在Python中区别大小写可以使用多种方法,包括字符串方法、正则表达式、以及手动比较等。常用的方法有:使用字符串方法如.lower()
、.upper()
、.casefold()
和str.islower()
、str.isupper()
等,以及通过正则表达式进行匹配。其中,使用字符串方法是最简单且直观的方式。
例如,通过调用字符串的.lower()
方法,可以将字符串中的所有字符转换为小写,然后与原始字符串进行比较,来判断原始字符串是否区分大小写。这种方法非常适合用于简单的大小写比较。
下面将详细介绍如何在Python中通过不同方法实现对大小写的区分。
一、字符串方法
1、str.lower()
和 str.upper()
str.lower()
方法将字符串中的所有字符转换为小写,而str.upper()
方法则将字符串中的所有字符转换为大写。通过这些方法,可以轻松实现对大小写的区分。
string = "Hello World"
lower_string = string.lower()
upper_string = string.upper()
print(lower_string) # 输出: "hello world"
print(upper_string) # 输出: "HELLO WORLD"
在实际应用中,可以使用这些方法进行比较:
string1 = "Hello"
string2 = "HELLO"
if string1.lower() == string2.lower():
print("The strings are the same when case is ignored.")
else:
print("The strings are different.")
2、str.islower()
和 str.isupper()
str.islower()
方法用于判断字符串中的所有字母是否都是小写,而str.isupper()
方法则用于判断字符串中的所有字母是否都是大写。
string1 = "hello"
string2 = "HELLO"
print(string1.islower()) # 输出: True
print(string2.isupper()) # 输出: True
这些方法非常适合用于需要判断字符串整体是否为某种大小写的情况。
3、str.casefold()
str.casefold()
方法是str.lower()
的一个更为强大的版本,它用于忽略大小写进行比较时,能够更好地处理一些特殊字符。
string1 = "straße"
string2 = "STRASSE"
if string1.casefold() == string2.casefold():
print("The strings are the same when case is ignored.")
else:
print("The strings are different.")
二、正则表达式
正则表达式提供了强大的字符串匹配和处理功能。在Python中,可以使用re
模块来处理正则表达式。
1、基本用法
re
模块中的re.IGNORECASE
标志(或re.I
)用于忽略大小写进行匹配。
import re
pattern = r"hello"
string = "Hello World"
if re.search(pattern, string, re.IGNORECASE):
print("Pattern found")
else:
print("Pattern not found")
2、复杂匹配
正则表达式还可以用于复杂的字符串匹配和替换操作。
import re
pattern = r"hello"
string = "Hello World, hello universe"
matches = re.findall(pattern, string, re.IGNORECASE)
print(matches) # 输出: ['Hello', 'hello']
三、手动比较
有时,手动比较字符串的每个字符也可以实现对大小写的区分。这种方法虽然不如前两种方法简洁,但在某些特定情况下可能会更灵活。
1、逐字符比较
可以通过遍历字符串的每个字符进行比较。
string1 = "Hello"
string2 = "HELLO"
def case_insensitive_compare(str1, str2):
if len(str1) != len(str2):
return False
for char1, char2 in zip(str1, str2):
if char1.lower() != char2.lower():
return False
return True
print(case_insensitive_compare(string1, string2)) # 输出: True
2、使用ASCII值比较
也可以通过比较字符的ASCII值来实现。
def case_insensitive_compare(str1, str2):
if len(str1) != len(str2):
return False
for char1, char2 in zip(str1, str2):
if ord(char1.lower()) != ord(char2.lower()):
return False
return True
print(case_insensitive_compare(string1, string2)) # 输出: True
四、综合应用
在实际应用中,可能需要综合使用上述方法来处理字符串的大小写区分。例如,在处理用户输入时,常常需要忽略大小写进行匹配和验证。
1、用户输入验证
在处理用户输入时,可以忽略大小写进行比较,以提高用户体验。
user_input = input("Enter a command: ")
if user_input.lower() == "exit":
print("Exiting the program")
else:
print(f"Received command: {user_input}")
2、文件名比较
在文件系统操作中,通常需要忽略文件名的大小写进行比较。
import os
def find_file_ignore_case(directory, filename):
for root, _, files in os.walk(directory):
for file in files:
if file.lower() == filename.lower():
return os.path.join(root, file)
return None
directory = "/path/to/directory"
filename = "example.txt"
file_path = find_file_ignore_case(directory, filename)
if file_path:
print(f"File found: {file_path}")
else:
print("File not found")
通过上述方法,可以在Python中灵活地处理字符串的大小写区分问题。无论是简单的字符串比较,还是复杂的正则表达式匹配,Python都提供了丰富的工具和方法来满足各种需求。
相关问答FAQs:
如何在Python中判断一个字符串是否是全大写或全小写?
在Python中,可以使用字符串的isupper()
和islower()
方法来判断一个字符串是否全为大写或全为小写。调用这些方法时,如果字符串完全由大写字母组成,isupper()
将返回True;如果字符串完全由小写字母组成,islower()
将返回True。例如:
text = "HELLO"
print(text.isupper()) # 输出: True
text = "hello"
print(text.islower()) # 输出: True
在Python中如何进行大小写转换?
Python提供了多种方法来转换字符串的大小写。使用upper()
方法可以将字符串转换为全大写,而使用lower()
方法可以将字符串转换为全小写。如果需要将字符串的每个单词的首字母大写,可以使用title()
方法。例如:
text = "hello world"
print(text.upper()) # 输出: HELLO WORLD
print(text.lower()) # 输出: hello world
print(text.title()) # 输出: Hello World
如何在Python中区分大小写的字符串比较?
在Python中,字符串的比较是区分大小写的。使用==
运算符比较两个字符串时,只有当它们的内容和大小写完全相同,结果才会返回True。例如:
str1 = "Python"
str2 = "python"
print(str1 == str2) # 输出: False
如果希望进行不区分大小写的比较,可以将两个字符串都转换为相同的大小写后再进行比较,例如使用lower()
或upper()
方法。
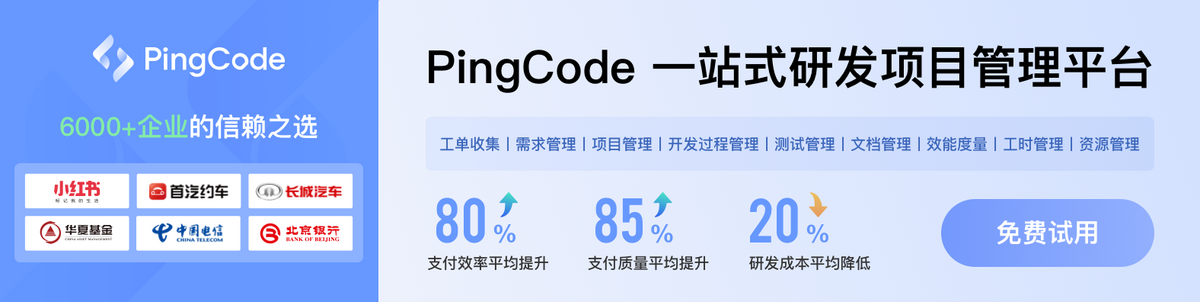