要用Python模拟超市购物,可以通过创建一个包含商品、库存和价格的虚拟超市系统,并且允许用户选择商品并生成购物清单。主要步骤包括:创建商品列表和库存、设计购物流程、计算总价、以及输出购物清单。其中,创建商品列表和库存是关键步骤,下面将详细描述。
一、创建商品列表和库存
首先,我们需要创建一个包含商品、库存和价格的商品列表。这可以通过Python中的字典或其他数据结构来实现。以下是一个简单的例子:
# 商品列表和库存
products = {
'apple': {'price': 0.5, 'stock': 50},
'banana': {'price': 0.3, 'stock': 100},
'milk': {'price': 1.2, 'stock': 30},
'bread': {'price': 1.0, 'stock': 20}
}
在这个例子中,我们用一个字典来存储商品信息,每个商品又是一个包含价格和库存的字典。
二、设计购物流程
接下来,我们需要设计一个购物流程,允许用户选择商品并输入购买数量。我们可以使用一个循环来不断询问用户的选择,直到用户决定结束购物。
def display_products(products):
print("Available products:")
for product, info in products.items():
print(f"{product}: ${info['price']} (Stock: {info['stock']})")
def get_user_choice():
choice = input("Enter the product name (or 'done' to finish): ").strip().lower()
return choice
def get_user_quantity():
quantity = int(input("Enter the quantity: "))
return quantity
shopping_cart = {}
while True:
display_products(products)
choice = get_user_choice()
if choice == 'done':
break
if choice in products:
quantity = get_user_quantity()
if quantity <= products[choice]['stock']:
if choice in shopping_cart:
shopping_cart[choice] += quantity
else:
shopping_cart[choice] = quantity
products[choice]['stock'] -= quantity
else:
print("Sorry, we do not have enough stock.")
else:
print("Product not found.")
三、计算总价
在用户完成购物后,我们需要计算购物车中的商品总价。我们可以遍历购物车并计算每种商品的总价格。
def calculate_total(cart, products):
total = 0
for product, quantity in cart.items():
total += products[product]['price'] * quantity
return total
total_price = calculate_total(shopping_cart, products)
print(f"Total price: ${total_price:.2f}")
四、输出购物清单
最后,我们需要输出购物清单,显示用户购买的所有商品及其数量和单价。
def display_cart(cart, products):
print("Your shopping cart:")
for product, quantity in cart.items():
price = products[product]['price']
print(f"{product}: {quantity} @ ${price} each")
display_cart(shopping_cart, products)
print(f"Total price: ${total_price:.2f}")
五、整合所有部分
将所有部分整合在一起,形成一个完整的超市购物模拟系统。
def display_products(products):
print("Available products:")
for product, info in products.items():
print(f"{product}: ${info['price']} (Stock: {info['stock']})")
def get_user_choice():
choice = input("Enter the product name (or 'done' to finish): ").strip().lower()
return choice
def get_user_quantity():
quantity = int(input("Enter the quantity: "))
return quantity
def calculate_total(cart, products):
total = 0
for product, quantity in cart.items():
total += products[product]['price'] * quantity
return total
def display_cart(cart, products):
print("Your shopping cart:")
for product, quantity in cart.items():
price = products[product]['price']
print(f"{product}: {quantity} @ ${price} each")
商品列表和库存
products = {
'apple': {'price': 0.5, 'stock': 50},
'banana': {'price': 0.3, 'stock': 100},
'milk': {'price': 1.2, 'stock': 30},
'bread': {'price': 1.0, 'stock': 20}
}
shopping_cart = {}
while True:
display_products(products)
choice = get_user_choice()
if choice == 'done':
break
if choice in products:
quantity = get_user_quantity()
if quantity <= products[choice]['stock']:
if choice in shopping_cart:
shopping_cart[choice] += quantity
else:
shopping_cart[choice] = quantity
products[choice]['stock'] -= quantity
else:
print("Sorry, we do not have enough stock.")
else:
print("Product not found.")
total_price = calculate_total(shopping_cart, products)
display_cart(shopping_cart, products)
print(f"Total price: ${total_price:.2f}")
这个代码实现了一个简单的超市购物模拟系统,包括商品列表和库存管理、用户购物流程、总价计算和购物清单输出。通过这种方式,我们可以在Python中模拟超市购物的整个过程。
六、进一步改进
- 用户输入错误处理:增加对用户输入错误的处理,比如输入无效的商品名称或数量。
- 持久化数据:可以使用文件或数据库来保存商品列表和购物车,使得数据在程序结束后仍然保留。
- 图形用户界面:可以使用Tkinter等库创建图形用户界面,使得程序更加友好和易用。
用户输入错误处理
用户在输入商品名称或数量时可能会犯错,因此需要增加一些错误处理逻辑。例如,当用户输入的商品名称不存在时,程序应提示错误信息并重新请求输入。
def get_user_choice():
while True:
choice = input("Enter the product name (or 'done' to finish): ").strip().lower()
if choice in products or choice == 'done':
return choice
else:
print("Product not found. Please try again.")
def get_user_quantity():
while True:
try:
quantity = int(input("Enter the quantity: "))
if quantity > 0:
return quantity
else:
print("Quantity must be a positive integer. Please try again.")
except ValueError:
print("Invalid input. Please enter a valid quantity.")
