在Python循环中调用Shell的方式有多种,包括使用os.system
、subprocess.run
和subprocess.Popen
等函数。其中,subprocess
模块提供了更灵活和强大的功能,它允许你在Python代码中调用和管理外部程序。下面将详细介绍使用subprocess
模块在Python循环中调用Shell的方法,并提供示例代码。
一、使用subprocess.run
在Python中,subprocess.run
是一个常用的函数,它用于执行外部命令并等待其完成。它是Python 3.5引入的高层接口,推荐使用。subprocess.run
可以捕获命令的输出,并且可以通过参数传递不同的选项,如输入、输出、错误重定向等。
import subprocess
commands = ['echo Hello', 'ls -l', 'pwd']
for command in commands:
result = subprocess.run(command, shell=True, capture_output=True, text=True)
print(f"Command: {command}")
print(f"Return Code: {result.returncode}")
print(f"Output: {result.stdout}")
print(f"Error: {result.stderr}")
在上面的代码中,我们创建了一个包含多个Shell命令的列表,并使用for
循环遍历每个命令。对于每个命令,我们使用subprocess.run
来执行,并且捕获输出和错误信息。通过指定shell=True
,我们可以在Shell中执行命令。capture_output=True
参数用于捕获标准输出和标准错误,text=True
用于将输出作为字符串处理。
二、使用subprocess.Popen
subprocess.Popen
是一个底层接口,提供了更大的灵活性和控制。它允许你异步地与外部进程进行交互,并且可以使用管道重定向输入和输出。
import subprocess
commands = ['echo Hello', 'ls -l', 'pwd']
for command in commands:
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(f"Command: {command}")
print(f"Return Code: {process.returncode}")
print(f"Output: {stdout}")
print(f"Error: {stderr}")
在上面的代码中,我们使用subprocess.Popen
来启动外部进程,并通过communicate
方法与其进行交互。stdout=subprocess.PIPE
和stderr=subprocess.PIPE
参数用于重定向标准输出和标准错误。text=True
用于将输出作为字符串处理。
三、捕获实时输出
有时候,你可能希望在外部命令执行时实时捕获和处理输出。可以通过迭代读取标准输出流来实现这一点。
import subprocess
commands = ['ping -c 4 google.com', 'ping -c 4 yahoo.com']
for command in commands:
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(f"Output: {output.strip()}")
rc = process.poll()
print(f"Return Code: {rc}")
在上面的代码中,我们使用process.stdout.readline
逐行读取标准输出。这种方法适用于长时间运行的命令,可以实时处理输出。
四、传递输入给外部进程
有时候,你可能需要向外部进程传递输入数据。可以通过stdin
参数和communicate
方法来实现。
import subprocess
commands = ['grep python', 'grep shell']
for command in commands:
process = subprocess.Popen(command, shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate(input='python shell subprocess example')
print(f"Command: {command}")
print(f"Return Code: {process.returncode}")
print(f"Output: {stdout}")
print(f"Error: {stderr}")
在上面的代码中,我们使用communicate
方法向外部进程传递输入数据。通过指定stdin=subprocess.PIPE
,我们可以将输入数据传递给外部进程。
五、处理错误和异常
在调用Shell命令时,可能会遇到错误和异常情况。可以通过捕获异常和检查返回码来处理这些情况。
import subprocess
commands = ['echo Hello', 'invalid_command', 'pwd']
for command in commands:
try:
result = subprocess.run(command, shell=True, capture_output=True, text=True, check=True)
print(f"Command: {command}")
print(f"Return Code: {result.returncode}")
print(f"Output: {result.stdout}")
print(f"Error: {result.stderr}")
except subprocess.CalledProcessError as e:
print(f"Command '{e.cmd}' failed with return code {e.returncode}")
print(f"Error Output: {e.stderr}")
在上面的代码中,我们使用try...except
结构来捕获subprocess.CalledProcessError
异常。通过指定check=True
,我们可以在命令返回非零退出码时引发异常。
六、并行执行多个命令
有时候,你可能希望并行执行多个命令。可以使用concurrent.futures
模块来实现这一点。
import subprocess
import concurrent.futures
commands = ['echo Hello', 'sleep 2', 'pwd']
def run_command(command):
result = subprocess.run(command, shell=True, capture_output=True, text=True)
return (command, result.returncode, result.stdout, result.stderr)
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(run_command, cmd) for cmd in commands]
for future in concurrent.futures.as_completed(futures):
command, returncode, stdout, stderr = future.result()
print(f"Command: {command}")
print(f"Return Code: {returncode}")
print(f"Output: {stdout}")
print(f"Error: {stderr}")
在上面的代码中,我们使用ThreadPoolExecutor
来并行执行多个命令。通过提交任务给线程池,我们可以同时执行多个命令并收集结果。
七、总结
在Python循环中调用Shell命令可以通过subprocess
模块实现,推荐使用subprocess.run
和subprocess.Popen
函数。subprocess.run
提供了简单易用的接口,适合大多数场景,而subprocess.Popen
提供了更大的灵活性和控制。通过捕获输出、传递输入、处理错误和并行执行命令,可以满足不同的需求。希望通过本文的介绍,能够帮助你更好地在Python循环中调用Shell命令。
相关问答FAQs:
如何在Python循环中执行Shell命令?
在Python中,可以使用subprocess
模块来执行Shell命令。通过在循环中调用subprocess.run()
或subprocess.Popen()
,可以轻松实现多次执行Shell命令。例如,以下代码展示了如何在循环中调用Shell命令并获取输出:
import subprocess
for i in range(5):
result = subprocess.run(['echo', f'Hello from iteration {i}'], capture_output=True, text=True)
print(result.stdout)
使用Python循环调用Shell命令时,有哪些注意事项?
在调用Shell命令时,确保命令及其参数的正确性,以避免运行错误或产生不必要的安全风险。此外,使用capture_output=True
选项能够捕获命令的输出,便于后续处理。需要谨慎处理来自Shell的输出和错误信息,以确保程序的稳定性。
如何在Python中处理Shell命令的错误?
可以通过检查subprocess.run()
或subprocess.Popen()
的返回码来判断Shell命令是否执行成功。如果返回码不为零,说明命令执行出错。可以使用try-except
语句来捕获异常,并根据需要进行错误处理。例如:
import subprocess
for i in range(3):
try:
result = subprocess.run(['ls', f'directory_{i}'], check=True, capture_output=True, text=True)
print(result.stdout)
except subprocess.CalledProcessError as e:
print(f"Error occurred: {e.stderr}")
这种方法有助于调试和提升代码的健壮性。
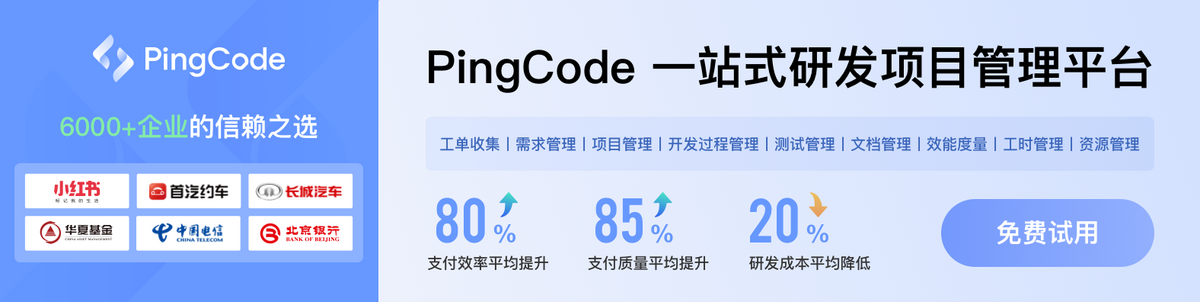