在Python中,我们可以通过多个库来实现按钮操作Excel文件的功能。常用的库包括openpyxl
、pandas
、tkinter
等。其中,tkinter
用于创建图形用户界面(GUI),而openpyxl
和pandas
则用于处理Excel文件。以下是一个详细的描述:
要创建一个按钮操作Excel文件的应用程序,首先要搭建一个用户界面,然后编写处理Excel文件的逻辑。我们可以通过tkinter
来创建按钮,通过openpyxl
来读取和写入Excel文件。
一、创建图形用户界面(GUI)
tkinter
是Python内置的GUI库,可以用来创建按钮、文本框等控件。
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename(filetypes=[("Excel files", "*.xlsx *.xls")])
if file_path:
# 在此处添加处理文件的代码
print(f"打开的文件路径: {file_path}")
root = tk.Tk()
root.title("Excel 操作")
open_button = tk.Button(root, text="打开Excel文件", command=open_file)
open_button.pack(pady=20)
root.mainloop()
二、读取Excel文件
使用openpyxl
库可以方便地读取和写入Excel文件。
import openpyxl
def read_excel(file_path):
workbook = openpyxl.load_workbook(file_path)
sheet = workbook.active
data = []
for row in sheet.iter_rows(values_only=True):
data.append(row)
return data
三、写入Excel文件
同样使用openpyxl
库可以将数据写入到Excel文件中。
def write_excel(file_path, data):
workbook = openpyxl.Workbook()
sheet = workbook.active
for row in data:
sheet.append(row)
workbook.save(file_path)
四、综合示例
我们将以上的代码综合起来,创建一个完整的应用程序,用户可以通过按钮来选择文件,然后读取文件内容并打印出来,最后将修改后的内容写入到新的Excel文件中。
import tkinter as tk
from tkinter import filedialog
import openpyxl
def open_file():
file_path = filedialog.askopenfilename(filetypes=[("Excel files", "*.xlsx *.xls")])
if file_path:
data = read_excel(file_path)
print(f"读取的数据: {data}")
new_data = process_data(data)
save_file(new_data)
def read_excel(file_path):
workbook = openpyxl.load_workbook(file_path)
sheet = workbook.active
data = []
for row in sheet.iter_rows(values_only=True):
data.append(row)
return data
def process_data(data):
# 在此处添加处理数据的逻辑
processed_data = [list(map(lambda x: x*2 if isinstance(x, int) else x, row)) for row in data]
return processed_data
def save_file(data):
file_path = filedialog.asksaveasfilename(defaultextension=".xlsx", filetypes=[("Excel files", "*.xlsx *.xls")])
if file_path:
write_excel(file_path, data)
print(f"保存的文件路径: {file_path}")
def write_excel(file_path, data):
workbook = openpyxl.Workbook()
sheet = workbook.active
for row in data:
sheet.append(row)
workbook.save(file_path)
root = tk.Tk()
root.title("Excel 操作")
open_button = tk.Button(root, text="打开Excel文件", command=open_file)
open_button.pack(pady=20)
root.mainloop()
五、详细描述
1、用户界面设计
在上述代码中,我们使用tkinter
创建了一个简单的用户界面,包括一个按钮,当用户点击按钮时,会弹出文件选择对话框,用户可以选择一个Excel文件。filedialog.askopenfilename
方法用于打开文件选择对话框,filedialog.asksaveasfilename
方法用于打开保存文件对话框。
2、读取Excel文件
读取Excel文件时,我们使用openpyxl
库的load_workbook
方法加载工作簿,然后通过workbook.active
获取活动的工作表。sheet.iter_rows(values_only=True)
方法用于遍历工作表的所有行,并返回每一行的值。
3、处理数据
在process_data
函数中,我们对读取的数据进行了简单的处理。这个函数中的逻辑可以根据具体需求进行修改。例如,上述示例中我们只是将所有整数值乘以2。
4、写入Excel文件
写入Excel文件时,我们使用openpyxl
库的Workbook
类创建一个新的工作簿,然后通过sheet.append(row)
方法将处理后的数据逐行写入工作表,最后使用workbook.save(file_path)
方法保存工作簿。
六、使用pandas
库进行高级处理
除了openpyxl
,我们还可以使用pandas
