要用Python打印购物小票,有几种常见的方法,包括使用文本文件、使用打印机库、使用GUI库等。本文将详细介绍如何通过不同的方法来打印购物小票,并提供代码示例以帮助理解。
一、使用文本文件
使用文本文件是实现购物小票打印的一种简单方法。你可以将购物小票信息写入文本文件,然后通过系统的打印功能将该文件打印出来。
def print_receipt(items, total, filename="receipt.txt"):
with open(filename, "w") as f:
f.write("<strong></strong><strong> 购物小票 </strong><strong></strong>\n")
for item in items:
f.write(f"{item['name']} x{item['quantity']} @ {item['price']} each = {item['quantity'] * item['price']}\n")
f.write(f"----------------------\n")
f.write(f"Total: {total}\n")
f.write("<strong></strong><strong> 感谢您的惠顾 </strong><strong></strong>\n")
items = [
{"name": "苹果", "quantity": 2, "price": 3.5},
{"name": "香蕉", "quantity": 1, "price": 2.0},
{"name": "橙子", "quantity": 3, "price": 4.0}
]
total = sum(item['quantity'] * item['price'] for item in items)
print_receipt(items, total)
详细描述: 使用文本文件的方式比较直观且易于理解。你可以将购物小票的信息按行写入文件中,并且可以灵活地控制每一行的格式。此方法适用于简单的打印需求,但在需要更复杂的格式或特性时可能不太适用。
二、使用打印机库
使用打印机库可以直接与打印机交互,这样可以方便地打印购物小票。Python中有一些库,如win32print
和escpos
,可以用于与打印机交互。
- 使用
win32print
库(仅适用于Windows)
import win32print
import win32ui
from PIL import Image, ImageWin
def print_receipt(items, total):
printer_name = win32print.GetDefaultPrinter()
hDC = win32ui.CreateDC()
hDC.CreatePrinterDC(printer_name)
hDC.StartDoc("Receipt")
hDC.StartPage()
text = "<strong></strong><strong> 购物小票 </strong><strong></strong>\n"
for item in items:
text += f"{item['name']} x{item['quantity']} @ {item['price']} each = {item['quantity'] * item['price']}\n"
text += "----------------------\n"
text += f"Total: {total}\n"
text += "<strong></strong><strong> 感谢您的惠顾 </strong><strong></strong>\n"
hDC.TextOut(100, 100, text)
hDC.EndPage()
hDC.EndDoc()
hDC.DeleteDC()
items = [
{"name": "苹果", "quantity": 2, "price": 3.5},
{"name": "香蕉", "quantity": 1, "price": 2.0},
{"name": "橙子", "quantity": 3, "price": 4.0}
]
total = sum(item['quantity'] * item['price'] for item in items)
print_receipt(items, total)
- 使用
escpos
库(适用于热敏打印机)
首先需要安装python-escpos
库:
pip install python-escpos
然后使用以下代码来打印购物小票:
from escpos.printer import Usb
def print_receipt(items, total):
# 连接到打印机(根据实际情况修改)
p = Usb(0x04b8, 0x0e15)
p.text("<strong></strong><strong> 购物小票 </strong><strong></strong>\n")
for item in items:
p.text(f"{item['name']} x{item['quantity']} @ {item['price']} each = {item['quantity'] * item['price']}\n")
p.text("----------------------\n")
p.text(f"Total: {total}\n")
p.text("<strong></strong><strong> 感谢您的惠顾 </strong><strong></strong>\n")
p.cut()
items = [
{"name": "苹果", "quantity": 2, "price": 3.5},
