Python数据库备份的方法包括:使用数据库自带的备份工具、利用Python的数据库连接库执行备份命令、使用ORM框架提供的备份功能、编写自定义脚本。
其中,利用Python的数据库连接库执行备份命令是一种较为灵活且常用的方法。可以通过Python的数据库连接库(如sqlite3
、psycopg2
、pymysql
等)连接到数据库,然后执行数据库的备份命令。具体操作包括:连接数据库、执行备份语句、保存备份文件。以下将详细介绍这种方法。
一、使用数据库自带的备份工具
1、MySQL的备份工具
MySQL提供了mysqldump
工具,可以通过命令行备份数据库。可以在Python中使用subprocess
模块调用该工具进行备份。
import subprocess
def backup_mysql(db_name, user, password, output_file):
command = f"mysqldump -u {user} -p{password} {db_name} > {output_file}"
subprocess.run(command, shell=True)
backup_mysql('your_database', 'your_user', 'your_password', 'backup.sql')
2、PostgreSQL的备份工具
PostgreSQL提供了pg_dump
工具,同样可以通过命令行备份数据库。可以在Python中使用subprocess
模块调用该工具进行备份。
import subprocess
def backup_postgresql(db_name, user, output_file):
command = f"pg_dump -U {user} {db_name} > {output_file}"
subprocess.run(command, shell=True)
backup_postgresql('your_database', 'your_user', 'backup.sql')
3、SQLite的备份工具
SQLite的备份相对简单,因为它本身就是一个单一的文件。可以直接复制文件进行备份。
import shutil
def backup_sqlite(db_file, backup_file):
shutil.copyfile(db_file, backup_file)
backup_sqlite('your_database.db', 'backup.db')
二、利用Python的数据库连接库执行备份命令
1、连接数据库
首先需要安装相应的数据库连接库,例如sqlite3
、psycopg2
、pymysql
等。
import sqlite3
import psycopg2
import pymysql
2、执行备份命令
1、SQLite的备份
import sqlite3
def backup_sqlite(db_file, backup_file):
conn = sqlite3.connect(db_file)
with open(backup_file, 'w') as f:
for line in conn.iterdump():
f.write('%s\n' % line)
conn.close()
backup_sqlite('your_database.db', 'backup.sql')
2、MySQL的备份
import pymysql
def backup_mysql(db_name, user, password, host, output_file):
conn = pymysql.connect(host=host, user=user, password=password, db=db_name)
cursor = conn.cursor()
with open(output_file, 'w') as f:
for line in cursor.execute("SHOW TABLES"):
f.write('%s\n' % line)
conn.close()
backup_mysql('your_database', 'your_user', 'your_password', 'localhost', 'backup.sql')
3、PostgreSQL的备份
import psycopg2
def backup_postgresql(db_name, user, password, host, output_file):
conn = psycopg2.connect(database=db_name, user=user, password=password, host=host)
cursor = conn.cursor()
with open(output_file, 'w') as f:
for line in cursor.execute("COPY (SELECT * FROM tablename) TO STDOUT WITH CSV HEADER"):
f.write('%s\n' % line)
conn.close()
backup_postgresql('your_database', 'your_user', 'your_password', 'localhost', 'backup.csv')
三、使用ORM框架提供的备份功能
1、Django ORM的备份
Django提供了dumpdata
命令,可以方便地导出数据库内容。
import os
def backup_django(output_file):
command = f"python manage.py dumpdata > {output_file}"
os.system(command)
backup_django('backup.json')
2、SQLAlchemy ORM的备份
SQLAlchemy没有直接的备份命令,但可以通过查询数据库并导出数据来实现备份。
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
def backup_sqlalchemy(connection_string, output_file):
engine = create_engine(connection_string)
Session = sessionmaker(bind=engine)
session = Session()
with open(output_file, 'w') as f:
for table in engine.table_names():
for row in session.execute(f"SELECT * FROM {table}"):
f.write('%s\n' % row)
session.close()
backup_sqlalchemy('sqlite:///your_database.db', 'backup.sql')
四、编写自定义脚本
1、自定义SQLite备份脚本
import sqlite3
def custom_backup_sqlite(db_file, backup_file):
conn = sqlite3.connect(db_file)
cursor = conn.cursor()
with open(backup_file, 'w') as f:
for line in cursor.execute("SELECT * FROM sqlite_master"):
f.write('%s\n' % line)
for line in cursor.execute("SELECT * FROM sqlite_sequence"):
