Python画图时,可以使用matplotlib中的subplot功能来实现一轴默认、提高图形的可读性和美观度。通过配置subplot参数、调整图形大小、设置标签和标题,可以更好地控制图形的布局。其中,subplot功能尤其重要。
下面详细描述如何使用subplot功能:
matplotlib 是 Python 中一个常用的绘图库,它提供了一系列用于创建静态、动态和交互式图形的工具。subplot 功能允许在一个图形窗口中创建多个子图,每个子图都有自己的坐标轴。这样可以在一个窗口中展示多个数据集,便于比较和分析。
一、MATPLOTLIB基础
1、安装与导入
在开始绘图之前,首先需要安装并导入 matplotlib。可以通过以下命令安装:
pip install matplotlib
安装完成后,在代码中导入 matplotlib:
import matplotlib.pyplot as plt
2、创建基本图形
在 matplotlib 中,最基本的图形是通过 plt.plot()
函数创建的。例如:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.xlabel('x-axis')
plt.ylabel('y-axis')
plt.title('Basic Plot')
plt.show()
二、SUBPLOT功能
1、什么是SUBPLOT
subplot
是 matplotlib 中一个非常强大的功能,它允许我们在一个图形窗口中创建多个子图。每个子图都有自己的坐标轴,可以独立显示不同的数据集。
2、如何使用SUBPLOT
subplot
函数的基本语法如下:
plt.subplot(nrows, ncols, index)
其中,nrows
和 ncols
分别表示图形的行数和列数,index
表示当前子图的位置。例如,创建一个包含四个子图(2 行 2 列)的图形:
import matplotlib.pyplot as plt
创建一个包含4个子图(2行2列)的图形
plt.subplot(2, 2, 1)
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Subplot 1')
plt.subplot(2, 2, 2)
plt.plot([1, 2, 3], [7, 8, 9])
plt.title('Subplot 2')
plt.subplot(2, 2, 3)
plt.plot([1, 2, 3], [10, 11, 12])
plt.title('Subplot 3')
plt.subplot(2, 2, 4)
plt.plot([1, 2, 3], [13, 14, 15])
plt.title('Subplot 4')
plt.tight_layout()
plt.show()
在这个例子中,我们创建了一个包含 4 个子图(2 行 2 列)的图形,并在每个子图中绘制了一条简单的折线图。使用 plt.tight_layout()
函数可以自动调整子图之间的间距,使图形更加美观。
三、SUBPLOT参数配置
1、调整子图大小
可以通过 figsize
参数调整整个图形的大小。例如:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(10, 8))
axs[0, 0].plot([1, 2, 3], [4, 5, 6])
axs[0, 0].set_title('Subplot 1')
axs[0, 1].plot([1, 2, 3], [7, 8, 9])
axs[0, 1].set_title('Subplot 2')
axs[1, 0].plot([1, 2, 3], [10, 11, 12])
axs[1, 0].set_title('Subplot 3')
axs[1, 1].plot([1, 2, 3], [13, 14, 15])
axs[1, 1].set_title('Subplot 4')
plt.tight_layout()
plt.show()
2、共享坐标轴
在某些情况下,可能希望多个子图共享相同的坐标轴。可以通过 sharex
和 sharey
参数实现。例如:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(10, 8), sharex=True, sharey=True)
axs[0, 0].plot([1, 2, 3], [4, 5, 6])
axs[0, 0].set_title('Subplot 1')
axs[0, 1].plot([1, 2, 3], [7, 8, 9])
axs[0, 1].set_title('Subplot 2')
axs[1, 0].plot([1, 2, 3], [10, 11, 12])
axs[1, 0].set_title('Subplot 3')
axs[1, 1].plot([1, 2, 3], [13, 14, 15])
axs[1, 1].set_title('Subplot 4')
plt.tight_layout()
plt.show()
在这个例子中,所有子图共享相同的 x 轴和 y 轴。
四、图形美化
1、设置标签和标题
为了使图形更加直观,可以为每个子图设置标签和标题。例如:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(10, 8))
axs[0, 0].plot([1, 2, 3], [4, 5, 6])
axs[0, 0].set_title('Subplot 1')
axs[0, 0].set_xlabel('x-axis')
