如何用Python去执行bat
使用subprocess
模块、使用os
模块、创建脚本的绝对路径是常用的方法。使用subprocess
模块是最推荐的方法,因为它提供了更强大的功能和更好的控制。具体来说,subprocess
模块可以捕获输出、处理错误和执行更复杂的命令。
使用subprocess
模块
subprocess
模块是Python 3.x中执行外部命令的推荐方式。它提供了一种更灵活和强大的方法来执行外部程序或命令。使用subprocess.run
或subprocess.call
可以执行bat文件并捕获其输出和错误。
import subprocess
def execute_bat_file(bat_file_path):
try:
result = subprocess.run([bat_file_path], capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file("path_to_your_bat_file.bat")
使用os
模块
os
模块提供了简单的接口来与操作系统进行交互,可以用来执行bat文件。不过这种方法相对subprocess
模块来说,功能较为有限,适用于简单的任务。
import os
def execute_bat_file(bat_file_path):
try:
os.system(f'cmd /c "{bat_file_path}"')
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file("path_to_your_bat_file.bat")
创建脚本的绝对路径
在执行bat文件时,指定其绝对路径可以避免路径相关的问题。这样可以确保脚本能够在任何工作目录下正确运行。
import subprocess
import os
def execute_bat_file(bat_file_path):
try:
abs_path = os.path.abspath(bat_file_path)
result = subprocess.run([abs_path], capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file("path_to_your_bat_file.bat")
如何选择合适的方法
选择哪种方法取决于具体需求和场景。如果需要更强大的功能和控制,推荐使用subprocess
模块;如果只是简单地执行一个bat文件,os.system
也可以胜任。此外,考虑到路径问题,最好使用绝对路径来指定bat文件的位置。
详细描述:使用subprocess
模块
1、捕获输出和错误
使用subprocess.run
或subprocess.Popen
可以捕获bat文件的输出和错误信息,方便调试和记录日志。
import subprocess
def execute_bat_file(bat_file_path):
try:
result = subprocess.run([bat_file_path], capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file("path_to_your_bat_file.bat")
2、处理复杂命令
subprocess
模块允许执行更复杂的命令,如带有参数的bat文件或需要在特定环境下运行的脚本。
import subprocess
def execute_bat_file_with_args(bat_file_path, *args):
try:
command = [bat_file_path] + list(args)
result = subprocess.run(command, capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_with_args("path_to_your_bat_file.bat", "arg1", "arg2")
3、执行异步任务
可以使用subprocess.Popen
来执行异步任务,不会阻塞主线程。
import subprocess
def execute_bat_file_async(bat_file_path):
try:
process = subprocess.Popen([bat_file_path], shell=True)
process.wait()
print("Process completed")
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_async("path_to_your_bat_file.bat")
4、指定工作目录和环境变量
subprocess.run
和subprocess.Popen
允许指定工作目录和环境变量,更灵活地控制执行环境。
import subprocess
import os
def execute_bat_file_in_directory(bat_file_path, working_directory):
try:
result = subprocess.run([bat_file_path], cwd=working_directory, capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_in_directory("path_to_your_bat_file.bat", "path_to_working_directory")
import subprocess
import os
def execute_bat_file_with_env(bat_file_path, env_vars):
try:
env = os.environ.copy()
env.update(env_vars)
result = subprocess.run([bat_file_path], env=env, capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_with_env("path_to_your_bat_file.bat", {"MY_VAR": "value"})
5、处理超时
可以设置超时时间,避免bat文件执行时间过长导致程序卡住。
import subprocess
def execute_bat_file_with_timeout(bat_file_path, timeout):
try:
result = subprocess.run([bat_file_path], capture_output=True, text=True, shell=True, timeout=timeout)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except subprocess.TimeoutExpired:
print(f"The process timed out after {timeout} seconds")
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_with_timeout("path_to_your_bat_file.bat", 10)
6、检查返回码
可以检查bat文件的返回码,判断其执行是否成功。
import subprocess
