PHP 中的 Interface 在工程项目中是非常有用的。其最大的用处就在于为未来的类切换与拓展保留足够的兼容性这种灵活地兼容性,是一个 PHP项目多年维护后才能体会到的。

一、PHP的interface有什么用处
PHP 中的 Interface 在工程项目中是非常有用的。其最大的用处就在于
为未来的类切换与拓展保留足够的兼容性
这种灵活地兼容性,是一个 PHP项目多年维护后才能体会到的。
这里我以 Laravel 框架的工程项目举例说明。
比如我们有一个数据表,名为 products ,里面存储了许多商品的信息。
对于新手来说,要对这个类进行操作,一般的做法就是建立一个 控制器(Controller),将对于该表的 “新改读删(CURD)”都放在其中。这是官方教程中的方法
<?php
namespace App\Http\Controller;
…
class ProductController extends Controller
{
public function index(){ … };
public function create(Request $request){ … };
….
}
?>
这样并不错,但未来要做修改的时候,每次都要修改这个类,而有的操作其实是反复写的,无法复用。
所以在工程项目中,一般都不会按照 官方教程这么写,这里就需要用 interface 了。
我们先建立一个针对 Product 的服务操作接口
<?php
namespace App\Repositories\Product;
interface ProductRepository{
public function create(array $data);
public function find($id=null);
public function delete($id=null);
….
}
?>
这里定义了所需的功能函数,再实现一个对应的类
<?php
namespace App\Repositories\Product;
use App\Models\Product;
class ProductEloquent implememts ProductReposity
{
public function create(array $data){ … };
public function find($id=null){ … };
public function delete($id=null){ … };
….
}
?>
将原有的 Controller 类拆分到两个文件中,我们就可以完成如下工作:
- 以后该项目中所有与 Product 相关的操作,都不再关联到 ProductEloquent 类,而关联到 ProductReposity 接口上。
<?php
/** use App\Repositories\ProductEloquent; **/
use App\Repositories\ProductReposity;
?>
- 再利用 Laravel 的服务注册,将接口与实际类关联起来。这个功能在 AppServiceProviders.php 中增加如下的代码。
<?php
namespace App\Providers;
use App\Repositories\ProductRepository;
use App\Repositories\ProductEloquent;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->singleton(ProductRepository::class, ProductEloquent::class);
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
//
}
}
延伸阅读:
二、PHP接口(interface)的特点
1、接口的方法必须是公开的。
2、接口的方法默认是抽象的,所以不在方法名前面加abstract。
3、接口可以定义常量,但不能定义成员属性,常量的定义和用法和类中常量一样。
4、类可以实现多个接口(相当于把多个功能集于一身,如手机实现了小灵通、MP3、MP4的功能)
5、接口也可以继承接口。
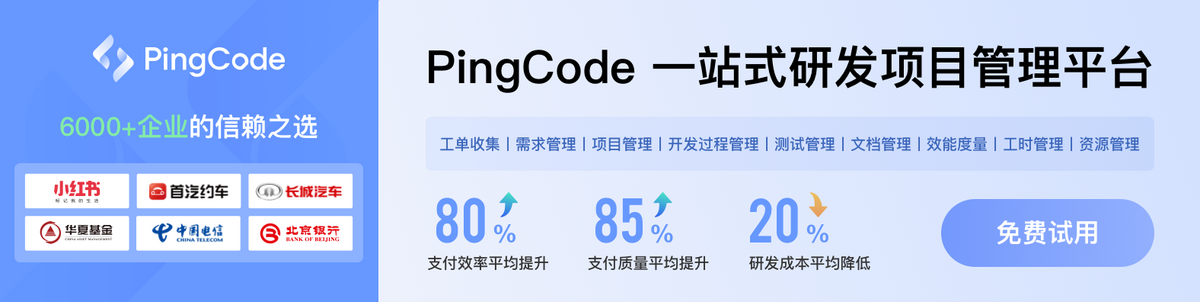