page = 1
while True:
url = base_url + str(page)
links = fetch_music_links(url)
if not links:
break
for link in links:
download_music(link)
page += 1
-
处理验证码:
有些网站在访问频率较高时,会出现验证码。可以使用第三方验证码识别服务或手动输入验证码来解决这个问题。
八、总结
Python爬取网页音乐的过程涉及多个步骤,包括选择合适的网页爬虫库、解析网页内容、获取音乐链接、处理反爬机制等。在实际操作中,需要根据具体情况调整代码,例如处理动态内容、分页和验证码等问题。通过不断实践和优化,可以提高爬虫的效率和稳定性。
相关问答FAQs:
如何使用Python爬取网页上的音乐资源?
使用Python爬取网页音乐通常涉及几个步骤,包括发送HTTP请求、解析网页内容以及下载音乐文件。您可以使用库如Requests来获取网页数据,并使用BeautifulSoup或lxml来解析HTML。下载音乐文件可以通过直接访问文件的URL并使用Requests库中的get
方法完成。
在爬取音乐时如何处理反爬虫机制?
许多网站会实施反爬虫机制以保护其内容。为了有效地爬取音乐,您可以使用代理服务器来隐藏您的IP地址,设置请求头以模拟浏览器行为,甚至使用时间间隔来降低请求频率,从而减少被封禁的风险。此外,您还可以利用Selenium等工具模拟用户在浏览器中的操作。
爬取的音乐文件可以存储在哪里?
下载的音乐文件可以存储在本地计算机的指定文件夹中,您可以选择使用Python的os
库来创建文件夹并管理文件存储。为了便于管理,可以根据歌曲的名称或其他属性命名文件,并确保文件格式与原始音频格式一致。这样可以确保在后续播放和整理时的便利性。
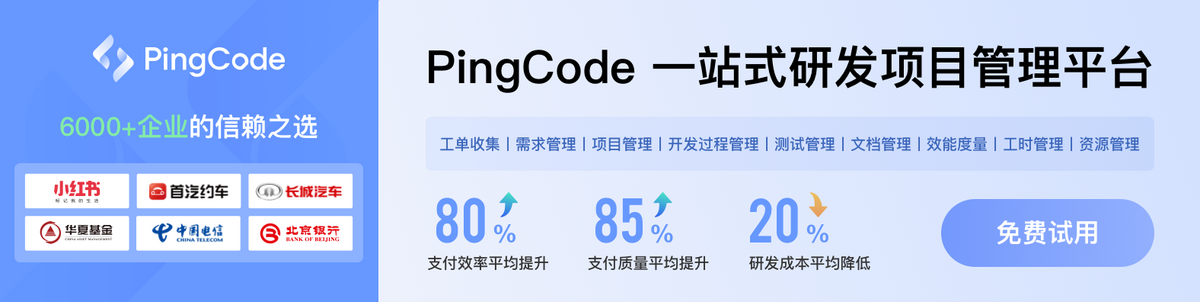