{"name": "香蕉", "quantity": 1, "price": 2.0},
{"name": "橙子", "quantity": 3, "price": 4.0}
]
total = sum(item['quantity'] * item['price'] for item in items)
print_receipt(items, total)
详细描述: 使用打印机库可以直接控制打印机打印购物小票,适用于需要频繁打印的场景。win32print
适用于Windows系统,而escpos
适用于热敏打印机,这两种方法都可以灵活地调整打印内容和格式。
三、使用GUI库
使用GUI库(如Tkinter)可以创建一个图形界面,并通过该界面打印购物小票。这种方法适用于需要用户交互的场景。
import tkinter as tk
from tkinter import messagebox
def print_receipt(items, total):
receipt_text = "<strong></strong><strong> 购物小票 </strong><strong></strong>\n"
for item in items:
receipt_text += f"{item['name']} x{item['quantity']} @ {item['price']} each = {item['quantity'] * item['price']}\n"
receipt_text += "----------------------\n"
receipt_text += f"Total: {total}\n"
receipt_text += "<strong></strong><strong> 感谢您的惠顾 </strong><strong></strong>\n"
messagebox.showinfo("购物小票", receipt_text)
def main():
items = [
{"name": "苹果", "quantity": 2, "price": 3.5},
{"name": "香蕉", "quantity": 1, "price": 2.0},
{"name": "橙子", "quantity": 3, "price": 4.0}
]
total = sum(item['quantity'] * item['price'] for item in items)
root = tk.Tk()
root.withdraw() # 隐藏主窗口
print_receipt(items, total)
if __name__ == "__main__":
main()
详细描述: 使用GUI库可以为用户提供一个友好的界面,用户可以通过该界面查看和打印购物小票。Tkinter是Python的标准GUI库,使用它可以轻松创建窗口和对话框。
四、总结
使用Python打印购物小票的方法有多种,可以根据具体需求选择合适的方法。使用文本文件方法简单易用、使用打印机库方法适用于频繁打印、使用GUI库方法适用于需要用户交互的场景。通过这些方法,你可以灵活地打印购物小票,并根据需求调整打印内容和格式。
相关问答FAQs:
1. 如何使用Python生成购物小票的格式?
生成购物小票的格式可以通过使用Python中的字符串格式化功能来实现。可以创建一个模板,包含商品名称、数量、单价和总价等信息。利用print()
函数将格式化后的字符串输出到控制台,或者使用文件操作将其保存为文本文件。示例代码如下:
def print_receipt(items):
total = 0
print("购物小票")
print("-------------------")
for item in items:
name = item['name']
price = item['price']
quantity = item['quantity']
item_total = price * quantity
total += item_total
print(f"{name}: {quantity} x {price} = {item_total}")
print("-------------------")
print(f"总计: {total}")
2. 如何在Python中打印购物小票的条形码?
在购物小票中添加条形码可以使用第三方库,如python-barcode
。安装该库后,可以生成条形码并将其保存为图像格式,然后使用打印功能将其打印到小票上。以下是基本步骤:
import barcode
from barcode.writer import ImageWriter
def generate_barcode(data):
code = barcode.get('ean13', data, writer=ImageWriter())
code.save('barcode')
这段代码会生成一个名为“barcode.png”的条形码图像,可以通过打印机将其打印到小票上。
3. 如何将Python打印的购物小票发送到热敏打印机?
要将购物小票直接发送到热敏打印机,可以使用pyusb
或python-escpos
等库。这些库支持与热敏打印机的通信,能够发送格式化的文本和图像。安装python-escpos
后,可以通过以下方式进行打印:
from escpos.printer import Usb
p = Usb(0x04b8, 0x0202) # 替换为你的打印机ID
p.text("购物小票\n")
p.text("-------------------\n")
# 添加更多打印内容
p.cut() # 切纸
确保在代码中使用正确的USB设备ID,并按需调整打印内容。
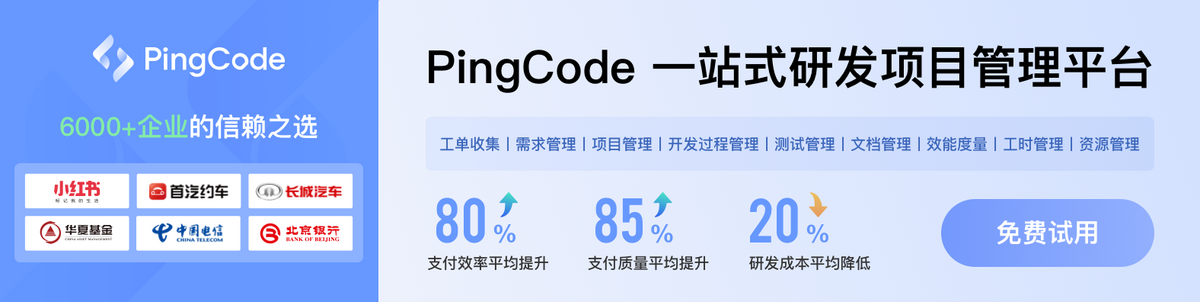