要抓取微信公众号文章,你需要掌握一些技术和工具,比如Python编程、微信公众平台接口、爬虫工具、数据解析技术等。具体来说,抓取微信公众号文章可以通过以下几种方式:使用微信公众号API接口、模拟微信公众号客户端请求、使用第三方爬虫工具。其中,使用微信公众号API接口是最为正规和可靠的方法。
使用微信公众号API接口是抓取微信公众号文章的正规途径。通过微信公众平台提供的开放接口,可以获取公众号文章的相关数据。你需要先申请微信公众平台的开发者账号,获取相应的接口权限。这样就可以通过API接口获取公众号的文章列表、内容等信息。具体步骤如下:
-
注册开发者账号并申请接口权限:首先,你需要注册一个微信公众平台的开发者账号,并申请相应的接口权限。可以在微信公众平台的管理后台进行操作。
-
获取Access Token:在调用微信公众平台提供的API接口之前,首先需要获取一个Access Token。Access Token是调用各接口的唯一凭证,可以通过API接口获取。具体代码如下:
import requests
def get_access_token(app_id, app_secret):
url = f'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={app_id}&secret={app_secret}'
response = requests.get(url)
data = response.json()
return data['access_token']
app_id = 'YOUR_APP_ID'
app_secret = 'YOUR_APP_SECRET'
access_token = get_access_token(app_id, app_secret)
- 获取公众号文章列表:有了Access Token之后,就可以调用微信公众平台提供的接口获取公众号文章列表。具体代码如下:
def get_articles_list(access_token, offset=0, count=10):
url = f'https://api.weixin.qq.com/cgi-bin/material/batchget_material?access_token={access_token}'
data = {
"type": "news",
"offset": offset,
"count": count
}
response = requests.post(url, json=data)
articles = response.json()
return articles
articles_list = get_articles_list(access_token)
print(articles_list)
- 解析文章内容:获取到文章列表后,就可以通过解析文章内容,提取有用的信息。一般来说,文章内容是以HTML格式存储的,可以使用BeautifulSoup等解析工具进行解析。具体代码如下:
from bs4 import BeautifulSoup
def parse_article_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('h1', class_='rich_media_title').text.strip()
author = soup.find('a', class_='rich_media_meta rich_media_meta_link rich_media_meta_nickname').text.strip()
content = soup.find('div', class_='rich_media_content').text.strip()
return {'title': title, 'author': author, 'content': content}
html_content = '<html_content_of_the_article>'
article_content = parse_article_content(html_content)
print(article_content)
通过以上步骤,你就可以使用Python抓取微信公众号文章了。下面是更详细的介绍:
一、注册开发者账号并申请接口权限
在抓取微信公众号文章之前,首先需要注册一个微信公众平台的开发者账号,并申请相应的接口权限。可以在微信公众平台的管理后台进行操作。具体步骤如下:
-
注册账号:访问微信公众平台(https://mp.weixin.qq.com/),点击“立即注册”,选择“公众号”,然后按照提示完成账号注册。
-
申请接口权限:登录微信公众平台管理后台,点击“开发”->“基本配置”,在“接口权限”栏目下申请相应的接口权限。一般来说,需要申请“获取素材列表”、“获取素材详情”等接口权限。
-
获取AppID和AppSecret:在“开发”->“基本配置”页面,可以看到该公众号的AppID和AppSecret。这两个参数在后续获取Access Token时会用到。
二、获取Access Token
在调用微信公众平台提供的API接口之前,首先需要获取一个Access Token。Access Token是调用各接口的唯一凭证,可以通过API接口获取。具体代码如下:
import requests
def get_access_token(app_id, app_secret):
url = f'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={app_id}&secret={app_secret}'
response = requests.get(url)
data = response.json()
return data['access_token']
app_id = 'YOUR_APP_ID'
app_secret = 'YOUR_APP_SECRET'
access_token = get_access_token(app_id, app_secret)
三、获取公众号文章列表
有了Access Token之后,就可以调用微信公众平台提供的接口获取公众号文章列表。具体代码如下:
def get_articles_list(access_token, offset=0, count=10):
url = f'https://api.weixin.qq.com/cgi-bin/material/batchget_material?access_token={access_token}'
data = {
"type": "news",
"offset": offset,
"count": count
}
response = requests.post(url, json=data)
articles = response.json()
