Python判定文件大小的方法有多种,包括使用os模块、Pathlib模块、shutil模块等。其中,os模块是最常用的一种方法,因为它内置于Python标准库中,且功能强大、操作简单。下面我们详细介绍使用os模块判定文件大小的方法。
在Python中,os模块提供了许多用于操作文件和目录的函数。要判定文件大小,可以使用os.path.getsize()函数。这个函数接受文件路径作为参数,并返回文件的大小(以字节为单位)。举个例子:
import os
file_path = 'example.txt'
file_size = os.path.getsize(file_path)
print(f'The size of {file_path} is {file_size} bytes.')
这个代码段将输出指定文件的大小。os.path.getsize()函数的优势在于它非常直接和高效,无需打开文件,仅通过文件路径即可获取文件大小。
一、OS模块判定文件大小
1、基本用法
os模块是Python标准库的一部分,无需额外安装。使用os.path.getsize()函数可以轻松获取文件的大小。以下是一个简单的示例:
import os
def get_file_size(file_path):
try:
size = os.path.getsize(file_path)
return size
except OSError as e:
print(f"Error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们定义了一个函数get_file_size(),它接受文件路径作为参数,并返回文件的大小。使用os.path.getsize()函数,我们可以获取文件的大小。如果出现错误(例如文件不存在),我们捕获异常并输出错误信息。
2、处理大文件
对于大文件,os.path.getsize()函数仍然适用,因为它不需要将文件内容读取到内存中。它直接从文件系统获取文件大小信息,因此即使是处理数GB甚至TB的大文件,也不会导致内存问题。
二、Pathlib模块判定文件大小
1、基本用法
Pathlib模块是Python 3.4引入的,用于面向对象地操作文件和目录。使用Pathlib模块,我们可以更直观地获取文件大小。以下是一个示例:
from pathlib import Path
def get_file_size(file_path):
try:
file = Path(file_path)
size = file.stat().st_size
return size
except FileNotFoundError as e:
print(f"Error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们使用Pathlib模块的Path类来表示文件路径。通过调用stat()方法,我们可以获取文件的统计信息,包括文件大小(st_size属性)。
2、处理符号链接
Pathlib模块还可以处理符号链接。如果文件是一个符号链接,我们可以使用resolve()方法获取实际文件的路径,然后再获取文件大小。例如:
from pathlib import Path
def get_file_size(file_path):
try:
file = Path(file_path).resolve()
size = file.stat().st_size
return size
except FileNotFoundError as e:
print(f"Error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
三、Shutil模块判定文件大小
1、基本用法
虽然shutil模块主要用于高级的文件操作(如复制、移动文件),但它也提供了获取文件大小的功能。以下是一个示例:
import shutil
def get_file_size(file_path):
try:
size = shutil.disk_usage(file_path).used
return size
except FileNotFoundError as e:
print(f"Error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们使用shutil模块的disk_usage()函数来获取文件的磁盘使用情况,包括已用空间、可用空间和总空间。通过访问used属性,我们可以获取文件的大小。
2、处理文件系统
shutil模块的disk_usage()函数还可以处理整个文件系统的磁盘使用情况。如果我们想要获取某个目录的总大小,包括其中的所有文件和子目录,我们可以递归遍历目录并累加所有文件的大小。例如:
import os
def get_directory_size(directory_path):
total_size = 0
for dirpath, dirnames, filenames in os.walk(directory_path):
for filename in filenames:
file_path = os.path.join(dirpath, filename)
try:
total_size += os.path.getsize(file_path)
except OSError as e:
print(f"Error: {e}")
return total_size
directory_path = "example_directory"
directory_size = get_directory_size(directory_path)
print(f"The total size of {directory_path} is {directory_size} bytes.")
在这个示例中,我们使用os.walk()函数递归遍历目录,并累加所有文件的大小。最终,我们可以获取整个目录的总大小。
四、结合多种方法的最佳实践
在实际应用中,我们可以结合多种方法来判定文件大小,以确保代码的健壮性和可维护性。以下是一个结合多种方法的示例:
import os
from pathlib import Path
import shutil
def get_file_size(file_path):
try:
# 尝试使用os模块
size = os.path.getsize(file_path)
return size
except OSError:
pass
try:
# 尝试使用Pathlib模块
file = Path(file_path)
size = file.stat().st_size
return size
except FileNotFoundError:
pass
try:
# 尝试使用shutil模块
size = shutil.disk_usage(file_path).used
return size
except FileNotFoundError:
pass
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们定义了一个函数get_file_size(),它依次尝试使用os模块、Pathlib模块和shutil模块来获取文件大小。如果所有方法都失败,则返回None。这样可以提高代码的健壮性,并确保在不同环境下都能正确获取文件大小。
五、处理特殊文件类型
1、压缩文件
对于压缩文件(如ZIP、TAR、GZ等),获取文件大小可能需要特殊处理。我们可以使用Python的内置模块(如zipfile、tarfile、gzip等)来处理这些文件。例如,以下是一个获取ZIP文件大小的示例:
import zipfile
def get_zip_file_size(zip_file_path):
try:
with zipfile.ZipFile(zip_file_path, 'r') as zip_file:
total_size = sum([zinfo.file_size for zinfo in zip_file.infolist()])
return total_size
except zipfile.BadZipFile as e:
print(f"Error: {e}")
return None
zip_file_path = "example.zip"
zip_file_size = get_zip_file_size(zip_file_path)
if zip_file_size is not None:
print(f"The total size of files in {zip_file_path} is {zip_file_size} bytes.")
