在Python中实现加锁的方法有多种,常见的有:使用threading
模块中的Lock
对象、使用RLock
来支持递归锁定、以及使用上下文管理器来自动获取和释放锁。在多线程编程中,加锁是为了确保某些代码块在任意时刻只能由一个线程执行,从而避免数据竞争和不一致的问题。下面我们将详细介绍这几种方法。
一、THREADING模块中的LOCK对象
threading.Lock
是Python中最基本的锁对象,通常用于控制对共享资源的访问。它提供了简单的锁定和解锁机制。
- 使用
threading.Lock
的基本方法
在多线程编程中,锁的使用可以防止多个线程同时访问共享数据,从而避免出现数据竞争问题。下面是使用threading.Lock
的基本步骤:
import threading
创建一个锁对象
lock = threading.Lock()
def critical_section():
# 获取锁
lock.acquire()
try:
# 进行需要加锁的操作
print("This is a critical section.")
finally:
# 释放锁
lock.release()
创建多个线程
threads = [threading.Thread(target=critical_section) for _ in range(5)]
启动线程
for t in threads:
t.start()
等待所有线程完成
for t in threads:
t.join()
在上面的例子中,acquire()
方法用于获取锁,而release()
方法用于释放锁。在执行完需要同步的代码后,务必确保锁被释放。
- 使用上下文管理器自动处理锁
为了简化锁的使用,Python的threading.Lock
支持上下文管理协议,可以使用with
语句自动获取和释放锁:
import threading
lock = threading.Lock()
def critical_section():
with lock:
print("This is a critical section.")
threads = [threading.Thread(target=critical_section) for _ in range(5)]
for t in threads:
t.start()
for t in threads:
t.join()
使用with
语句可以自动处理锁的获取和释放,这样可以避免忘记释放锁的问题,提高代码的可读性和可靠性。
二、RLOCK用于递归锁定
threading.RLock
是一个递归锁,它允许同一线程多次获取锁而不会引发死锁。递归锁特别适用于某个线程需要在持有锁的情况下再次获取锁的场景。
- RLock的使用场景
在某些情况下,一个线程可能需要在持有锁的同时再次调用需要加锁的函数,这时就需要使用RLock
:
import threading
rlock = threading.RLock()
def recursive_function(level):
with rlock:
print(f"Entering level {level}")
if level > 0:
recursive_function(level - 1)
print(f"Exiting level {level}")
thread = threading.Thread(target=recursive_function, args=(3,))
thread.start()
thread.join()
在这个例子中,recursive_function
在持有锁的情况下递归调用自身,使用RLock
可以避免死锁。
- RLock与Lock的区别
RLock
允许同一线程多次获取锁,而Lock
不允许。如果一个线程在持有Lock
的情况下再次尝试获取锁,会导致死锁。RLock
在每次成功获取锁时计数加一,释放锁时计数减一,只有当计数为零时,锁才真正被释放。
三、SEMAPHORE用于限制并发访问
threading.Semaphore
是另一种同步原语,它允许在多个线程之间共享一个资源池。例如,可以使用信号量来限制同时访问某个资源的线程数量。
- Semaphore的基本用法
信号量的使用可以控制并发线程的数量,以下是一个简单的例子:
import threading
import time
创建一个信号量对象,最多允许两个线程同时访问
semaphore = threading.Semaphore(2)
def worker():
with semaphore:
print(f"Thread {threading.current_thread().name} is working...")
time.sleep(1)
threads = [threading.Thread(target=worker) for _ in range(5)]
for t in threads:
t.start()
for t in threads:
t.join()
在这个例子中,最多有两个线程可以同时执行worker
函数。
- Semaphore与Lock的区别
Semaphore
与Lock
的主要区别在于,Lock
是二元的(0或1),而Semaphore
是多元的,可以允许多个线程同时访问。Lock
通常用于确保只有一个线程访问资源,而Semaphore
用于限制并发线程数。
四、CONDITION用于复杂的线程同步
threading.Condition
是一个更复杂的同步原语,允许线程等待某个条件发生变化。它通常与Lock
或RLock
一起使用。
- Condition的基本用法
Condition
对象提供了一种线程间通信的机制,可以用于实现复杂的同步模式:
import threading
import time
condition = threading.Condition()
def consumer():
with condition:
print("Consumer is waiting.")
