要在Python中输出文件路径,可以使用多种方法,包括使用os模块、使用Pathlib模块、获取当前工作目录、获取脚本所在目录、获取文件的绝对路径等。我们可以使用os模块中的os.path或者新推出的Pathlib模块,这两个模块提供了丰富的方法来处理文件路径。下面将详细介绍其中的一个方法,并在后续部分详细介绍所有这些方法。
其中,使用Pathlib模块是一个非常推荐的方法。Pathlib模块提供了面向对象的路径操作方式,代码简洁且功能强大。通过Pathlib,我们可以轻松获取文件的绝对路径、父目录等信息。以下是一个简单的例子:
from pathlib import Path
获取当前文件的路径
current_file = Path(__file__)
获取文件的绝对路径
absolute_path = current_file.resolve()
print(f"文件的绝对路径是: {absolute_path}")
这个例子中,首先导入了Pathlib模块,然后通过Path(file)获取当前文件的路径,再通过resolve()方法获取该文件的绝对路径,最后输出这个路径。
接下来,我们将详细介绍如何使用这些方法来输出文件路径。
一、使用os模块
1、获取当前工作目录
在Python中,可以使用os模块的os.getcwd()
函数获取当前工作目录的路径。当前工作目录是指Python脚本执行时所在的目录。
import os
获取当前工作目录
current_working_directory = os.getcwd()
print(f"当前工作目录是: {current_working_directory}")
2、获取脚本所在目录
有时候,我们需要获取脚本文件所在的目录,可以使用os.path.dirname()
和__file__
变量来实现。
import os
获取脚本文件的路径
script_path = os.path.dirname(os.path.abspath(__file__))
print(f"脚本文件所在目录是: {script_path}")
3、获取文件的绝对路径
要获取文件的绝对路径,可以使用os.path.abspath()
函数。这个函数可以将相对路径转换为绝对路径。
import os
获取文件的相对路径
relative_path = "example.txt"
获取文件的绝对路径
absolute_path = os.path.abspath(relative_path)
print(f"文件的绝对路径是: {absolute_path}")
4、拼接路径
在处理文件路径时,常常需要拼接多个路径,这可以使用os.path.join()
函数来实现。
import os
定义文件夹和文件名
folder = "documents"
file_name = "example.txt"
拼接路径
full_path = os.path.join(folder, file_name)
print(f"拼接后的路径是: {full_path}")
二、使用Pathlib模块
1、获取当前工作目录
Pathlib模块中的Path类提供了cwd()
方法来获取当前工作目录。
from pathlib import Path
获取当前工作目录
current_working_directory = Path.cwd()
print(f"当前工作目录是: {current_working_directory}")
2、获取脚本所在目录
使用Pathlib模块,可以通过Path(__file__).parent
来获取脚本文件所在的目录。
from pathlib import Path
获取脚本文件所在目录
script_path = Path(__file__).parent
print(f"脚本文件所在目录是: {script_path}")
3、获取文件的绝对路径
Pathlib模块中的resolve()
方法可以获取文件的绝对路径。
from pathlib import Path
获取文件的相对路径
relative_path = Path("example.txt")
获取文件的绝对路径
absolute_path = relative_path.resolve()
print(f"文件的绝对路径是: {absolute_path}")
4、拼接路径
Pathlib模块中的/
运算符可以用于拼接路径,这使得代码更加简洁。
from pathlib import Path
定义文件夹和文件名
folder = Path("documents")
file_name = "example.txt"
拼接路径
full_path = folder / file_name
print(f"拼接后的路径是: {full_path}")
5、更多Pathlib功能
Pathlib模块还提供了许多其他有用的方法,比如判断路径是否存在、创建目录等。
from pathlib import Path
判断路径是否存在
path = Path("example.txt")
if path.exists():
print(f"路径 {path} 存在")
else:
print(f"路径 {path} 不存在")
创建目录
new_folder = Path("new_folder")
new_folder.mkdir(exist_ok=True)
print(f"目录 {new_folder} 已创建")
三、获取当前工作目录
在Python中获取当前工作目录非常重要,因为它是文件操作的基准目录。无论是读取文件还是写入文件,都需要知道当前工作目录。
import os
获取当前工作目录
current_working_directory = os.getcwd()
print(f"当前工作目录是: {current_working_directory}")
或者使用Pathlib模块:
from pathlib import Path
获取当前工作目录
current_working_directory = Path.cwd()
print(f"当前工作目录是: {current_working_directory}")
四、获取脚本所在目录
有时候,我们需要知道脚本文件所在的目录。这在处理相对路径时尤其有用,可以避免因为工作目录不同而导致的路径问题。
import os
获取脚本文件的路径
script_path = os.path.dirname(os.path.abspath(__file__))
print(f"脚本文件所在目录是: {script_path}")
或者使用Pathlib模块:
from pathlib import Path
获取脚本文件所在目录
script_path = Path(__file__).parent
print(f"脚本文件所在目录是: {script_path}")
五、获取文件的绝对路径
获取文件的绝对路径可以确保文件操作的路径是正确的,避免因为相对路径导致的问题。
import os
获取文件的相对路径
relative_path = "example.txt"
