如何用python找女朋友这个问题乍一听有些荒谬,但其实我们可以通过Python编程来辅助我们的社交活动。核心观点:数据分析、机器学习、社交网络分析、自动化脚本。其中,数据分析是一个非常重要的方面。通过数据分析,我们可以了解目标人群的兴趣爱好、社交行为模式,从而制定更有针对性的社交策略。
一、数据分析
数据分析是利用Python处理和分析数据,以获取有用的洞察。通过数据分析,我们可以了解目标人群的特点,优化我们的社交策略,增加找到合适伴侣的概率。
1、收集数据
首先,需要收集目标人群的数据。可以通过社交媒体、交友网站等公开数据源获取。使用Python的爬虫库如BeautifulSoup、Scrapy等,可以方便地从网页上提取信息。例如,可以获取某个交友网站上用户的基本信息、兴趣爱好等。
from bs4 import BeautifulSoup
import requests
获取网页内容
url = 'http://example.com/dating_site'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
提取用户信息
profiles = soup.find_all('div', class_='profile')
for profile in profiles:
name = profile.find('h2').text
age = profile.find('span', class_='age').text
interests = profile.find('p', class_='interests').text
print(f'Name: {name}, Age: {age}, Interests: {interests}')
2、数据清洗
收集到的数据往往是杂乱无章的,需要进行清洗。数据清洗包括去除重复数据、处理缺失值、标准化数据格式等。
import pandas as pd
加载数据
data = pd.read_csv('profiles.csv')
去除重复数据
data.drop_duplicates(inplace=True)
处理缺失值
data.fillna(method='ffill', inplace=True)
标准化数据格式
data['age'] = data['age'].astype(int)
data['interests'] = data['interests'].str.lower()
3、数据分析
使用Python的分析库如Pandas、NumPy、Matplotlib等,可以对数据进行统计分析和可视化。通过分析,可以了解目标人群的分布、兴趣爱好等,从而制定更有针对性的策略。
import matplotlib.pyplot as plt
统计年龄分布
age_distribution = data['age'].value_counts()
age_distribution.plot(kind='bar')
plt.xlabel('Age')
plt.ylabel('Count')
plt.title('Age Distribution')
plt.show()
统计兴趣爱好
interests_distribution = data['interests'].value_counts()
interests_distribution.plot(kind='bar')
plt.xlabel('Interests')
plt.ylabel('Count')
plt.title('Interests Distribution')
plt.show()
二、机器学习
机器学习可以帮助我们从数据中挖掘更深层次的规律,预测潜在的匹配对象。通过训练模型,我们可以提高匹配的准确性,增加找到合适伴侣的概率。
1、特征选择
在进行机器学习之前,需要选择合适的特征。特征选择是指从原始数据中提取出对预测结果有重要影响的变量。在交友场景中,常用的特征包括年龄、兴趣爱好、地理位置等。
from sklearn.feature_selection import SelectKBest, chi2
提取特征
features = data[['age', 'interests', 'location']]
target = data['match']
选择最佳特征
selector = SelectKBest(score_func=chi2, k='all')
selector.fit(features, target)
scores = selector.scores_
打印特征得分
for feature, score in zip(features.columns, scores):
print(f'Feature: {feature}, Score: {score}')
2、模型训练
选择合适的模型进行训练。常用的模型包括决策树、随机森林、支持向量机等。在训练模型时,需要将数据分为训练集和测试集,以评估模型的性能。
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
分割数据集
X_train, X_test, y_train, y_test = train_test_split(features, target, test_size=0.2, random_state=42)
训练模型
model = RandomForestClassifier()
model.fit(X_train, y_train)
预测结果
y_pred = model.predict(X_test)
评估模型
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
3、模型优化
通过调整模型参数,可以进一步提高模型的性能。常用的优化方法包括交叉验证、网格搜索等。
from sklearn.model_selection import GridSearchCV
定义参数网格
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [10, 20, 30]
}
网格搜索
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X_train, y_train)
最佳参数
best_params = grid_search.best_params_
print(f'Best Parameters: {best_params}')
重新训练模型
model = RandomForestClassifier(best_params)
model.fit(X_train, y_train)
三、社交网络分析
社交网络分析是通过分析社交网络中的节点和边,了解人际关系的结构和特征。在交友场景中,可以利用社交网络分析找到潜在的匹配对象,增加找到合适伴侣的概率。
1、构建社交网络
首先,需要构建社交网络。社交网络由节点(用户)和边(用户之间的关系)组成。可以通过收集用户之间的交互数据,构建社交网络。
import networkx as nx
构建社交网络
G = nx.Graph()
G.add_edges_from([
('Alice', 'Bob'),
('Bob', 'Charlie'),
('Charlie', 'David'),
('David', 'Alice')
])
绘制社交网络
nx.draw(G, with_labels=True)
plt.show()
2、社交网络分析
通过社交网络分析,可以了解社交网络的结构和特征。例如,可以计算节点的度、聚类系数、最短路径等指标。
# 计算节点的度
degrees = dict(G.degree())
print(f'Degrees: {degrees}')
计算聚类系数
clustering = nx.clustering(G)
print(f'Clustering Coefficient: {clustering}')
计算最短路径
shortest_path = nx.shortest_path(G, source='Alice', target='Charlie')
print(f'Shortest Path: {shortest_path}')
3、社区发现
社区发现是指在社交网络中找到密切联系的用户群体。在交友场景中,可以利用社区发现找到潜在的匹配对象。
# 社区发现
from networkx.algorithms import community
communities = community.greedy_modularity_communities(G)
for i, community in enumerate(communities):
print(f'Community {i+1}: {community}')
四、自动化脚本
自动化脚本可以帮助我们节省时间和精力,提高社交效率。通过编写Python脚本,可以自动化完成一些重复性任务,如发送消息、更新个人资料等。
1、自动发送消息
可以编写脚本自动向潜在匹配对象发送消息,增加互动机会。
import smtplib
from email.mime.text import MIMEText
定义邮件内容
msg = MIMEText('Hello! I found your profile interesting. Would you like to chat?')
