要在Python中创建库(lib),可以遵循以下核心步骤:确定库的功能和结构、编写库的代码、创建setup.py文件、打包和发布库。接下来,我们将详细探讨这些步骤中的每一个。
一、确定库的功能和结构
在创建库之前,首先需要明确库的功能和用途,这将有助于在开发过程中保持代码的清晰和有条理。确定功能之后,设计库的结构,包括模块的划分和文件的组织。
-
确定库的功能和用途
- 了解目标用户的需求。
- 评估现有解决方案的不足,找到改进的切入点。
- 明确库的主要功能和扩展功能。
-
设计库的结构
- 确定库的主要模块和子模块。
- 设计文件和文件夹的组织结构,保持代码的可维护性。
例如,如果你打算创建一个处理图像的库,可以设计如下结构:
my_image_lib/
__init__.py
core/
__init__.py
image_processing.py
filters.py
utils/
__init__.py
file_io.py
tests/
__init__.py
test_image_processing.py
test_filters.py
二、编写库的代码
编写库的代码是最重要的一步,确保代码质量高且功能齐全。以下是一些关键点:
-
模块化编程
- 将功能划分到不同的模块中,保持代码的清晰和可维护。
- 使用合适的文件和函数命名,确保代码易读。
-
编写文档
- 在代码中添加注释,解释函数和类的用途。
- 编写详细的README文件,介绍库的功能和使用方法。
-
编写测试用例
- 使用单元测试框架(如unittest、pytest)编写测试用例,确保代码的正确性。
- 尽量覆盖所有功能,保证代码的健壮性。
例如,在image_processing.py中可以定义如下函数:
# image_processing.py
def resize_image(image, size):
"""
Resize the given image to the specified size.
Args:
image (PIL.Image): The image to resize.
size (tuple): The target size as (width, height).
Returns:
PIL.Image: The resized image.
"""
return image.resize(size)
def convert_to_grayscale(image):
"""
Convert the given image to grayscale.
Args:
image (PIL.Image): The image to convert.
Returns:
PIL.Image: The grayscale image.
"""
return image.convert('L')
三、创建setup.py文件
setup.py文件是Python项目的配置文件,用于定义项目的名称、版本、依赖项等信息。
-
基本配置
- 设置项目的名称、版本、作者等基本信息。
- 定义项目的依赖项,确保用户安装库时能够自动安装这些依赖项。
-
打包配置
- 配置打包选项,指定需要包含的文件和排除的文件。
- 设置入口点,指定库的主模块。
例如,以下是一个简单的setup.py文件:
from setuptools import setup, find_packages
setup(
name='my_image_lib',
version='0.1.0',
author='Your Name',
author_email='your.email@example.com',
description='A library for image processing',
long_description=open('README.md').read(),
long_description_content_type='text/markdown',
url='https://github.com/yourusername/my_image_lib',
packages=find_packages(),
install_requires=[
'Pillow',
],
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
python_requires='>=3.6',
)
四、打包和发布库
打包和发布库是最后一步,确保你的库可以被其他用户安装和使用。
-
打包库
- 使用setuptools和wheel工具打包库。
- 生成适合发布的分发文件(如.tar.gz和.whl文件)。
-
发布库
- 在PyPI(Python Package Index)上发布库,使其可以通过pip安装。
- 使用twine工具上传分发文件到PyPI。
例如,以下是打包和发布库的步骤:
- 安装打包和发布工具:
pip install setuptools wheel twine
- 生成分发文件:
python setup.py sdist bdist_wheel
- 上传分发文件到PyPI:
twine upload dist/*
五、维护和更新库
发布库后,维护和更新库是确保其长期稳定运行的关键。
-
修复bug
- 定期检查用户反馈,及时修复bug。
- 保持代码的高质量和稳定性。
-
添加新功能
- 根据用户需求,添加新的功能和模块。
- 保持库的灵活性和扩展性。
-
更新文档
- 及时更新文档,确保用户能够了解最新的功能和用法。
- 提供详细的使用示例和教程。
例如,可以在GitHub上创建一个项目仓库,方便用户提交问题和建议:
# my_image_lib
A library for image processing.
## Installation
pip install my_image_lib
## Usage
```python
from my_image_lib.core import image_processing
Load an image
image = Image.open('example.jpg')
Resize the image
resized_image = image_processing.resize_image(image, (100, 100))
Convert the image to grayscale
grayscale_image = image_processing.convert_to_grayscale(image)
Contributing
Feel free to open issues and submit pull requests.