else:
print("Could not determine the ZIP file size.")
在这个示例中,我们使用zipfile模块打开ZIP文件,并遍历文件中的所有条目,累加每个文件的大小,最终得到ZIP文件中所有文件的总大小。
2、网络文件
对于存储在远程服务器上的网络文件,我们可能需要使用HTTP请求获取文件大小。例如,使用requests模块可以轻松实现这一点:
import requests
def get_remote_file_size(url):
try:
response = requests.head(url)
size = int(response.headers.get('Content-Length', 0))
return size
except requests.RequestException as e:
print(f"Error: {e}")
return None
url = "https://example.com/example.txt"
file_size = get_remote_file_size(url)
if file_size is not None:
print(f"The size of the remote file at {url} is {file_size} bytes.")
else:
print("Could not determine the remote file size.")
在这个示例中,我们使用requests模块发送一个HEAD请求以获取文件的头信息,并从响应头中提取Content-Length字段,该字段表示文件的大小。
六、跨平台兼容性
在实际应用中,跨平台兼容性是一个重要的考量因素。不同操作系统可能有不同的文件系统和文件属性,因此在编写获取文件大小的代码时,需要考虑到这些差异。
1、Windows平台
在Windows平台上,os.path.getsize()和Pathlib模块都能正常工作。然而,对于某些特殊文件(如隐藏文件、系统文件),可能需要额外的权限。以下是一个处理权限问题的示例:
import os
def get_file_size(file_path):
try:
size = os.path.getsize(file_path)
return size
except PermissionError as e:
print(f"Error: {e}")
return None
file_path = "C:\\example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们捕获PermissionError异常,并输出错误信息。如果没有权限访问文件,我们将返回None。
2、Linux平台
在Linux平台上,获取文件大小的方法与Windows类似。然而,对于某些特殊文件(如符号链接、设备文件),可能需要特殊处理。例如:
import os
def get_file_size(file_path):
try:
if os.path.islink(file_path):
file_path = os.readlink(file_path)
size = os.path.getsize(file_path)
return size
except OSError as e:
print(f"Error: {e}")
return None
file_path = "/example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们首先检查文件是否为符号链接,如果是,则获取实际文件的路径,然后再获取文件大小。
七、性能优化
在处理大量文件或大文件时,性能优化是一个重要的考量因素。以下是一些优化建议:
1、批量处理
如果需要获取多个文件的大小,可以批量处理文件,以减少I/O操作的开销。例如:
import os
def get_files_size(file_paths):
sizes = []
for file_path in file_paths:
try:
size = os.path.getsize(file_path)
sizes.append(size)
except OSError as e:
print(f"Error: {e}")
sizes.append(None)
return sizes
file_paths = ["example1.txt", "example2.txt", "example3.txt"]
files_sizes = get_files_size(file_paths)
for file_path, file_size in zip(file_paths, files_sizes):
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print(f"Could not determine the size of {file_path}.")
在这个示例中,我们定义了一个函数get_files_size(),它接受一个文件路径列表,并返回每个文件的大小。通过批量处理文件,我们可以减少I/O操作的次数,提高性能。
2、并行处理
在处理大量文件时,可以使用多线程或多进程技术进行并行处理,以进一步提高性能。例如,使用concurrent.futures模块可以轻松实现多线程处理:
import os
from concurrent.futures import ThreadPoolExecutor
def get_file_size(file_path):
try:
size = os.path.getsize(file_path)
return size
except OSError as e:
print(f"Error: {e}")
return None
file_paths = ["example1.txt", "example2.txt", "example3.txt"]
with ThreadPoolExecutor() as executor:
files_sizes = list(executor.map(get_file_size, file_paths))
for file_path, file_size in zip(file_paths, files_sizes):
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print(f"Could not determine the size of {file_path}.")
