Python获取响应的header可以使用requests库、通过response.headers属性、使用requests库的response对象获取、使用response对象中的属性
首先,我们可以通过使用requests库来发送HTTP请求并接收响应。接收到响应后,可以通过response.headers属性来获取响应的header信息。header信息是以字典的形式存储的,因此可以轻松访问特定的header字段。例如:
import requests
response = requests.get('https://example.com')
headers = response.headers
print(headers)
在这个例子中,我们使用requests库发送了一个GET请求到'https://example.com',然后通过response.headers属性打印了响应的header。
requests库的response对象获取header信息非常方便,因为它将所有的header信息存储在一个字典中。
一、安装requests库
要使用requests库,我们需要先安装它。如果你还没有安装requests库,可以使用以下命令来安装:
pip install requests
安装完成后,我们可以开始使用requests库来发送HTTP请求并获取响应的header信息。
二、发送HTTP请求
使用requests库发送HTTP请求非常简单。我们可以使用requests.get()方法来发送一个GET请求,使用requests.post()方法来发送一个POST请求,等等。以下是一个发送GET请求的例子:
import requests
response = requests.get('https://example.com')
三、获取响应的header
获取响应的header信息也非常简单。我们只需要通过response.headers属性来获取header信息。header信息是以字典的形式存储的,因此我们可以轻松访问特定的header字段。以下是一个获取header信息的例子:
import requests
response = requests.get('https://example.com')
headers = response.headers
print(headers)
在这个例子中,我们使用requests.get()方法发送了一个GET请求到'https://example.com',然后通过response.headers属性打印了响应的header信息。
四、访问特定的header字段
由于header信息是以字典的形式存储的,因此我们可以轻松访问特定的header字段。以下是一个访问特定header字段的例子:
import requests
response = requests.get('https://example.com')
content_type = response.headers['Content-Type']
print('Content-Type:', content_type)
在这个例子中,我们获取了响应的Content-Type字段并打印了它的值。
五、处理header信息
在实际应用中,我们可能需要对header信息进行处理。例如,我们可能需要检查特定的header字段是否存在,或者根据header字段的值来执行不同的操作。以下是一些处理header信息的例子:
1、检查特定的header字段是否存在
import requests
response = requests.get('https://example.com')
if 'Content-Type' in response.headers:
print('Content-Type header is present')
else:
print('Content-Type header is not present')
在这个例子中,我们检查了Content-Type字段是否存在于响应的header中。
2、根据header字段的值来执行不同的操作
import requests
response = requests.get('https://example.com')
content_type = response.headers.get('Content-Type', '')
if content_type == 'application/json':
print('Response is in JSON format')
else:
print('Response is not in JSON format')
在这个例子中,我们根据Content-Type字段的值来执行不同的操作。如果Content-Type字段的值是'application/json',我们就认为响应是JSON格式的。
六、处理多个header字段
有时,我们可能需要处理多个header字段。以下是一些处理多个header字段的例子:
1、获取所有header字段及其值
import requests
response = requests.get('https://example.com')
for header, value in response.headers.items():
print(f'{header}: {value}')
在这个例子中,我们遍历了所有的header字段及其值并打印了它们。
2、根据多个header字段的值来执行不同的操作
import requests
response = requests.get('https://example.com')
content_type = response.headers.get('Content-Type', '')
cache_control = response.headers.get('Cache-Control', '')
if content_type == 'application/json' and 'no-cache' in cache_control:
