使用Python制作自动考勤的方法包括:使用OpenCV进行人脸识别、使用Pandas处理数据、使用SQLite或其他数据库存储考勤数据。 其中,使用OpenCV进行人脸识别是实现自动考勤的关键步骤。具体来说,可以通过以下步骤来实现人脸识别考勤系统:首先,使用OpenCV库捕获实时视频流并检测人脸,然后使用预训练的人脸识别模型来识别员工的身份,最后将识别结果与考勤数据进行匹配并记录到数据库中。
以下是详细的实现方法:
一、安装必要的库和工具
在开始编写代码之前,需要安装一些必要的库和工具。可以使用pip来安装这些库:
pip install opencv-python
pip install numpy
pip install pandas
pip install sqlite3
pip install face_recognition
二、获取和处理视频流
- 捕获视频流
import cv2
def capture_video():
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
这段代码使用OpenCV捕获视频流并显示在窗口中。按下“q”键可以退出视频流。
- 检测人脸
import cv2
def detect_faces(frame):
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2)
return frame
这段代码使用OpenCV的预训练人脸检测模型来检测视频帧中的人脸,并在检测到的人脸周围绘制矩形框。
三、人脸识别
- 加载已知人脸
import face_recognition
def load_known_faces():
known_face_encodings = []
known_face_names = []
# 加载已知人脸图像
image = face_recognition.load_image_file("known_person.jpg")
encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(encoding)
known_face_names.append("Known Person Name")
return known_face_encodings, known_face_names
这段代码加载已知员工的人脸图像,并计算其人脸编码。
- 识别人脸
def recognize_faces(frame, known_face_encodings, known_face_names):
rgb_frame = frame[:, :, ::-1]
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings):
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
cv2.putText(frame, name, (left + 6, bottom - 6), cv2.FONT_HERSHEY_DUPLEX, 0.5, (255, 255, 255), 1)
return frame
这段代码使用face_recognition库识别视频帧中的人脸,并绘制姓名标签。
四、处理和存储考勤数据
- 创建考勤数据库
import sqlite3
def create_database():
conn = sqlite3.connect('attendance.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS attendance
(id INTEGER PRIMARY KEY, name TEXT, date TEXT, time TEXT)''')
conn.commit()
conn.close()
这段代码创建一个SQLite数据库,并在其中创建一个考勤表。
- 记录考勤数据
import datetime
def record_attendance(name):
conn = sqlite3.connect('attendance.db')
cursor = conn.cursor()
now = datetime.datetime.now()
date = now.strftime("%Y-%m-%d")
time = now.strftime("%H:%M:%S")
cursor.execute("INSERT INTO attendance (name, date, time) VALUES (?, ?, ?)", (name, date, time))
conn.commit()
conn.close()
这段代码将识别到的员工姓名、日期和时间记录到考勤数据库中。
五、整合代码
import cv2
import face_recognition
import sqlite3
import datetime
def capture_video():
known_face_encodings, known_face_names = load_known_faces()
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
frame = recognize_faces(frame, known_face_encodings, known_face_names)
