在Python中遍历一个集合及其下标可以通过使用内置函数enumerate()
实现、这个函数会返回每个元素的下标和值。例如,你可以这样使用它:
for index, value in enumerate(your_collection):
# 在这里处理下标 (index) 和值 (value)
enumerate()
函数为集合中的每个元素提供了两个信息:元素的索引和对应的值,这让我们在需要同时访问它们的情况下变得非常方便。
一、使用enumerate()
函数
二、遍历列表
在Python中,列表是最常见的集合数据类型之一。我们通常需要遍历列表中的每个元素,为了同时获得元素的索引,我们可以使用enumerate()
函数。
my_list = ["apple", "banana", "cherry"]
for index, fruit in enumerate(my_list):
print(f"Index {index}: {fruit}")
三、遍历字典
字典在Python中用于存储键值对。尽管enumerate()
通常用于序列类型,如列表或元组,但也可以在将字典转换为可迭代的项序列时使用。
my_dict = {'a': 1, 'b': 2, 'c': 3}
for index, (key, value) in enumerate(my_dict.items()):
print(f"Index {index}: Key {key}, Value {value}")
四、遍历字符串
字符串也可以视为字符的集合,使用enumerate()
可以在迭代字符串时获得字符位置。
my_string = "Hello"
for index, char in enumerate(my_string):
print(f"Index {index}: Character {char}")
五、遍历自定义对象
如果你有一个自定义的集合对象,你需要确保它是可迭代的。一旦你的对象实现了迭代协议,你就可以使用enumerate()
。
class MyCollection:
def __init__(self, elements):
self.elements = elements
def __iter__(self):
return iter(self.elements)
my_collection = MyCollection(["a", "b", "c"])
for index, item in enumerate(my_collection):
print(f"Index {index}: Item {item}")
六、高级用途
enumerate()
函数还可接受一个可选的参数来指定起始索引。这在某些情境下很有用,比如,从非零基索引的集合开始。
my_list = ['item1', 'item2', 'item3']
for index, item in enumerate(my_list, start=1):
print(f"Position {index}: {item}")
七、遍历多个集合
有时你可能需要同时遍历多个集合。在这种情况下,你可以使用zip()
与enumerate()
结合实现。
list1 = ["a", "b", "c"]
list2 = [10, 20, 30]
for index, (item1, item2) in enumerate(zip(list1, list2)):
print(f"Index {index}: Item1 {item1}, Item2 {item2}")
以上介绍的方法,无论是在数据处理、机器学习数据集的管理、Web开发中处理表单数据还是其他任意需要索引信息的场景中,都是非常实用的技巧。掌握这些技术能够大大提高Python编程的灵活性和效率。
相关问答FAQs:
1. 如何在 Python 中遍历集合元素并获取下标?
遍历一个集合并获取每个元素的下标可以使用 enumerate()
函数。这个函数会返回一个迭代器对象,包含每个元素的索引和对应的值。你可以用一个 for 循环来遍历这个迭代器,并通过解包操作将索引和值分别赋给两个变量。
示例代码:
my_list = ['apple', 'banana', 'orange']
for index, value in enumerate(my_list):
print(f"The element at index {index} is {value}.")
输出结果:
The element at index 0 is apple.
The element at index 1 is banana.
The element at index 2 is orange.
2. 在 Python 中怎样遍历一个集合并同时获取元素下标和值的对应关系?
如果你需要同时获取元素下标和对应的值,并将它们映射到一个字典或其他数据结构中,你可以使用字典推导式(dict comprehension)来实现。
示例代码:
my_list = ['apple', 'banana', 'orange']
indexed_dict = {index: value for index, value in enumerate(my_list)}
print(indexed_dict)
输出结果:
{0: 'apple', 1: 'banana', 2: 'orange'}
3. 我如何在 Python 中遍历一个集合的元素,但跳过某个特定下标?
如果你想在遍历集合时跳过某个特定的下标,你可以使用 continue
语句来实现。 continue
语句会跳过当前迭代的剩余代码,直接进入下一次迭代。
示例代码:
my_list = ['apple', 'banana', 'orange', 'mango']
skip_index = 1
for index, value in enumerate(my_list):
if index == skip_index:
continue
print(f"The element at index {index} is {value}.")
输出结果:
The element at index 0 is apple.
The element at index 2 is orange.
The element at index 3 is mango.
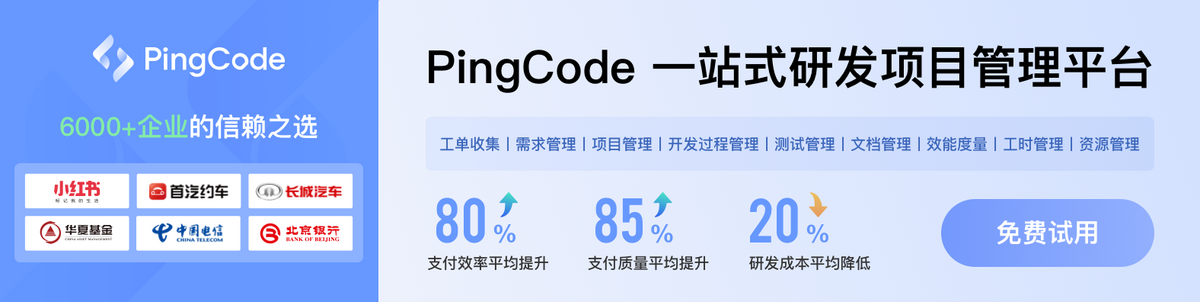