axs[0, 0].set_ylabel('y-axis')
axs[0, 1].plot([1, 2, 3], [7, 8, 9])
axs[0, 1].set_title('Subplot 2')
axs[0, 1].set_xlabel('x-axis')
axs[0, 1].set_ylabel('y-axis')
axs[1, 0].plot([1, 2, 3], [10, 11, 12])
axs[1, 0].set_title('Subplot 3')
axs[1, 0].set_xlabel('x-axis')
axs[1, 0].set_ylabel('y-axis')
axs[1, 1].plot([1, 2, 3], [13, 14, 15])
axs[1, 1].set_title('Subplot 4')
axs[1, 1].set_xlabel('x-axis')
axs[1, 1].set_ylabel('y-axis')
plt.tight_layout()
plt.show()
2、添加网格
可以通过 grid
函数为子图添加网格线。例如:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2, figsize=(10, 8))
axs[0, 0].plot([1, 2, 3], [4, 5, 6])
axs[0, 0].set_title('Subplot 1')
axs[0, 0].grid(True)
axs[0, 1].plot([1, 2, 3], [7, 8, 9])
axs[0, 1].set_title('Subplot 2')
axs[0, 1].grid(True)
axs[1, 0].plot([1, 2, 3], [10, 11, 12])
axs[1, 0].set_title('Subplot 3')
axs[1, 0].grid(True)
axs[1, 1].plot([1, 2, 3], [13, 14, 15])
axs[1, 1].set_title('Subplot 4')
axs[1, 1].grid(True)
plt.tight_layout()
plt.show()
五、综合示例
下面是一个综合示例,展示如何在一个图形窗口中创建多个子图,并进行美化:
import matplotlib.pyplot as plt
import numpy as np
数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
创建一个包含4个子图(2行2列)的图形
fig, axs = plt.subplots(2, 2, figsize=(12, 10))
子图1
axs[0, 0].plot(x, y1, 'r')
axs[0, 0].set_title('Sine')
axs[0, 0].set_xlabel('x')
axs[0, 0].set_ylabel('y')
axs[0, 0].grid(True)
子图2
axs[0, 1].plot(x, y2, 'g')
axs[0, 1].set_title('Cosine')
axs[0, 1].set_xlabel('x')
axs[0, 1].set_ylabel('y')
axs[0, 1].grid(True)
子图3
axs[1, 0].plot(x, y3, 'b')
axs[1, 0].set_title('Tangent')
axs[1, 0].set_xlabel('x')
axs[1, 0].set_ylabel('y')
axs[1, 0].grid(True)
子图4
axs[1, 1].plot(x, y4, 'm')
axs[1, 1].set_title('Exponential')
axs[1, 1].set_xlabel('x')
axs[1, 1].set_ylabel('y')
axs[1, 1].grid(True)
plt.tight_layout()
plt.show()
在这个综合示例中,我们创建了一个包含 4 个子图(2 行 2 列)的图形,每个子图展示一个不同的函数(正弦、余弦、正切和指数函数)。我们还为每个子图设置了标题、标签和网格线,并调整了整个图形的大小。
通过以上方法,可以在 Python 中使用 matplotlib 创建多个子图,并进行美化和调整,提高图形的可读性和美观度。希望这些内容对您有所帮助!
相关问答FAQs:
如何在Python中设置默认坐标轴?
在Python的绘图库中,比如Matplotlib,您可以通过plt.axis()
函数来设置坐标轴的范围和样式。使用plt.axis('default')
可以将坐标轴恢复到默认设置,或者使用plt.xlim()
和plt.ylim()
来手动设置x轴和y轴的范围,从而达到控制坐标轴的目的。
在绘图时,如何自定义坐标轴的刻度和标签?
要自定义坐标轴的刻度和标签,可以使用plt.xticks()
和plt.yticks()
函数。这两个函数允许您指定刻度的位置和标签的文本内容。例如,您可以设置特定的刻度值和对应的标签,以便更好地展示数据。
在Python中绘图时,怎样使坐标轴的样式更加美观?
为了让绘图的坐标轴更加美观,可以通过设置线条样式、颜色以及字体大小等来实现。Matplotlib提供了丰富的选项,例如使用plt.tick_params()
来调整刻度线的长度、宽度和颜色。此外,可以使用plt.xlabel()
和plt.ylabel()
来添加带有样式的坐标轴标签,从而提高整体的视觉效果。
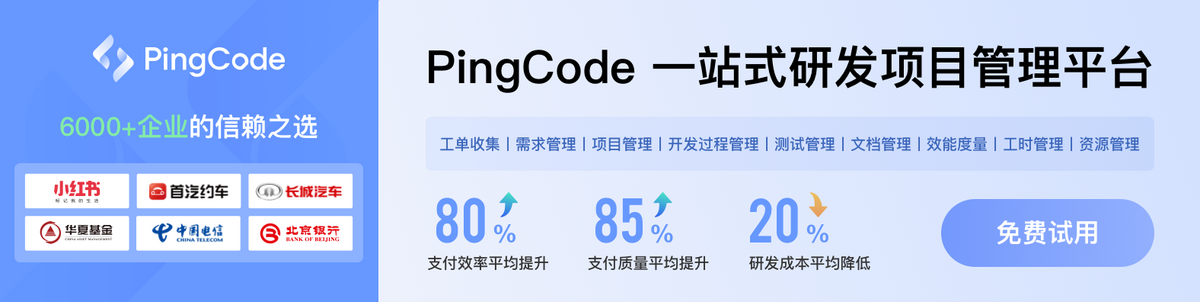