return articles
articles_list = get_articles_list(access_token)
print(articles_list)
在获取公众号文章列表时,可以通过设置offset
和count
参数来指定获取文章的起始位置和数量。
四、解析文章内容
获取到文章列表后,就可以通过解析文章内容,提取有用的信息。一般来说,文章内容是以HTML格式存储的,可以使用BeautifulSoup等解析工具进行解析。具体代码如下:
from bs4 import BeautifulSoup
def parse_article_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('h1', class_='rich_media_title').text.strip()
author = soup.find('a', class_='rich_media_meta rich_media_meta_link rich_media_meta_nickname').text.strip()
content = soup.find('div', class_='rich_media_content').text.strip()
return {'title': title, 'author': author, 'content': content}
html_content = '<html_content_of_the_article>'
article_content = parse_article_content(html_content)
print(article_content)
在解析文章内容时,可以根据实际情况调整解析逻辑,以提取更多有用的信息。
五、存储和展示抓取到的文章
抓取到微信公众号文章后,可以将文章内容存储到数据库中,方便后续的展示和分析。常用的数据库有MySQL、MongoDB等。具体代码如下:
import pymysql
def save_article_to_db(article):
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor)
try:
with connection.cursor() as cursor:
sql = "INSERT INTO `articles` (`title`, `author`, `content`) VALUES (%s, %s, %s)"
cursor.execute(sql, (article['title'], article['author'], article['content']))
connection.commit()
finally:
connection.close()
article = {'title': 'example_title', 'author': 'example_author', 'content': 'example_content'}
save_article_to_db(article)
在展示抓取到的文章时,可以使用Django、Flask等Web框架开发一个简单的Web应用,将文章内容展示出来。具体代码如下:
from flask import Flask, render_template
import pymysql
app = Flask(__name__)
def get_articles_from_db():
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor)
try:
with connection.cursor() as cursor:
sql = "SELECT `title`, `author`, `content` FROM `articles`"
cursor.execute(sql)
articles = cursor.fetchall()
return articles
finally:
connection.close()
@app.route('/')
def index():
articles = get_articles_from_db()
return render_template('index.html', articles=articles)
if __name__ == '__main__':
app.run(debug=True)
在上述代码中,通过Flask框架开发了一个简单的Web应用,并从数据库中读取文章数据进行展示。可以根据实际需求对代码进行调整和优化。
六、常见问题及解决方案
在抓取微信公众号文章的过程中,可能会遇到一些常见问题,下面列举几个常见问题及解决方案:
-
接口权限不足:在调用微信公众平台API接口时,可能会遇到接口权限不足的问题。解决方案是确保已经申请了相应的接口权限,并检查接口权限是否在有效期内。
-
Access Token过期:Access Token有一定的有效期,过期后需要重新获取。解决方案是定期刷新Access Token,确保在调用API接口时使用的是有效的Access Token。
-
网络请求失败:在调用API接口时,可能会遇到网络请求失败的问题。解决方案是检查网络连接是否正常,确保API请求的URL和参数正确无误。
-
数据解析失败:在解析文章内容时,可能会遇到数据解析失败的问题。解决方案是检查HTML内容的结构,确保解析逻辑正确无误,可以使用调试工具辅助检查。
通过以上介绍的步骤和解决方案,可以有效地抓取微信公众号文章。需要注意的是,抓取微信公众号文章时应遵守相关法律法规,尊重原创内容的版权。
相关问答FAQs:
如何使用Python抓取微信公众号的文章内容?
要抓取微信公众号的文章内容,通常需要使用Python的爬虫库,如Requests和BeautifulSoup。首先,通过Requests库发送HTTP请求获取文章页面的HTML代码。然后,利用BeautifulSoup解析HTML并提取所需的信息,如标题、发布时间和正文内容。需要注意的是,某些公众号可能会对爬虫行为进行限制,因此可以考虑使用代理或模拟人类操作来降低被封的风险。
抓取公众号文章时需要注意哪些法律和道德问题?
在抓取公众号文章时,应遵循相关法律法规,确保不侵犯版权。尽量避免大规模抓取和复制他人的内容,建议在使用抓取的数据时进行适当的变更或引用。同时,尊重作者的创作权,尽量在使用或分享抓取内容时标明出处,保持良好的网络道德。
抓取公众号文章的效率如何提高?
要提高抓取公众号文章的效率,可以考虑使用多线程或异步编程来同时处理多个请求。这种方式能够显著提高数据抓取的速度。此外,合理设置请求的间隔时间,避免过于频繁的请求,可以减少被封禁的风险。同时,使用数据库或文件系统缓存已经抓取的数据,避免重复抓取相同内容,也能节省时间和资源。
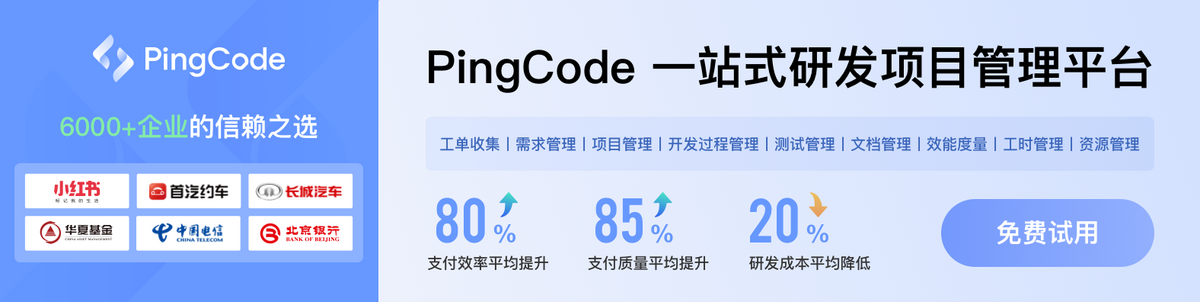