msg['Subject'] = 'Hello!'
msg['From'] = 'your_email@example.com'
msg['To'] = 'target_email@example.com'
发送邮件
with smtplib.SMTP('smtp.example.com') as server:
server.login('your_email@example.com', 'your_password')
server.sendmail(msg['From'], [msg['To']], msg.as_string())
2、自动更新资料
可以编写脚本自动更新个人资料,保持资料的最新状态。
import requests
定义个人资料
profile = {
'name': 'John Doe',
'age': 30,
'interests': 'Reading, Traveling, Music'
}
更新个人资料
url = 'http://example.com/update_profile'
response = requests.post(url, data=profile)
if response.status_code == 200:
print('Profile updated successfully')
else:
print('Failed to update profile')
五、总结
通过数据分析、机器学习、社交网络分析和自动化脚本,我们可以利用Python编程辅助我们的社交活动,提高找到合适伴侣的概率。数据分析可以帮助我们了解目标人群的特点,制定更有针对性的社交策略;机器学习可以帮助我们从数据中挖掘规律,预测潜在的匹配对象;社交网络分析可以帮助我们了解人际关系的结构和特征,找到潜在的匹配对象;自动化脚本可以帮助我们节省时间和精力,提高社交效率。通过综合运用这些技术,我们可以更有效地找到合适的伴侣。
相关问答FAQs:
如何利用Python编程来提升社交技能?
Python可以用来开发一些社交应用程序,帮助你更好地与他人互动。通过创建聊天机器人、推荐系统或社交媒体分析工具,你可以在与他人交流时展现出你的编程能力和创造力。这不仅能吸引潜在的伴侣,还能提升你在社交场合中的自信心。
是否有Python库可以帮助我进行约会分析?
是的,有许多Python库可以用于数据分析和可视化,像Pandas和Matplotlib。你可以利用这些工具来分析自己的约会历史、评估成功与失败的因素,从而在未来的约会中做出更明智的选择。这种数据驱动的方法将帮助你更好地理解自己的社交模式。
如何通过编程项目增加与他人交流的机会?
参与开源项目或组织Python编程聚会可以成为结识新朋友和潜在伴侣的好机会。通过这些活动,你不仅可以展示自己的编程技能,还能与志同道合的人建立联系。这种社交方式既自然又有效,有助于拓宽你的人际关系网络。
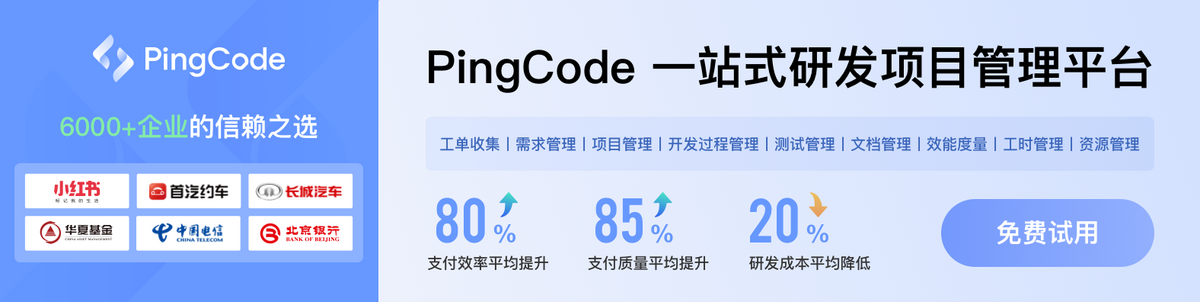