Python框架实现多协程的方法包括使用asyncio模块、使用concurrent.futures模块、使用gevent库等。
其中asyncio模块是Python内置库之一,它提供了对异步I/O、事件循环、协程以及任务等的支持,可以方便地实现多协程编程。使用asyncio模块,可以通过定义协程函数、创建事件循环、将任务添加到事件循环并运行等步骤来实现多协程执行。具体步骤如下:
- 定义协程函数:使用
async def
关键字定义一个协程函数。 - 创建事件循环:使用
asyncio.get_event_loop()
获取当前的事件循环。 - 将任务添加到事件循环:使用
loop.create_task()
将协程函数添加为任务。 - 运行事件循环:使用
loop.run_until_complete()
运行事件循环,直到所有任务完成。
例如,以下代码示例演示了如何使用asyncio模块实现多协程:
import asyncio
async def my_coroutine(name, delay):
await asyncio.sleep(delay)
print(f'Coroutine {name} executed after {delay} seconds')
async def main():
# 定义多个协程任务
tasks = [
asyncio.create_task(my_coroutine('A', 1)),
asyncio.create_task(my_coroutine('B', 2)),
asyncio.create_task(my_coroutine('C', 3))
]
# 等待所有任务完成
await asyncio.gather(*tasks)
获取事件循环并运行
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
通过上述步骤,可以实现多协程的并发执行。
以下将详细介绍Python框架实现多协程的不同方法。
一、asyncio模块
1、asyncio简介
Asyncio是Python 3.3版本引入的标准库,用于编写异步程序。它提供了事件循环、协程、任务等功能,使得在I/O密集型操作中,可以提高程序的并发性和性能。Asyncio的核心是事件循环(Event Loop),它是一个无限循环,用于调度协程。
2、定义协程
协程是Python中一种特殊的函数,它可以在运行过程中暂停,并在需要时恢复运行。定义协程函数需要使用async def
关键字。例如:
import asyncio
async def my_coroutine():
print('Hello, world!')
await asyncio.sleep(1)
print('Goodbye, world!')
3、创建事件循环
事件循环是Asyncio的核心,它负责调度协程。可以使用asyncio.get_event_loop()
获取当前事件循环。例如:
loop = asyncio.get_event_loop()
4、将任务添加到事件循环
可以使用loop.create_task()
将协程函数添加为任务。例如:
task = loop.create_task(my_coroutine())
5、运行事件循环
使用loop.run_until_complete()
运行事件循环,直到所有任务完成。例如:
loop.run_until_complete(task)
6、完整示例
结合上述步骤,可以编写一个完整的示例程序:
import asyncio
async def my_coroutine(name, delay):
await asyncio.sleep(delay)
print(f'Coroutine {name} executed after {delay} seconds')
async def main():
tasks = [
asyncio.create_task(my_coroutine('A', 1)),
asyncio.create_task(my_coroutine('B', 2)),
asyncio.create_task(my_coroutine('C', 3))
]
await asyncio.gather(*tasks)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
二、concurrent.futures模块
1、concurrent.futures简介
concurrent.futures
模块是Python 3.2版本引入的标准库,用于管理线程池和进程池。它提供了ThreadPoolExecutor
和ProcessPoolExecutor
两个类,用于分别管理线程和进程。