在这个示例中,我们使用ThreadPoolExecutor创建一个线程池,并通过executor.map()方法并行处理文件大小的获取操作。这样可以显著提高处理大量文件时的性能。
八、错误处理和日志记录
在实际应用中,错误处理和日志记录是保证代码健壮性的重要手段。以下是一些建议:
1、捕获和处理异常
在获取文件大小的过程中,可能会遇到各种异常(如文件不存在、权限不足、文件系统错误等)。我们应捕获并处理这些异常,以确保程序不会因为异常而崩溃。例如:
import os
def get_file_size(file_path):
try:
size = os.path.getsize(file_path)
return size
except FileNotFoundError as e:
print(f"Error: {e}")
return None
except PermissionError as e:
print(f"Error: {e}")
return None
except OSError as e:
print(f"Error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们分别捕获FileNotFoundError、PermissionError和OSError异常,并输出错误信息。
2、日志记录
使用Python的logging模块可以方便地记录日志信息,包括错误信息、调试信息等。例如:
import os
import logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def get_file_size(file_path):
try:
size = os.path.getsize(file_path)
logging.info(f"File size of {file_path}: {size} bytes")
return size
except FileNotFoundError as e:
logging.error(f"File not found: {e}")
return None
except PermissionError as e:
logging.error(f"Permission denied: {e}")
return None
except OSError as e:
logging.error(f"OS error: {e}")
return None
file_path = "example.txt"
file_size = get_file_size(file_path)
if file_size is not None:
print(f"The size of {file_path} is {file_size} bytes.")
else:
print("Could not determine the file size.")
在这个示例中,我们使用logging模块记录日志信息,包括文件大小和错误信息。通过设置日志级别和格式,我们可以灵活地控制日志输出。
九、总结
判定文件大小是一个常见的任务,Python提供了多种方法来实现这一功能。os模块、Pathlib模块和shutil模块都是常用的工具,每种方法都有其优势和适用场景。在实际应用中,我们可以根据需求选择合适的方法,并结合多种方法以提高代码的健壮性和兼容性。
此外,处理特殊文件类型、跨平台兼容性、性能优化和错误处理都是需要考虑的重要因素。通过合理地设计和优化代码,我们可以高效、准确地获取文件大小,并确保程序的稳定性和可维护性。
最后,日志记录是保证代码健壮性的重要手段。使用Python的logging模块可以方便地记录和管理日志信息,帮助我们在开发和调试过程中快速定位和解决问题。希望本文对您理解和掌握Python判定文件大小的方法有所帮助。
相关问答FAQs:
如何在Python中获取文件的大小?
在Python中,可以使用内置的os
模块来获取文件的大小。具体方法是使用os.path.getsize()
函数,传入文件的路径,返回值是文件的字节数。例如:
import os
file_size = os.path.getsize('your_file.txt')
print(f'文件大小为: {file_size} 字节')
使用Python检查文件是否为空?
要判断文件是否为空,可以在获取文件大小之后进行判断。如果文件大小为0,则说明文件为空。使用os.path.getsize()
函数可以轻松实现这一点。例如:
if os.path.getsize('your_file.txt') == 0:
print('文件为空')
else:
print('文件不为空')
Python中如何处理大文件的大小?
在处理大文件时,可以使用os.path.getsize()
同样获取文件大小,但为了避免内存不足的问题,建议使用文件流读取的方式来分块处理文件内容。例如,可以逐行读取文件,以此来有效管理内存并处理大文件。使用with open()
语句可以确保文件在操作后正确关闭。
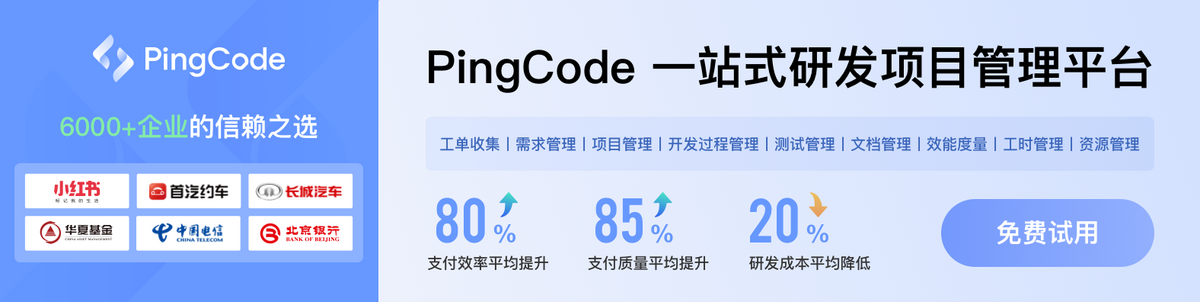