利用多态、使用字典映射、使用策略模式、使用函数代替条件判断,这些方法都可以在Python中避免频繁使用if-else语句。下面将详细展开其中的一点:使用字典映射。
使用字典映射是一种常见且有效的方法来避免过多的if-else语句。通过将条件作为键值对存储在字典中,程序可以更简洁地选择要执行的代码逻辑。例如,如果你有多种操作需要根据不同的输入来执行,可以将这些操作函数存储在一个字典中,然后根据输入直接调用相应的函数。
一、利用多态
多态是面向对象编程中的一个概念,它允许不同的对象以不同的方式响应相同的消息。在Python中,可以通过继承和方法重写来实现多态。
1.1 定义基类和子类
首先,我们定义一个基类和一些子类,每个子类实现一个特定的行为。这样,基于对象的类型,我们可以调用相同的方法,而无需使用if-else语句。
class Operation:
def execute(self, a, b):
raise NotImplementedError("This method should be overridden by subclasses")
class AddOperation(Operation):
def execute(self, a, b):
return a + b
class SubtractOperation(Operation):
def execute(self, a, b):
return a - b
class MultiplyOperation(Operation):
def execute(self, a, b):
return a * b
class DivideOperation(Operation):
def execute(self, a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
1.2 使用多态执行操作
在使用这些类时,我们不需要使用if-else来选择操作。相反,我们可以根据需要动态地创建对象并调用它们的execute
方法。
def perform_operation(operation, a, b):
return operation.execute(a, b)
add = AddOperation()
subtract = SubtractOperation()
multiply = MultiplyOperation()
divide = DivideOperation()
print(perform_operation(add, 10, 5)) # 输出: 15
print(perform_operation(subtract, 10, 5)) # 输出: 5
print(perform_operation(multiply, 10, 5)) # 输出: 50
print(perform_operation(divide, 10, 5)) # 输出: 2.0
二、使用字典映射
2.1 基础用法
字典映射是一种将条件与操作对应起来的简单而强大的方法。通过将操作封装在函数中并将它们存储在字典中,我们可以根据输入直接调用相应的函数。
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
operations = {
'add': add,
'subtract': subtract,
'multiply': multiply,
'divide': divide
}
2.2 使用字典映射执行操作
通过字典映射,我们可以避免使用if-else语句来选择操作。
def perform_operation(operation_name, a, b):
operation = operations.get(operation_name)
if operation is None:
raise ValueError(f"Unsupported operation: {operation_name}")
return operation(a, b)
print(perform_operation('add', 10, 5)) # 输出: 15
print(perform_operation('subtract', 10, 5)) # 输出: 5
print(perform_operation('multiply', 10, 5)) # 输出: 50
print(perform_operation('divide', 10, 5)) # 输出: 2.0
三、使用策略模式
策略模式是一种行为设计模式,它允许在运行时选择算法。通过定义一系列算法并将它们封装在独立的类中,我们可以动态地选择和使用这些算法,而无需使用if-else语句。
3.1 定义策略接口和具体策略
首先,我们定义一个策略接口和一些具体的策略类。
class Strategy:
def execute(self, a, b):
raise NotImplementedError("This method should be overridden by subclasses")
class AddStrategy(Strategy):
def execute(self, a, b):
return a + b
class SubtractStrategy(Strategy):
def execute(self, a, b):
return a - b
class MultiplyStrategy(Strategy):
def execute(self, a, b):
return a * b
class DivideStrategy(Strategy):
def execute(self, a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
3.2 定义上下文类
然后,我们定义一个上下文类来使用这些策略。
class Context:
def __init__(self, strategy):
self.strategy = strategy
def execute_strategy(self, a, b):
return self.strategy.execute(a, b)
3.3 使用策略模式
通过策略模式,我们可以在运行时动态地选择和使用不同的算法,而无需使用if-else语句。
context = Context(AddStrategy())
print(context.execute_strategy(10, 5)) # 输出: 15
context = Context(SubtractStrategy())
print(context.execute_strategy(10, 5)) # 输出: 5
context = Context(MultiplyStrategy())
print(context.execute_strategy(10, 5)) # 输出: 50
context = Context(DivideStrategy())
print(context.execute_strategy(10, 5)) # 输出: 2.0
四、使用函数代替条件判断
在Python中,函数是一等公民,可以将它们作为参数传递给其他函数。通过这种方式,我们可以使用函数来代替条件判断。
4.1 定义操作函数
首先,我们定义一组操作函数。
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
4.2 使用函数代替条件判断
通过将操作函数作为参数传递给另一个函数,我们可以避免使用if-else语句。
def perform_operation(operation, a, b):
return operation(a, b)
print(perform_operation(add, 10, 5)) # 输出: 15
print(perform_operation(subtract, 10, 5)) # 输出: 5
print(perform_operation(multiply, 10, 5)) # 输出: 50
print(perform_operation(divide, 10, 5)) # 输出: 2.0
五、使用装饰器模式