print('Response is in JSON format and should not be cached')
else:
print('Response does not meet the criteria')
在这个例子中,我们根据Content-Type字段和Cache-Control字段的值来执行不同的操作。如果Content-Type字段的值是'application/json'并且Cache-Control字段包含'no-cache',我们就认为响应是JSON格式的并且不应该被缓存。
七、处理header字段的大小写问题
HTTP header字段是不区分大小写的。因此,在处理header字段时,我们需要注意这一点。requests库的response.headers属性会将所有的header字段转换为小写,以确保我们在访问header字段时不需要考虑大小写问题。
1、访问header字段时不考虑大小写
import requests
response = requests.get('https://example.com')
content_type = response.headers.get('content-type', '')
print('Content-Type:', content_type)
在这个例子中,我们访问了content-type字段,而不考虑其大小写。
八、处理header字段的默认值
在访问header字段时,如果header字段不存在,我们可以为其提供一个默认值。这样可以避免在header字段不存在时出现KeyError异常。
1、为header字段提供默认值
import requests
response = requests.get('https://example.com')
content_type = response.headers.get('Content-Type', 'application/octet-stream')
print('Content-Type:', content_type)
在这个例子中,如果Content-Type字段不存在,我们会使用'application/octet-stream'作为默认值。
九、处理响应的Set-Cookie字段
Set-Cookie字段是HTTP响应中常见的一个字段,用于设置cookie。我们可以通过response.headers属性来获取Set-Cookie字段的值。
1、获取Set-Cookie字段的值
import requests
response = requests.get('https://example.com')
set_cookie = response.headers.get('Set-Cookie', '')
print('Set-Cookie:', set_cookie)
在这个例子中,我们获取了响应的Set-Cookie字段并打印了它的值。
十、处理多个Set-Cookie字段
有时,HTTP响应中可能包含多个Set-Cookie字段。requests库会将这些字段的值连接成一个字符串,并用逗号分隔。
1、处理多个Set-Cookie字段
import requests
response = requests.get('https://example.com')
set_cookies = response.headers.get('Set-Cookie', '').split(', ')
for set_cookie in set_cookies:
print('Set-Cookie:', set_cookie)
在这个例子中,我们将Set-Cookie字段的值拆分成多个cookie并逐个打印它们。
十一、使用requests.Session来管理cookie
在使用requests库时,我们可以通过requests.Session对象来管理cookie。requests.Session对象会自动处理cookie的设置和发送。
1、使用requests.Session来管理cookie
import requests
session = requests.Session()
response = session.get('https://example.com')
获取响应的Set-Cookie字段
set_cookie = response.headers.get('Set-Cookie', '')
print('Set-Cookie:', set_cookie)
发送另一个请求,并自动带上cookie
response = session.get('https://example.com/protected')
print(response.text)
在这个例子中,我们使用requests.Session对象发送了一个GET请求到'https://example.com',然后获取了响应的Set-Cookie字段。接着,我们发送了另一个请求到'https://example.com/protected',requests.Session对象会自动带上之前设置的cookie。
十二、处理自定义header字段
在发送HTTP请求时,我们可以通过requests库来设置自定义的header字段。我们可以在请求方法中传递一个headers参数,该参数是一个包含header字段及其值的字典。
1、设置自定义header字段
import requests
headers = {
'User-Agent': 'my-app/0.0.1',
'Authorization': 'Bearer my-token'
}
response = requests.get('https://example.com', headers=headers)
print(response.text)
在这个例子中,我们在发送GET请求时设置了自定义的User-Agent和Authorization header字段。
十三、处理响应的header字段
