去除标点和数字的方法有多种,可以使用正则表达式、字符串方法、列表解析等。推荐使用正则表达式,因为它更简洁高效。
正则表达式方法:正则表达式是一种强大的工具,可以用来匹配和操作字符串中的特定模式。使用正则表达式可以很方便地去除标点符号和数字。以下是具体的步骤:
- 导入正则表达式模块:在Python中,正则表达式操作需要使用
re
模块。 - 编写正则表达式模式:标点符号和数字都可以通过正则表达式模式来匹配。
- 使用 re.sub() 函数:这个函数可以根据正则表达式模式替换字符串中的内容。
举个例子:
import re
text = "Hello, World! 123"
去除标点和数字
cleaned_text = re.sub(r'[^\w\s]', '', text)
cleaned_text = re.sub(r'\d+', '', cleaned_text)
print(cleaned_text)
在上面的代码中,[^\w\s]
的意思是匹配所有非字母数字和空白字符的内容,re.sub()
函数会把匹配到的内容替换成空字符串,从而去除标点符号。然后再次使用 re.sub(r'\d+', '', cleaned_text)
去除数字。
一、正则表达式去除标点和数字
1、导入正则表达式模块
在Python中,处理正则表达式需要导入 re
模块。使用 import re
语句导入正则表达式模块。
2、编写正则表达式模式
编写正则表达式模式以匹配标点符号和数字。标点符号可以通过 \W
来匹配,而数字可以通过 \d
来匹配。注意, \W
匹配所有非单词字符,包括标点符号和空格,为了精确匹配标点符号,需要使用 [^\w\s]
。
3、使用 re.sub() 函数
re.sub(pattern, repl, string)
函数用于替换字符串中所有匹配正则表达式模式的内容。 pattern
是正则表达式模式, repl
是替换的内容, string
是要处理的字符串。
import re
def remove_punctuation_and_digits(text):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 去除数字
text = re.sub(r'\d+', '', text)
return text
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
二、字符串方法去除标点和数字
1、使用 str.translate() 方法
str.translate()
方法可以通过映射表替换字符串中的字符。首先,创建一个映射表,将标点符号和数字映射为空字符串,然后使用 str.translate()
方法进行替换。
import string
def remove_punctuation_and_digits(text):
# 创建映射表
translator = str.maketrans('', '', string.punctuation + string.digits)
return text.translate(translator)
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
2、使用 str.replace() 方法
str.replace(old, new)
方法可以替换字符串中的旧内容为新内容。可以多次调用 str.replace()
方法来替换标点符号和数字。
def remove_punctuation_and_digits(text):
# 替换标点符号
for char in string.punctuation:
text = text.replace(char, '')
# 替换数字
for digit in string.digits:
text = text.replace(digit, '')
return text
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
三、列表解析去除标点和数字
1、使用列表解析和 join() 方法
可以使用列表解析来过滤掉标点符号和数字,然后使用 join()
方法将过滤后的字符连接成字符串。
import string
def remove_punctuation_and_digits(text):
return ''.join([char for char in text if char not in string.punctuation and not char.isdigit()])
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
2、使用 filter() 函数
filter(function, iterable)
函数可以过滤掉不符合条件的元素。可以使用 filter()
函数和 str.isalnum()
方法来去除标点符号和数字。
def remove_punctuation_and_digits(text):
return ''.join(filter(lambda char: char.isalnum() or char.isspace(), text))
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
四、综合应用场景
在实际应用中,去除标点符号和数字的需求可能会与其他文本处理需求结合在一起。以下是一些综合应用场景的例子。
1、去除标点符号、数字和多余空白
在一些文本处理中,除了去除标点符号和数字,还需要去除多余的空白字符。
import re
def clean_text(text):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 去除数字
text = re.sub(r'\d+', '', text)
# 去除多余空白
text = ' '.join(text.split())
return text
text = "Hello, World! 123"
cleaned_text = clean_text(text)
print(cleaned_text)
2、去除标点符号、数字和停用词
在自然语言处理(NLP)中,停用词(如 "the", "is", "in" 等)通常对文本分析没有贡献,因此需要去除。
import re
from nltk.corpus import stopwords
def clean_text(text):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 去除数字
text = re.sub(r'\d+', '', text)
# 去除多余空白
text = ' '.join(text.split())
# 去除停用词
stop_words = set(stopwords.words('english'))
text = ' '.join([word for word in text.split() if word.lower() not in stop_words])
return text
text = "This is a sample text with numbers 123 and punctuation!"
cleaned_text = clean_text(text)
print(cleaned_text)
3、去除标点符号、数字和特定字符
在某些情况下,除了标点符号和数字,还需要去除特定的字符。
import re
def clean_text(text, chars_to_remove):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 去除数字
text = re.sub(r'\d+', '', text)
# 去除多余空白
text = ' '.join(text.split())
# 去除特定字符
for char in chars_to_remove:
text = text.replace(char, '')
return text
text = "Sample text with special characters *&^%$#@! and numbers 123."
chars_to_remove = "*&^%$#@!"