f.write('%s\n' % line)
conn.close()
custom_backup_sqlite('your_database.db', 'custom_backup.sql')
2、自定义MySQL备份脚本
import pymysql
def custom_backup_mysql(db_name, user, password, host, output_file):
conn = pymysql.connect(host=host, user=user, password=password, db=db_name)
cursor = conn.cursor()
with open(output_file, 'w') as f:
for table in cursor.execute("SHOW TABLES"):
f.write('%s\n' % table)
for row in cursor.execute(f"SELECT * FROM {table}"):
f.write('%s\n' % row)
conn.close()
custom_backup_mysql('your_database', 'your_user', 'your_password', 'localhost', 'custom_backup.sql')
3、自定义PostgreSQL备份脚本
import psycopg2
def custom_backup_postgresql(db_name, user, password, host, output_file):
conn = psycopg2.connect(database=db_name, user=user, password=password, host=host)
cursor = conn.cursor()
with open(output_file, 'w') as f:
for table in cursor.execute("SELECT table_name FROM information_schema.tables WHERE table_schema='public'"):
f.write('%s\n' % table)
for row in cursor.execute(f"SELECT * FROM {table}"):
f.write('%s\n' % row)
conn.close()
custom_backup_postgresql('your_database', 'your_user', 'your_password', 'localhost', 'custom_backup.sql')
五、备份的自动化和调度
1、使用cron定时任务
可以使用cron定时任务来自动化备份过程。例如,每天晚上12点备份数据库。
在Linux系统中,可以编辑cron任务:
crontab -e
添加以下内容:
0 0 * * * /usr/bin/python3 /path/to/your_backup_script.py
2、使用Windows任务计划
在Windows系统中,可以使用任务计划程序来自动化备份过程。例如,每天晚上12点备份数据库。
- 打开任务计划程序。
- 创建基本任务。
- 设置触发器为每日。
- 设置操作为启动程序,选择Python解释器和备份脚本。
3、使用Python脚本调度
可以使用Python的调度库,如schedule
,来实现定时备份。
import schedule
import time
def backup():
# 调用备份函数
backup_sqlite('your_database.db', 'backup.sql')
schedule.every().day.at("00:00").do(backup)
while True:
schedule.run_pending()
time.sleep(1)
六、备份文件的管理和存储
1、备份文件的命名
为了便于管理和查找备份文件,可以在备份文件名中添加时间戳。
import datetime
timestamp = datetime.datetime.now().strftime('%Y%m%d%H%M%S')
output_file = f"backup_{timestamp}.sql"
backup_sqlite('your_database.db', output_file)
2、备份文件的存储位置
可以将备份文件存储在本地磁盘、外部存储设备、云存储等位置。
1、本地磁盘
将备份文件存储在本地磁盘的指定目录。
backup_dir = '/path/to/backup/dir'
output_file = f"{backup_dir}/backup_{timestamp}.sql"
backup_sqlite('your_database.db', output_file)
2、外部存储设备
将备份文件复制到外部存储设备,如U盘、移动硬盘等。
import shutil
external_storage = '/path/to/external/storage'
shutil.copyfile(output_file, f"{external_storage}/backup_{timestamp}.sql")
3、云存储
将备份文件上传到云存储服务,如Amazon S3、Google Cloud Storage等。
import boto3
def upload_to_s3(file_name, bucket, object_name=None):
s3_client = boto3.client('s3')
if object_name is None:
object_name = file_name
s3_client.upload_file(file_name, bucket, object_name)
upload_to_s3(output_file, 'your_bucket_name')
七、备份文件的恢复
1、恢复SQLite数据库
import sqlite3
def restore_sqlite(backup_file, db_file):
conn = sqlite3.connect(db_file)
with open(backup_file, 'r') as f:
sql = f.read()
conn.executescript(sql)
conn.close()
restore_sqlite('backup.sql', 'your_database.db')
2、恢复MySQL数据库
import pymysql
def restore_mysql(backup_file, db_name, user, password, host):
conn = pymysql.connect(host=host, user=user, password=password, db=db_name)
cursor = conn.cursor()
with open(backup_file, 'r') as f:
sql = f.read()
cursor.execute(sql)
conn.close()
restore_mysql('backup.sql', 'your_database', 'your_user', 'your_password', 'localhost')
3、恢复PostgreSQL数据库
import psycopg2
def restore_postgresql(backup_file, db_name, user, password, host):
conn = psycopg2.connect(database=db_name, user=user, password=password, host=host)