获取文件的绝对路径
absolute_path = os.path.abspath(relative_path)
print(f"文件的绝对路径是: {absolute_path}")
或者使用Pathlib模块:
from pathlib import Path
获取文件的相对路径
relative_path = Path("example.txt")
获取文件的绝对路径
absolute_path = relative_path.resolve()
print(f"文件的绝对路径是: {absolute_path}")
六、拼接路径
在处理文件路径时,经常需要拼接多个路径。这可以通过os.path.join()
函数或者Pathlib模块中的/
运算符来实现。
import os
定义文件夹和文件名
folder = "documents"
file_name = "example.txt"
拼接路径
full_path = os.path.join(folder, file_name)
print(f"拼接后的路径是: {full_path}")
或者使用Pathlib模块:
from pathlib import Path
定义文件夹和文件名
folder = Path("documents")
file_name = "example.txt"
拼接路径
full_path = folder / file_name
print(f"拼接后的路径是: {full_path}")
七、判断路径是否存在
在进行文件操作前,通常需要判断路径是否存在。这可以避免因路径不存在而导致的错误。
import os
判断路径是否存在
path = "example.txt"
if os.path.exists(path):
print(f"路径 {path} 存在")
else:
print(f"路径 {path} 不存在")
或者使用Pathlib模块:
from pathlib import Path
判断路径是否存在
path = Path("example.txt")
if path.exists():
print(f"路径 {path} 存在")
else:
print(f"路径 {path} 不存在")
八、创建目录
在处理文件操作时,有时候需要创建目录。可以使用os.makedirs()
或者Pathlib模块中的mkdir()
方法。
import os
创建目录
new_folder = "new_folder"
os.makedirs(new_folder, exist_ok=True)
print(f"目录 {new_folder} 已创建")
或者使用Pathlib模块:
from pathlib import Path
创建目录
new_folder = Path("new_folder")
new_folder.mkdir(exist_ok=True)
print(f"目录 {new_folder} 已创建")
九、处理文件路径的其他方法
1、获取文件名和扩展名
可以使用os.path.splitext()
函数来获取文件名和扩展名。
import os
获取文件名和扩展名
file_path = "example.txt"
file_name, file_extension = os.path.splitext(file_path)
print(f"文件名: {file_name}, 扩展名: {file_extension}")
或者使用Pathlib模块:
from pathlib import Path
获取文件名和扩展名
file_path = Path("example.txt")
file_name = file_path.stem
file_extension = file_path.suffix
print(f"文件名: {file_name}, 扩展名: {file_extension}")
2、获取父目录
可以使用os.path.dirname()
函数或者Pathlib模块中的parent
属性来获取文件的父目录。
import os
获取文件的父目录
file_path = "folder/example.txt"
parent_directory = os.path.dirname(file_path)
print(f"父目录是: {parent_directory}")
或者使用Pathlib模块:
from pathlib import Path
获取文件的父目录
file_path = Path("folder/example.txt")
parent_directory = file_path.parent
print(f"父目录是: {parent_directory}")
3、遍历目录
可以使用os.listdir()
函数或者Pathlib模块中的iterdir()
方法来遍历目录中的文件和子目录。
import os
遍历目录
directory = "folder"
for item in os.listdir(directory):
print(item)
或者使用Pathlib模块:
from pathlib import Path
遍历目录
directory = Path("folder")
for item in directory.iterdir():
print(item)
4、获取文件大小
可以使用os.path.getsize()
函数或者Pathlib模块中的stat()
方法来获取文件大小。
import os
获取文件大小
file_path = "example.txt"
file_size = os.path.getsize(file_path)
print(f"文件大小是: {file_size} 字节")
或者使用Pathlib模块:
from pathlib import Path
获取文件大小
file_path = Path("example.txt")
file_size = file_path.stat().st_size
print(f"文件大小是: {file_size} 字节")
5、复制文件
可以使用shutil
模块中的copyfile()
函数来复制文件。
import shutil
复制文件
src = "example.txt"
dst = "copy_example.txt"
shutil.copyfile(src, dst)
print(f"文件已复制到: {dst}")
或者使用Pathlib模块中的shutil
集成方法:
from pathlib import Path
import shutil
复制文件
src = Path("example.txt")
dst = Path("copy_example.txt")
shutil.copyfile(src, dst)
print(f"文件已复制到: {dst}")
6、删除文件
可以使用os.remove()
函数或者Pathlib模块中的unlink()
方法来删除文件。
import os
删除文件
