数字月份的英文名称可以通过数组和索引方式输出。在C语言中,使用一个字符串数组来存储每个月份的英文名称是最直观的做法。借助数组索引、条件判断语句、以及输入输出函数,就可以实现这一要求。
首先,声明并初始化一个包含所有月份名称的字符串数组。然后,从用户那里获取数字月份,通过数组索引的方式检索对应的月份英文名称,并输出。需要注意的是,月份索引应该减1,因为数组索引从0开始,而月份从1开始。
一、数组和索引的使用
数组的声明和初始化
首先声明一个类型为char
的二维数组,每个元素是一个指向char
类型的指针,用于存储英文月份名称的字符串:
char *months[] = {
"January", "February", "March",
"April", "May", "June",
"July", "August", "September",
"October", "November", "December"
};
输入月份并输出对应的英文名称
接下来,要求用户输入数字月份,然后根据用户提供的月份输出对应的英文名称:
#include <stdio.h>
int mAIn() {
int month_number;
printf("Please enter the month number (1-12): ");
scanf("%d", &month_number);
// 数组索引检查
if(month_number >= 1 && month_number <= 12) {
// 输出对应的月份名称
printf("The English name of month number %d is %s.\n", month_number, months[month_number - 1]);
} else {
printf("Invalid month number. Please enter a number between 1 and 12.\n");
}
return 0;
}
二、函数的应用
除了直接在main
函数中实现上述功能,还可以将其封装成一个单独的函数,提高代码的复用性和可维护性。
声明和定义月份名称函数
创建一个名为getMonthName
的函数,它接受数字月份作为参数,并返回对应的英文名称。
const char* getMonthName(int month_number) {
const char *months[] = {
"January", "February", "March",
"April", "May", "June",
"July", "August", "September",
"October", "November", "December"
};
// 检查月份的有效性
if(month_number >= 1 && month_number <= 12) {
return months[month_number - 1];
} else {
return NULL;
}
}
使用函数获取月份名称
函数被定义后,可以在main
函数中调用它来获取月份名称:
int main() {
int month_number;
printf("Please enter the month number (1-12): ");
scanf("%d", &month_number);
const char *month_name = getMonthName(month_number);
if(month_name) {
printf("The English name of month number %d is %s.\n", month_number, month_name);
} else {
printf("Invalid month number. Please enter a number between 1 and 12.\n");
}
return 0;
}
三、错误处理与用户输入验证
在用户输入数字月份后,程序应该验证输入是否有效,同时提供错误处理机制。
用户输入的验证
程序应检查输入的月份是否在1到12之间。如果输入无效,向用户提供明确的错误消息:
if(month_number < 1 || month_number > 12) {
printf("Invalid month number. Please enter a number between 1 and 12.\n");
return 1; // 使用非0值表示程序遇到错误
}
错误处理
若用户输入的月份不在期望的范围内,程序应该告知用户并可能提供重新输入的机会。
四、代码整合与测试
将所有内容整合到一个完整的程序中,并通过测试确保其正确性和鲁棒性。
完整代码示例
#include <stdio.h>
const char* getMonthName(int month_number) {
const char *months[] = {
"January", "February", "March",
"April", "May", "June",
"July", "August", "September",
"October", "November", "December"
};
if(month_number >= 1 && month_number <= 12) {
return months[month_number - 1];
} else {
return NULL;
}
}
int main() {
int month_number;
printf("Please enter the month number (1-12): ");
scanf("%d", &month_number);
const char *month_name = getMonthName(month_number);
if(month_name) {
printf("The English name of month number %d is %s.\n", month_number, month_name);
} else {
printf("Invalid month number. Please enter a number between 1 and 12.\n");
}
return 0;
}
程序测试
对程序进行一系列测试,包括边界条件(例如输入为1和12的情况)和无效输入(例如0或13)。确保程序能够在各种情况下正常工作,并提供准确的输出或适当的错误消息。
相关问答FAQs:
C语言如何将数字月份转换为英文名称?
-
如何使用C语言编写一个函数来将数字月份转换为英文名称?
你可以使用C语言中的switch语句来编写一个函数,该函数将一个代表月份的数字作为输入,然后根据这个数字返回对应的英文月份名称。#include <stdio.h> void printMonthName(int month) { switch (month) { case 1: printf("January"); break; case 2: printf("February"); break; case 3: printf("March"); break; case 4: printf("April"); break; case 5: printf("May"); break; case 6: printf("June"); break; case 7: printf("July"); break; case 8: printf("August"); break; case 9: printf("September"); break; case 10: printf("October"); break; case 11: printf("November"); break; case 12: printf("December"); break; default: printf("Invalid month"); break; } } int main() { int month; printf("Enter the month number (1-12): "); scanf("%d", &month); printMonthName(month); return 0; }
-
有没有其他的方法可以将数字月份转换为英文名称,而不使用switch语句?
是的,你可以使用一个数组来存储英文月份名称,然后根据输入的数字索引访问数组元素来获取对应的英文月份名称。#include <stdio.h> void printMonthName(int month) { char *monthNames[] = {"Invalid month", "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; printf("%s", monthNames[month]); } int main() { int month; printf("Enter the month number (1-12): "); scanf("%d", &month); printMonthName(month); return 0; }
-
如何设置C语言函数,使其能够处理无效的月份输入?
如果使用switch语句的方法,可以在默认case中处理无效的月份输入,打印一个错误消息告知用户输入无效。如果使用数组的方法,可以使用一个条件语句来检查输入月份是否在有效范围内,如果无效则打印一个错误消息。这样可以提高程序的鲁棒性,避免处理无效输入时出现意外的结果。#include <stdio.h> void printMonthName(int month) { char *monthNames[] = {"Invalid month", "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; if (month >= 1 && month <= 12) { printf("%s", monthNames[month]); } else { printf("Invalid month"); } } int main() { int month; printf("Enter the month number (1-12): "); scanf("%d", &month); printMonthName(month); return 0; }
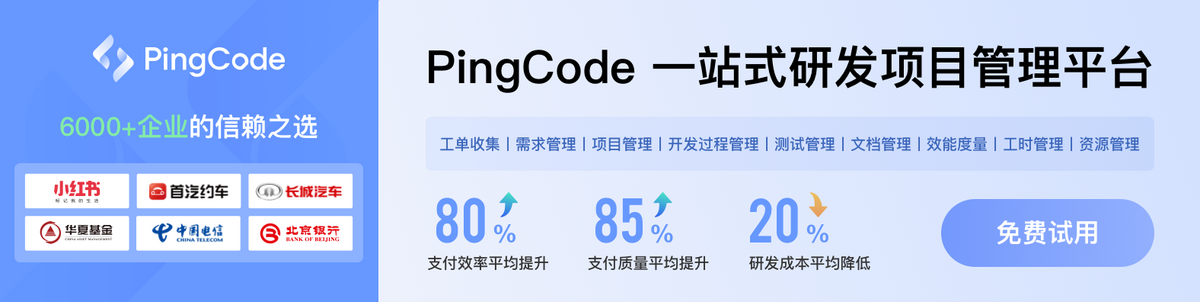