2、使用ThreadPoolExecutor
ThreadPoolExecutor
用于管理线程池,可以方便地执行多线程任务。例如:
from concurrent.futures import ThreadPoolExecutor
import time
def my_task(name, delay):
time.sleep(delay)
print(f'Task {name} executed after {delay} seconds')
with ThreadPoolExecutor(max_workers=3) as executor:
futures = [
executor.submit(my_task, 'A', 1),
executor.submit(my_task, 'B', 2),
executor.submit(my_task, 'C', 3)
]
for future in futures:
future.result()
3、使用ProcessPoolExecutor
ProcessPoolExecutor
用于管理进程池,可以方便地执行多进程任务。例如:
from concurrent.futures import ProcessPoolExecutor
import time
def my_task(name, delay):
time.sleep(delay)
print(f'Task {name} executed after {delay} seconds')
with ProcessPoolExecutor(max_workers=3) as executor:
futures = [
executor.submit(my_task, 'A', 1),
executor.submit(my_task, 'B', 2),
executor.submit(my_task, 'C', 3)
]
for future in futures:
future.result()
三、gevent库
1、gevent简介
Gevent是一个第三方库,用于提供高性能的异步I/O和协程。它基于协程和事件循环,使得在I/O密集型操作中,可以提高程序的并发性和性能。
2、安装gevent
可以使用pip安装gevent库:
pip install gevent
3、定义协程
在Gevent中,协程是通过gevent.spawn()
函数创建的。例如:
import gevent
from gevent import monkey
monkey.patch_all()
def my_coroutine(name, delay):
gevent.sleep(delay)
print(f'Coroutine {name} executed after {delay} seconds')
tasks = [
gevent.spawn(my_coroutine, 'A', 1),
gevent.spawn(my_coroutine, 'B', 2),
gevent.spawn(my_coroutine, 'C', 3)
]
gevent.joinall(tasks)
四、总结
Python框架实现多协程的方法主要包括使用asyncio模块、使用concurrent.futures模块、使用gevent库等。其中asyncio模块是Python内置库之一,提供了对异步I/O、事件循环、协程以及任务等的支持,可以方便地实现多协程编程。通过定义协程函数、创建事件循环、将任务添加到事件循环并运行等步骤,可以实现多协程的并发执行。
相关问答FAQs:
在Python中,如何有效地实现多协程?
要有效地实现多协程,您可以使用asyncio
库,这是Python的标准库之一,专为异步编程而设计。通过async
和await
关键字,您可以定义异步函数,并在需要时并发地运行多个协程。利用事件循环,您可以调度和管理多个协程的执行,确保高效利用系统资源。
使用Python框架时,如何处理协程中的异常?
在协程中处理异常可以通过try
和except
块来实现。确保在每个协程的关键部分使用异常处理,以捕获可能发生的错误。这有助于避免整个程序因单个协程的错误而崩溃。此外,可以考虑使用asyncio.gather()
来同时运行多个协程,并捕获所有协程的异常,以便进行集中处理。
Python协程在网络编程中有哪些优势?
Python协程在网络编程中提供了显著的优势,尤其是在处理大量并发连接时。由于协程是轻量级的,它们比线程更节省资源,使得在高并发场景下,您可以同时处理更多的请求。此外,协程的非阻塞特性意味着在等待I/O操作时,CPU可以处理其他任务,从而提高了整体性能和响应速度。
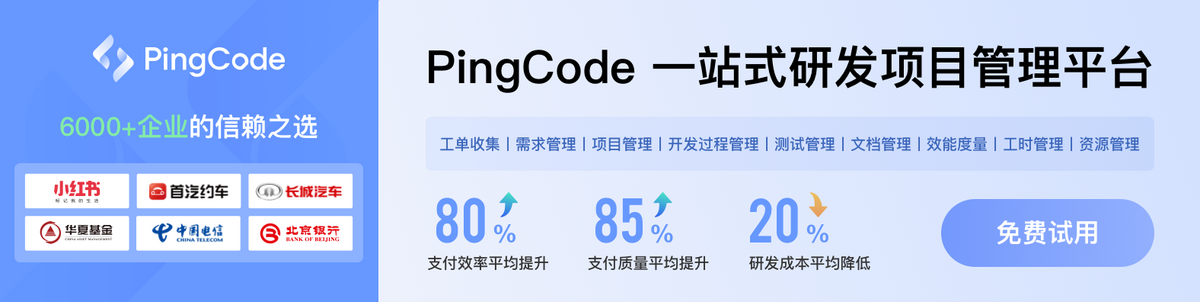