用Python判断图片白边的方法包括:计算边缘像素的颜色值、使用图像处理库检测白色区域、通过直方图分析白色像素的分布。 其中,计算边缘像素的颜色值是一种简单而有效的方法。我们可以通过加载图像并提取其边缘像素,然后计算这些像素的颜色值是否接近白色来判断是否存在白边。
一、加载图像
在Python中,我们可以使用PIL(Pillow)库来加载和处理图像。首先,我们需要安装Pillow库:
pip install pillow
然后,我们可以使用以下代码加载一张图片:
from PIL import Image
加载图像
image = Image.open('image.jpg')
二、提取边缘像素
我们需要提取图像的四个边缘像素,以便后续进行颜色值的计算。可以使用以下代码实现:
import numpy as np
将图像转换为NumPy数组
image_array = np.array(image)
提取边缘像素
top_edge = image_array[0, :]
bottom_edge = image_array[-1, :]
left_edge = image_array[:, 0]
right_edge = image_array[:, -1]
三、计算颜色值
接下来,我们需要计算提取出的边缘像素的颜色值,判断这些颜色值是否接近白色。通常,白色的RGB值为(255, 255, 255)。我们可以设置一个阈值来判断颜色值是否接近白色:
# 定义白色的RGB值和阈值
white_color = np.array([255, 255, 255])
threshold = 240
判断边缘像素是否接近白色
def is_white_edge(edge):
return np.all(edge > threshold, axis=1).mean() > 0.95
判断四个边缘是否存在白边
has_white_top = is_white_edge(top_edge)
has_white_bottom = is_white_edge(bottom_edge)
has_white_left = is_white_edge(left_edge)
has_white_right = is_white_edge(right_edge)
输出结果
print(f"Top edge has white border: {has_white_top}")
print(f"Bottom edge has white border: {has_white_bottom}")
print(f"Left edge has white border: {has_white_left}")
print(f"Right edge has white border: {has_white_right}")
四、使用图像处理库检测白色区域
除了上述方法,还可以使用OpenCV库进行图像处理。首先,我们需要安装OpenCV库:
pip install opencv-python
然后,我们可以使用以下代码检测白色区域:
import cv2
加载图像
image = cv2.imread('image.jpg')
转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
二值化处理,将白色区域变为255,其他区域变为0
_, binary_image = cv2.threshold(gray_image, 240, 255, cv2.THRESH_BINARY)
提取四个边缘的白色像素
top_edge_white = binary_image[0, :]
bottom_edge_white = binary_image[-1, :]
left_edge_white = binary_image[:, 0]
right_edge_white = binary_image[:, -1]
判断四个边缘是否存在白边
has_white_top = np.mean(top_edge_white) > 240
has_white_bottom = np.mean(bottom_edge_white) > 240
has_white_left = np.mean(left_edge_white) > 240
has_white_right = np.mean(right_edge_white) > 240
输出结果
print(f"Top edge has white border: {has_white_top}")
print(f"Bottom edge has white border: {has_white_bottom}")
print(f"Left edge has white border: {has_white_left}")
print(f"Right edge has white border: {has_white_right}")
五、通过直方图分析白色像素的分布
