在Python中,可以通过os模块、sys模块、pathlib模块获得文件存储路径、os.path.abspath()函数、sys.argv[]函数、pathlib.Path.resolve()函数。 其中,os模块和pathlib模块是最常用的两种方法。接下来将详细介绍如何使用这些方法来获取文件存储路径。
一、OS模块
os模块是Python标准库中的一个模块,提供了一些与操作系统进行交互的函数。使用os模块获取文件存储路径的主要函数是os.path.abspath()。
1. os.path.abspath()函数
os.path.abspath()函数用于返回文件的绝对路径。绝对路径是指从根目录开始的路径,包含了文件的完整路径信息。示例如下:
import os
获取当前文件的绝对路径
current_file_path = os.path.abspath(__file__)
print(f"Current file absolute path: {current_file_path}")
获取指定文件的绝对路径
file_name = "example.txt"
file_path = os.path.abspath(file_name)
print(f"Example file absolute path: {file_path}")
在上述代码中,os.path.abspath()函数返回了当前文件和指定文件的绝对路径。
2. os.getcwd()函数
os.getcwd()函数用于获取当前工作目录的路径。示例如下:
import os
获取当前工作目录
current_directory = os.getcwd()
print(f"Current working directory: {current_directory}")
在上述代码中,os.getcwd()函数返回了当前工作目录的路径。
二、SYS模块
sys模块是Python标准库中的另一个模块,提供了一些与Python解释器进行交互的函数。使用sys模块获取文件存储路径的主要方法是sys.argv[]。
1. sys.argv[]数组
sys.argv[]数组是一个列表,包含了命令行参数。第一个元素(即sys.argv[0])是脚本的名称,包含了脚本的路径信息。示例如下:
import sys
import os
获取当前脚本的路径
script_path = sys.argv[0]
print(f"Script path: {script_path}")
将脚本路径转换为绝对路径
absolute_script_path = os.path.abspath(script_path)
print(f"Absolute script path: {absolute_script_path}")
在上述代码中,sys.argv[0]返回了当前脚本的路径,并通过os.path.abspath()函数将其转换为绝对路径。
三、Pathlib模块
pathlib模块是Python 3.4引入的一个模块,提供了面向对象的路径操作方法。使用pathlib模块获取文件存储路径的主要方法是pathlib.Path.resolve()。
1. pathlib.Path.resolve()函数
pathlib.Path.resolve()函数用于返回文件的绝对路径。示例如下:
from pathlib import Path
获取当前文件的绝对路径
current_file_path = Path(__file__).resolve()
print(f"Current file absolute path: {current_file_path}")
获取指定文件的绝对路径
file_name = "example.txt"
file_path = Path(file_name).resolve()
print(f"Example file absolute path: {file_path}")
在上述代码中,pathlib.Path.resolve()函数返回了当前文件和指定文件的绝对路径。
四、实例应用
在实际应用中,我们经常需要获取文件存储路径,以便进行文件读写操作。以下是一个示例,展示了如何使用上述方法来实现文件的读写操作。
1. 读取文件内容
首先,我们创建一个名为example.txt的文件,并写入一些内容。然后,我们使用os模块和pathlib模块读取文件内容。
# 创建example.txt文件,并写入内容
with open("example.txt", "w") as file:
file.write("Hello, world!")
使用os模块读取文件内容
import os
file_path = os.path.abspath("example.txt")
with open(file_path, "r") as file:
content = file.read()
print(f"Content read using os module: {content}")
使用pathlib模块读取文件内容
from pathlib import Path
file_path = Path("example.txt").resolve()
with open(file_path, "r") as file:
content = file.read()
print(f"Content read using pathlib module: {content}")
在上述代码中,我们创建了一个名为example.txt的文件,并写入了"Hello, world!"内容。然后,我们分别使用os模块和pathlib模块读取文件内容,并打印出来。
2. 写入文件内容
接下来,我们使用os模块和pathlib模块向example.txt文件写入新的内容。
# 使用os模块写入文件内容
import os
file_path = os.path.abspath("example.txt")
with open(file_path, "w") as file:
file.write("New content written using os module.")
使用pathlib模块写入文件内容
from pathlib import Path
file_path = Path("example.txt").resolve()
with open(file_path, "w") as file:
file.write("New content written using pathlib module.")
在上述代码中,我们分别使用os模块和pathlib模块向example.txt文件写入了新的内容。
通过上述示例,我们可以看到,使用os模块和pathlib模块获取文件存储路径是非常方便的,并且可以轻松实现文件的读写操作。
五、总结
在Python中,获取文件存储路径的方法有多种,常用的有os模块、sys模块和pathlib模块。os.path.abspath()函数、sys.argv[]数组、pathlib.Path.resolve()函数是获取文件存储路径的主要方法。通过这些方法,我们可以方便地获取文件的绝对路径,并进行文件读写操作。
在实际应用中,根据具体需求选择合适的方法来获取文件存储路径,可以提高代码的可读性和可维护性。希望通过本文的介绍,能够帮助大家更好地理解和使用Python获取文件存储路径的方法。
相关问答FAQs:
如何在Python中获取当前工作目录?
可以使用os
模块的getcwd()
函数来获取当前工作目录。示例代码如下:
import os
current_directory = os.getcwd()
print("当前工作目录:", current_directory)
这将返回当前Python脚本运行时的目录路径。
如何通过Python获取特定文件的绝对路径?
使用os.path.abspath()
函数可以获取特定文件的绝对路径。只需传入文件名或相对路径即可。示例代码如下:
import os
file_name = "example.txt"
absolute_path = os.path.abspath(file_name)
print("文件的绝对路径:", absolute_path)
此代码将输出指定文件的绝对路径。
在Python中如何获取文件存储路径的父目录?
可以使用os.path.dirname()
函数结合os.path.abspath()
来获取文件存储路径的父目录。示例代码如下:
import os
file_path = "example.txt"
parent_directory = os.path.dirname(os.path.abspath(file_path))
print("文件存储路径的父目录:", parent_directory)
这将返回指定文件的父目录路径,方便后续的文件管理操作。
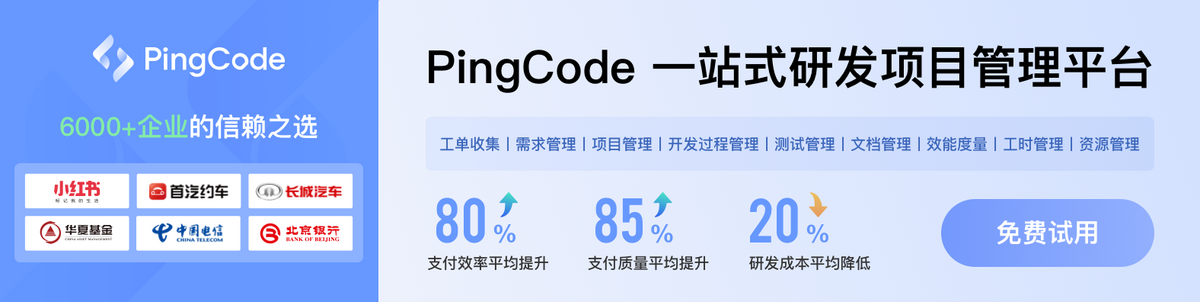