condition.wait()
print("Consumer is done waiting.")
def producer():
with condition:
print("Producer is producing.")
time.sleep(2)
print("Producer is done producing.")
condition.notify()
consumer_thread = threading.Thread(target=consumer)
producer_thread = threading.Thread(target=producer)
consumer_thread.start()
time.sleep(1)
producer_thread.start()
consumer_thread.join()
producer_thread.join()
在这个例子中,消费者线程会等待直到生产者线程调用notify()
方法。
- Condition的高级用法
Condition
可以实现更复杂的同步机制,如生产者-消费者模型、读写锁等。在使用Condition
时,要确保使用正确的锁来保护共享资源,并正确处理条件的等待和通知。
五、其他加锁策略
除了上述常见的加锁方式,Python还有其他用于线程同步的工具,例如Event
、Barrier
等。这些工具用于特定场景的同步需求,可以根据实际需要选择合适的工具。
- Event
threading.Event
可以用于线程之间的简单通信。一个线程可以等待事件的发生,而另一个线程可以设置事件:
import threading
import time
event = threading.Event()
def wait_for_event():
print("Waiting for event to be set.")
event.wait()
print("Event has been set.")
def set_event():
time.sleep(2)
print("Setting event.")
event.set()
waiter = threading.Thread(target=wait_for_event)
setter = threading.Thread(target=set_event)
waiter.start()
setter.start()
waiter.join()
setter.join()
- Barrier
threading.Barrier
用于阻塞一组线程,直到指定数量的线程都达到一个点:
import threading
barrier = threading.Barrier(3)
def worker():
print(f"Thread {threading.current_thread().name} is waiting at the barrier.")
barrier.wait()
print(f"Thread {threading.current_thread().name} has passed the barrier.")
threads = [threading.Thread(target=worker) for _ in range(3)]
for t in threads:
t.start()
for t in threads:
t.join()
使用这些同步工具,Python开发者可以有效地管理多线程程序中的并发和同步问题,提高程序的稳定性和性能。选择合适的同步工具可以根据具体的应用场景和需求来决定。
相关问答FAQs:
Python 中加锁的目的是什么?
加锁在 Python 中主要用于多线程或多进程编程,以防止多个线程或进程同时访问共享资源,从而避免数据不一致或冲突的问题。通过加锁,可以确保同一时间只有一个线程或进程能够访问被保护的代码块或数据,从而提高程序的稳定性和可靠性。
在 Python 中使用哪些库可以实现加锁?
Python 提供了多种方式来实现加锁。最常用的库是 threading
,其中的 Lock
和 RLock
类可以轻松创建和管理锁。此外,对于更高级的场景,可以使用 multiprocessing
库中的 Lock
类,适用于多进程环境。还有 asyncio
库中的 Lock
,用于异步编程场景。
在使用加锁时需要注意哪些问题?
使用加锁时,要注意避免死锁的发生。死锁是指两个或多个线程在等待对方释放锁,导致程序无法继续执行。为了避免死锁,可以采取一些措施,例如确保锁的获取顺序一致,或者设定超时机制。此外,尽量减少锁的持有时间,避免长时间占用锁,这样能够提高程序的并发性能。
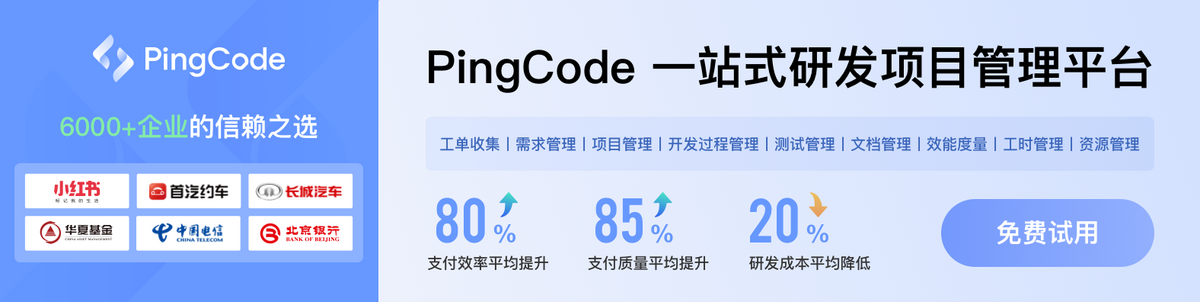