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
def load_known_faces():
known_face_encodings = []
known_face_names = []
image = face_recognition.load_image_file("known_person.jpg")
encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(encoding)
known_face_names.append("Known Person Name")
return known_face_encodings, known_face_names
def recognize_faces(frame, known_face_encodings, known_face_names):
rgb_frame = frame[:, :, ::-1]
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings):
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
if True in matches:
first_match_index = matches.index(True)
name = known_face_names[first_match_index]
record_attendance(name)
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
cv2.putText(frame, name, (left + 6, bottom - 6), cv2.FONT_HERSHEY_DUPLEX, 0.5, (255, 255, 255), 1)
return frame
def create_database():
conn = sqlite3.connect('attendance.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS attendance
(id INTEGER PRIMARY KEY, name TEXT, date TEXT, time TEXT)''')
conn.commit()
conn.close()
def record_attendance(name):
conn = sqlite3.connect('attendance.db')
cursor = conn.cursor()
now = datetime.datetime.now()
date = now.strftime("%Y-%m-%d")
time = now.strftime("%H:%M:%S")
cursor.execute("INSERT INTO attendance (name, date, time) VALUES (?, ?, ?)", (name, date, time))
conn.commit()
conn.close()
if __name__ == "__main__":
create_database()
capture_video()
六、进一步优化
-
优化人脸识别模型
可以使用更先进的深度学习模型来提高人脸识别的准确性。例如,使用Dlib库的ResNet模型。
-
处理多个已知人脸
可以将多个已知员工的人脸图像和编码存储在数据库中,并在识别时加载这些数据。
-
改进用户界面
可以使用Tkinter或PyQt等GUI库来创建更友好的用户界面,包括显示识别结果和考勤记录等。
-
集成其他功能
可以集成如邮件通知、报表生成等功能,进一步提高系统的实用性和便捷性。
七、总结
通过上述步骤,我们使用Python实现了一个简单的自动考勤系统。这个系统使用OpenCV进行人脸检测,face_recognition库进行人脸识别,并将考勤数据记录到SQLite数据库中。通过进一步优化和扩展,这个系统可以应用于实际的考勤管理中。
相关问答FAQs:
如何用Python创建一个自动考勤系统的基本步骤是什么?
要创建一个自动考勤系统,您需要明确几个基本步骤。首先,选择一个合适的数据库来存储考勤记录,例如SQLite或MySQL。接着,使用Python的Flask或Django框架搭建一个简单的Web应用,以便用户可以方便地访问系统。此外,您还需要编写代码来处理用户的打卡时间、计算出勤天数,以及生成考勤报告。最后,确保系统具有用户友好的界面,以便于使用。
使用Python制作考勤系统需要哪些库或工具?
开发自动考勤系统时,您可以使用多个Python库来提高效率。例如,Pandas库可以用于数据处理和分析,NumPy则可以帮助进行数学计算。Flask或Django是流行的Web框架,可用于构建前端和后端。对于数据存储,您可以考虑使用SQLAlchemy与数据库交互。图形化界面方面,可以使用Tkinter或PyQt来创建桌面应用程序。
如何确保自动考勤系统的安全性和数据隐私?
在创建自动考勤系统时,确保用户数据的安全性至关重要。您可以通过使用HTTPS加密通讯来保护数据传输过程。对于存储在数据库中的信息,确保使用适当的加密算法和访问控制。此外,定期进行安全审计和漏洞检测可以帮助及时发现潜在问题,保护用户的隐私和数据安全。
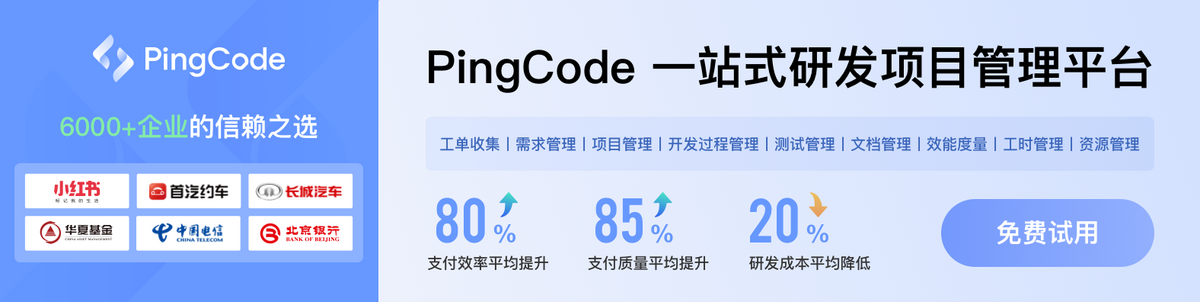