### 六、示例项目
<strong>为了更好地理解创建库的过程,我们可以通过一个示例项目来实践</strong>。
1. <strong>创建项目文件夹和结构:</strong>
```bash
mkdir my_image_lib
cd my_image_lib
mkdir core utils tests
touch core/__init__.py core/image_processing.py core/filters.py
touch utils/__init__.py utils/file_io.py
touch tests/__init__.py tests/test_image_processing.py tests/test_filters.py
touch setup.py README.md
- 编写核心代码:
# core/image_processing.py
from PIL import Image
def resize_image(image, size):
"""
Resize the given image to the specified size.
Args:
image (PIL.Image): The image to resize.
size (tuple): The target size as (width, height).
Returns:
PIL.Image: The resized image.
"""
return image.resize(size)
def convert_to_grayscale(image):
"""
Convert the given image to grayscale.
Args:
image (PIL.Image): The image to convert.
Returns:
PIL.Image: The grayscale image.
"""
return image.convert('L')
- 编写测试用例:
# tests/test_image_processing.py
import unittest
from PIL import Image
from core.image_processing import resize_image, convert_to_grayscale
class TestImageProcessing(unittest.TestCase):
def setUp(self):
self.image = Image.new('RGB', (200, 200), color = 'red')
def test_resize_image(self):
resized_image = resize_image(self.image, (100, 100))
self.assertEqual(resized_image.size, (100, 100))
def test_convert_to_grayscale(self):
grayscale_image = convert_to_grayscale(self.image)
self.assertEqual(grayscale_image.mode, 'L')
if __name__ == '__main__':
unittest.main()
- 编写setup.py文件:
from setuptools import setup, find_packages
setup(
name='my_image_lib',
version='0.1.0',
author='Your Name',
author_email='your.email@example.com',
description='A library for image processing',
long_description=open('README.md').read(),
long_description_content_type='text/markdown',
url='https://github.com/yourusername/my_image_lib',
packages=find_packages(),
install_requires=[
'Pillow',
],
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
python_requires='>=3.6',
)
- 打包和发布库:
pip install setuptools wheel twine
python setup.py sdist bdist_wheel
twine upload dist/*
通过以上步骤,我们创建了一个简单的图像处理库,并成功发布到PyPI。这个过程不仅展示了如何创建库的基本步骤,还提供了一个完整的示例项目,帮助你更好地理解和实践。
七、总结
创建Python库是一个系统性的过程,需要从明确库的功能和结构开始,到编写代码、创建setup.py文件、打包和发布库,最后维护和更新库。每一个步骤都至关重要,确保库的高质量和易用性。通过以上详细的步骤和示例项目,相信你已经掌握了创建Python库的基本方法和技巧。希望你能在实际开发中应用这些知识,创建出功能强大、用户友好的Python库。
相关问答FAQs:
如何在Python中创建一个库?
创建Python库的过程相对简单,您需要首先定义库的功能并组织代码。通常,您需要创建一个文件夹,包含一个或多个Python模块(.py文件),并在其中添加一个__init__.py
文件,以便Python能够识别该文件夹为一个包。之后,您可以在这些模块中编写您的函数和类,最后通过setup.py
文件将其打包和分发。
在创建Python库时如何管理依赖关系?
在创建库时,管理依赖关系是至关重要的。您可以在setup.py
文件中使用install_requires
参数来声明您库所需的外部库。此外,使用requirements.txt
文件可以更方便地列出所有依赖项,以便其他开发者在安装时轻松获取。
如何测试我的Python库以确保其功能正常?
测试是确保库功能正常的重要步骤。您可以使用Python的内置unittest
模块或第三方库如pytest
来编写测试用例。创建一个tests
文件夹,在其中写下针对库各个功能的测试代码。运行测试后,确保所有测试都通过,以验证库的可靠性。
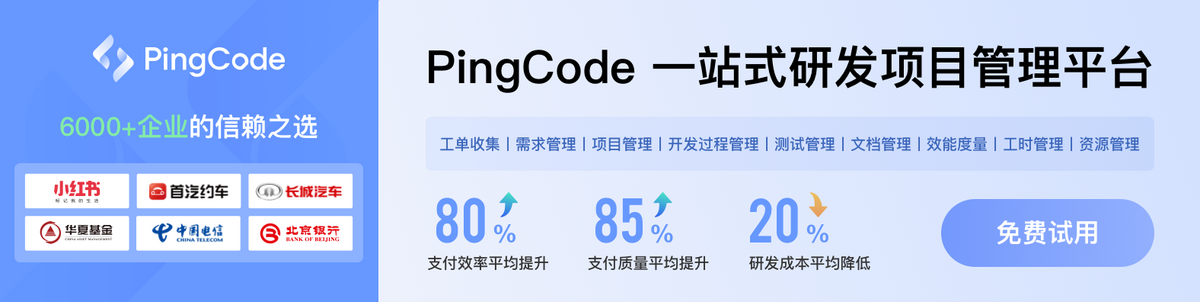