def execute_bat_file_and_check_return_code(bat_file_path):
try:
result = subprocess.run([bat_file_path], capture_output=True, text=True, shell=True)
if result.returncode == 0:
print("The process completed successfully")
else:
print(f"The process failed with return code {result.returncode}")
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_and_check_return_code("path_to_your_bat_file.bat")
7、处理大输出
对于输出较大的bat文件,可以使用subprocess.PIPE
来处理,以避免输出过多导致的阻塞。
import subprocess
def execute_bat_file_with_large_output(bat_file_path):
try:
process = subprocess.Popen([bat_file_path], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True, shell=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
errors = process.stderr.read()
if errors:
print("Errors:")
print(errors)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_with_large_output("path_to_your_bat_file.bat")
8、处理特殊字符
在执行包含特殊字符的命令时,确保正确转义字符,以避免命令执行失败。
import subprocess
def execute_bat_file_with_special_chars(bat_file_path, special_chars):
try:
command = [bat_file_path] + [f'"{char}"' for char in special_chars]
result = subprocess.run(command, capture_output=True, text=True, shell=True)
print("Output:")
print(result.stdout)
print("Errors:")
print(result.stderr)
except Exception as e:
print(f"An error occurred: {e}")
execute_bat_file_with_special_chars("path_to_your_bat_file.bat", ["arg with space", "arg&with&special^chars"])
9、使用日志记录
使用日志记录执行过程和结果,便于后续分析和调试。
import subprocess
import logging
logging.basicConfig(filename='bat_execution.log', level=logging.INFO)
def execute_bat_file_with_logging(bat_file_path):
try:
result = subprocess.run([bat_file_path], capture_output=True, text=True, shell=True)
logging.info(f"Output:\n{result.stdout}")
if result.stderr:
logging.error(f"Errors:\n{result.stderr}")
except Exception as e:
logging.error(f"An error occurred: {e}")
execute_bat_file_with_logging("path_to_your_bat_file.bat")
10、集成到应用程序
将执行bat文件的功能集成到更大的应用程序中,结合其他功能实现复杂的任务。
import subprocess
class BatExecutor:
def __init__(self, bat_file_path):
self.bat_file_path = bat_file_path
def execute(self):
try:
result = subprocess.run([self.bat_file_path], capture_output=True, text=True, shell=True)
return result.stdout, result.stderr
except Exception as e:
return None, str(e)
def main():
executor = BatExecutor("path_to_your_bat_file.bat")
output, error = executor.execute()
if output:
print("Output:")
print(output)
if error:
print("Errors:")
print(error)
if __name__ == "__main__":
main()
总结
使用subprocess
模块、使用os
模块、创建脚本的绝对路径是常用的方法。其中,使用subprocess
模块是最推荐的方法,因为它提供了更强大的功能和更好的控制。通过详细介绍使用subprocess
模块的方法,可以更好地理解和应用这种方法,满足实际需求。
相关问答FAQs:
如何在Python中调用一个.bat文件?
在Python中,可以使用subprocess
模块来执行.bat文件。通过subprocess.run()
或subprocess.Popen()
方法,可以轻松地调用.bat文件并获取其执行结果。示例代码如下:
import subprocess
subprocess.run(['path_to_your_bat_file.bat'])
确保提供正确的.bat文件路径,并根据需要处理任何输出或错误。
执行.bat文件时需要注意哪些事项?
在执行.bat文件时,需确保文件路径正确,且具有相应的执行权限。此外,环境变量和依赖项也可能影响.bat文件的执行。建议在命令行中先手动运行.bat文件,确认其能够正常执行,再在Python中调用。
如何处理.bat文件执行后的输出或错误信息?
可以在subprocess.run()
中使用参数capture_output=True
来捕获标准输出和错误信息。示例代码如下:
result = subprocess.run(['path_to_your_bat_file.bat'], capture_output=True, text=True)
print('Output:', result.stdout)
print('Error:', result.stderr)
这样可以方便地查看.bat文件执行的结果和可能出现的错误,便于后续的调试和处理。
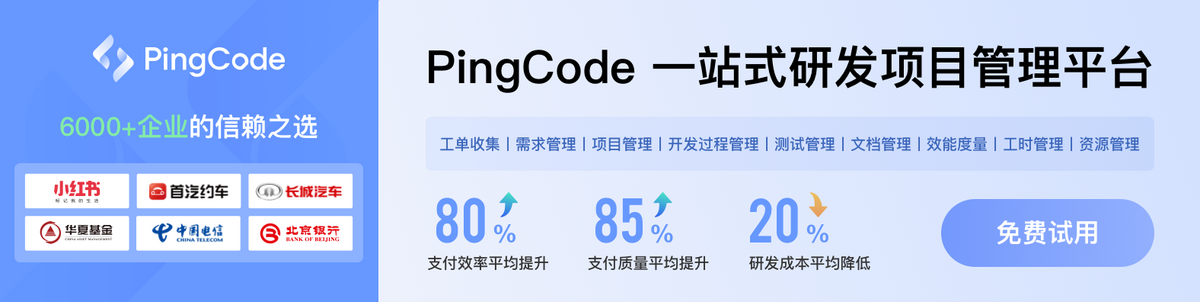