直方图是一种非常有效的图像分析工具。我们可以通过计算图像的颜色直方图来分析白色像素的分布情况。以下是使用PIL和NumPy计算图像直方图的方法:
# 计算图像的颜色直方图
histogram = image.histogram()
提取红色、绿色和蓝色通道的直方图
red_hist = histogram[0:256]
green_hist = histogram[256:512]
blue_hist = histogram[512:768]
计算白色像素的数量
white_pixels = sum([red_hist[255], green_hist[255], blue_hist[255]])
计算白色像素的比例
total_pixels = image.size[0] * image.size[1]
white_ratio = white_pixels / total_pixels
输出结果
print(f"White pixel ratio: {white_ratio:.2%}")
六、综合判断
为了提高判断的准确性,可以将多种方法结合使用,综合分析图像的边缘像素和整体白色像素比例。以下是一个综合判断的示例代码:
# 综合判断图像是否存在白边
def has_white_border(image_path):
image = Image.open(image_path)
image_array = np.array(image)
# 提取边缘像素
top_edge = image_array[0, :]
bottom_edge = image_array[-1, :]
left_edge = image_array[:, 0]
right_edge = image_array[:, -1]
# 定义白色的RGB值和阈值
white_color = np.array([255, 255, 255])
threshold = 240
# 判断边缘像素是否接近白色
def is_white_edge(edge):
return np.all(edge > threshold, axis=1).mean() > 0.95
# 判断四个边缘是否存在白边
has_white_top = is_white_edge(top_edge)
has_white_bottom = is_white_edge(bottom_edge)
has_white_left = is_white_edge(left_edge)
has_white_right = is_white_edge(right_edge)
# 计算图像的颜色直方图
histogram = image.histogram()
red_hist = histogram[0:256]
green_hist = histogram[256:512]
blue_hist = histogram[512:768]
white_pixels = sum([red_hist[255], green_hist[255], blue_hist[255]])
total_pixels = image.size[0] * image.size[1]
white_ratio = white_pixels / total_pixels
# 综合判断
has_white_border = (
has_white_top or has_white_bottom or has_white_left or has_white_right
) and white_ratio > 0.1
return has_white_border
输出结果
image_path = 'image.jpg'
print(f"Image has white border: {has_white_border(image_path)}")
通过以上方法,我们可以有效地判断图像是否存在白边,并且可以根据需要选择适合的方法进行应用。希望这些方法能够帮助你更好地处理图像白边的检测问题。
相关问答FAQs:
如何使用Python检测图片中的白边?
在Python中,可以使用OpenCV和Pillow等库来检测图片的白边。具体方法包括加载图像,转换为灰度图,应用阈值,然后计算边缘区域的像素值,从而判断白边的存在与否。
我需要安装哪些Python库来处理图片白边?
要处理图片白边,推荐安装OpenCV和Pillow。可以使用以下命令安装:
pip install opencv-python pillow
这两个库提供了强大的图像处理功能,适合用于白边检测和处理。
检测到白边后,我如何处理这些白边?
一旦检测到图片中的白边,可以通过裁剪、填充或更改图像尺寸等方式进行处理。使用OpenCV的cv2.resize()
或cv2.crop()
方法可以轻松地对图像进行调整,以去除不需要的白边。
为什么我的白边检测效果不理想?
白边检测效果可能受到多个因素影响,包括图像质量、白边颜色的微妙差异以及图像背景的复杂性。尝试调整阈值设置,或使用不同的图像预处理技术(如模糊处理)来提高检测效果。
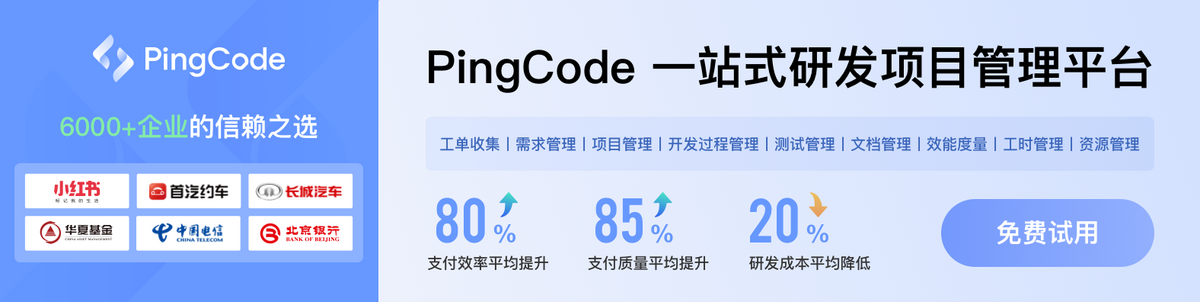