Python制作图纸库的方法包括:使用Python的绘图库(如Matplotlib、Plotly、Seaborn)、利用Pandas进行数据处理、结合Flask或Django创建Web应用、利用SQLAlchemy进行数据库操作。 其中,使用Python的绘图库是实现图纸库的关键,因为这些库提供了强大的绘图功能和丰富的可视化工具。
一、使用Python的绘图库
Python有多个强大的绘图库,下面将介绍一些常用的库及其基本使用方法。
1、Matplotlib
Matplotlib 是Python中最常用的绘图库之一,适用于创建静态、动态和交互式图表。以下是Matplotlib的基本使用步骤:
import matplotlib.pyplot as plt
创建数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 4, 5, 6]
创建图形
plt.plot(x, y)
添加标题和标签
plt.title("Simple Plot")
plt.xlabel("x-axis")
plt.ylabel("y-axis")
显示图形
plt.show()
2、Seaborn
Seaborn 是基于Matplotlib的高级绘图库,提供了更简洁的接口和更漂亮的默认样式。以下是Seaborn的基本使用步骤:
import seaborn as sns
import matplotlib.pyplot as plt
加载示例数据集
tips = sns.load_dataset("tips")
创建图形
sns.scatterplot(x="total_bill", y="tip", data=tips)
添加标题和标签
plt.title("Scatter Plot")
plt.xlabel("Total Bill")
plt.ylabel("Tip")
显示图形
plt.show()
3、Plotly
Plotly 是一个强大的绘图库,支持交互式图表,并且可以很方便地嵌入到Web应用中。以下是Plotly的基本使用步骤:
import plotly.express as px
创建示例数据
df = px.data.iris()
创建图形
fig = px.scatter(df, x="sepal_width", y="sepal_length", color="species")
显示图形
fig.show()
二、利用Pandas进行数据处理
Pandas 是Python中最常用的数据处理库,提供了强大的数据操作功能。以下是Pandas的基本使用步骤:
1、读取数据
import pandas as pd
读取CSV文件
df = pd.read_csv("data.csv")
显示前五行数据
print(df.head())
2、数据清洗
# 处理缺失值
df.fillna(0, inplace=True)
删除重复行
df.drop_duplicates(inplace=True)
3、数据分析
# 计算统计指标
mean_value = df['column_name'].mean()
sum_value = df['column_name'].sum()
分组汇总
grouped_df = df.groupby('group_column').sum()
三、结合Flask或Django创建Web应用
要将图纸库发布到Web上,可以使用Flask或Django创建Web应用。以下是Flask的基本使用步骤:
1、安装Flask
pip install Flask
2、创建Flask应用
from flask import Flask, render_template
import matplotlib.pyplot as plt
import io
import base64
app = Flask(__name__)
@app.route('/')
def index():
# 创建图形
plt.figure()
x = [1, 2, 3, 4, 5]
y = [2, 3, 4, 5, 6]
plt.plot(x, y)
# 保存图形到内存
img = io.BytesIO()
plt.savefig(img, format='png')
img.seek(0)
plot_url = base64.b64encode(img.getvalue()).decode()
return render_template('index.html', plot_url=plot_url)
if __name__ == '__main__':
app.run(debug=True)
3、创建HTML模板
在templates目录下创建index.html:
<!DOCTYPE html>
<html>
<head>
<title>Plot</title>
</head>
<body>
<h1>My Plot</h1>
<img src="data:image/png;base64,{{ plot_url }}">
</body>
</html>
四、利用SQLAlchemy进行数据库操作
SQLAlchemy 是Python的一个SQL工具包和对象关系映射(ORM)库,可以用于数据库操作。以下是SQLAlchemy的基本使用步骤:
1、安装SQLAlchemy
pip install SQLAlchemy
2、创建数据库连接
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
创建数据库引擎
engine = create_engine('sqlite:///database.db')
创建基类
Base = declarative_base()
创建会话
Session = sessionmaker(bind=engine)
session = Session()
3、定义数据模型
from sqlalchemy import Column, Integer, String
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
创建表
Base.metadata.create_all(engine)
4、数据增删改查
# 创建新用户
new_user = User(name='John', age=30)
session.add(new_user)
session.commit()
查询用户
user = session.query(User).filter_by(name='John').first()
print(user.name, user.age)
更新用户
user.age = 31
session.commit()
删除用户
session.delete(user)
session.commit()
五、综合示例:创建一个图纸库Web应用
以下是一个综合示例,展示了如何使用Flask、Matplotlib、Pandas和SQLAlchemy创建一个图纸库Web应用。
1、安装依赖
pip install Flask SQLAlchemy pandas matplotlib
2、创建Flask应用