cursor = conn.cursor()
with open(backup_file, 'r') as f:
sql = f.read()
cursor.execute(sql)
conn.close()
restore_postgresql('backup.sql', 'your_database', 'your_user', 'your_password', 'localhost')
八、备份文件的安全性
1、加密备份文件
为了保护备份文件的安全,可以对备份文件进行加密。
from cryptography.fernet import Fernet
def encrypt_file(file_name, key):
with open(file_name, 'rb') as f:
data = f.read()
fernet = Fernet(key)
encrypted_data = fernet.encrypt(data)
with open(file_name, 'wb') as f:
f.write(encrypted_data)
def decrypt_file(file_name, key):
with open(file_name, 'rb') as f:
encrypted_data = f.read()
fernet = Fernet(key)
data = fernet.decrypt(encrypted_data)
with open(file_name, 'wb') as f:
f.write(data)
key = Fernet.generate_key()
encrypt_file('backup.sql', key)
decrypt_file('backup.sql', key)
2、备份文件的访问控制
限制备份文件的访问权限,确保只有授权人员能够访问和操作备份文件。
chmod 600 backup.sql
九、备份策略的制定
1、完全备份
完全备份是对整个数据库进行完整备份,适用于数据量较小的情况。
backup_sqlite('your_database.db', 'full_backup.sql')
2、增量备份
增量备份只备份自上次完全备份或增量备份以来发生变化的数据,适用于数据量较大的情况。
import os
import sqlite3
def incremental_backup(db_file, backup_file, last_backup_time):
conn = sqlite3.connect(db_file)
cursor = conn.cursor()
with open(backup_file, 'w') as f:
for row in cursor.execute("SELECT * FROM your_table WHERE last_modified > ?", (last_backup_time,)):
f.write('%s\n' % row)
conn.close()
last_backup_time = os.path.getmtime('last_backup.sql')
incremental_backup('your_database.db', 'incremental_backup.sql', last_backup_time)
3、差异备份
差异备份是对自上次完全备份以来发生变化的数据进行备份,适用于数据量较大的情况。
import os
import sqlite3
def differential_backup(db_file, backup_file, last_full_backup_time):
conn = sqlite3.connect(db_file)
cursor = conn.cursor()
with open(backup_file, 'w') as f:
for row in cursor.execute("SELECT * FROM your_table WHERE last_modified > ?", (last_full_backup_time,)):
f.write('%s\n' % row)
conn.close()
last_full_backup_time = os.path.getmtime('full_backup.sql')
differential_backup('your_database.db', 'differential_backup.sql', last_full_backup_time)
十、备份的验证和测试
1、备份文件的完整性验证
确保备份文件在传输和存储过程中未被篡改,可以使用哈希校验。
import hashlib
def calculate_hash(file_name):
hash_md5 = hashlib.md5()
with open(file_name, 'rb') as f:
for chunk in iter(lambda: f.read(4096), b""):
hash_md5.update(chunk)
return hash_md5.hexdigest()
original_hash = calculate_hash('backup.sql')
传输或存储后
new_hash = calculate_hash('backup.sql')
assert original_hash == new_hash, "Backup file has been modified"
2、备份恢复的测试
定期测试备份文件的恢复过程,确保在需要时能够正确恢复数据库。
def test_restore():
restore_sqlite('backup.sql', 'test_database.db')
# 验证恢复后的数据
conn = sqlite3.connect('test_database.db')
cursor = conn.cursor()
cursor.execute("SELECT COUNT(*) FROM your_table")
assert cursor.fetchone()[0] == expected_row_count, "Restore failed"
conn.close()
test_restore()
总结
备份是数据库管理中至关重要的环节,通过使用数据库自带的备份工具、利用Python的数据库连接库执行备份命令、使用ORM框架提供的备份功能、编写自定义脚本等方法,可以实现数据库的备份和恢复。为了确保备份的安全性和可靠性,建议制定合理的备份策略,定期进行备份文件的验证和测试,并采取必要的安全措施。
相关问答FAQs:
如何选择合适的数据库备份工具?
选择合适的数据库备份工具取决于多个因素,包括你的数据库类型、备份频率和数据量。对于Python用户,常见的备份工具包括pg_dump
(用于PostgreSQL)、mysqldump
(用于MySQL)等。确保备份工具支持你的数据库版本,并考虑是否需要自动化备份过程以及易用性。
Python中如何实现数据库的定时备份?
实现数据库的定时备份可以使用cron
任务或Windows任务调度程序来定期运行备份脚本。通过Python编写的脚本可以调用数据库的备份命令并将备份文件存储在指定位置。结合Python的schedule
库,也能实现更灵活的定时任务。
在备份数据库时,如何确保数据的完整性和安全性?
为了确保数据的完整性和安全性,备份过程中应考虑使用事务性备份,确保在备份完成后数据的一致性。此外,定期检查备份文件的完整性,确保能够顺利恢复。同时,可以考虑将备份文件加密并存储在安全的位置,以防止数据泄露。
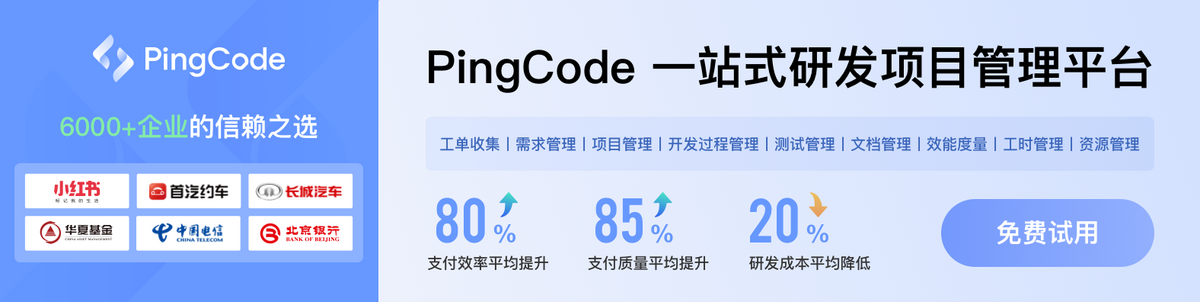