装饰器模式是一种结构设计模式,它允许动态地将行为附加到对象上。通过使用装饰器模式,我们可以在不改变对象类的情况下扩展对象的功能。
5.1 定义装饰器和被装饰的函数
首先,我们定义一个装饰器和一些被装饰的函数。
def operation_decorator(operation):
def wrapper(a, b):
print(f"Performing {operation.__name__} operation on {a} and {b}")
result = operation(a, b)
print(f"Result: {result}")
return result
return wrapper
@operation_decorator
def add(a, b):
return a + b
@operation_decorator
def subtract(a, b):
return a - b
@operation_decorator
def multiply(a, b):
return a * b
@operation_decorator
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
5.2 使用装饰器模式执行操作
通过装饰器模式,我们可以在执行操作时动态地添加行为,而无需使用if-else语句。
print(add(10, 5)) # 输出: Performing add operation on 10 and 5, Result: 15, 15
print(subtract(10, 5)) # 输出: Performing subtract operation on 10 and 5, Result: 5, 5
print(multiply(10, 5)) # 输出: Performing multiply operation on 10 and 5, Result: 50, 50
print(divide(10, 5)) # 输出: Performing divide operation on 10 and 5, Result: 2.0, 2.0
六、使用lambda表达式
Lambda表达式是一种匿名函数,可以用来简化代码并避免使用if-else语句。在Python中,lambda表达式是一种简洁的方式来定义简单的函数。
6.1 定义操作lambda表达式
首先,我们定义一组操作lambda表达式。
operations = {
'add': lambda a, b: a + b,
'subtract': lambda a, b: a - b,
'multiply': lambda a, b: a * b,
'divide': lambda a, b: a / b if b != 0 else ValueError("Cannot divide by zero!")
}
6.2 使用lambda表达式执行操作
通过lambda表达式,我们可以避免使用if-else语句来选择操作。
def perform_operation(operation_name, a, b):
operation = operations.get(operation_name)
if operation is None:
raise ValueError(f"Unsupported operation: {operation_name}")
return operation(a, b)
print(perform_operation('add', 10, 5)) # 输出: 15
print(perform_operation('subtract', 10, 5)) # 输出: 5
print(perform_operation('multiply', 10, 5)) # 输出: 50
print(perform_operation('divide', 10, 5)) # 输出: 2.0
七、使用闭包
闭包是一种可以捕捉和携带自由变量的函数。在Python中,闭包可以用来封装逻辑并避免使用if-else语句。
7.1 定义操作闭包
首先,我们定义一组操作闭包。
def create_operation(operation):
def perform(a, b):
return operation(a, b)
return perform
add = create_operation(lambda a, b: a + b)
subtract = create_operation(lambda a, b: a - b)
multiply = create_operation(lambda a, b: a * b)
divide = create_operation(lambda a, b: a / b if b != 0 else ValueError("Cannot divide by zero!"))
7.2 使用闭包执行操作
通过闭包,我们可以避免使用if-else语句来选择操作。
print(add(10, 5)) # 输出: 15
print(subtract(10, 5)) # 输出: 5
print(multiply(10, 5)) # 输出: 50
print(divide(10, 5)) # 输出: 2.0
八、使用类和方法
在面向对象编程中,我们可以使用类和方法来封装逻辑,并避免使用if-else语句。
8.1 定义操作类和方法
首先,我们定义一个操作基类和一些具体的操作类。
class Operation:
def execute(self, a, b):
raise NotImplementedError("This method should be overridden by subclasses")
class AddOperation(Operation):
def execute(self, a, b):
return a + b
class SubtractOperation(Operation):
def execute(self, a, b):
return a - b
class MultiplyOperation(Operation):
def execute(self, a, b):
return a * b
class DivideOperation(Operation):
def execute(self, a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
8.2 使用类和方法执行操作
通过使用类和方法,我们可以避免使用if-else语句来选择操作。
def perform_operation(operation, a, b):
return operation.execute(a, b)
add = AddOperation()
subtract = SubtractOperation()
multiply = MultiplyOperation()
divide = DivideOperation()
print(perform_operation(add, 10, 5)) # 输出: 15
print(perform_operation(subtract, 10, 5)) # 输出: 5
print(perform_operation(multiply, 10, 5)) # 输出: 50
print(perform_operation(divide, 10, 5)) # 输出: 2.0
九、使用数据驱动编程
数据驱动编程是一种通过数据来控制程序行为的编程方式。通过使用数据结构(如字典、列表等)来存储条件和操作,我们可以避免使用if-else语句。
9.1 定义操作数据
首先,我们定义一组操作数据。
operations = {
'add': lambda a, b: a + b,
'subtract': lambda a, b: a - b,
'multiply': lambda a, b: a * b,
'divide': lambda a, b: a / b if b != 0 else ValueError("Cannot divide by zero!")