from flask import Flask, render_template, request, redirect
import pandas as pd
import matplotlib.pyplot as plt
import io
import base64
from sqlalchemy import create_engine, Column, Integer, String, Float
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
app = Flask(__name__)
创建数据库引擎
engine = create_engine('sqlite:///database.db')
Base = declarative_base()
创建会话
Session = sessionmaker(bind=engine)
session = Session()
定义数据模型
class PlotData(Base):
__tablename__ = 'plot_data'
id = Column(Integer, primary_key=True)
name = Column(String)
x_values = Column(String)
y_values = Column(String)
创建表
Base.metadata.create_all(engine)
@app.route('/')
def index():
plots = session.query(PlotData).all()
return render_template('index.html', plots=plots)
@app.route('/add', methods=['GET', 'POST'])
def add():
if request.method == 'POST':
name = request.form['name']
x_values = request.form['x_values']
y_values = request.form['y_values']
new_plot = PlotData(name=name, x_values=x_values, y_values=y_values)
session.add(new_plot)
session.commit()
return redirect('/')
return render_template('add.html')
@app.route('/plot/<int:plot_id>')
def plot(plot_id):
plot_data = session.query(PlotData).filter_by(id=plot_id).first()
x = list(map(float, plot_data.x_values.split(',')))
y = list(map(float, plot_data.y_values.split(',')))
# 创建图形
plt.figure()
plt.plot(x, y)
# 保存图形到内存
img = io.BytesIO()
plt.savefig(img, format='png')
img.seek(0)
plot_url = base64.b64encode(img.getvalue()).decode()
return render_template('plot.html', plot_url=plot_url, name=plot_data.name)
if __name__ == '__main__':
app.run(debug=True)
3、创建HTML模板
在templates目录下创建index.html:
<!DOCTYPE html>
<html>
<head>
<title>Plot Library</title>
</head>
<body>
<h1>Plot Library</h1>
<a href="/add">Add New Plot</a>
<ul>
{% for plot in plots %}
<li><a href="/plot/{{ plot.id }}">{{ plot.name }}</a></li>
{% endfor %}
</ul>
</body>
</html>
在templates目录下创建add.html:
<!DOCTYPE html>
<html>
<head>
<title>Add Plot</title>
</head>
<body>
<h1>Add New Plot</h1>
<form method="post">
Name: <input type="text" name="name"><br>
X Values (comma separated): <input type="text" name="x_values"><br>
Y Values (comma separated): <input type="text" name="y_values"><br>
<input type="submit" value="Add">
</form>
<a href="/">Back to Plot Library</a>
</body>
</html>
在templates目录下创建plot.html:
<!DOCTYPE html>
<html>
<head>
<title>{{ name }}</title>
</head>
<body>
<h1>{{ name }}</h1>
<img src="data:image/png;base64,{{ plot_url }}">
<a href="/">Back to Plot Library</a>
</body>
</html>
结论
通过以上步骤,我们展示了如何使用Python的绘图库、数据处理库、Web框架和数据库操作库,来创建一个功能强大且易于扩展的图纸库。关键在于选择合适的库和工具,根据具体需求进行组合和优化。希望本文对你制作图纸库有所帮助。
相关问答FAQs:
如何在Python中创建一个图纸库?
要在Python中创建一个图纸库,您可以使用图形库如Matplotlib或Pygame来绘制各种图形。首先,您需要定义图纸的基本结构,例如图纸的尺寸和比例。接着,您可以编写函数来绘制不同的形状和图案,并将这些图纸保存为图像文件或PDF格式,以便后续使用和共享。
我该选择哪种库来制作图纸库?
根据您的需求,Matplotlib非常适合数据可视化和科学图形,而Pygame更适合创建游戏或交互式图形。如果您需要制作更复杂的图纸,考虑使用Cairo或Pillow等图形处理库。这些库提供了丰富的功能,可以帮助您实现多种绘图需求。
如何将制作的图纸导出为不同格式?
在Python中,您可以使用Matplotlib的savefig()
函数将图纸导出为PNG、JPEG、PDF等格式。对于其他库,如Pillow,您可以使用save()
方法轻松保存图像。此外,确保在导出前设置好图纸的分辨率和尺寸,以保证输出质量符合需求。
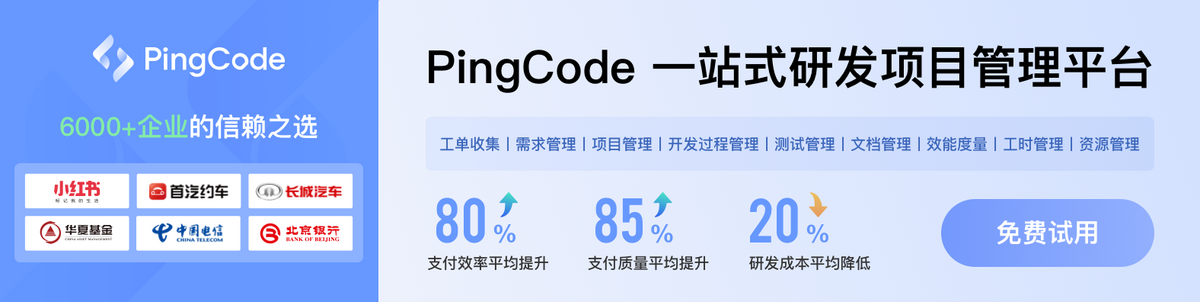