要将 Python 输出保存到文件,你可以使用内置的 open
函数和文件对象的 write
方法。使用 open
函数、使用文件对象的 write
方法、使用上下文管理器(with 语句) 是实现这一目的的常见方法。以下是详细描述其中一种方法:
使用上下文管理器(with 语句):这是推荐的方式,因为它可以确保文件在使用完后被正确关闭,即使在处理过程中发生了错误。上下文管理器能自动管理资源的分配和释放,从而提高代码的健壮性和可读性。
with open('output.txt', 'w') as file:
file.write('Hello, world!\n')
file.write('This is a test.\n')
在这个例子中,open
函数以写模式('w')打开名为 output.txt
的文件,如果该文件不存在,它将被创建。如果文件存在,其内容将被覆盖。file.write
方法将字符串写入文件中。以下是更详细的内容介绍。
一、使用 open 函数
open
函数是 Python 中用于打开文件的内置函数。其基本语法为:
file_object = open(file_name, mode)
其中,file_name
是文件的名称或路径,mode
是文件的打开模式。常见的模式有:
'r'
:只读模式(默认)'w'
:写入模式(会覆盖文件)'a'
:追加模式(在文件末尾添加内容)'b'
:二进制模式(与其他模式结合使用,如'rb'
或'wb'
)
例如,以下代码打开一个文件进行写入:
file = open('output.txt', 'w')
二、使用文件对象的 write 方法
一旦文件被打开,你可以使用文件对象的 write
方法将数据写入文件。例如:
file.write('Hello, world!\n')
file.write('This is a test.\n')
上述代码将字符串写入文件,每个字符串末尾的 \n
表示换行符。
三、使用上下文管理器(with 语句)
使用上下文管理器(with
语句)是管理文件的最佳实践。这不仅简化了代码,还确保文件在操作完成后被正确关闭。以下是示例代码:
with open('output.txt', 'w') as file:
file.write('Hello, world!\n')
file.write('This is a test.\n')
在 with
语句块中,file
是文件对象。离开 with
语句块时,文件会自动关闭。
四、写入多行数据
如果你有多行数据要写入文件,可以使用一个循环。例如:
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('output.txt', 'w') as file:
for line in lines:
file.write(line)
或者,使用 writelines
方法:
lines = ['First line\n', 'Second line\n', 'Third line\n']
with open('output.txt', 'w') as file:
file.writelines(lines)
五、写入二进制数据
对于二进制数据,你需要以二进制模式打开文件。例如:
with open('output.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
在此示例中,b'\x00\x01\x02\x03'
是一个字节串(bytes
对象)。
六、读取文件并写入新文件
你也可以将一个文件的内容读取并写入到另一个文件中。例如:
with open('input.txt', 'r') as infile, open('output.txt', 'w') as outfile:
for line in infile:
outfile.write(line)
在这个示例中,input.txt
中的每一行被读取并写入到 output.txt
中。
七、追加模式
如果你想在文件末尾添加内容而不是覆盖现有内容,可以使用追加模式 'a'
:
with open('output.txt', 'a') as file:
file.write('Another line\n')
八、错误处理
在处理文件时,可能会发生各种错误(如文件不存在、权限不足等)。你可以使用 try...except
语句来处理这些错误:
try:
with open('output.txt', 'w') as file:
file.write('Hello, world!\n')
except IOError as e:
print(f"An IOError occurred: {e}")
九、使用日志记录
在实际应用中,你可能希望将程序的输出记录到日志文件中。Python 的 logging
模块提供了强大的日志记录功能。例如:
import logging
logging.basicConfig(filename='app.log', level=logging.INFO)
logging.info('This is an info message')
在此示例中,日志消息将被写入 app.log
文件。
十、使用标准输出重定向
有时你可能想将标准输出重定向到文件。例如:
import sys
with open('output.txt', 'w') as file:
sys.stdout = file
print('This will be written to the file')
在此示例中,print
函数的输出将被重定向到 output.txt
文件。
十一、读取和写入 CSV 文件
对于结构化数据,如 CSV 文件,Python 提供了 csv
模块。例如:
import csv
写入 CSV 文件
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 30])
writer.writerow(['Bob', 25])
读取 CSV 文件
with open('output.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
十二、读取和写入 JSON 文件
对于 JSON 格式的数据,Python 提供了 json
模块。例如:
import json
data = {'name': 'Alice', 'age': 30}
写入 JSON 文件
with open('output.json', 'w') as file:
json.dump(data, file)
读取 JSON 文件
with open('output.json', 'r') as file:
data = json.load(file)
print(data)
十三、写入多种格式文件
有时候,你可能需要写入不同格式的文件,如 XML、YAML 等。Python 提供了相应的库:
- XML:使用
xml.etree.ElementTree
模块 - YAML:使用
PyYAML
库(需要安装pyyaml
)
例如,写入 XML 文件:
import xml.etree.ElementTree as ET
root = ET.Element("root")
child = ET.SubElement(root, "child")
child.text = "Hello, world!"