file_path = "example.txt"
os.remove(file_path)
print(f"文件 {file_path} 已删除")
或者使用Pathlib模块:
from pathlib import Path
删除文件
file_path = Path("example.txt")
file_path.unlink()
print(f"文件 {file_path} 已删除")
7、重命名文件
可以使用os.rename()
函数或者Pathlib模块中的rename()
方法来重命名文件。
import os
重命名文件
old_name = "example.txt"
new_name = "renamed_example.txt"
os.rename(old_name, new_name)
print(f"文件已重命名为: {new_name}")
或者使用Pathlib模块:
from pathlib import Path
重命名文件
old_name = Path("example.txt")
new_name = Path("renamed_example.txt")
old_name.rename(new_name)
print(f"文件已重命名为: {new_name}")
8、移动文件
可以使用shutil
模块中的move()
函数来移动文件。
import shutil
移动文件
src = "example.txt"
dst = "moved_example.txt"
shutil.move(src, dst)
print(f"文件已移动到: {dst}")
或者使用Pathlib模块中的shutil
集成方法:
from pathlib import Path
import shutil
移动文件
src = Path("example.txt")
dst = Path("moved_example.txt")
shutil.move(src, dst)
print(f"文件已移动到: {dst}")
9、列出目录中的所有文件
可以使用os.walk()
函数来递归地列出目录中的所有文件。
import os
列出目录中的所有文件
directory = "folder"
for root, dirs, files in os.walk(directory):
for file in files:
print(os.path.join(root, file))
或者使用Pathlib模块的rglob()
方法:
from pathlib import Path
列出目录中的所有文件
directory = Path("folder")
for file in directory.rglob('*'):
print(file)
10、文件路径的其他操作
除了以上常见操作,文件路径处理还有许多其他方法,比如获取文件的创建时间、修改时间等。
import os
import time
获取文件的创建时间
file_path = "example.txt"
creation_time = os.path.getctime(file_path)
print(f"文件创建时间是: {time.ctime(creation_time)}")
获取文件的修改时间
modification_time = os.path.getmtime(file_path)
print(f"文件修改时间是: {time.ctime(modification_time)}")
或者使用Pathlib模块:
from pathlib import Path
获取文件的创建时间
file_path = Path("example.txt")
creation_time = file_path.stat().st_ctime
print(f"文件创建时间是: {time.ctime(creation_time)}")
获取文件的修改时间
modification_time = file_path.stat().st_mtime
print(f"文件修改时间是: {time.ctime(modification_time)}")
11、读取和写入文件
读取和写入文件是文件操作中最基本的功能之一。可以使用内置的open()
函数来实现。
# 写入文件
with open("example.txt", "w") as file:
file.write("Hello, World!")
读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
或者使用Pathlib模块中的write_text()
和read_text()
方法:
from pathlib import Path
写入文件
file_path = Path("example.txt")
file_path.write_text("Hello, World!")
读取文件
content = file_path.read_text()
print(content)
总结
在Python中处理文件路径有多种方法,可以根据具体需求选择合适的方式。使用os模块和Pathlib模块是处理文件路径的常见方式,其中Pathlib模块提供了更加面向对象的路径操作方式,使代码更加简洁和可读。通过掌握以上介绍的方法和技巧,可以在实际项目中更加高效地处理文件路径。
相关问答FAQs:
如何在Python中获取当前工作目录?
在Python中,可以使用os
模块的getcwd()
函数来获取当前工作目录。示例代码如下:
import os
current_directory = os.getcwd()
print("当前工作目录是:", current_directory)
这段代码将输出当前脚本所在的目录路径。
如何输出特定文件的绝对路径?
要获取特定文件的绝对路径,可以使用os.path.abspath()
函数。示例代码如下:
import os
file_name = "example.txt"
absolute_path = os.path.abspath(file_name)
print("文件的绝对路径是:", absolute_path)
这段代码将返回example.txt
文件的绝对路径,前提是该文件存在于当前工作目录中。
如何列出指定目录中的所有文件及其路径?
若想列出某个目录中的所有文件及其路径,可以使用os.listdir()
和os.path.join()
组合。示例代码如下:
import os
directory = "/path/to/directory"
for file_name in os.listdir(directory):
full_path = os.path.join(directory, file_name)
print("文件路径:", full_path)
这段代码将遍历指定目录中的所有文件,并输出每个文件的完整路径。确保将"/path/to/directory"
替换为实际的目录路径。
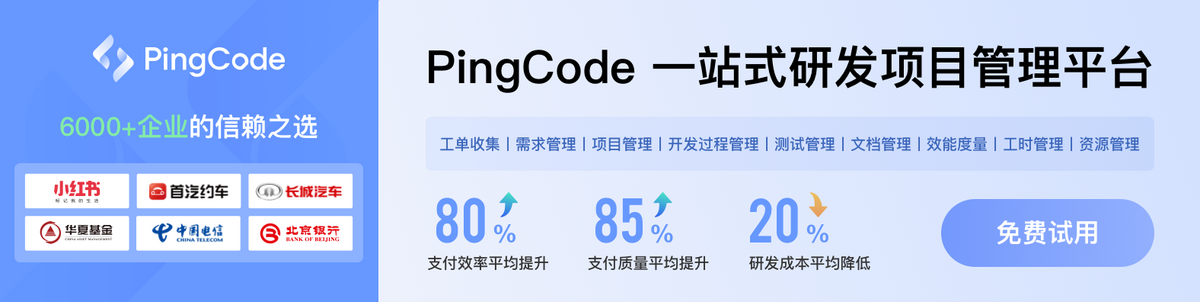