cleaned_text = clean_text(text, chars_to_remove)
print(cleaned_text)
五、提高代码性能
在处理大量文本数据时,代码性能可能会成为一个关键问题。以下是一些提高代码性能的建议。
1、使用 compile() 函数
re.compile(pattern)
函数可以预编译正则表达式模式,从而提高多次匹配的性能。
import re
def remove_punctuation_and_digits(text):
# 预编译正则表达式模式
pattern_punctuation = re.compile(r'[^\w\s]')
pattern_digits = re.compile(r'\d+')
# 去除标点符号
text = pattern_punctuation.sub('', text)
# 去除数字
text = pattern_digits.sub('', text)
return text
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
2、使用生成器表达式
生成器表达式比列表解析更高效,因为它们不会一次性创建整个列表,而是按需生成元素。
import string
def remove_punctuation_and_digits(text):
return ''.join(char for char in text if char not in string.punctuation and not char.isdigit())
text = "Hello, World! 123"
cleaned_text = remove_punctuation_and_digits(text)
print(cleaned_text)
3、批量处理文本
在处理大量文本数据时,可以将文本批量处理,以减少函数调用的开销。
import re
def remove_punctuation_and_digits_batch(texts):
pattern_punctuation = re.compile(r'[^\w\s]')
pattern_digits = re.compile(r'\d+')
cleaned_texts = []
for text in texts:
text = pattern_punctuation.sub('', text)
text = pattern_digits.sub('', text)
cleaned_texts.append(text)
return cleaned_texts
texts = ["Hello, World! 123", "Sample text with numbers 456."]
cleaned_texts = remove_punctuation_and_digits_batch(texts)
print(cleaned_texts)
六、应用实例
以下是一些实际应用实例,展示如何在不同场景下使用去除标点符号和数字的方法。
1、文本预处理
在自然语言处理(NLP)任务中,文本预处理是一个重要步骤。去除标点符号和数字可以提高模型的性能。
import re
from nltk.corpus import stopwords
def preprocess_text(text):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 去除数字
text = re.sub(r'\d+', '', text)
# 去除多余空白
text = ' '.join(text.split())
# 去除停用词
stop_words = set(stopwords.words('english'))
text = ' '.join([word for word in text.split() if word.lower() not in stop_words])
return text
text = "This is a sample text with numbers 123 and punctuation!"
preprocessed_text = preprocess_text(text)
print(preprocessed_text)
2、网页数据清洗
在网页数据清洗中,去除标点符号和数字可以帮助提取有用的信息。
import re
import requests
from bs4 import BeautifulSoup
def clean_webpage_content(url):
# 获取网页内容
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
# 去除标点符号和数字
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
# 去除多余空白
text = ' '.join(text.split())
return text
url = "https://www.example.com"
cleaned_content = clean_webpage_content(url)
print(cleaned_content)
3、日志文件处理
在日志文件处理中,去除标点符号和数字可以帮助分析日志内容。
import re
def clean_log_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
cleaned_lines = []
for line in lines:
# 去除标点符号和数字
line = re.sub(r'[^\w\s]', '', line)
line = re.sub(r'\d+', '', line)
# 去除多余空白
line = ' '.join(line.split())
cleaned_lines.append(line)
return cleaned_lines
file_path = "logfile.txt"
cleaned_log = clean_log_file(file_path)
print(cleaned_log)
七、总结
去除标点和数字在文本处理中是一个常见的需求,可以通过多种方法来实现。使用正则表达式是最简洁高效的方法,但在某些情况下,字符串方法和列表解析也可以提供灵活的解决方案。在处理大量文本数据时,可以考虑提高代码性能的方法,如使用预编译正则表达式模式、生成器表达式和批量处理。综合应用场景和实际应用实例展示了去除标点和数字的多种用法,希望对读者有所帮助。
相关问答FAQs:
如何在Python中去除字符串中的标点符号和数字?
要在Python中去除字符串中的标点和数字,可以使用str.isalpha()
方法结合列表推导式。示例代码如下:
import string
def remove_punctuation_and_digits(text):
return ''.join(char for char in text if char.isalpha() or char.isspace())
sample_text = "Hello, World! 2023 is amazing."
cleaned_text = remove_punctuation_and_digits(sample_text)
print(cleaned_text) # 输出: Hello World is amazing
这种方法会保留字母和空格,同时删除所有标点和数字。
使用正则表达式去除标点和数字的简单方法是什么?
正则表达式是处理字符串的强大工具。在Python中,可以使用re
模块来轻松去除标点和数字。以下是一个示例:
import re
def clean_text(text):
return re.sub(r'[^a-zA-Z\s]', '', text)
sample_text = "Hello, World! 2023 is amazing."
cleaned_text = clean_text(sample_text)
print(cleaned_text) # 输出: Hello World is amazing
这个方法通过匹配非字母和空格的字符并将其替换为空字符串,达到去除效果。
在处理文本数据时,去除标点和数字有什么好处?
去除标点和数字可以帮助在文本分析和自然语言处理任务中提高数据的质量。它能够减少噪音,使模型更专注于字词的含义,进而提升文本分类、情感分析等任务的准确率。通过清理数据,分析结果更具一致性和可靠性。
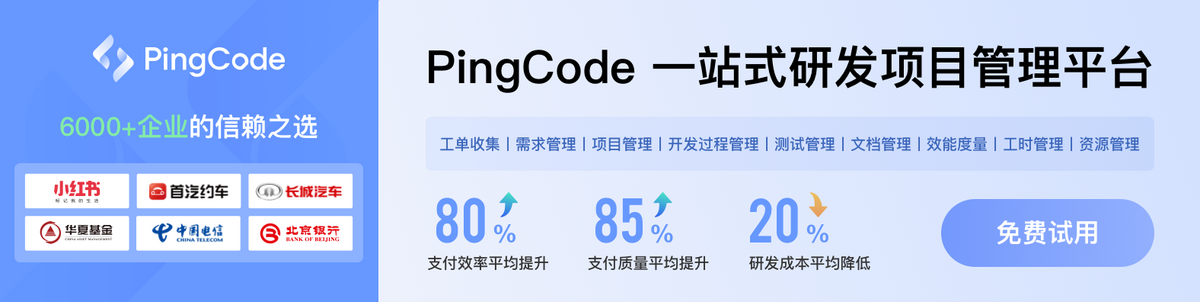