tree = ET.ElementTree(root)
tree.write("output.xml")
写入 YAML 文件:
import yaml
data = {'name': 'Alice', 'age': 30}
写入 YAML 文件
with open('output.yaml', 'w') as file:
yaml.dump(data, file)
读取 YAML 文件
with open('output.yaml', 'r') as file:
data = yaml.load(file, Loader=yaml.FullLoader)
print(data)
十四、使用 pandas 库写入文件
pandas
库是数据分析中常用的工具,它支持读写多种格式的文件,如 CSV、Excel、JSON 等。例如:
import pandas as pd
创建 DataFrame
df = pd.DataFrame({'Name': ['Alice', 'Bob'], 'Age': [30, 25]})
写入 CSV 文件
df.to_csv('output.csv', index=False)
写入 Excel 文件
df.to_excel('output.xlsx', index=False)
写入 JSON 文件
df.to_json('output.json')
十五、写入大文件
当处理大文件时,逐行写入是一种常见的方法,以避免内存不足。例如:
with open('large_output.txt', 'w') as file:
for i in range(1000000):
file.write(f'Line {i}\n')
十六、文件路径管理
在处理文件时,管理文件路径是一个重要的方面。Python 的 os
和 pathlib
模块提供了丰富的文件路径操作功能。例如:
import os
from pathlib import Path
使用 os 模块
file_path = os.path.join('directory', 'file.txt')
使用 pathlib 模块
file_path = Path('directory') / 'file.txt'
十七、文件权限管理
在某些情况下,你可能需要设置文件权限。Python 的 os
模块提供了相应的功能。例如:
import os
file_path = 'output.txt'
os.chmod(file_path, 0o644) # 设置文件权限为 rw-r--r--
十八、文件压缩和解压
Python 的 zipfile
和 tarfile
模块可以用来处理压缩文件。例如:
import zipfile
创建 ZIP 文件
with zipfile.ZipFile('output.zip', 'w') as zipf:
zipf.write('output.txt')
解压 ZIP 文件
with zipfile.ZipFile('output.zip', 'r') as zipf:
zipf.extractall('extracted')
十九、文件加密和解密
在某些情况下,你可能需要对文件进行加密和解密。Python 的 cryptography
库可以用来处理加密和解密。例如:
from cryptography.fernet import Fernet
生成密钥
key = Fernet.generate_key()
cipher = Fernet(key)
加密文件
with open('output.txt', 'rb') as file:
encrypted_data = cipher.encrypt(file.read())
with open('output.encrypted', 'wb') as file:
file.write(encrypted_data)
解密文件
with open('output.encrypted', 'rb') as file:
decrypted_data = cipher.decrypt(file.read())
with open('output_decrypted.txt', 'wb') as file:
file.write(decrypted_data)
二十、文件锁定
在处理多进程或多线程环境时,文件锁定可以防止竞争条件。Python 的 fcntl
模块(仅适用于 Unix 系统)和 portalocker
库可以用来实现文件锁定。例如:
import portalocker
with open('output.txt', 'w') as file:
portalocker.lock(file, portalocker.LOCK_EX)
file.write('Hello, world!\n')
portalocker.unlock(file)
综上所述,Python 提供了丰富的文件操作功能,从基本的文件读写到高级的文件加密、压缩、锁定等。掌握这些技巧可以帮助你在实际项目中更加高效地处理文件。
相关问答FAQs:
如何在Python中将数据写入文件?
在Python中,可以使用内置的open()
函数来创建或打开一个文件,并使用write()
或writelines()
方法将数据写入文件。以下是一个简单的示例:
with open('output.txt', 'w') as file:
file.write('Hello, World!\n')
file.writelines(['Line 1\n', 'Line 2\n'])
上述代码创建一个名为output.txt
的文件,并在其中写入两行文本。
可以将哪些类型的数据输出到文件?
Python支持多种数据类型的输出,包括字符串、数字、列表和字典等。对于非字符串类型的数据,您需要先将其转换为字符串,例如使用str()
函数。对于更复杂的数据结构,使用json
模块可以方便地将字典或列表等转换为JSON格式并写入文件。示例:
import json
data = {'name': 'Alice', 'age': 30}
with open('data.json', 'w') as file:
json.dump(data, file)
如何在写入文件时处理异常?
在文件操作中,处理异常非常重要,以避免程序崩溃。在Python中,可以使用try...except
语句来捕获可能发生的异常,并采取相应的措施。例如:
try:
with open('output.txt', 'w') as file:
file.write('Writing data to file.')
except IOError as e:
print(f'An error occurred: {e}')
这样可以确保如果在写入文件时发生错误,程序将正常处理并给出提示,而不是直接终止运行。
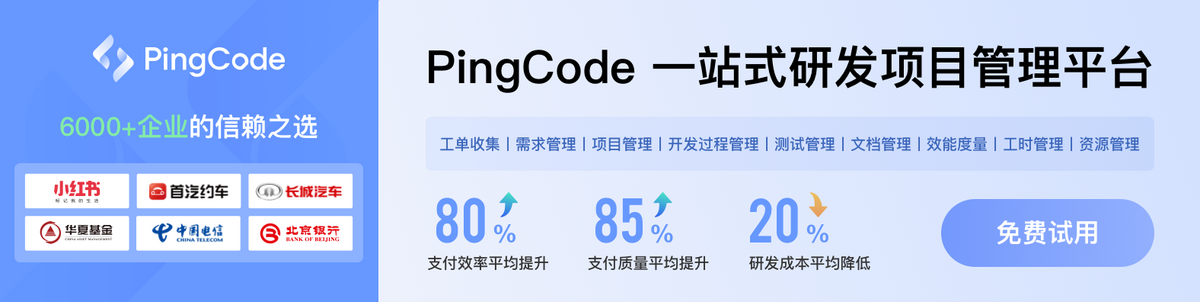