库进行更高级的数据处理。pandas
库的read_excel
和to_excel
方法可以方便地读取和写入Excel文件,并且提供了强大的数据处理功能。
import pandas as pd
def read_excel_with_pandas(file_path):
df = pd.read_excel(file_path)
return df
def write_excel_with_pandas(file_path, df):
df.to_excel(file_path, index=False)
def process_data_with_pandas(df):
# 在此处添加处理数据的逻辑
df['Processed'] = df['Column1'] * 2 # 示例:将"Column1"列的值乘以2
return df
def open_file_with_pandas():
file_path = filedialog.askopenfilename(filetypes=[("Excel files", "*.xlsx *.xls")])
if file_path:
df = read_excel_with_pandas(file_path)
print(f"读取的数据: \n{df}")
processed_df = process_data_with_pandas(df)
save_file_with_pandas(processed_df)
def save_file_with_pandas(df):
file_path = filedialog.asksaveasfilename(defaultextension=".xlsx", filetypes=[("Excel files", "*.xlsx *.xls")])
if file_path:
write_excel_with_pandas(file_path, df)
print(f"保存的文件路径: {file_path}")
root = tk.Tk()
root.title("Excel 操作")
open_button = tk.Button(root, text="打开Excel文件", command=open_file_with_pandas)
open_button.pack(pady=20)
root.mainloop()
在上述代码中,我们使用pandas
库的read_excel
方法读取Excel文件,to_excel
方法写入Excel文件,并通过DataFrame
对象进行数据处理。这种方法更加灵活和强大,适合处理复杂的数据分析任务。
七、添加更多功能
1、添加多个按钮
我们可以在用户界面中添加多个按钮,以实现不同的功能。例如,添加一个按钮用于读取数据,另一个按钮用于保存数据。
def create_gui():
root = tk.Tk()
root.title("Excel 操作")
read_button = tk.Button(root, text="读取Excel文件", command=open_file_with_pandas)
read_button.pack(pady=10)
save_button = tk.Button(root, text="保存Excel文件", command=lambda: save_file_with_pandas(processed_df))
save_button.pack(pady=10)
root.mainloop()
create_gui()
2、添加更多的控件
除了按钮,我们还可以添加文本框、下拉菜单等控件,以实现更多的交互功能。
def create_gui():
root = tk.Tk()
root.title("Excel 操作")
label = tk.Label(root, text="请输入处理参数:")
label.pack(pady=5)
entry = tk.Entry(root)
entry.pack(pady=5)
read_button = tk.Button(root, text="读取Excel文件", command=open_file_with_pandas)
read_button.pack(pady=10)
save_button = tk.Button(root, text="保存Excel文件", command=lambda: save_file_with_pandas(processed_df))
save_button.pack(pady=10)
root.mainloop()
create_gui()
在上述代码中,我们添加了一个标签和一个文本框,用户可以在文本框中输入处理参数,然后点击按钮执行相应的操作。
八、总结
通过上述步骤,我们可以使用Python创建一个带有按钮的应用程序,用户可以通过按钮来操作Excel文件。tkinter
库用于创建图形用户界面,openpyxl
和pandas
库用于读取和写入Excel文件。这种方法可以方便地实现Excel文件的处理和分析,适合各种数据处理任务。希望这篇文章对你有所帮助!
相关问答FAQs:
如何在Python中创建一个按钮来操作Excel文件?
要在Python中创建一个按钮以操作Excel文件,您可以使用tkinter
库来构建图形用户界面(GUI),并结合pandas
或openpyxl
库来处理Excel文件。首先,安装所需的库(如pip install pandas openpyxl
),接着设计一个简单的界面,添加一个按钮,并在按钮点击事件中编写处理Excel文件的逻辑。例如,您可以创建一个按钮,点击后自动读取Excel数据并显示在界面上。
使用Python操作Excel时,可以实现哪些功能?
Python提供了多种库来操作Excel文件,您可以执行如读取数据、写入数据、更新单元格、删除行列、生成图表等功能。使用pandas
库,可以方便地进行数据分析与处理;而openpyxl
则适用于创建和修改Excel文件的格式与样式。结合按钮操作,用户可以实现自动化的数据处理流程。
在Python中处理Excel时遇到错误该如何解决?
处理Excel文件时,常见的错误包括文件路径不正确、格式不支持或权限问题。确保文件路径正确并且文件未被其他程序占用是基础。此外,若使用pandas
或openpyxl
读取文件时出现错误,检查是否安装了最新版本的库,以及文件格式是否符合要求。如果错误信息不明确,可以尝试在网上搜索该错误代码或信息,通常可以找到解决方案。
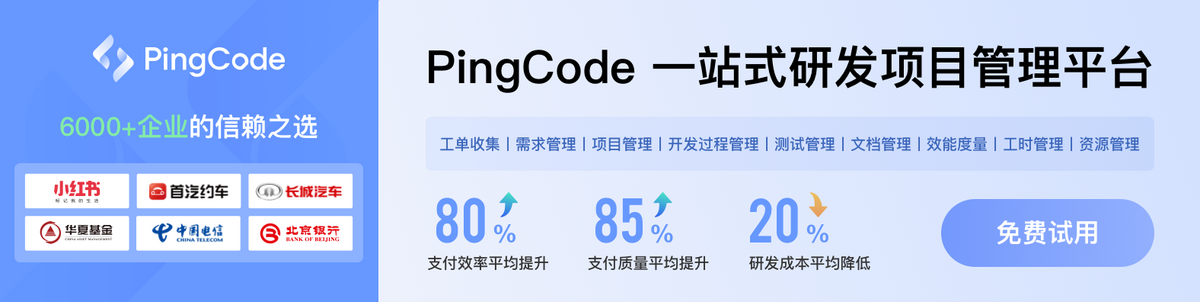