在处理响应的header字段时,我们可以使用response.headers属性来获取header信息。header信息是以字典的形式存储的,因此我们可以轻松访问特定的header字段。
1、访问响应的header字段
import requests
response = requests.get('https://example.com')
content_type = response.headers['Content-Type']
print('Content-Type:', content_type)
在这个例子中,我们访问了响应的Content-Type字段并打印了它的值。
十四、检查响应的header字段是否存在
在处理响应的header字段时,我们可能需要检查特定的header字段是否存在。我们可以使用'in'运算符来检查header字段是否存在于response.headers中。
1、检查特定的header字段是否存在
import requests
response = requests.get('https://example.com')
if 'Content-Type' in response.headers:
print('Content-Type header is present')
else:
print('Content-Type header is not present')
在这个例子中,我们检查了Content-Type字段是否存在于响应的header中。
十五、根据响应的header字段执行不同的操作
在处理响应的header字段时,我们可能需要根据header字段的值来执行不同的操作。例如,我们可以根据Content-Type字段的值来判断响应的格式,并执行相应的操作。
1、根据header字段的值执行不同的操作
import requests
response = requests.get('https://example.com')
content_type = response.headers.get('Content-Type', '')
if content_type == 'application/json':
print('Response is in JSON format')
else:
print('Response is not in JSON format')
在这个例子中,我们根据Content-Type字段的值来判断响应是否为JSON格式。
十六、处理多个响应的header字段
在处理响应的header字段时,我们可能需要处理多个header字段。我们可以遍历response.headers字典来获取所有的header字段及其值。
1、获取所有的header字段及其值
import requests
response = requests.get('https://example.com')
for header, value in response.headers.items():
print(f'{header}: {value}')
在这个例子中,我们遍历了所有的header字段及其值并打印了它们。
十七、总结
在本文中,我们介绍了如何使用requests库来获取响应的header信息,并详细描述了如何处理header信息。我们讨论了如何安装requests库、发送HTTP请求、获取响应的header、访问特定的header字段、处理header字段的大小写问题、处理header字段的默认值、处理Set-Cookie字段、使用requests.Session来管理cookie、设置自定义header字段、检查响应的header字段是否存在、根据响应的header字段执行不同的操作、处理多个响应的header字段等。
通过这些介绍,相信你已经掌握了如何使用requests库来获取和处理响应的header信息。希望本文对你有所帮助。
相关问答FAQs:
如何在Python中提取HTTP响应的header信息?
在Python中,您可以使用requests
库轻松获取HTTP响应的header信息。发送请求后,可以通过访问响应对象的.headers
属性来获取header。这是一个字典格式的对象,包含所有的header信息。示例代码如下:
import requests
response = requests.get('https://example.com')
headers = response.headers
print(headers)
使用其他库获取响应header的方式有哪些?
除了requests
库,您还可以使用http.client
或urllib
等标准库获取HTTP响应的header信息。http.client
允许直接与HTTP服务进行交互,您可以通过getresponse()
方法访问响应header。urllib
则可以通过urlopen()
获取相应的header。以下是http.client
的示例:
import http.client
conn = http.client.HTTPSConnection("example.com")
conn.request("GET", "/")
response = conn.getresponse()
print(response.getheaders())
conn.close()
如何处理获取到的header数据以便于使用?
获取到的header数据通常是以字典形式呈现,您可以轻松访问特定的header信息。例如,您可以通过键名获取特定的header值,如Content-Type
或Set-Cookie
。如果需要进一步处理这些数据,可以将其转化为JSON格式或存入数据库,以便后续使用。以下是一个提取特定header值的示例:
content_type = headers.get('Content-Type')
print(f'Content-Type: {content_type}')
这些方法可以帮助您在Python中灵活地获取和处理HTTP响应的header信息。
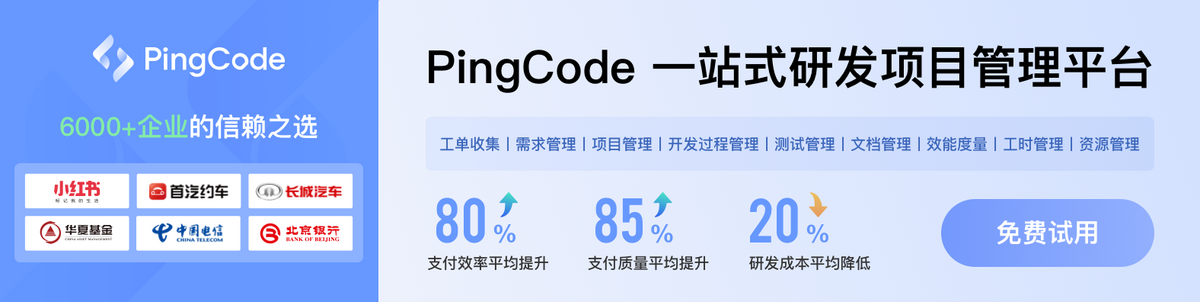