}
9.2 使用数据驱动编程执行操作
通过数据驱动编程,我们可以避免使用if-else语句来选择操作。
def perform_operation(operation_name, a, b):
operation = operations.get(operation_name)
if operation is None:
raise ValueError(f"Unsupported operation: {operation_name}")
return operation(a, b)
print(perform_operation('add', 10, 5)) # 输出: 15
print(perform_operation('subtract', 10, 5)) # 输出: 5
print(perform_operation('multiply', 10, 5)) # 输出: 50
print(perform_operation('divide', 10, 5)) # 输出: 2.0
十、使用生成器
生成器是一种特殊的迭代器,可以逐步生成值。在Python中,生成器可以用来封装逻辑并避免使用if-else语句。
10.1 定义操作生成器
首先,我们定义一组操作生成器。
def add(a, b):
yield a + b
def subtract(a, b):
yield a - b
def multiply(a, b):
yield a * b
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero!")
yield a / b
10.2 使用生成器执行操作
通过生成器,我们可以避免使用if-else语句来选择操作。
def perform_operation(generator, a, b):
return next(generator(a, b))
print(perform_operation(add, 10, 5)) # 输出: 15
print(perform_operation(subtract, 10, 5)) # 输出: 5
print(perform_operation(multiply, 10, 5)) # 输出: 50
print(perform_operation(divide, 10, 5)) # 输出: 2.0
结论
通过利用多态、使用字典映射、使用策略模式、使用函数代替条件判断、使用装饰器模式、使用lambda表达式、使用闭包、使用类和方法、使用数据驱动编程以及使用生成器,我们可以在Python中有效地避免频繁使用if-else语句。这些方法不仅可以使代码更加简洁和可读,还能提高代码的可维护性和扩展性。选择合适的方法取决于具体的应用场景和需求。
相关问答FAQs:
如何在Python中使用替代方案来避免if-else结构?
在Python中,可以使用多种方法来替代if-else结构,比如利用字典来映射条件与结果,或者使用三元运算符来简化代码。使用字典可以实现条件映射,从而避免多个if-else的嵌套。例如,可以创建一个函数,通过字典查找相应的返回值,而不是一一判断条件。
使用Python的内置函数来避免if-else的逻辑有哪些?
Python提供了一些内置函数,如filter、map和reduce,可以在处理集合数据时避免使用if-else。通过这些函数,可以直接对数据进行操作和过滤,减少条件判断的需要。例如,使用filter可以筛选满足特定条件的元素,而不需要手动编写if语句。
在Python中,如何利用异常处理来替代if-else?
异常处理是另一种避免使用if-else的有效方式。当你预期某个操作可能会失败时,可以使用try-except语句来捕获异常并处理,而不需要一开始就进行条件判断。这种方式不仅能使代码更加简洁,还能提高代码的可读性和可维护性。
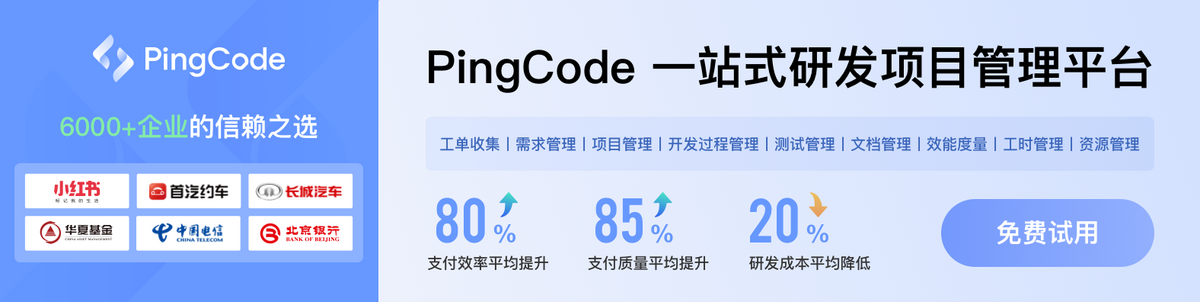