持久化数据
为了使商品列表和购物车数据在程序结束后仍然保留,可以使用文件或数据库进行数据持久化。以下是使用JSON文件保存商品列表和购物车的例子:
import json
def load_products(filename):
with open(filename, 'r') as file:
return json.load(file)
def save_products(products, filename):
with open(filename, 'w') as file:
json.dump(products, file)
def load_cart(filename):
try:
with open(filename, 'r') as file:
return json.load(file)
except FileNotFoundError:
return {}
def save_cart(cart, filename):
with open(filename, 'w') as file:
json.dump(cart, file)
加载商品列表和购物车
products = load_products('products.json')
shopping_cart = load_cart('cart.json')
购物流程
while True:
display_products(products)
choice = get_user_choice()
if choice == 'done':
break
if choice in products:
quantity = get_user_quantity()
if quantity <= products[choice]['stock']:
if choice in shopping_cart:
shopping_cart[choice] += quantity
else:
shopping_cart[choice] = quantity
products[choice]['stock'] -= quantity
else:
print("Sorry, we do not have enough stock.")
else:
print("Product not found.")
total_price = calculate_total(shopping_cart, products)
display_cart(shopping_cart, products)
print(f"Total price: ${total_price:.2f}")
保存商品列表和购物车
save_products(products, 'products.json')
save_cart(shopping_cart, 'cart.json')
图形用户界面
使用Tkinter库创建一个简单的图形用户界面,可以使程序更加友好和易用。以下是一个基本的Tkinter界面例子:
import tkinter as tk
from tkinter import messagebox
加载商品列表和购物车
products = load_products('products.json')
shopping_cart = load_cart('cart.json')
def update_product_listbox():
product_listbox.delete(0, tk.END)
for product, info in products.items():
product_listbox.insert(tk.END, f"{product}: ${info['price']} (Stock: {info['stock']})")
def add_to_cart():
selected_product = product_listbox.get(tk.ACTIVE).split(':')[0]
quantity = int(quantity_entry.get())
if quantity <= products[selected_product]['stock']:
if selected_product in shopping_cart:
shopping_cart[selected_product] += quantity
else:
shopping_cart[selected_product] = quantity
products[selected_product]['stock'] -= quantity
update_product_listbox()
messagebox.showinfo("Success", f"Added {quantity} {selected_product}(s) to cart.")
else:
messagebox.showerror("Error", "Not enough stock available.")
def checkout():
total_price = calculate_total(shopping_cart, products)
display_cart(shopping_cart, products)
messagebox.showinfo("Total Price", f"Total price: ${total_price:.2f}")
save_products(products, 'products.json')
save_cart(shopping_cart, 'cart.json')
创建Tkinter窗口
root = tk.Tk()
root.title("Supermarket Shopping")
产品列表
product_listbox = tk.Listbox(root, width=50)
product_listbox.pack()
数量输入框
quantity_label = tk.Label(root, text="Quantity:")
quantity_label.pack()
quantity_entry = tk.Entry(root)
quantity_entry.pack()
添加到购物车按钮
add_button = tk.Button(root, text="Add to Cart", command=add_to_cart)
add_button.pack()
结账按钮
checkout_button = tk.Button(root, text="Checkout", command=checkout)
checkout_button.pack()
更新产品列表
update_product_listbox()
运行Tkinter主循环
root.mainloop()
以上代码使用Tkinter库创建了一个简单的图形用户界面,用户可以通过选择商品、输入数量并点击按钮将商品添加到购物车,最后点击“Checkout”按钮进行结账。这个程序可以进一步扩展和美化,例如增加删除购物车商品的功能、显示购物车内容等。
通过这种方式,我们可以用Python创建一个功能齐全的超市购物模拟系统,包括商品管理、用户购物流程、数据持久化和图形用户界面。这个系统不仅可以用于学习和练习编程,还可以作为一个基础框架进行扩展和应用于更复杂的项目中。
相关问答FAQs:
在使用Python模拟超市购物时,需要哪些基本模块或库?
在进行超市购物模拟时,可以使用Python的内置模块,例如random
用于生成随机数,帮助模拟商品的随机选择和价格。此外,datetime
模块可以用来记录购物时间。为了更复杂的模拟,可以考虑使用pandas
库来管理商品数据和购物车,matplotlib
库用于可视化购物数据。
如何设计一个简单的超市购物系统的类结构?
设计一个超市购物系统时,可以创建多个类,比如Product
类用于定义商品属性(如名称、价格、库存),ShoppingCart
类用于管理用户的购物车(添加、删除商品,计算总价),以及Checkout
类来处理结账过程。这样的结构可以帮助代码模块化,便于维护和扩展。
在模拟超市购物时,如何处理用户输入和异常?
用户输入和异常处理是模拟超市购物的重要部分。可以通过try...except
结构来捕捉用户输入中的错误,例如输入无效商品ID或超出库存的数量。同时,使用循环结构提示用户重新输入有效信息,确保程序的健壮性和用户体验的流畅性。这样能够有效避免因输入错误导致的程序崩溃。
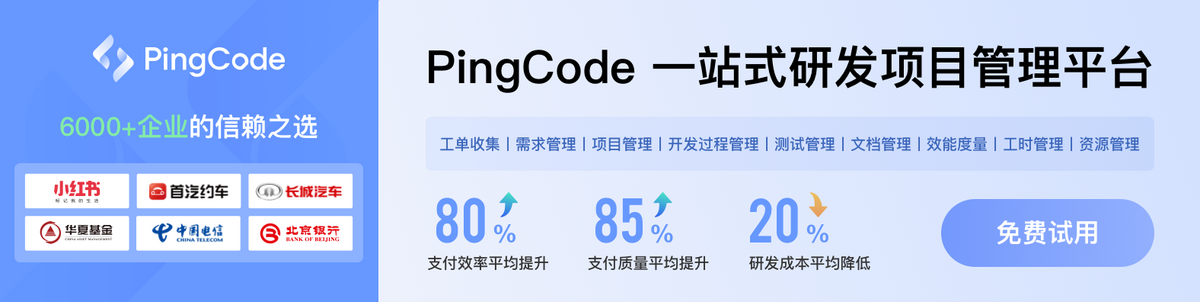